Cohere Chat
提供 Bedrock Cohere Chat 客户端。将生成式 AI 功能集成到核心应用程序和提高业务成果的工作流中。 AWS Bedrock Cohere Model Page和 Amazon Bedrock User Guide包含有关如何使用 AWS 托管模型的详细信息。
Prerequisites
请参阅 Spring AI documentation on Amazon Bedrock 以设置 API 访问。
Add Repositories and BOM
Spring AI 工件发布在 Spring Milestone 和 Snapshot 存储库中。有关将这些存储库添加到你的构建系统的说明,请参阅 Repositories 部分。
为了帮助进行依赖项管理,Spring AI 提供了一个 BOM(物料清单)以确保在整个项目中使用一致版本的 Spring AI。有关将 Spring AI BOM 添加到你的构建系统的说明,请参阅 Dependency Management 部分。
Auto-configuration
将 spring-ai-bedrock-ai-spring-boot-starter
依赖项添加到项目 Maven 的 pom.xml
文件:
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-bedrock-ai-spring-boot-starter</artifactId>
</dependency>
或添加到 Gradle build.gradle
构建文件中。
dependencies {
implementation 'org.springframework.ai:spring-ai-bedrock-ai-spring-boot-starter'
}
|
Enable Cohere Chat Support
默认情况下,Cohere 模型被禁用。若要启用它,请将 spring.ai.bedrock.cohere.chat.enabled
属性设置为 true
。导出环境变量是一种设置此配置属性的方法:
export SPRING_AI_BEDROCK_COHERE_CHAT_ENABLED=true
Chat Properties
spring.ai.bedrock.aws
前缀是配置与 AWS Bedrock 的连接的属性前缀。
Property | Description | Default |
---|---|---|
spring.ai.bedrock.aws.region |
AWS region to use. |
us-east-1 |
spring.ai.bedrock.aws.access-key |
AWS access key. |
- |
spring.ai.bedrock.aws.secret-key |
AWS secret key. |
- |
spring.ai.bedrock.cohere.chat
前缀是配置 Cohere 的聊天客户端实现的属性前缀。
Property | Description | Default |
---|---|---|
spring.ai.bedrock.cohere.chat.enabled |
启用或禁用对 Cohere 的支持 |
false |
spring.ai.bedrock.cohere.chat.model |
要使用的模型 ID。请参阅 CohereChatModel以了解支持的模型。 |
cohere.command-text-v14 |
spring.ai.bedrock.cohere.chat.options.temperature |
控制输出的随机性。值可介于 [0.0,1.0]。 |
0.7 |
spring.ai.bedrock.cohere.chat.options.topP |
采样时要考虑的标记的最大累积概率。 |
AWS Bedrock default |
spring.ai.bedrock.cohere.chat.options.topK |
指定模型用于生成下一个标记的标记选择数 |
AWS Bedrock default |
spring.ai.bedrock.cohere.chat.options.maxTokens |
指定在生成响应中要使用的最大标记数。 |
AWS Bedrock default |
spring.ai.bedrock.cohere.chat.options.stopSequences |
配置模型识别的多达四个序列。 |
AWS Bedrock default |
spring.ai.bedrock.cohere.chat.options.returnLikelihoods |
标记似然性将随响应一起返回。 |
AWS Bedrock default |
spring.ai.bedrock.cohere.chat.options.numGenerations |
模型应该返回的最大生成数量。 |
AWS Bedrock default |
spring.ai.bedrock.cohere.chat.options.logitBias |
防止模型生成不需要的标记或激励模型包括所需的标记。 |
AWS Bedrock default |
spring.ai.bedrock.cohere.chat.options.truncate |
指定 API 如何处理长度超过最大标记长度的输入 |
AWS Bedrock default |
查看 CohereChatModel以了解其他模型 ID。支持的值包括:cohere.command-light-text-v14`和 `cohere.command-text-v14
。还可以从 AWS Bedrock documentation for base model IDs中找到模型 ID 值。
所有以 |
Chat Options
BedrockCohereChatOptions.java提供模型配置,例如 temperature、topK、topP 等。
启动时,可以使用 BedrockCohereChatClient(api, options)
构造函数或 spring.ai.bedrock.cohere.chat.options.*
属性配置默认选项。
在运行时,你可以通过向 Prompt
调用添加新的请求特定选项来覆盖默认选项。例如,覆盖特定请求的默认温度:
ChatResponse response = chatClient.call(
new Prompt(
"Generate the names of 5 famous pirates.",
BedrockCohereChatOptions.builder()
.withTemperature(0.4)
.build()
));
|
Sample Controller (Auto-configuration)
Create一个新的 Spring Boot 项目,并将 `spring-ai-bedrock-ai-spring-boot-starter`添加到您的 pom(或 gradle)依赖项。
在 src/main/resources
目录下添加一个 application.properties
文件,以启用和配置 Cohere Chat 客户端:
spring.ai.bedrock.aws.region=eu-central-1
spring.ai.bedrock.aws.access-key=${AWS_ACCESS_KEY_ID}
spring.ai.bedrock.aws.secret-key=${AWS_SECRET_ACCESS_KEY}
spring.ai.bedrock.cohere.chat.enabled=true
spring.ai.bedrock.cohere.chat.options.temperature=0.8
将 |
这将创建一个你可以注入到你的类中的 BedrockCohereChatClient
实现。下面是一个简单的 @Controller
类的示例,它使用聊天客户端来生成文本。
@RestController
public class ChatController {
private final BedrockCohereChatClient chatClient;
@Autowired
public ChatController(BedrockCohereChatClient chatClient) {
this.chatClient = chatClient;
}
@GetMapping("/ai/generate")
public Map generate(@RequestParam(value = "message", defaultValue = "Tell me a joke") String message) {
return Map.of("generation", chatClient.call(message));
}
@GetMapping("/ai/generateStream")
public Flux<ChatResponse> generateStream(@RequestParam(value = "message", defaultValue = "Tell me a joke") String message) {
Prompt prompt = new Prompt(new UserMessage(message));
return chatClient.stream(prompt);
}
}
Manual Configuration
BedrockCohereChatClient实现 ChatClient`和 `StreamingChatClient
,并使用 Low-level CohereChatBedrockApi Client连接到 Bedrock Cohere 服务。
将 spring-ai-bedrock
依赖项添加到项目的 Maven pom.xml
文件:
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-bedrock</artifactId>
</dependency>
或添加到 Gradle build.gradle
构建文件中。
dependencies {
implementation 'org.springframework.ai:spring-ai-bedrock'
}
|
接下来,创建一个 BedrockCohereChatClient并使用它进行文本生成:
CohereChatBedrockApi api = new CohereChatBedrockApi(CohereChatModel.COHERE_COMMAND_V14.id(),
EnvironmentVariableCredentialsProvider.create(), Region.US_EAST_1.id(), new ObjectMapper());
BedrockCohereChatClient chatClient = new BedrockCohereChatClient(api,
BedrockCohereChatOptions.builder()
.withTemperature(0.6f)
.withTopK(10)
.withTopP(0.5f)
.withMaxTokens(678)
.build()
ChatResponse response = chatClient.call(
new Prompt("Generate the names of 5 famous pirates."));
// Or with streaming responses
Flux<ChatResponse> response = chatClient.stream(
new Prompt("Generate the names of 5 famous pirates."));
Low-level CohereChatBedrockApi Client
CohereChatBedrockApi提供轻量级 Java 客户端,基于 AWS Bedrock Cohere Command models。
以下类图说明了 CohereChatBedrockApi 接口及其构建模块:
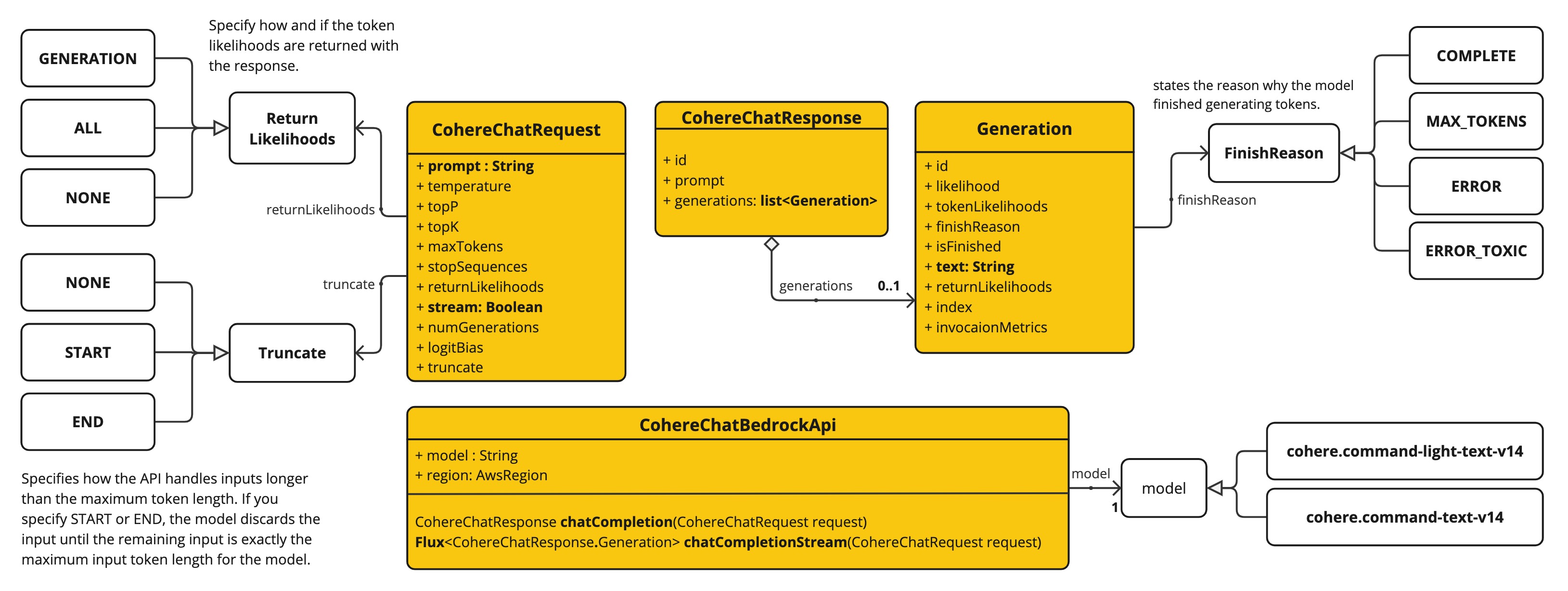
CohereChatBedrockApi 支持 cohere.command-light-text-v14
和 cohere.command-text-v14
模型,用于同步(例如 chatCompletion()
)和流式(例如 chatCompletionStream()
)请求。
下面是一个简单的片段,说明如何以编程方式使用 API:
CohereChatBedrockApi cohereChatApi = new CohereChatBedrockApi(
CohereChatModel.COHERE_COMMAND_V14.id(),
Region.US_EAST_1.id());
var request = CohereChatRequest
.builder("What is the capital of Bulgaria and what is the size? What it the national anthem?")
.withStream(false)
.withTemperature(0.5f)
.withTopP(0.8f)
.withTopK(15)
.withMaxTokens(100)
.withStopSequences(List.of("END"))
.withReturnLikelihoods(CohereChatRequest.ReturnLikelihoods.ALL)
.withNumGenerations(3)
.withLogitBias(null)
.withTruncate(Truncate.NONE)
.build();
CohereChatResponse response = cohereChatApi.chatCompletion(request);
var request = CohereChatRequest
.builder("What is the capital of Bulgaria and what is the size? What it the national anthem?")
.withStream(true)
.withTemperature(0.5f)
.withTopP(0.8f)
.withTopK(15)
.withMaxTokens(100)
.withStopSequences(List.of("END"))
.withReturnLikelihoods(CohereChatRequest.ReturnLikelihoods.ALL)
.withNumGenerations(3)
.withLogitBias(null)
.withTruncate(Truncate.NONE)
.build();
Flux<CohereChatResponse.Generation> responseStream = cohereChatApi.chatCompletionStream(request);
List<CohereChatResponse.Generation> responses = responseStream.collectList().block();