Javafx 简明教程
JavaFX - Rotation Transformation
依次旋转,我们从它的原点以一个特定的角度 θ (theta) 旋转对象。从下图中,我们可以看到 point P(X, Y) 位于距离原点 r 的水平 X 坐标 angle φ 处。

Rotation Transformation in JavaFX
旋转变换是使用 javafx.scene.transform 包中的 Rotate 类应用于 JavaFX 节点的。在此变换中,将仿射对象绕着具有指定角度的特定坐标旋转,并设置该坐标作为锚点。
Example 1
以下是演示 JavaFX 中旋转变换的程序。在其中,我们在同一位置创建 2 个矩形节点,具有相同的维度,但具有不同的颜色(米黄色和蓝色)。我们还对米黄色矩形应用旋转变换。
将此代码保存在名为 RotationExample.java 的文件中。
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.scene.transform.Rotate;
import javafx.stage.Stage;
public class RotationExample extends Application {
@Override
public void start(Stage stage) {
//Drawing Rectangle1
Rectangle rectangle1 = new Rectangle(150, 75, 200, 150);
rectangle1.setFill(Color.BLUE);
rectangle1.setStroke(Color.BLACK);
//Drawing Rectangle2
Rectangle rectangle2 = new Rectangle(150, 75, 200, 150);
//Setting the color of the rectangle
rectangle2.setFill(Color.BURLYWOOD);
//Setting the stroke color of the rectangle
rectangle2.setStroke(Color.BLACK);
//creating the rotation transformation
Rotate rotate = new Rotate();
//Setting the angle for the rotation
rotate.setAngle(20);
//Setting pivot points for the rotation
rotate.setPivotX(150);
rotate.setPivotY(225);
//Adding the transformation to rectangle2
rectangle2.getTransforms().addAll(rotate);
//Creating a Group object
Group root = new Group(rectangle1, rectangle2);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Rotation transformation example");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls RotationExample.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls RotationExample
在执行时,上述程序会生成一个 JavaFX 窗口,如下所示。
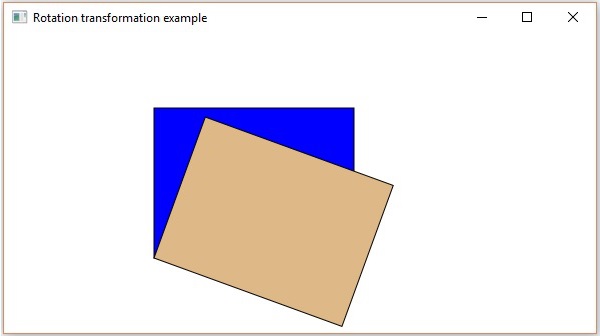
Example 2
在此示例中,我们尝试旋转一个 3D 对象,比如一个盒子。在这里,让我们创建两个盒子:一个表示它的原始位置,另一个显示变换后的位置。
将此代码保存在名为 RotationExample3D.java 的文件中。
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.shape.Box;
import javafx.scene.transform.Rotate;
import javafx.scene.transform.Translate;
import javafx.stage.Stage;
public class RotationExample3D extends Application {
@Override
public void start(Stage stage) {
//Drawing a Box
Box box1 = new Box();
Box box2 = new Box();
//Setting the properties of the Box
box1.setWidth(150.0);
box1.setHeight(150.0);
box1.setDepth(150.0);
//Setting the properties of the Box
box2.setWidth(150.0);
box2.setHeight(150.0);
box2.setDepth(150.0);
//Creating the translation transformation
Translate translate = new Translate();
translate.setX(200);
translate.setY(150);
translate.setZ(25);
Rotate rxBox = new Rotate(0, 0, 0, 0, Rotate.X_AXIS);
Rotate ryBox = new Rotate(0, 0, 0, 0, Rotate.Y_AXIS);
Rotate rzBox = new Rotate(0, 0, 0, 0, Rotate.Z_AXIS);
rxBox.setAngle(30);
ryBox.setAngle(50);
rzBox.setAngle(30);
box2.getTransforms().addAll(translate,rxBox, ryBox, rzBox);
//Creating a Group object
Group root = new Group(box1, box2);
//Creating a scene object
Scene scene = new Scene(root, 400, 300);
//Setting title to the Stage
stage.setTitle("Transformation of a Box");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls RotationExample3D.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls RotationExample3D
在执行时,上述程序会生成一个 JavaFX 窗口,如下所示。我们可以观察到盒子的原始位置不在应用程序内,因此,应用变换变得必要。
