Android 简明教程
Android - Sending Email
在开始邮件活动之前,您必须了解搭配启动意图的邮件功能,启动意图是在应用程序中或应用程序外将数据从一个组件传送到另一个组件。
Before starting Email Activity, You must know Email functionality with intent, Intent is carrying data from one component to another component with-in the application or outside the application.
如果想从应用程序发送电子邮件,则不必从头开始实现电子邮件客户端,而是可以使用现成的客户端,例如 Android 提供的默认邮件应用程序、Gmail、Outlook、K-9 邮件等。为此,我们需要编写一项活动来启动电子邮件客户端,使用带有正确操作和数据的隐式启动意图。在此示例中,我们要通过使用启动现有电子邮件客户端的启动意图对象从我们的应用程序发送电子邮件。
To send an email from your application, you don’t have to implement an email client from the beginning, but you can use an existing one like the default Email app provided from Android, Gmail, Outlook, K-9 Mail etc. For this purpose, we need to write an Activity that launches an email client, using an implicit Intent with the right action and data. In this example, we are going to send an email from our app by using an Intent object that launches existing email clients.
以下段落解释了发送电子邮件所需的启动意图对象的不同部分。
Following section explains different parts of our Intent object required to send an email.
Intent Object - Action to send Email
您将使用 ACTION_SEND 操作来启动安装在您的 Android 设备上的电子邮件客户端。以下为创建带有 ACTION_SEND 操作的启动意图的简单语法。
You will use ACTION_SEND action to launch an email client installed on your Android device. Following is simple syntax to create an intent with ACTION_SEND action.
Intent emailIntent = new Intent(Intent.ACTION_SEND);
Intent Object - Data/Type to send Email
若要发送电子邮件,您需要使用 setData() 方法将 mailto: 指定为 URI,并且数据类型应如下所示使用 setType() 方法为 text/plain 。
To send an email you need to specify mailto: as URI using setData() method and data type will be to text/plain using setType() method as follows −
emailIntent.setData(Uri.parse("mailto:"));
emailIntent.setType("text/plain");
Intent Object - Extra to send Email
Android 具有内置支持,可以在将启动意图发送到目标电子邮件客户端之前将 TO、SUBJECT、CC、TEXT 等字段附加到启动意图中。您可以在电子邮件中使用以下额外字段,
Android has built-in support to add TO, SUBJECT, CC, TEXT etc. fields which can be attached to the intent before sending the intent to a target email client. You can use following extra fields in your email −
Sr.No. |
Extra Data & Description |
1 |
EXTRA_BCC A String[] holding e-mail addresses that should be blind carbon copied. |
2 |
EXTRA_CC A String[] holding e-mail addresses that should be carbon copied. |
3 |
EXTRA_EMAIL A String[] holding e-mail addresses that should be delivered to. |
4 |
EXTRA_HTML_TEXT A constant String that is associated with the Intent, used with ACTION_SEND to supply an alternative to EXTRA_TEXT as HTML formatted text. |
5 |
EXTRA_SUBJECT A constant string holding the desired subject line of a message. |
6 |
EXTRA_TEXT A constant CharSequence that is associated with the Intent, used with ACTION_SEND to supply the literal data to be sent. |
7 |
EXTRA_TITLE A CharSequence dialog title to provide to the user when used with a ACTION_CHOOSER. |
这是一个示例,向您展示如何为启动意图分配额外数据
Here is an example showing you how to assign extra data to your intent −
emailIntent.putExtra(Intent.EXTRA_EMAIL , new String[]{"Recipient"});
emailIntent.putExtra(Intent.EXTRA_SUBJECT, "subject");
emailIntent.putExtra(Intent.EXTRA_TEXT , "Message Body");
上述代码的输出如下所示
The out-put of above code is as below shown an image
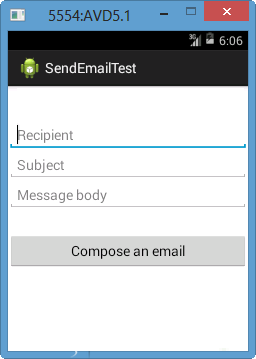
Example
以下示例实际向您展示如何使用启动意图对象启动电子邮件客户端,以便向给定的收件人发送电子邮件。
Following example shows you in practical how to use Intent object to launch Email client to send an Email to the given recipients.
Step |
Description |
1 |
You will use Android studio to create an Android application and name it as Tutorialspoint under a package com.example.tutorialspoint. |
2 |
Modify src/MainActivity.java file and add required code to take care of sending email. |
3 |
Modify layout XML file res/layout/activity_main.xml add any GUI component if required. I’m adding a simple button to launch Email Client. |
4 |
Modify res/values/strings.xml to define required constant values |
5 |
Modify AndroidManifest.xml as shown below |
6 |
Run the application to launch Android emulator and verify the result of the changes done in the application. |
以下是已修改后的主活动文件 src/com.example.Tutorialspoint/MainActivity.java 的内容。
Following is the content of the modified main activity file src/com.example.Tutorialspoint/MainActivity.java.
package com.example.tutorialspoint;
import android.net.Uri;
import android.os.Bundle;
import android.app.Activity;
import android.content.Intent;
import android.util.Log;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button startBtn = (Button) findViewById(R.id.sendEmail);
startBtn.setOnClickListener(new View.OnClickListener() {
public void onClick(View view) {
sendEmail();
}
});
}
protected void sendEmail() {
Log.i("Send email", "");
String[] TO = {""};
String[] CC = {""};
Intent emailIntent = new Intent(Intent.ACTION_SEND);
emailIntent.setData(Uri.parse("mailto:"));
emailIntent.setType("text/plain");
emailIntent.putExtra(Intent.EXTRA_EMAIL, TO);
emailIntent.putExtra(Intent.EXTRA_CC, CC);
emailIntent.putExtra(Intent.EXTRA_SUBJECT, "Your subject");
emailIntent.putExtra(Intent.EXTRA_TEXT, "Email message goes here");
try {
startActivity(Intent.createChooser(emailIntent, "Send mail..."));
finish();
Log.i("Finished sending email...", "");
} catch (android.content.ActivityNotFoundException ex) {
Toast.makeText(MainActivity.this, "There is no email client installed.", Toast.LENGTH_SHORT).show();
}
}
}
以下是 res/layout/activity_main.xml 文件的内容——
Following will be the content of res/layout/activity_main.xml file −
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Sending Mail Example"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:textSize="30dp" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tutorials point "
android:textColor="#ff87ff09"
android:textSize="30dp"
android:layout_above="@+id/imageButton"
android:layout_alignRight="@+id/imageButton"
android:layout_alignEnd="@+id/imageButton" />
<ImageButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/imageButton"
android:src="@drawable/abc"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true" />
<Button
android:id="@+id/sendEmail"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/compose_email"/>
</LinearLayout>
以下是 res/values/strings.xml 的内容,用于定义两个新常量——
Following will be the content of res/values/strings.xml to define two new constants −
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">Tutorialspoint</string>
<string name="compose_email">Compose Email</string>
</resources>
以下是 AndroidManifest.xml 的默认内容−
Following is the default content of AndroidManifest.xml −
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.Tutorialspoint" >
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name="com.example.tutorialspoint.MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
让我们尝试运行您的 tutorialspoint 应用程序。我假设您已将实际 Android 移动设备连接到计算机。要从 Android Studio 运行应用,请打开项目的活动文件之一,然后从工具栏中单击“运行”图标。在启动应用程序之前,Android Studio 安装程序将显示以下窗口以选择您要运行 Android 应用程序的位置。选择移动设备作为选项,然后检查移动设备上将显示以下屏幕的内容 -
Let’s try to run your tutorialspoint application. I assume you have connected your actual Android Mobile device with your computer. To run the app from Android Studio, open one of your project’s activity files and click Run icon from the toolbar. Before starting your application, Android studio installer will display following window to select an option where you want to run your Android application.Select your mobile device as an option and then check your mobile device which will display following screen −

现在使用 Compose Email 按钮列出所有已安装的电子邮件客户端。您可在列表中选择一个电子邮件客户端来发送电子邮件。我将使用 Gmail 客户端发送邮件,这里将显示所有提供的默认字段,如下所示。在此, From: 将是您为 Android 设备注册的默认电子邮件 ID。
Now use Compose Email button to list down all the installed email clients. From the list, you can choose one of email clients to send your email. I’m going to use Gmail client to send my email which will have all the provided defaults fields available as shown below. Here From: will be default email ID you have registered for your Android device.
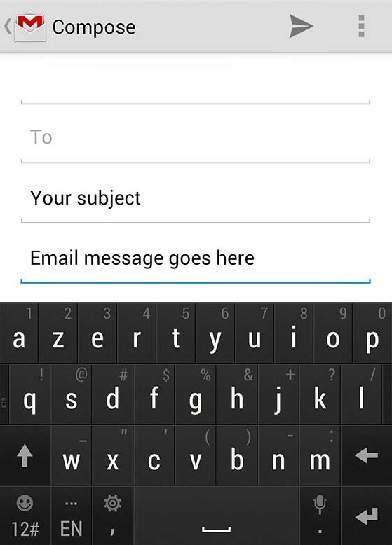
您可以修改任何已给出的默认字段,最后使用“发送电子邮件”按钮将电子邮件发送给所提到的收件人。
You can modify either of the given default fields and finally use send email button to send your email to the mentioned recipients.