Android 简明教程
Android - UI Design
在本章中,我们将研究 Android 屏幕的不同 UI 组件。本章还涵盖了创建更好的 UI 设计的提示,并解释了如何设计 UI。
In this chapter we will look at the different UI components of android screen. This chapter also covers the tips to make a better UI design and also explains how to design a UI.
UI screen components
Android 应用程序的典型用户界面由操作栏和应用程序内容区域组成。
A typical user interface of an android application consists of action bar and the application content area.
-
Main Action Bar
-
View Control
-
Content Area
-
Split Action Bar
这些组件也在下图中显示 −
These components have also been shown in the image below −
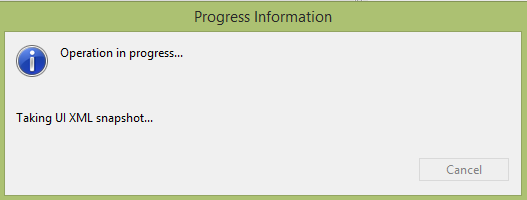
Understanding Screen Components
Android 应用程序的基本单元是 Activity。UI 在 XML 文件中定义。在编译过程中,XML 中的每个元素都被编译成等效的 Android GUI 类,其中属性由方法表示。
The basic unit of android application is the activity. A UI is defined in an xml file. During compilation, each element in the XML is compiled into equivalent Android GUI class with attributes represented by methods.
View and ViewGroups
Activity 由视图组成。视图只是一个出现在屏幕上的小部件。它可以是按钮等。一个或多个视图可以分组到一个 GroupView 中。ViewGroup 的示例包括布局。
An activity is consist of views. A view is just a widget that appears on the screen. It could be button e.t.c. One or more views can be grouped together into one GroupView. Example of ViewGroup includes layouts.
Types of layout
有许多类型的布局。其中一些如下所列 −
There are many types of layout. Some of which are listed below −
-
Linear Layout
-
Absolute Layout
-
Table Layout
-
Frame Layout
-
Relative Layout
Linear Layout
线性布局进一步分为水平布局和垂直布局。这意味着它可以将视图排列在单列或单行中。以下是包含文本视图的线性布局(垂直)代码。
Linear layout is further divided into horizontal and vertical layout. It means it can arrange views in a single column or in a single row. Here is the code of linear layout(vertical) that includes a text view.
<?xml version=”1.0” encoding=”utf-8”?>
<LinearLayout xmlns:android=”http://schemas.android.com/apk/res/android”
android:layout_width=”fill_parent”
android:layout_height=”fill_parent”
android:orientation=”vertical” >
<TextView
android:layout_width=”fill_parent”
android:layout_height=”wrap_content”
android:text=”@string/hello” />
</LinearLayout>
AbsoluteLayout
AbsoluteLayout 使您能够指定其子项的确切位置。可以这样声明。
The AbsoluteLayout enables you to specify the exact location of its children. It can be declared like this.
<AbsoluteLayout
android:layout_width=”fill_parent”
android:layout_height=”fill_parent”
xmlns:android=”http://schemas.android.com/apk/res/android” >
<Button
android:layout_width=”188dp”
android:layout_height=”wrap_content”
android:text=”Button”
android:layout_x=”126px”
android:layout_y=”361px” />
</AbsoluteLayout>
TableLayout
TableLayout 将视图分组到行和列中。可以这样声明。
The TableLayout groups views into rows and columns. It can be declared like this.
<TableLayout
xmlns:android=”http://schemas.android.com/apk/res/android”
android:layout_height=”fill_parent”
android:layout_width=”fill_parent” >
<TableRow>
<TextView
android:text=”User Name:”
android:width =”120dp”
/>
<EditText
android:id=”@+id/txtUserName”
android:width=”200dp” />
</TableRow>
</TableLayout>
RelativeLayout
RelativeLayout 使您能够指定子视图如何相对于彼此定位。可以这样声明。
The RelativeLayout enables you to specify how child views are positioned relative to each other.It can be declared like this.
<RelativeLayout
android:id=”@+id/RLayout”
android:layout_width=”fill_parent”
android:layout_height=”fill_parent”
xmlns:android=”http://schemas.android.com/apk/res/android” >
</RelativeLayout>
FrameLayout
FrameLayout 是屏幕上的占位符,可用于显示单个视图。可以这样声明。
The FrameLayout is a placeholder on screen that you can use to display a single view. It can be declared like this.
<?xml version=”1.0” encoding=”utf-8”?>
<FrameLayout
android:layout_width=”wrap_content”
android:layout_height=”wrap_content”
android:layout_alignLeft=”@+id/lblComments”
android:layout_below=”@+id/lblComments”
android:layout_centerHorizontal=”true” >
<ImageView
android:src = “@drawable/droid”
android:layout_width=”wrap_content”
android:layout_height=”wrap_content” />
</FrameLayout>
除了这些属性外,所有视图和视图组中还有其他一些常见的属性。它们如下所示:
Apart form these attributes, there are other attributes that are common in all views and ViewGroups. They are listed below −
Sr.No |
View & description |
1 |
layout_width Specifies the width of the View or ViewGroup |
2 |
layout_height Specifies the height of the View or ViewGroup |
3 |
layout_marginTop Specifies extra space on the top side of the View or ViewGroup |
4 |
layout_marginBottom Specifies extra space on the bottom side of the View or ViewGroup |
5 |
layout_marginLeft Specifies extra space on the left side of the View or ViewGroup |
6 |
layout_marginRight Specifies extra space on the right side of the View or ViewGroup |
7 |
layout_gravity Specifies how child Views are positioned |
8 |
layout_weight Specifies how much of the extra space in the layout should be allocated to the View |
Units of Measurement
当您指定安卓用户界面元素的大小时,应记住以下度量单位。
When you are specifying the size of an element on an Android UI, you should remember the following units of measurement.
Sr.No |
Unit & description |
1 |
dp Density-independent pixel. 1 dp is equivalent to one pixel on a 160 dpi screen. |
2 |
sp Scale-independent pixel. This is similar to dp and is recommended for specifying font sizes |
3 |
pt Point. A point is defined to be 1/72 of an inch, based on the physical screen size. |
4 |
px Pixel. Corresponds to actual pixels on the screen |