Cypress 简明教程
Cypress - Assertions
Cypress 拥有多种断言类型,这些类型来自不同的库,例如 Mocha、Chai 等等。断言类型分为显式和隐式。
Cypress has more than one type of assertions obtained from various libraries like Mocha,Chai, and so on. The assertion types are explicit and implicit.
Implicit Assertions
如果一个断言适用于链中从父命令获取的对象,则称为隐式断言。流行的隐式断言包括 .and/.should。
If an assertion is applicable to the object obtained from the parent command in a chain, it is known as the implicit assertion. The popular implicit assertions include .and/.should.
这些命令不能作为独立命令使用。通常,当我们必须对特定对象执行多个检查时,我们使用它们。
These commands cannot be used as standalone. Generally, they are used when we have to verify multiple checks on a particular object.
让我们通过下面的示例来说明隐式断言 −
Let us illustrate implicit assertion with an example given below −
// test suite
describe('Tutorialspoint', function () {
it('Scenario 1', function (){
// test step to launch a URL
cy.visit("https://www.tutorialspoint.com/videotutorials/index.php")
// assertion to validate count of sub-elements and class attribute value
cy.get('.toc chapters').find('li').should('have.length',5)
.and('have.class', 'dropdown')
});
});
Execution Results
Execution Results
输出如下 −
The output is as follows −
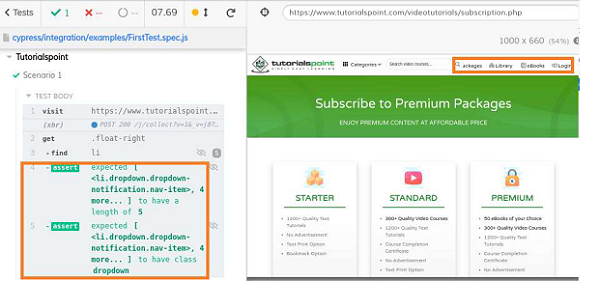
输出日志显示了使用 should 和命令获得的两个断言。
The output logs show two assertions obtained with should, and commands.
Explicit Assertions
如果一个断言适用于对象本身,则称为显式断言。流行的显式断言包括 assert/expect。
If an assertion is applicable to an object directly, it is known as the explicit assertion. The popular explicit assertions include assert/expect.
用于显式断言的命令如下 −
The command for explicit assertion is as follows −
// test suite
describe('Tutorialspoint', function () {
// it function to identify test
it('Scenario 1', function (){
// test step to launch a URL
cy.visit("https://accounts.google.com")
// identify element
cy.get('h1#headingText').find('span').then(function(e){
const t = e.text()
// assertion expect
expect(t).to.contains('Sign')
})
})
})
Execution Results
Execution Results
输出如下 −
The output is given below −
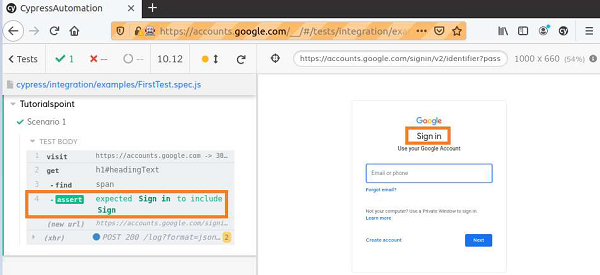
输出日志显示了使用 expect 命令直接应用于对象的断言。
The output logs show assertions directly applied to objects with the expect command.
Cypress 拥有默认断言,这些断言在内部处理,不需要专门调用。
Cypress has Default Assertions which are internally handled and do not require to be invoked specifically.
以下是一些示例 −
Few examples are as follows −
-
cy.visit () − Expects the page to show the content with 200 status code.
-
cy.request () − Expects the remote server to be available and sends a response.
-
cy.contains () − Expects the web element with its properties to be available in DOM.
-
cy.get () − Expects the web element to be available in DOM.
-
.find () − Expects the web element to be available in DOM.
-
.type () − Expects the web element to turn to a type able state.
-
.click () − Expects the web element to turn to a clickable state.
-
.its () − Expects for a web element property on the existing subject.
Other Cypress assertions
其他 Cypress 断言如下:
The other Cypress assertions are as follows −
length
It 检查从先前链接命令中获得的元素的计数。
It checks the count of elements obtained from the previously chained command.
例如,
For example,
cy.get('#txt-fld').should('have.length',5)
value
It 检查 Web 元素是否具有某个值。
It checks whether the web element has a certain value.
例如,
For example,
cy.get('#txt-fld').should('have.length',5)
value
It 检查 Web 元素是否具有某个值。
It checks whether the web element has a certain value.
例如,
For example,
cy.get(' #txt-fld').should('have.value', 'Cypress')
class
It 检查 Web 元素是否具有某个 class。
It checks whether the web element possesses a certain class.
例如,
For example,
cy.get('#txt-fld'').should('have.class', 'txt')
contain
It 检查 Web 元素是否具有某个文本。
It checks whether the web element possesses a certain text.
例如,
For example,
cy.get('#txt-fld'').should('contain', 'Cypress')
visible
It 检查 Web 元素是否可见。
It checks whether the web element is visible.
例如,
For example,
cy.get('#txt-fld'').should('be.visible')