Javafx 简明教程
JavaFX - Stroke Transition
我们已了解到填充过渡用于改变形状区域的颜色。与之类似,描边过渡用于改变形状的描边颜色。
We have learned that the fill transition is used to change the colors of a shape area. Similar to it, the stroke transition is used to change the stroke color of a shape.
JavaFX 中的形状可以包含三种类型的描边:内部、外部和居中;并且对其应用不同的属性。此过渡忽视描边的属性,而只是在指定的持续时间内改变其颜色。
A shape in JavaFX can consist of three types of strokes: inside, outside and centered; with different properties applied to it. This transition is ignorant to the property of a stroke and instead just changes its color over a course of duration specified.
Stroke Transition
JavaFX 使用属于 javafx.animation 包的 StrokeTransition 类提供描边过渡。通过导入此类,我们可以在 JavaFX 应用程序中更改任何形状的描边颜色。要实现此动画,此类提供了多种属性:
JavaFX offers stroke transition using the StrokeTransition class which belongs to the javafx.animation package. By importing this class, we can change the stroke color of any shape on a JavaFX application. To implement this animation, there are various properties this class provides −
-
duration − The duration of this StrokeTransition.
-
shape − The target shape of this StrokeTransition.
-
fromValue − Specifies the start color value for this StrokeTransition.
-
toValue − Specifies the stop color value for this StrokeTransition.
Example
以下是演示 JavaFX 中 Stoke 过渡的程序。将此代码保存在名为 StrokeTransitionExample.java 的文件中。
Following is the program which demonstrates Stoke Transition in JavaFX. Save this code in a file with the name StrokeTransitionExample.java.
import javafx.animation.StrokeTransition;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
import javafx.util.Duration;
public class StrokeTransitionExample extends Application {
@Override
public void start(Stage stage) {
//Drawing a Circle
Circle circle = new Circle();
//Setting the position of the circle
circle.setCenterX(300.0f);
circle.setCenterY(135.0f);
//Setting the radius of the circle
circle.setRadius(100.0f);
//Setting the color of the circle
circle.setFill(Color.BROWN);
//Setting the stroke width of the circle
circle.setStrokeWidth(20);
//creating stroke transition
StrokeTransition strokeTransition = new StrokeTransition();
//Setting the duration of the transition
strokeTransition.setDuration(Duration.millis(1000));
//Setting the shape for the transition
strokeTransition.setShape(circle);
//Setting the fromValue property of the transition (color)
strokeTransition.setFromValue(Color.BLACK);
//Setting the toValue property of the transition (color)
strokeTransition.setToValue(Color.BROWN);
//Setting the cycle count for the transition
strokeTransition.setCycleCount(50);
//Setting auto reverse value to false
strokeTransition.setAutoReverse(false);
//Playing the animation
strokeTransition.play();
//Creating a Group object
Group root = new Group(circle);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Stroke transition example");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeTransitionExample.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeTransitionExample
执行后,上述程序会生成如下所示的 JavaFX 窗口。
On executing, the above program generates a JavaFX window as shown below.
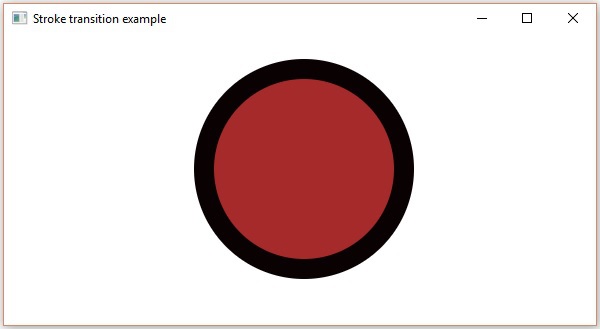