Javafx 简明教程
JavaFX - ToolBar
ToolBar 是一种图形用户界面控件,具有按钮、菜单或其他控件的水平或垂直条带。它通常用于快速访问常用的命令或功能。我们可以将工具栏放置在任何应用程序或网页的顶部,如下图所示:
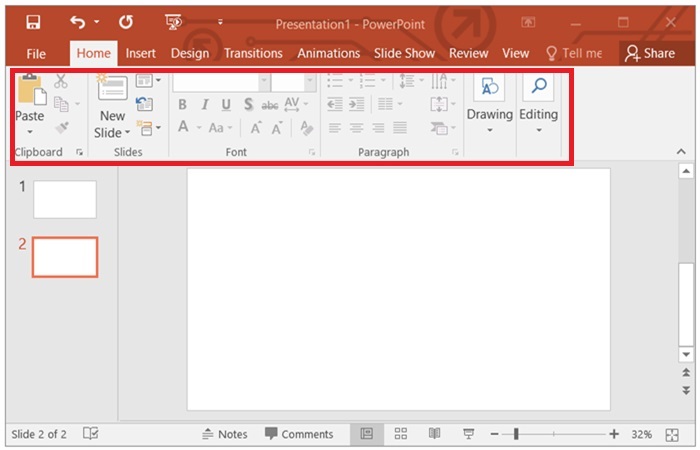
ToolBar in JavaFX
在 JavaFX 中,工具栏由 javafx.scene.control 类表示。此类是名为 @{s15} 的包的一部分。通过实例化此类,可以在 JavaFX 中创建一个 ToolBar 节点。它具有以下构造函数:
-
ToolBar() - 它是用于创建不带任何预定义节点的工具栏的默认构造函数。
-
ToolBar(Node nodes) - 它是由 ToolBar 类带参数的构造函数,可创建一个带指定节点的新工具栏。
Steps to create a tool bar in JavaFX
按照以下步骤在 JavaFX 中创建一个工具栏。
Step 1: Create nodes to display within the ToolBar
首先,我们需要创建一个要在 ToolBar 中显示的节点列表,其中,每个节点代表一个不同的命令或函数。在这里,我们将通过使用以下代码块创建按钮、分隔符和文本字段 -
// Creating buttons and a text field
Button btnNew = new Button("New");
Button btnOpen = new Button("Open");
Button btnSave = new Button("Save");
Button btnExit = new Button("Exit");
TextField txtSearch = new TextField();
txtSearch.setPromptText("Search");
// creating separators
Separator sepOne = new Separator();
Separator sepTwo = new Separator();
Step 2: Instantiate the ToolBar class
如前所述,工具栏是使用名为 ToolBar 的类创建的。因此,如以下代码块所示实例化此类 -
// creating a toolbar
ToolBar toolsB = new ToolBar();
Step 3: Add the nodes to the ToolBar object
要将所有节点添加到 ToolBar,我们使用 getItems() 和 addAll() 方法。以下给出的代码对此进行了演示 -
// adding the nodes
toolsB.getItems().addAll(btnNew, btnOpen, btnSave, sepOne, txtSearch, sepTwo, btnExit);
Step 4: Launching Application
创建工具栏并添加所有节点后,按照以下给定的步骤正确启动应用程序 -
-
首先,通过将其 ToolBar 对象作为其构造函数的参数值传递来实例化名为 BorderPane 的类。但是,我们可以使用任何布局窗格,例如 GridPane 或 StackPane。
-
然后,通过将 BorderPane 对象作为参数值传递给其构造函数,来实例化名为 Scene 的类。我们也可以将应用程序屏幕尺寸作为可选参数传递给此构造函数。
-
然后,使用 Stage 类的 setTitle() 方法设置阶段标题。
-
现在,使用名为 Stage 的类的 setScene() 方法将 Scene 对象添加到阶段。
-
使用名为 show() 的方法显示场景的内容。
-
最后,借助 launch() 方法启动应用程序。
Example
以下是将使用 JavaFX 创建 ToolBar 的程序。将此代码保存在名为 ToolbarExample.java 的文件中。
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Separator;
import javafx.scene.control.TextField;
import javafx.scene.control.ToolBar;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class ToolbarExample extends Application {
@Override
public void start(Stage stage) throws Exception {
// Creating buttons and a text field
Button btnNew = new Button("New");
Button btnOpen = new Button("Open");
Button btnSave = new Button("Save");
Button btnExit = new Button("Exit");
TextField txtSearch = new TextField();
txtSearch.setPromptText("Search");
// creating separators
Separator sepOne = new Separator();
Separator sepTwo = new Separator();
// creating a toolbar
ToolBar toolsB = new ToolBar();
// adding the nodes
toolsB.getItems().addAll(btnNew, btnOpen, btnSave, sepOne, txtSearch, sepTwo, btnExit);
// Creating a BorderPane as root
BorderPane root = new BorderPane();
// adding the ToolBar at the top
root.setTop(toolsB);
// Create a Scene and set it to the Stage
Scene scene = new Scene(root, 400, 300);
stage.setTitle("ToolBar in JavaFX");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
使用以下命令从命令提示符处编译并执行已保存的 Java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls ToolbarExample.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls ToolbarExample
执行以上程序后,会生成一个 JavaFX 窗口,显示带有指定按钮和文本字段的 ToolBar,如下所示。
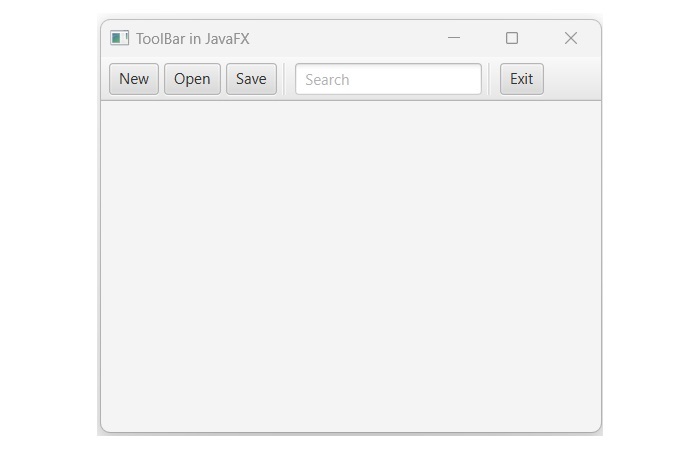
Setting the Orientation of the ToolBar
要更改 ToolBar 的方向,我们可以使用 setOrientation() 方法并传递 Orientation.HORIZONTAL 或 Orientation.VERTICAL 作为参数。
Example
在以下 JavaFX 程序中,我们将创建一个垂直 ToolBar。将此代码保存在名为 VerticalToolbar.java 的文件中。
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Separator;
import javafx.scene.control.TextField;
import javafx.scene.control.ToolBar;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
import javafx.geometry.Orientation;
public class VerticalToolbar extends Application {
@Override
public void start(Stage stage) throws Exception {
// Creating buttons
Button btnNew = new Button("New");
Button btnOpen = new Button("Open");
Button btnSave = new Button("Save");
Button btnExit = new Button("Exit");
// creating separators
Separator sepOne = new Separator();
Separator sepTwo = new Separator();
Separator sepThree = new Separator();
// creating a toolbar
ToolBar toolsB = new ToolBar();
// adding the nodes
toolsB.getItems().addAll(btnNew, sepOne, btnOpen, sepTwo, btnSave, sepThree, btnExit);
toolsB.setOrientation(Orientation.VERTICAL);
// Creating a BorderPane as root
BorderPane root = new BorderPane();
// adding the ToolBar at the top
root.setTop(toolsB);
// Create a Scene and set it to the Stage
Scene scene = new Scene(root, 400, 300);
stage.setTitle("ToolBar in JavaFX");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
使用以下命令从命令提示符处编译并执行已保存的 Java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls VerticalToolbar.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls VerticalToolbar
当我们执行上述代码时,它将生成以下输出。
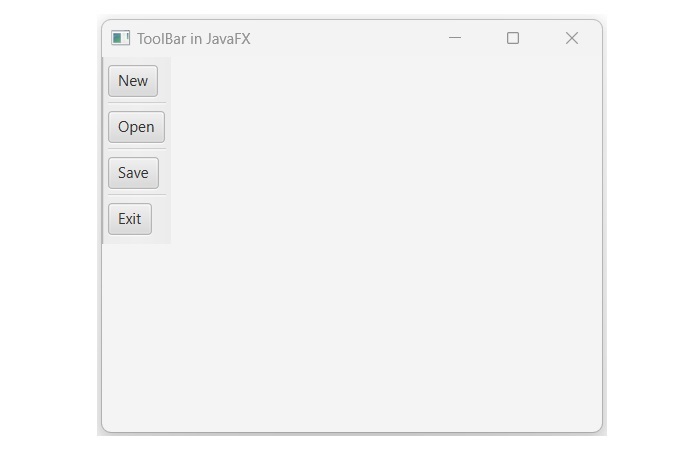