Matplotlib 简明教程
Matplotlib - Annotations
在 Matplotlib 库中,注释是指在绘图中的特定位置添加文本或标记的能力,以提供其他信息或突出显示特定功能。注释允许用户标记数据点并指示趋势或向绘图的不同部分添加描述。
Key Aspects of Annotations in Matplotlib
以下是 matplotlib 库中注释的关键方面。
Marker Annotations
数据可视化中的标记注释通常涉及在绘图中感兴趣的特定点上放置标记或符号,以突出显示或提供有关这些点的其他信息。这些注释可以是文本性的或图形性的,通常用于引起人们对重要的数据点、峰值、波谷、异常值或可视表示中的任何关键信息的注意。
Callouts
数据可视化中的旁注是指一种特殊的注释类型,它使用箭头、线条或文本等视觉元素来引起对绘图中某一特定区域或特征的注意。它们通常用于提供有关特定数据点或感兴趣区域的附加上下文或解释。
Matplotlib 库中的 plt.annotate() 函数用于向绘图添加注释。它允许我们在绘图上放置文本注释,并带可选箭头,指向绘图上的特定数据点。
Syntax
以下是 plt.annotate() 函数的语法。
plt.annotate(text, xy, xytext=None, xycoords='data', textcoords='data', arrowprops=None, annotation_clip=None, kwargs)
其中,
-
text (str) − 注释的文本。
-
xy (tuple or array) − 要注释的点 (x, y)。
-
xytext (tuple or array, optional) − 放置文本的位置 (x, y)。如果 None 默认为
xy
。 -
xycoords (str, Artist, or Transform, optional) − 提供
xy
的坐标系。默认为“数据”。 -
textcoords (str, Artist, or Transform, optional) - 其中
xytext
提供坐标系。默认情况下为“数据”。 -
arrowprops (dict, optional) - 箭头属性字典。如果不是 None,则从注释绘制一条箭头到文本。
-
annotation_clip (bool or None, optional) - 如果为 True ,仅当注释点在坐标轴内时才绘制文本。如果为 None ,则它将获取 rcParams["text.clip"] 中的值。
-
kwargs (optional) - 传递给 Text 的其他关键字参数。
Adding the annotation to the plot
在此示例中,我们使用 plt.annotate() 函数在绘图中点 (3, 5) 添加包含文本 'Peak' 的注释。
import matplotlib.pyplot as plt
# Plotting data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.plot(x, y, marker='o', linestyle='-', color='blue')
# Adding annotation
plt.annotate('Peak', xy=(3, 5), xytext=(3.5, 6), arrowprops=dict(facecolor='black', arrowstyle='->'), fontsize=10)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Annotated Plot')
plt.grid(True)
plt.show()
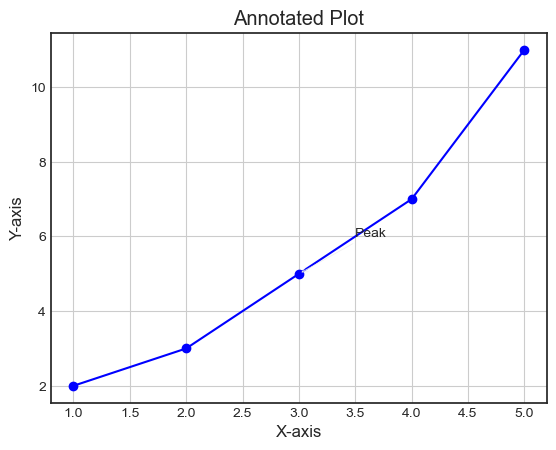
以下是使用 ‘plt.annotations’ 函数将注释添加到图像的另一个示例。
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# Plotting data
plt.plot(x, y, marker='+', linestyle='-')
# Adding an annotation
plt.annotate('Point of Interest', xy=(3, 6), xytext=(3.5, 7), arrowprops=dict(facecolor='black', arrowstyle='->'))
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Annotated Plot')
plt.grid(True)
plt.show()

Insert statistical annotations (stars or p-values)
在此示例中,我们插入了带有星形 r p 值的统计注释。
import numpy as np
from matplotlib import pyplot as plt
plt.rcParams["figure.figsize"] = [7.00, 3.50]
plt.rcParams["figure.autolayout"] = True
x = np.linspace(-1, 1, 5)
y = np.linspace(-2, 2, 5)
mean_x = np.mean(x)
mean_y = np.mean(y)
fig, ax = plt.subplots()
ax.plot(x, y, linestyle='-.')
ax.annotate('*', (mean_y, mean_y), xytext=(-.50, 1), arrowprops=dict(arrowstyle='-|>'))
fig.autofmt_xdate()
plt.show()
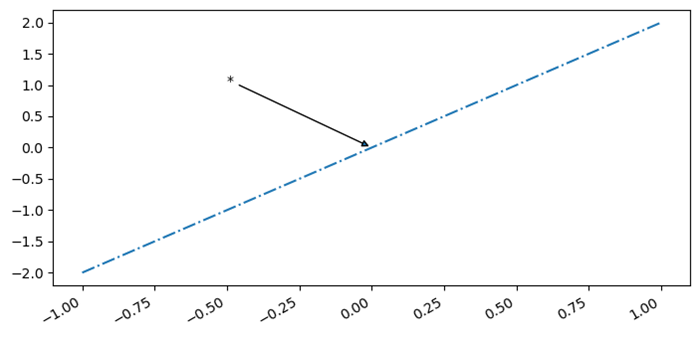
Annotate Matplotlib Scatter Plots
在此示例中,我们向已经绘制好的散点图添加注释。
# Import necessary libraries
import matplotlib.pyplot as plt
import numpy as np
# Create data points to be plotted
x = np.random.rand(30)
y = np.random.rand(30)
# Define the scatter plot using Matplotlib
fig, ax = plt.subplots()
ax.scatter(x, y)
# Add annotations to specific data points using text or arrow annotations
ax.annotate('Outlier', xy=(0.9, 0.9), xytext=(0.7, 0.7),arrowprops=dict(facecolor='black', shrink=0.05))
ax.annotate('Important point', xy=(0.5, 0.3), xytext=(0.3, 0.1),arrowprops=dict(facecolor='red', shrink=0.05))
ax.annotate('Cluster of points', xy=(0.2, 0.5), xytext=(0.05, 0.7),arrowprops=dict(facecolor='green', shrink=0.05))
# Adjust the annotation formatting as needed
plt.title('Annotated Scatter Plot')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
# Show the scatter plot with annotations
plt.show()

Annotate the points on a scatter plot with automatically placed arrows
在此示例中,我们使用自动放置的箭头对散点图上的点进行注释。
import numpy as np
from matplotlib import pyplot as plt
plt.rcParams["figure.figsize"] = [7.00, 3.50]
plt.rcParams["figure.autolayout"] = True
xpoints = np.linspace(1, 10, 25)
ypoints = np.random.rand(25)
labels = ["%.2f" % i for i in xpoints]
plt.scatter(xpoints, ypoints, c=xpoints)
for label, x, y in zip(labels, xpoints, ypoints):
plt.annotate(
label,
xy=(x, y), xytext=(-20, 20),
textcoords='offset points', ha='right', va='bottom',
bbox=dict(boxstyle='round,pad=0.5', fc='yellow', alpha=0.5),
arrowprops=dict(arrowstyle='->', connectionstyle='arc3,rad=0')
)
plt.show()
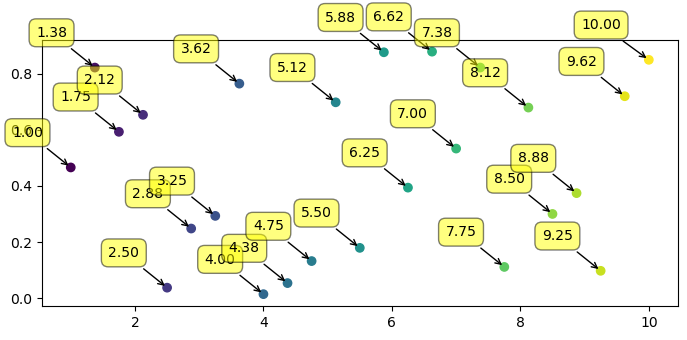