Java 简明教程
Java - Packages
Java Packages
在 Java 中使用 *Packages*是为了防止命名冲突、控制访问权限、使查找/定位和使用类、接口、枚举和注释等更轻松。
Packages are used in Java in order to prevent naming conflicts, control access, make searching/locating and usage of classes, interfaces, enumerations, and annotations easier, etc.
可以 将 Java package 定义为相关类型( classes 、 interfaces 、 enumerations 和注释)的分组,提供访问保护和命名空间管理。
A Java package can be defined as a grouping of related types (classes, interfaces, enumerations, and annotations ) providing access protection and namespace management.
Types of Java Packages
Java 包分为两种类型:
Java packages are of two types:
-
Built-in Java Packages
-
User-defined Java Packages
Java 中一些现存的包有:
Some of the existing packages in Java are −
-
java.lang − bundles the fundamental classes
-
java.io − classes for input , output functions are bundled in this package
User-defined Java Packages
你可以定义自己的包来汇集类/接口等等。按你实现的相关类进行分组是一种好做法,以便程序员可以轻松确定类、接口、枚举和注解是相关的。
You can define your own packages to bundle groups of classes/interfaces, etc. It is a good practice to group related classes implemented by you so that a programmer can easily determine that the classes, interfaces, enumerations, and annotations are related.
由于包创建了一个新的名称空间,因此不会与其他包中的名称发生名称冲突。使用包,可以更轻松地提供访问控制,也可以更轻松地找到相关类。
Since the package creates a new namespace there won’t be any name conflicts with names in other packages. Using packages, it is easier to provide access control and it is also easier to locate the related classes.
Creating a Java Package
创建包时,你应当为包选择一个名称,并在包中想要包含的类、接口、枚举和注解类型的每个源文件的顶部包含一条 package 语句。
While creating a package, you should choose a name for the package and include a package statement along with that name at the top of every source file that contains the classes, interfaces, enumerations, and annotation types that you want to include in the package.
包语句应当是源文件中的第一行。每个源文件中只能有一个包语句,它适用于文件中的所有类型。
The package statement should be the first line in the source file. There can be only one package statement in each source file, and it applies to all types in the file.
如果未使用包语句,则类、接口、枚举和注解类型将放置在当前默认包中。
If a package statement is not used then the class, interfaces, enumerations, and annotation types will be placed in the current default package.
Compiling with Java Package
若要编译带包语句的 Java 程序,你必须按如下所示使用 -d 选项。
To compile the Java programs with package statements, you have to use -d option as shown below.
javac -d Destination_folder file_name.java
然后在指定的目标位置创建一个带有给定包名称的文件夹,并将编译的类文件放置在该文件夹中。
Then a folder with the given package name is created in the specified destination, and the compiled class files will be placed in that folder.
Java Package Example
我们来看一个创建名为 animals 的包的示例。最好使用小写字母作为包的名称,以避免与类和接口的名称发生冲突。
Let us look at an example that creates a package called animals. It is a good practice to use names of packages with lower case letters to avoid any conflicts with the names of classes and interfaces.
以下包示例包含名为 animals 的接口:
Following package example contains interface named animals −
/* File name : Animal.java */
package animals;
interface Animal {
public void eat();
public void travel();
}
现在让我们在同一包 animals 中实现上述接口:
Now, let us implement the above interface in the same package animals −
package animals;
/* File name : MammalInt.java */
public class MammalInt implements Animal {
public void eat() {
System.out.println("Mammal eats");
}
public void travel() {
System.out.println("Mammal travels");
}
public int noOfLegs() {
return 0;
}
public static void main(String args[]) {
MammalInt m = new MammalInt();
m.eat();
m.travel();
}
}
interface Animal {
public void eat();
public void travel();
}
现在按如下所示编译 java 文件:
Now compile the java files as shown below −
$ javac -d . Animal.java
$ javac -d . MammalInt.java
现在在当前目录中将创建一个名称为 animals 的包/文件夹,并将这些类文件放置其中,如下所示。
Now a package/folder with the name animals will be created in the current directory and these class files will be placed in it as shown below.
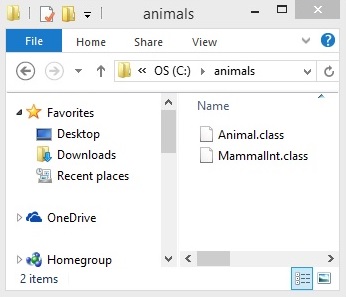
你可以在包中执行类文件并获取如下所示的结果。
You can execute the class file within the package and get the result as shown below.
Mammal eats
Mammal travels
Importing Java Package
如果某个类想要使用同一包中的另一个类,则不必使用包名称。同一包中的类可在没有任何特殊语法的条件下找到彼此。
If a class wants to use another class in the same package, the package name need not be used. Classes in the same package find each other without any special syntax.
Example
在这里,名为 Boss 的一个类被添加到已包含 Employee 的 payroll 包中。然后 Boss 可引用 Employee 类,而无需使用 payroll 前缀,如下面的 Boss 类所示。
Here, a class named Boss is added to the payroll package that already contains Employee. The Boss can then refer to the Employee class without using the payroll prefix, as demonstrated by the following Boss class.
package payroll;
public class Boss {
public void payEmployee(Employee e) {
e.mailCheck();
}
}
如果 Employee 类不在 payroll 包中会发生什么?现在 Boss 类必须使用以下技巧之一来引用一个在另一个包中的类。
What happens if the Employee class is not in the payroll package? The Boss class must then use one of the following techniques for referring to a class in a different package.
-
The fully qualified name of the class can be used. For example −
payroll.Employee
-
The package can be imported using the import keyword and the wild card (*). For example −
import payroll.*;
-
The class itself can be imported using the import keyword. For example −
import payroll.Employee;
Example
package payroll;
public class Employee {
public void mailCheck() {
System.out.println("Pay received.");
}
}
Example
package payroll;
import payroll.Employee;
public class Boss {
public void payEmployee(Employee e) {
e.mailCheck();
}
public static void main(String[] args) {
Boss boss = new Boss();
Employee e = new Employee();
boss.payEmployee(e);
}
}
Pay received.
Note − 一个类文件可包含任意数量的 import 语句。这些 import 语句必须出现在 package 语句之后并出现在类声明之前。
Note − A class file can contain any number of import statements. The import statements must appear after the package statement and before the class declaration.
Directory Structure of a Java Package
将一个类放入一个包中时,会产生两个主要结果 −
Two major results occur when a class is placed in a package −
-
The name of the package becomes a part of the name of the class, as we just discussed in the previous section.
-
The name of the package must match the directory structure where the corresponding bytecode resides.
下面是一种管理你在 Java 中的文件的简单方法 −
Here is simple way of managing your files in Java −
将类的源代码、接口、枚举或注释类型放入一个文本文件中,其名称是该类型的简单名称,其扩展名为 .java。
Put the source code for a class, interface, enumeration, or annotation type in a text file whose name is the simple name of the type and whose extension is .java.
例如 -
For example −
// File Name : Car.java
package vehicle;
public class Car {
// Class implementation.
}
现在,将该源文件放到一个目录中,其名称反映了该类所属的包的名称 −
Now, put the source file in a directory whose name reflects the name of the package to which the class belongs −
....\vehicle\Car.java
现在,限定类名和路径名如下 −
Now, the qualified class name and pathname would be as follows −
-
Class name → vehicle.Car
-
Path name → vehicle\Car.java (in windows)
通常情况下,一家公司会使用它反转的互联网域名作为其包名称。
In general, a company uses its reversed Internet domain name for its package names.
Example − 一家公司的互联网域名是 apple.com,那么它所有的包名称都将以 com.apple 开头。包名的每个部分对应于一个子目录。
Example − A company’s Internet domain name is apple.com, then all its package names would start with com.apple. Each component of the package name corresponds to a subdirectory.
Example − 该公司有一个 com.apple.computers 包,它包含一个 Dell.java 源文件,它会被包含在这个一系列的子目录中 −
Example − The company had a com.apple.computers package that contained a Dell.java source file, it would be contained in a series of subdirectories like this −
....\com\apple\computers\Dell.java
在编译时,编译器会为其中定义的每个类、接口和枚举创建一个独立的输出文件。该输出文件的基名是该类型的名称,而它的扩展名为 .class。
At the time of compilation, the compiler creates a different output file for each class, interface and enumeration defined in it. The base name of the output file is the name of the type, and its extension is .class.
例如 -
For example −
// File Name: Dell.java
package com.apple.computers;
public class Dell {
}
class Ups {
}
现在,使用 -d 选项编译这个文件,如下 −
Now, compile this file as follows using -d option −
$javac -d . Dell.java
文件将按如下方式编译 −
The files will be compiled as follows −
.\com\apple\computers\Dell.class
.\com\apple\computers\Ups.class
你可以按如下方式导入在 \com\apple\computer\ 中定义的所有类或接口 −
You can import all the classes or interfaces defined in \com\apple\computers\ as follows −
import com.apple.computers.*;
与 .java 源文件类似,编译的 .class 文件应位于一系列反映包名的目录中。但是,通往 .class 文件的路径不必与通往 .java 源文件的路径相同。你可以单独排列你的源文件和类目录,如下 −
Like the .java source files, the compiled .class files should be in a series of directories that reflect the package name. However, the path to the .class files does not have to be the same as the path to the .java source files. You can arrange your source and class directories separately, as −
<path-one>\sources\com\apple\computers\Dell.java
<path-two>\classes\com\apple\computers\Dell.class
通过执行此操作,可以在不透露你的源代码的情况下,让其他程序员访问 classes 目录。你同样需要管理源代码和类文件,以便编译器和 Java 虚拟机 (JVM) 可以找到你的程序所使用的所有类型。
By doing this, it is possible to give access to the classes directory to other programmers without revealing your sources. You also need to manage source and class files in this manner so that the compiler and the Java Virtual Machine (JVM) can find all the types your program uses.
classes 目录的完整路径 <path-two>\classes 称为类路径,并使用 CLASSPATH 系统变量进行设置。编译器和 JVM 都通过向类路径中添加包名称,来构建到 .class 文件的路径。
The full path to the classes directory, <path-two>\classes, is called the class path, and is set with the CLASSPATH system variable. Both the compiler and the JVM construct the path to your .class files by adding the package name to the class path.
假设 <path-two>\classes 是类路径,并且包名称为 com.apple.computers,那么编译器和 JVM 会在 <path-two>\classes\com\apple\computers 中寻找 .class 文件。
Say <path-two>\classes is the class path, and the package name is com.apple.computers, then the compiler and JVM will look for .class files in <path-two>\classes\com\apple\computers.
一个类路径中可包含多个路径。多个路径应使用分号(Windows)或冒号(Unix)分隔。默认情况下,编译器和 JVM 会搜索当前目录和包含 Java 平台类的 JAR 文件,以便这些目录自动位于类路径中。
A class path may include several paths. Multiple paths should be separated by a semicolon (Windows) or colon (Unix). By default, the compiler and the JVM search the current directory and the JAR file containing the Java platform classes so that these directories are automatically in the class path.
Set CLASSPATH System Variable
要显示当前的 CLASSPATH 变量,请在 Windows 和 UNIX(Bourne shell)中使用以下命令:
To display the current CLASSPATH variable, use the following commands in Windows and UNIX (Bourne shell) −
-
In Windows → C:> set CLASSPATH
-
In UNIX → % echo $CLASSPATH
要删除 CLASSPATH 变量的当前内容,请使用:
To delete the current contents of the CLASSPATH variable, use −
-
In Windows → C:> set CLASSPATH =
-
In UNIX → % unset CLASSPATH; export CLASSPATH
要设置 CLASSPATH 变量,请使用:
To set the CLASSPATH variable −
-
In Windows → set CLASSPATH = C:\users\jack\java\classes
-
In UNIX → % CLASSPATH = /home/jack/java/classes; export CLASSPATH