Django 简明教程
Django Admin – Set Fields to Display
当一个模型注册到 Django 的管理站点时,当您点击该模型的名称时,它对象列表会显示在列表中。
在下图中, Employees 模型中的对象列表会显示在列表中−
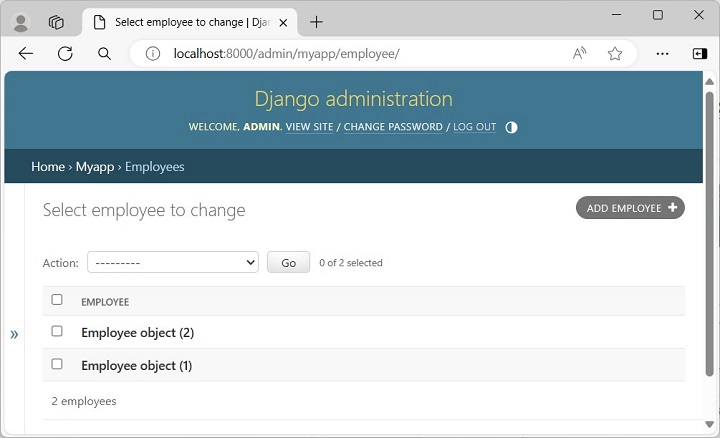
然而,上述页面以 Employee object(1) 的表格显示对象,这不会显示 name 或 contact 等属性。为了让对象描述更加用户友好,我们需要在 employee model class 中覆盖 str() method 。
employee class 被重写如下,以给出其对象的替代字符串表示。
from django.db import models
class Employee(models.Model):
empno = models.CharField(max_length=20)
empname = models.CharField(max_length=100)
contact = models.CharField(max_length=15)
salary = models.IntegerField()
joined_date = models.DateField(null=True)
class Meta:
db_table = "employee"
def __str__(self):
return "Name: {}, Contact: {}".format(self.empname, self.contact)
对模型定义进行这些更改,并刷新管理站点的首页(如果 Django 服务器尚未运行,则启动)。
在 MYAPP 中点击“员工”模型以显示 employee object 详细信息 −
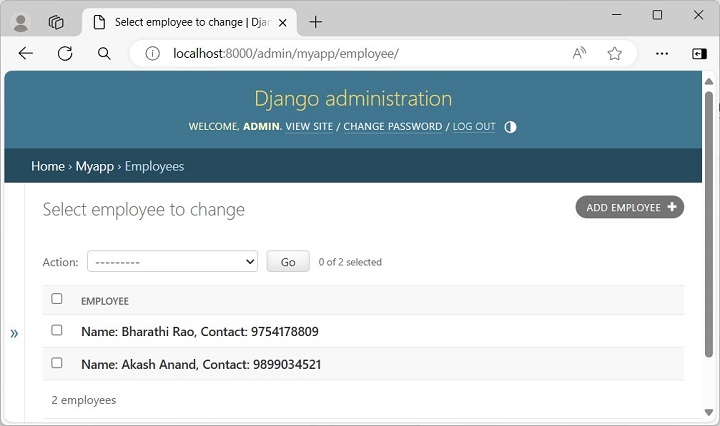
如果你点击任一对象详细信息,会出现显示对象属性的窗体,你可以在此处更新对象或删除对象。
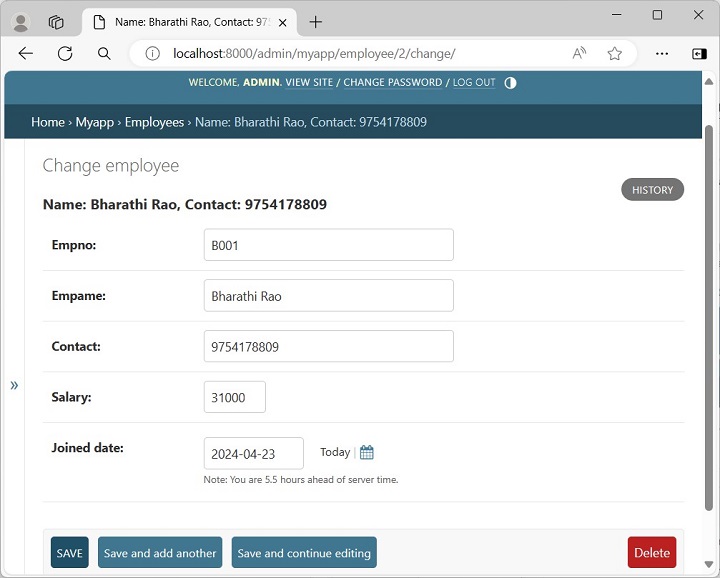
你可以使用 admin.ModelAdmin 对象的“ list_display ”属性来自定义“更改列表页面”,该属性指定在更改列表中显示的列。
ModelAdmin class 是模型在管理员界面中的表现形式。通常,这些存储在应用程序中名为 admin.py 的文件中。
通过继承 ModelAdmin class 来定义 EmployeeAdmin class 。将 list_display 属性设置为要显示的字段的元组。
from django.contrib import admin
# Register your models here.
from .models import Employee
class EmployeeAdmin(admin.ModelAdmin):
list_display = ("empname", "contact", "joined_date")
按如下所示注册 EmployeeAdmin 类 −
admin.site.register(Employee, EmployeeAdmin)
你也可以使用 @admin.register() decorator 注册此模型 −
from django.contrib import admin
# Register your models here.
from .models import Employee
@admin.register(Employee)
class EmployeeAdmin(admin.ModelAdmin):
list_display = ("empname", "contact", "joined_date")
保存以上更改并刷新管理员站点以显示员工对象列表 −
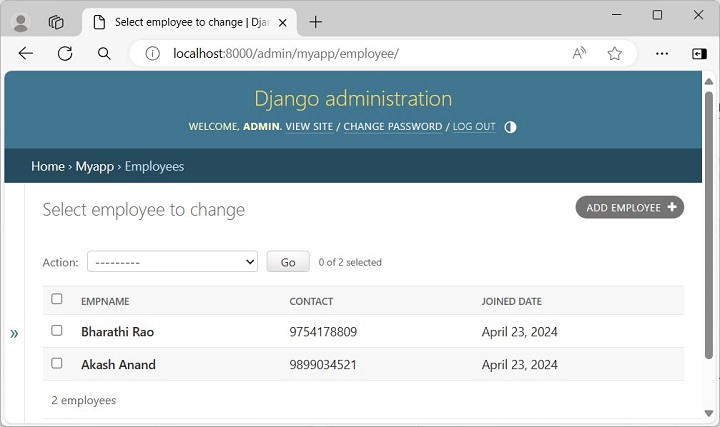
你还可以向列表页面提供搜索功能。在 EmployeeAdmin 类中添加 search_fields 属性 −
@admin.register(Employee)
class EmployeeAdmin(admin.ModelAdmin):
list_display = ("empname", "contact", "joined_date")
search_fields = ("empname__startswith", )
现在,列表页面在顶部显示搜索字段。搜索基于员工姓名进行。 __startswith 修饰符将搜索限制为以搜索参数开头的名称。

因此,Django 管理员界面是完全可定制的。你可以通过在管理员类中提供字段属性来设置在更新窗体中要提供的字段。