Asp.net 简明教程
ASP.NET - Web Services
Web 服务是基于 Web 的功能,它使用 Web 协议以供 Web 应用程序使用。开发 Web 服务有三个方面:
A web service is a web-based functionality accessed using the protocols of the web to be used by the web applications. There are three aspects of web service development:
-
Creating the web service
-
Creating a proxy
-
Consuming the web service
Creating a Web Service
Web 服务是一项 Web 应用程序,它基本上是一个类,该类由可供其他应用程序使用的方法组成。它还遵循代码分离架构,例如 ASP.NET 网页,尽管它没有用户界面。
A web service is a web application which is basically a class consisting of methods that could be used by other applications. It also follows a code-behind architecture such as the ASP.NET web pages, although it does not have a user interface.
为了理解此概念,让我们创建一个 Web 服务以提供股票价格信息。客户端可以根据股票代码查询股票的名称和价格。为了使此示例更简单,我们将值硬编码到二维数组中。此 Web 服务有三种方法:
To understand the concept let us create a web service to provide stock price information. The clients can query about the name and price of a stock based on the stock symbol. To keep this example simple, the values are hardcoded in a two-dimensional array. This web service has three methods:
-
A default HelloWorld method
-
A GetName Method
-
A GetPrice Method
执行以下步骤以创建 Web 服务:
Take the following steps to create the web service:
Step (1) : 在 Visual Studio 中选择 File → New → Web Site,然后选择 ASP.NET Web Service。
Step (1) : Select File → New → Web Site in Visual Studio, and then select ASP.NET Web Service.
Step (2) : 在该项目的 App_Code 目录中创建名为 Service.asmx 的 Web 服务文件及其背后的代码文件 Service.cs。
Step (2) : A web service file called Service.asmx and its code behind file, Service.cs is created in the App_Code directory of the project.
Step (3) : 将文件名称更改为 StockService.asmx 和 StockService.cs。
Step (3) : Change the names of the files to StockService.asmx and StockService.cs.
Step (4) : .asmx 文件中仅有一个 WebService 指令:
Step (4) : The .asmx file has simply a WebService directive on it:
<%@ WebService Language="C#" CodeBehind="~/App_Code/StockService.cs" Class="StockService" %>
Step (5) : 打开 StockService.cs 文件,其中生成的基本代码是 Hello World 服务。默认 Web 服务背后的代码文件如下所示:
Step (5) : Open the StockService.cs file, the code generated in it is the basic Hello World service. The default web service code behind file looks like the following:
using System;
using System.Collections;
using System.ComponentModel;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Web.Services.Protocols;
using System.Xml.Linq;
namespace StockService
{
// <summary>
// Summary description for Service1
// <summary>
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[ToolboxItem(false)]
// To allow this Web Service to be called from script,
// using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class Service1 : System.Web.Services.WebService
{
[WebMethod]
public string HelloWorld()
{
return "Hello World";
}
}
}
Step (6) : 更改背后的代码以添加股票代码、名称和价格的二维字符串数组和两个获取股票信息的 Web 方法。
Step (6) : Change the code behind file to add the two dimensional array of strings for stock symbol, name and price and two web methods for getting the stock information.
using System;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Web.Services.Protocols;
using System.Xml.Linq;
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
// To allow this Web Service to be called from script,
// using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class StockService : System.Web.Services.WebService
{
public StockService () {
//Uncomment the following if using designed components
//InitializeComponent();
}
string[,] stocks =
{
{"RELIND", "Reliance Industries", "1060.15"},
{"ICICI", "ICICI Bank", "911.55"},
{"JSW", "JSW Steel", "1201.25"},
{"WIPRO", "Wipro Limited", "1194.65"},
{"SATYAM", "Satyam Computers", "91.10"}
};
[WebMethod]
public string HelloWorld() {
return "Hello World";
}
[WebMethod]
public double GetPrice(string symbol)
{
//it takes the symbol as parameter and returns price
for (int i = 0; i < stocks.GetLength(0); i++)
{
if (String.Compare(symbol, stocks[i, 0], true) == 0)
return Convert.ToDouble(stocks[i, 2]);
}
return 0;
}
[WebMethod]
public string GetName(string symbol)
{
// It takes the symbol as parameter and
// returns name of the stock
for (int i = 0; i < stocks.GetLength(0); i++)
{
if (String.Compare(symbol, stocks[i, 0], true) == 0)
return stocks[i, 1];
}
return "Stock Not Found";
}
}
Step (7) : 运行 Web 服务应用程序会提供一个 Web 服务测试页,允许测试服务方法。
Step (7) : Running the web service application gives a web service test page, which allows testing the service methods.

Step (8) : 单击方法名称,并检查它是否正常运行。
Step (8) : Click on a method name, and check whether it runs properly.
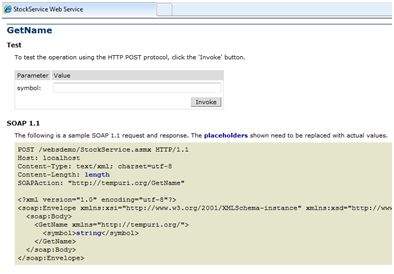
Step (9) : 为了测试 GetName 方法,提供一个硬编码的股票代码,它会返回股票名称
Step (9) : For testing the GetName method, provide one of the stock symbols, which are hard coded, it returns the name of the stock
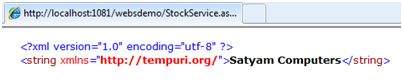
Consuming the Web Service
要使用 Web 服务,请在同一解决方案下创建一个 Web 站点。这可以通过在 Solution Explorer 中右键单击 Solution 名称来完成。调用 Web 服务的 Web 页应包含一个标签控件,以显示返回的结果,并且两个按钮控件,分别用于回发和调用服务。
For using the web service, create a web site under the same solution. This could be done by right clicking on the Solution name in the Solution Explorer. The web page calling the web service should have a label control to display the returned results and two button controls one for post back and another for calling the service.
Web 应用程序的内容文件如下:
The content file for the web application is as follows:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="wsclient._Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>
Untitled Page
</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h3>Using the Stock Service</h3>
<br /> <br />
<asp:Label ID="lblmessage" runat="server"></asp:Label>
<br /> <br />
<asp:Button ID="btnpostback" runat="server" onclick="Button1_Click" Text="Post Back" style="width:132px" />
<asp:Button ID="btnservice" runat="server" onclick="btnservice_Click" Text="Get Stock" style="width:99px" />
</div>
</form>
</body>
</html>
Web 应用程序背后的代码文件如下:
The code behind file for the web application is as follows:
using System;
using System.Collections;
using System.Configuration;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
//this is the proxy
using localhost;
namespace wsclient
{
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
lblmessage.Text = "First Loading Time: " + DateTime.Now.ToLongTimeString
}
else
{
lblmessage.Text = "PostBack at: " + DateTime.Now.ToLongTimeString();
}
}
protected void btnservice_Click(object sender, EventArgs e)
{
StockService proxy = new StockService();
lblmessage.Text = String.Format("Current SATYAM Price:{0}",
proxy.GetPrice("SATYAM").ToString());
}
}
}
Creating the Proxy
代理是 Web 服务代码的替身。在使用 Web 服务之前,必须创建一个代理。该代理会注册到客户端应用程序中。然后,客户端应用程序会进行对 Web 服务的调用,就像在使用本地方法一样。
A proxy is a stand-in for the web service codes. Before using the web service, a proxy must be created. The proxy is registered with the client application. Then the client application makes the calls to the web service as it were using a local method.
代理接收调用,将其包装成适当的格式,作为 SOAP 请求发送给服务器。SOAP 代表简单对象访问协议。该协议用于交换 Web 服务数据。
The proxy takes the calls, wraps it in proper format and sends it as a SOAP request to the server. SOAP stands for Simple Object Access Protocol. This protocol is used for exchanging web service data.
当服务器将 SOAP 包裹返回给客户端时,代理将对其进行解码,并将其显示给客户端应用程序。
When the server returns the SOAP package to the client, the proxy decodes everything and presents it to the client application.
在使用 btnservice_Click 调用 Web 服务之前,应向应用程序添加 Web 引用。这将透明地创建一个代理类,该类由 btnservice_Click 事件使用。
Before calling the web service using the btnservice_Click, a web reference should be added to the application. This creates a proxy class transparently, which is used by the btnservice_Click event.
protected void btnservice_Click(object sender, EventArgs e)
{
StockService proxy = new StockService();
lblmessage.Text = String.Format("Current SATYAM Price: {0}",
proxy.GetPrice("SATYAM").ToString());
}
执行以下步骤来创建代理:
Take the following steps for creating the proxy:
Step (1) : 在 Solution Explorer 中右键单击 Web 应用程序项,然后单击“Add Web Reference”。
Step (1) : Right click on the web application entry in the Solution Explorer and click on 'Add Web Reference'.

Step (2) : 选择“Web Services in this solution”。它会返回 StockService 引用。
Step (2) : Select 'Web Services in this solution'. It returns the StockService reference.

Step (3) : 单击该服务会打开测试 Web 页。默认情况下,创建的代理名为“localhost”,您可以重命名它。单击“Add Reference”以将该代理添加到客户端应用程序。
Step (3) : Clicking on the service opens the test web page. By default the proxy created is called 'localhost', you can rename it. Click on 'Add Reference' to add the proxy to the client application.
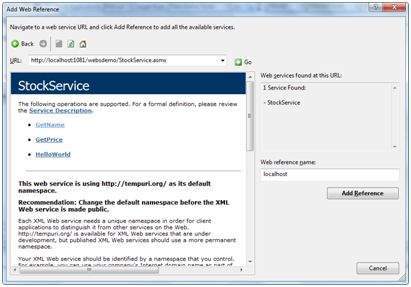
在代码文件中通过添加以下内容,以包含代理:
Include the proxy in the code behind file by adding:
using localhost;