Coffeescript 简明教程
CoffeeScript - Loops
在编码时,你可能会遇到需要一遍又一遍执行代码块的情况。在这种情况下,你可以使用循环语句。
While coding, you may encounter a situation where you need to execute a block of code over and over again. In such situations, you can use loop statements.
通常,语句按顺序执行:函数中的第一个语句首先执行,然后是第二个,依此类推。
In general, statements are executed sequentially: The first statement in a function is executed first, followed by the second, and so on.
循环语句允许我们多次执行一个语句或一组语句。以下是大多数编程语言中循环语句的一般形式
A loop statement allows us to execute a statement or group of statements multiple times. Given below is the general form of a loop statement in most of the programming languages
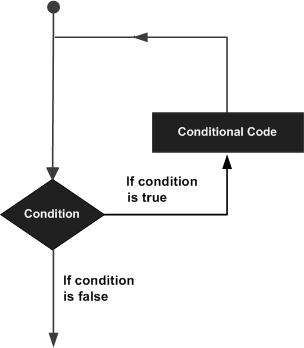
JavaScript 提供了 while, for 和 for..in 循环。CoffeeScript 中的循环类似于 JavaScript 中的循环。
JavaScript provides while, for and for..in loops. The loops in CoffeeScript are similar to those in JavaScript.
while 循环及其变体是 CoffeeScript 中唯一的循环构造。CoffeeScript 没有采用常用的 for 循环,而是为您提供了 Comprehensions ,这些内容将在后面的章节中详细讨论。
while loop and its variants are the only loop constructs in CoffeeScript. Instead of the commonly used for loop, CoffeeScript provides you Comprehensions which are discussed in detail in later chapters.
The while loop in CoffeeScript
while 循环是 CoffeeScript 唯一提供的低级循环。它包含一个布尔表达式和一个语句块。只要给定的布尔表达式为真, while 循环就会重复执行指定的语句块。一旦表达式变为假,循环就会终止。
The while loop is the only low-level loop that CoffeeScript provides. It contains a Boolean expression and a block of statements. The while loop executes the specified block of statements repeatedly as long as the given Boolean expression is true. Once the expression becomes false, the loop terminates.
Syntax
以下是在 CoffeeScript 中 while 循环的语法。在此处,无需使用圆括号来指定布尔表达式,并且我们必须使用(一致数量的)空格缩进循环主体,而不是用大括号将其括起来。
Following is the syntax of the while loop in CoffeeScript. Here, there is no need of the parenthesis to specify the Boolean expression and we have to indent the body of the loop using (consistent number of) whitespaces instead of wrapping it with curly braces.
while expression
statements to be executed
Example
以下示例演示了在 CoffeeScript 中使用 while 循环。将此代码保存在名为 while_loop_example.coffee 的文件中
The following example demonstrates the usage of while loop in CoffeeScript. Save this code in a file with name while_loop_example.coffee
console.log "Starting Loop "
count = 0
while count < 10
console.log "Current Count : " + count
count++;
console.log "Set the variable to different value and then try"
打开 command prompt 并按照以下所示编译 .coffee 文件。
Open the command prompt and compile the .coffee file as shown below.
c:\> coffee -c while_loop_example.coffee
编译后,它会给你以下 JavaScript。
On compiling, it gives you the following JavaScript.
// Generated by CoffeeScript 1.10.0
(function() {
var count;
console.log("Starting Loop ");
count = 0;
while (count < 10) {
console.log("Current Count : " + count);
count++;
}
console.log("Set the variable to different value and then try");
}).call(this);
现在,再次打开 command prompt 并按照以下所示运行 CoffeeScript 文件。
Now, open the command prompt again and run the CoffeeScript file as shown below.
c:\> coffee while_loop_example.coffee
执行后,CoffeeScript 文件产生以下输出。
On executing, the CoffeeScript file produces the following output.
Starting Loop
Current Count : 0
Current Count : 1
Current Count : 2
Current Count : 3
Current Count : 4
Current Count : 5
Current Count : 6
Current Count : 7
Current Count : 8
Current Count : 9
Set the variable to different value and then try
Variants of while
CoffeeScript 中的 While 循环有两个变体,即 until variant 和 loop variant 。
The While loop in CoffeeScript have two variants namely the until variant and the loop variant.
S.No. |
Loop Type & Description |
1 |
until variant of whileThe until variant of the while loop contains a Boolean expression and a block of code. The code block of this loop is executed as long as the given Boolean expression is false. |
2 |
loop variant of whileThe loop variant is equivalent to the while loop with true value (while true). The statements in this loop will be executed repeatedly until we exit the loop using the Break statement. |