Android 简明教程
Android - Push Notification
通知是一条您可以显示给用户且位于您的应用程序的常规 UI 之外的消息。您可以在 Android 中非常容易地创建自己的通知。
A notification is a message you can display to the user outside of your application’s normal UI. You can create your own notifications in android very easily.
为此目的,Android 提供 NotificationManager 类。如需使用此类,您需要通过 getSystemService() method 向 Android 请求此类的对象。其语法如下 −
Android provides NotificationManager class for this purpose. In order to use this class, you need to instantiate an object of this class by requesting the android system through getSystemService() method. Its syntax is given below −
NotificationManager NM;
NM=(NotificationManager)getSystemService(Context.NOTIFICATION_SERVICE);
之后,您将通过 Notification 类创建通知,并指定其属性,例如图标、标题和时间等。其语法如下 −
After that you will create Notification through Notification class and specify its attributes such as icon,title and time e.t.c. Its syntax is given below −
Notification notify = new Notification(android.R.drawable.stat_notify_more,title,System.currentTimeMillis());
您需要做的下一件事是通过将上下文和意图作为参数传递来创建一个 PendingIntent 。通过向另一个应用程序提供 PendingIntent,您授予它执行您指定的操作的权利,就好像另一个应用程序就是您自己一样。
The next thing you need to do is to create a PendingIntent by passing context and intent as a parameter. By giving a PendingIntent to another application, you are granting it the right to perform the operation you have specified as if the other application was yourself.
PendingIntent pending = PendingIntent.getActivity(getApplicationContext(), 0, new Intent(),0);
您需要做的最后一件事是调用 Notification 类的 setLatestEventInfo 方法并传入 PendingIntent,以及通知主体和正文详细信息。其语法如下。最后再调用 NotificationManager 类的通知方法。
The last thing you need to do is to call setLatestEventInfo method of the Notification class and pass the pending intent along with notification subject and body details. Its syntax is given below. And then finally call the notify method of the NotificationManager class.
notify.setLatestEventInfo(getApplicationContext(), subject, body,pending);
NM.notify(0, notify);
除了通知方法外,NotificationManager 类中还有其他可用的方法。如下所列 −
Apart from the notify method, there are other methods available in the NotificationManager class. They are listed below −
Sr.No |
Method & description |
1 |
cancel(int id) This method cancel a previously shown notification. |
2 |
cancel(String tag, int id) This method also cancel a previously shown notification. |
3 |
cancelAll() This method cancel all previously shown notifications. |
4 |
notify(int id, Notification notification) This method post a notification to be shown in the status bar. |
5 |
notify(String tag, int id, Notification notification) This method also Post a notification to be shown in the status bar. |
Example
下面的示例演示了 NotificationManager 类的使用。它创建了一个允许创建通知的基本应用程序。
The below example demonstrates the use of NotificationManager class. It crates a basic application that allows you to create a notification.
要尝试本示例,您需要在实际设备或模拟器上运行它。
To experiment with this example, you need to run this on an actual device or in an emulator.
Steps |
Description |
1 |
You will use Android studio to create an Android application under a packagecom.example.sairamkrishna.myapplication. |
2 |
Modify src/MainActivity.java file to add Notification code. |
3 |
Modify layout XML file res/layout/activity_main.xml add any GUI component if required. |
4 |
Run the application and choose a running android device and install the application on it and verify the results. |
以下是 MainActivity.java 的内容。
Here is the content of MainActivity.java.
package com.example.sairamkrishna.myapplication;
import android.app.Notification;
import android.app.NotificationManager;
import android.content.Context;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends ActionBarActivity {
EditText ed1,ed2,ed3;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ed1=(EditText)findViewById(R.id.editText);
ed2=(EditText)findViewById(R.id.editText2);
ed3=(EditText)findViewById(R.id.editText3);
Button b1=(Button)findViewById(R.id.button);
b1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String tittle=ed1.getText().toString().trim();
String subject=ed2.getText().toString().trim();
String body=ed3.getText().toString().trim();
NotificationManager notif=(NotificationManager)getSystemService(Context.NOTIFICATION_SERVICE);
Notification notify=new Notification.Builder
(getApplicationContext()).setContentTitle(tittle).setContentText(body).
setContentTitle(subject).setSmallIcon(R.drawable.abc).build();
notify.flags |= Notification.FLAG_AUTO_CANCEL;
notif.notify(0, notify);
}
});
}
}
以下是 activity_main.xml 的内容。
Here is the content of activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Notification"
android:id="@+id/textView"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:textSize="30dp" />
.
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tutorials Point"
android:id="@+id/textView2"
android:layout_below="@+id/textView"
android:layout_centerHorizontal="true"
android:textSize="35dp"
android:textColor="#ff16ff01" />
<EditText
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/editText"
android:layout_below="@+id/textView2"
android:layout_alignLeft="@+id/textView2"
android:layout_alignStart="@+id/textView2"
android:layout_marginTop="52dp"
android:layout_alignRight="@+id/textView2"
android:layout_alignEnd="@+id/textView2"
android:hint="Name" />
<EditText
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/editText2"
android:hint="Subject"
android:layout_below="@+id/editText"
android:layout_alignLeft="@+id/editText"
android:layout_alignStart="@+id/editText"
android:layout_alignRight="@+id/editText"
android:layout_alignEnd="@+id/editText" />
<EditText
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:inputType="textPersonName"
android:ems="10"
android:id="@+id/editText3"
android:hint="Body"
android:layout_below="@+id/editText2"
android:layout_alignLeft="@+id/editText2"
android:layout_alignStart="@+id/editText2"
android:layout_alignRight="@+id/editText2"
android:layout_alignEnd="@+id/editText2" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Notification"
android:id="@+id/button"
android:layout_marginTop="77dp"
android:layout_below="@+id/editText3"
android:layout_alignRight="@+id/textView"
android:layout_alignEnd="@+id/textView" />
</RelativeLayout>
以下是 AndroidManifest.xml 的内容。
Here is the content of AndroidManifest.xml.
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.sairamkrishna.myapplication" >
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
让我们尝试运行我们的应用程序。要从 Android Studio 运行该应用程序,请打开项目中的一个活动文件,并单击工具栏中的运行图标。在启动应用程序前,Android Studio 将显示以下窗口,供您选择运行 Android 应用程序的位置。
Let’s try to run our application. To run the app from Android studio, open one of your project’s activity files and click Run icon from the tool bar. Before starting your application, Android studio will display following window to select an option where you want to run your Android application.

现在填写标题、主题和正文字段。如下所示 −
Now fill in the field with the title , subject and the body. This has been shown below in the figure −
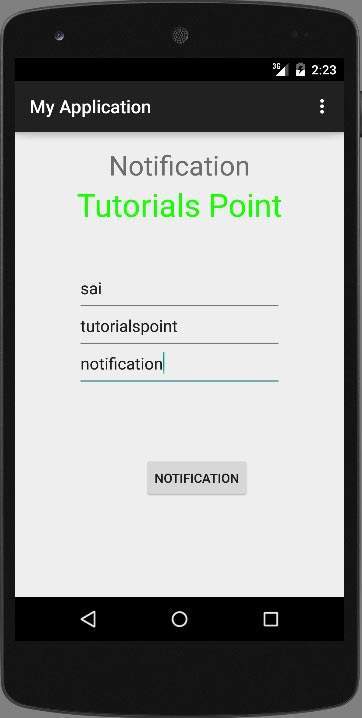
现在点击通知按钮,您将在顶部通知栏中看到一条通知。已如下所示 −
Now click on the notify button and you will see a notification in the top notification bar. It has been shown below −
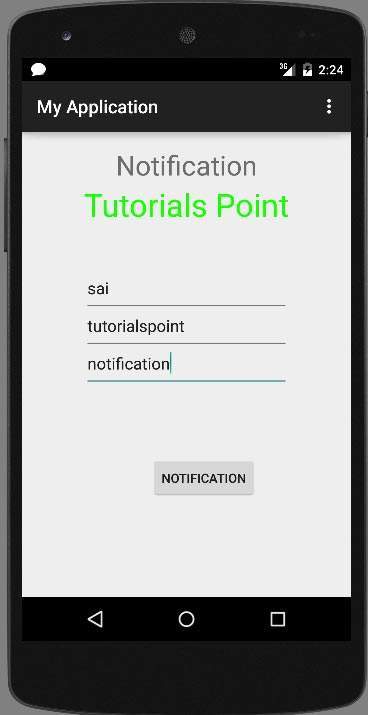
现在向下滚动通知栏,查看通知。已如下所示 −
Now scroll down the notification bar and see the notification. This has been shown below in the figure −
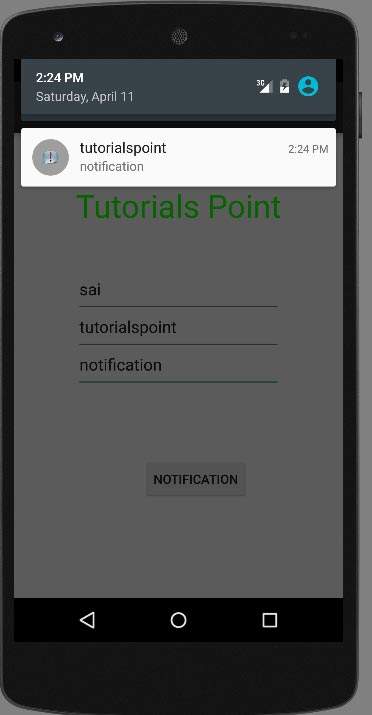