Cypress 简明教程
Cypress - Asynchronous Behavior
Cypress 源自 node.js,后者基于 JavaScript。Cypress 命令本质上是同步的,因为它们依赖于 node 服务器。异步流意味着测试步骤在执行时不依赖于其先前的步骤。
Cypress is derived from node.js, which is based on JavaScript. Cypress commands are synchronous in nature, since they are dependent on node server. Asynchronous flow means that the test step does not depend on its prior step for execution.
没有依赖,每个步骤都作为独立的标识符执行。尽管测试步骤按顺序排列,但单个测试步骤不考虑前一步骤的结果,而仅仅执行自身。
There is no dependency and each of the steps is executed as a standalone identity. Though the test steps are arranged in a sequence, an individual test step does not consider the outcome of the previous step and simply executes itself.
Example
以下是在 Cypress 中异步行为的一个示例:
Following is an example of asynchronous behavior in Cypress −
// test suite
describe('Tutorialspoint', function () {
it('Scenario 1', function (){
// test step to launch a URL
cy.visit("https://accounts.google.com")
// identify element
cy.get('h1#headingText').find('span').should('have.text', 'Sign in')
cy.get('h1#headingText').find('span').then(function(e){
const t = e.text()
// get in Console
console.log(t)
})
// Console message
console.log("Tutorialspoint-Cypress")
})
})
Execution Results
Execution Results
输出如下 −
The output is given below −
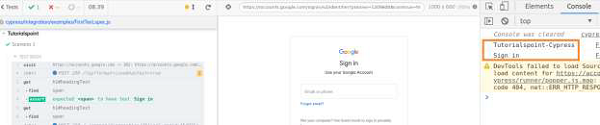
Promise
右键单击测试运行器,然后单击 Inspect,我们可以在控制台验证结果。此处, Tutorialspoint-Cypress (先前的步骤)在控制台登录,早于 Sign – in (稍后添加的步骤)。
Right-click on Test Runner and click on Inspect, and we can verify results in the Console.Here, Tutorialspoint-Cypress (an earlier step) is logged in the Console before Sign – in (step added later).
Cypress 命令的设计方式是按照顺序执行每一步,而不是同时触发它们。但是,它们按顺序排列。因此,它使得流变得同步。这是通过 Promise 实现的。
Cypress commands are designed in such a way that every step is executed in sequence and they are not triggered simultaneously. But, they are lined up one behind another.Thereby, it makes the flow as synchronous. This is achieved with Promise.
在上面的示例中, console.log 是一个纯 JavaScript 语句。它没有像 Cypress 命令那样排队等待的能力。Promise 使我们可以以串行模式执行 Cypress 命令。
In the above example, console.log is a pure JavaScript statement. It does not have the ability to line up and wait similar to Cypress commands. Promise allows us to execute Cypress commands in a serial mode.
Modes in Promise
Promise 有三种模式来对命令执行的状态进行分类。它们如下:
A Promise has three modes to categorise the state of a command execution. They are as follows −
-
Resolved − This outcome occurs, if the test step runs successfully.
-
Pending − This is the outcome, if the test step run result is being awaited.
-
Rejected − This is the outcome, if the test step runs unsuccessfully.
仅在先前的步骤已成功执行或收到解析后的 promise 响应后,才会执行 Cypress 命令。然后,该方法用于在 Cypress 中实现 Promise。
A Cypress command gets executed, only if the prior step has been executed successfully or a resolved promise response is received. Then, the method is used to implement Promise in Cypress.
Example
以下是在 Cypress 中 Promise 的示例:
Following is an example of Promise in Cypress −
describe('Tutorialspoint Test', function () {
it('Promise', function (){
return cy.visit('https://accounts.google.com')
.then(() => {
return cy.get('h1#heading');
})
})
})
Cypress 对 Promise 的实现是封装的,不可见的。因此,这有助于获得更紧凑的代码。此外,在自动化测试时,我们不必考虑 Promise 的状态。
Cypress implementation for Promise is encapsulated and not visible. Thus, it helps to have a more compact code. Also, we do not have to consider the state of Promise, while automating the tests.
Implementation without Promise
以下命令解释了如何在 Cypress 中在没有 promise 的情况下完成实现:
Following command explains how an implementation can be done without promise in Cypress −
describe('Tutorialspoint Test', function () {
it('Without Promise', function (){
cy.visit('https://accounts.google.com')
cy.get('h1#heading')
})
})