Matplotlib 简明教程
Matplotlib - Axis Ticks
What are Axis Ticks?
Matplotlib 中的轴刻度标记是指沿轴的标记,它们表示特定的数据值。它们有助于理解绘图的范围,并为数据可视化提供参考点。让我们深入了解轴刻度标记的详细信息 −
Key Concepts in Axis ticks
以下是由轴刻度标记提供的关键概念。
Major Ticks − 这些是沿轴的突出刻度,表示显著的数据值。
Minor Ticks − 这些是主要刻度之间的较小刻度,它们在刻度上提供了更精细的粒度,但通常不太突出。
Setting Ticks
可以用两种方法设置轴刻度线,一种是手动设置,另一种是自动调整。
Manual Setting
可以使用 plt.xticks() 或 plt.yticks() 函数为轴设置特定刻度线位置和标签。
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.plot(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Plot with Custom Axis Ticks')
# Customize x-axis ticks
plt.xticks([0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10], ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K'])
# Customize y-axis ticks and labels
plt.yticks([-1, 0, 1], ['Min', 'Zero', 'Max'])
plt.show()

Automatic Adjustment
在 Matplotlib 中,轴刻度线的自动调整涉及让程序库根据数据范围确定刻度线的标注和位置。当我们创建图形时,此进程默认处理,但我们可以使用各种格式化选项或调整定位器和格式设置来微调自动调整。以下是一些与轴刻度线自动调整相关的要点。
在此示例中,Matplotlib 根据数据范围自动调整刻度线的标注和位置。
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# Creating a plot (automatic adjustment of ticks)
plt.plot(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Automatic Adjustment of Axis Ticks')
plt.show()

Customizing Automatic Adjustment
我们可以使用 pyplot 中的一些函数自动自定义标注。可以针对我们数据的特性进行调整,以增强图形的可读性。
理解和利用轴刻度线的自动调整对于在 Matplotlib 中创建清晰且具有信息性的可视化至关重要。
Adjusting the Number of Ticks
我们可以使用 plt.locator_params(axis='x', nbins=5) 控件 x 轴上的刻度线数。调整设置为所需数字的参数 nbins 。
Scientific Notation
要以科学计数法显示刻度线标签,我们可以使用 plt.ticklabel_format(style='sci', axis='both', scilimits=(min, max)) 。
Date Ticks (For Time Series Data)
如果我们正在处理日期/时间数据,那么 Matplotlib 可以自动格式化日期刻度线。
在此示例中,我们将应用自定义自动调整到图形的轴刻度线。
import matplotlib.dates as mdates
from datetime import datetime
# Example date data
dates = [datetime(2022, 1, 1), datetime(2022, 2, 1), datetime(2022, 3, 1)]
# Creating a plot with automatic adjustment of date ticks
plt.plot(dates, [2, 4, 6])
# Formatting x-axis as date
plt.gca().xaxis.set_major_locator(mdates.MonthLocator())
plt.gca().xaxis.set_major_formatter(mdates.DateFormatter('%Y-%m-%d'))
plt.xlabel('Date')
plt.ylabel('Y-axis')
plt.title('Automatic Adjustment of Date Ticks')
plt.show()

Tick Formatting
我们可以使用 fontsize, color 和 rotation 参数基于字体大小、颜色和旋转自定义刻度线标签的外观。
Example
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# Creating a plot (automatic adjustment of ticks)
plt.plot(x, y)
plt.xticks(fontsize=10, color='red', rotation=45)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Automatic Adjustment of Axis Ticks')
plt.show()

Tick Frequency and Appearance
Setting Tick Frequency
我们可以使用 plt.locator_params(axis='x', nbins=5) 调整刻度线的频率,以控制显示的刻度线数。
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# Creating a plot (automatic adjustment of ticks)
plt.plot(x, y)
plt.locator_params(axis='x', nbins=10)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Automatic Adjustment of Axis Ticks')
plt.show()
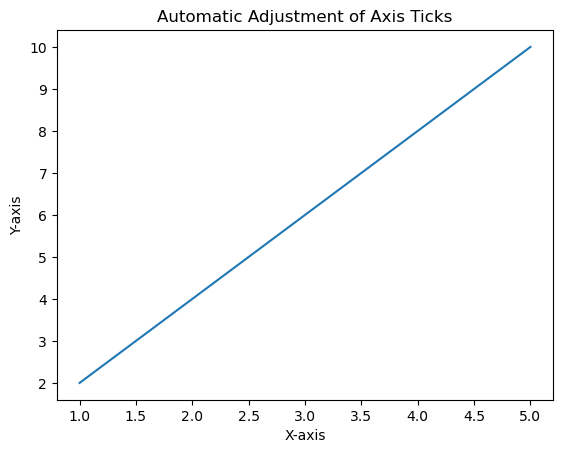
Minor Ticks
我们可以使用 plt.minorticks_on() 启用次要刻度线。我们可以使用 plt.minorticks_on(), plt.minorticks_off() 自行定制它们的外观或指定它们的位置。
Use Cases
Precision Control - 调整刻度线以提供有关数据值更精确的信息。
Enhanced Readability - 自行定制刻度线标签和外观,增强可读性。
Fine-tuning - 手动设置刻度线以强调特定数据点或区间。
了解和自定义轴刻度线对于在图形中有效传递信息至关重要,因为它允许我们根据可视化需要调整数据展示。
Customize X-axis ticks
在此示例中,为了添加其他刻度线,我们使用 xticks() 函数,并将刻度线的范围从 1 到 10 增加到 1 到 20。
Example
import numpy as np
import matplotlib.pyplot as plt
plt.rcParams["figure.figsize"] = [7.50, 3.50]
plt.rcParams["figure.autolayout"] = True
height = [3, 12, 5, 18, 45]
bars = ('A', 'B', 'C', 'D', 'E')
y_pos = np.arange(len(bars))
plt.bar(y_pos, height, color='yellow')
plt.tick_params(axis='x', colors='red', direction='out', length=7, width=2)
plt.show()

Remove the X-axis ticks while keeping the grids
要删除 X 刻度线同时保留网格,我们可以使用以下代码作为参考。
Example
import matplotlib.pyplot as plt
import numpy as np
plt.rcParams["figure.figsize"] = [7.00, 3.50]
plt.rcParams["figure.autolayout"] = True
x = np.linspace(0, 2*np.pi, 100)
ax = plt.gca()
ax.plot(x, np.sin(x), c='r', lw=5, label='y=sin(x)')
ax.set_xticklabels([])
ax.set_yticklabels([])
ax.grid(True)
plt.legend(loc="upper right")
plt.show()
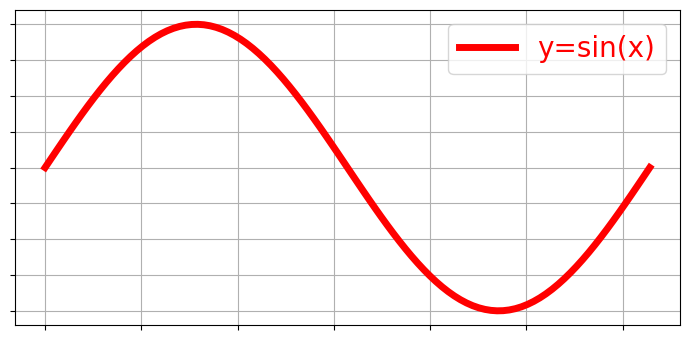
Turn off the ticks and marks
在此示例中,我们关闭 matplotlib 坐标轴的刻度线和标记,我们可以使用 set_tick_params() 隐藏 X 和 Y 坐标轴标记,set_xticks() 和 set_yticks() 隐藏 X 和 Y 坐标轴刻度线标记。
Example
import numpy as np
from matplotlib import pyplot as plt
# Set the figure size
plt.rcParams["figure.figsize"] = [7.00, 3.50]
plt.rcParams["figure.autolayout"] = True
# Create x and y data points
x = np.linspace(-10, 10, 100)
y = np.sin(x)
plt.plot(x, y)
ax = plt.gca()
# Hide X and Y axes label marks
ax.xaxis.set_tick_params(labelbottom=False)
ax.yaxis.set_tick_params(labelleft=False)
# Hide X and Y axes tick marks
ax.set_xticks([])
ax.set_yticks([])
plt.show()

Remove the digits after the decimal point in axis ticks
在此示例中,我们使用 x.astype(int) 函数仅将刻度线标记设置为数字。
Example
import matplotlib.pyplot as plt
import numpy as np
plt.rcParams["figure.figsize"] = [7.50, 3.50]
plt.rcParams["figure.autolayout"] = True
x = np.array([1.110, 2.110, 4.110, 5.901, 6.00, 7.90, 8.90])
y = np.array([2.110, 1.110, 3.110, 9.00, 4.001, 2.095, 5.890])
fig, ax = plt.subplots()
ax.plot(x, y)
ax.set_xticks(x.astype(int))
plt.show()
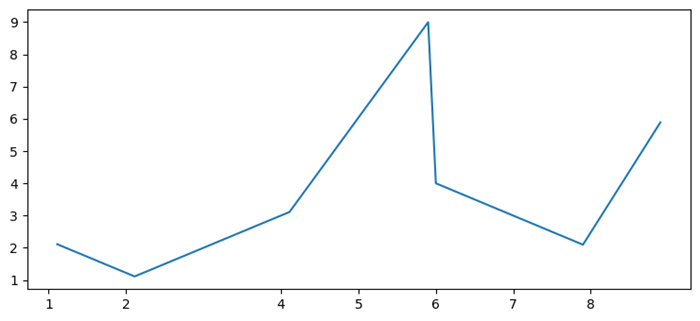
Add Matplotlib Colorbar Ticks
在此示例中,我们为一个 ScalarMappable 实例 mappable 创建一个颜色轴,其中参数 ticks=ticks 用于添加颜色轴刻度线。
Example
import numpy as np
import matplotlib.pyplot as plt
plt.rcParams["figure.figsize"] = [7.50, 3.50]
plt.rcParams["figure.autolayout"] = True
x, y = np.mgrid[-1:1:100j, -1:1:100j]
z = (x + y) * np.exp(-5.0 * (x ** 2 + y ** 2))
plt.imshow(z, extent=[-1, 1, -1, 1])
ticks = np.linspace(z.min(), z.max(), 5, endpoint=True)
cb = plt.colorbar(ticks=ticks)
plt.show()

Adjusting gridlines and ticks in Matplotlib imshow
在此示例中,我们使用 set_xticklabels 和 set_yticklabels 方法设置 x 刻度线标签和 y 刻度线标签。
Example
import numpy as np
from matplotlib import pyplot as plt
plt.rcParams["figure.figsize"] = [7.00, 3.50]
plt.rcParams["figure.autolayout"] = True
data = np.random.rand(9, 9)
plt.imshow(data, interpolation="nearest")
ax = plt.gca()
ax.set_xticks(np.arange(-.5, 9, 1))
ax.set_yticks(np.arange(-.5, 9, 1))
ax.set_xticklabels(np.arange(0, 10, 1))
ax.set_yticklabels(np.arange(0, 10, 1))
ax.grid(color='red', linestyle='-.', linewidth=1)
plt.show()
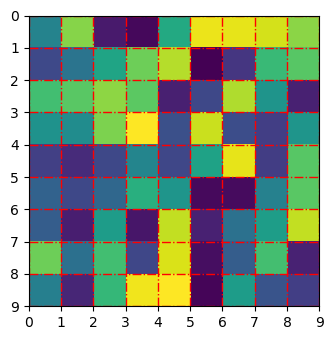
Move the Y-axis ticks from the left side of the plot to the right side
在此示例中,要将 Y 坐标轴刻度线从左向右移动,我们使用 ax.yaxis.tick_right()。
Add third level of ticks
此示例是添加第三级刻度线的参考。
Example
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.ticker
plt.rcParams["figure.figsize"] = [7.50, 3.50]
plt.rcParams["figure.autolayout"] = True
t = np.arange(0.0, 100.0, 0.1)
s = np.sin(0.1 * np.pi * t) * np.exp(-t * 0.01)
fig, ax = plt.subplots()
plt.plot(t, s)
ax1 = ax.twiny()
ax1.plot(t, s)
ax1.xaxis.set_ticks_position('bottom')
majors = np.linspace(0, 100, 6)
minors = np.linspace(0, 100, 11)
thirds = np.linspace(0, 100, 101)
ax.xaxis.set_major_locator(matplotlib.ticker.FixedLocator(majors))
ax.xaxis.set_minor_locator(matplotlib.ticker.FixedLocator(minors))
ax1.xaxis.set_major_locator(matplotlib.ticker.FixedLocator([]))
ax1.xaxis.set_minor_locator(matplotlib.ticker.FixedLocator(thirds))
ax1.tick_params(which='minor', length=2)
ax.tick_params(which='minor', length=4)
ax.tick_params(which='major', length=6)
ax.grid(which='both', axis='x', linestyle='--')
plt.axhline(color='gray')
plt.show()

Create minor ticks for a polar plot
此示例是添加第三级刻度线的参考。
Example
import numpy as np
import matplotlib.pyplot as plt
# Set the figure size
plt.rcParams["figure.figsize"] = [7.00, 3.50]
plt.rcParams["figure.autolayout"] = True
# radius and theta for the polar plot
r = np.arange(0, 5, 0.1)
theta = 2 * np.pi * r
# Add a subplot
ax = plt.subplot(111, projection='polar')
tick = [ax.get_rmax(), ax.get_rmax() * 0.97]
# Iterate the points between 0 to 360 with step=10
for t in np.deg2rad(np.arange(0, 360, 10)):
ax.plot([t, t], tick, lw=1, color="red")
# Display the plot
plt.show()

Overlapping Y-axis tick label and X-axis tick label
在此示例中,我们减少了 matplotlib 中 x 和 y 刻度线标签重叠的机会。
Example
import matplotlib.pyplot as plt
from matplotlib.ticker import MaxNLocator
import numpy as np
plt.rcParams["figure.figsize"] = [7.00, 3.50]
plt.rcParams["figure.autolayout"] = True
xs = np.linspace(0, 5, 10)
ys = np.linspace(0, 5, 10)
plt.subplot(121)
plt.margins(x=0, y=0)
plt.plot(xs, ys)
plt.title("Overlapping")
plt.subplot(122)
plt.margins(x=0, y=0)
plt.plot(xs, ys)
plt.title("Non overlapping")
plt.gca().xaxis.set_major_locator(MaxNLocator(prune='lower'))
plt.gca().yaxis.set_major_locator(MaxNLocator(prune='lower'))
plt.show()

Disable the minor ticks of a log-plot
此示例通过使用 minorticks_off() 函数禁用 matplotlib 中对数图的次要刻度线。
Example
import numpy as np
from matplotlib import pyplot as plt
plt.rcParams["figure.figsize"] = [7.50, 3.50]
plt.rcParams["figure.autolayout"] = True
x = np.random.randint(-3, 3, 10)
y = np.exp(x)
plt.subplot(121)
plt.plot(y, x, c='red')
plt.xscale('log')
plt.title("With minor ticks")
plt.subplot(122)
plt.plot(y, x, c='green')
plt.xscale('log')
plt.minorticks_off()
plt.title("Without minor ticks")
plt.show()
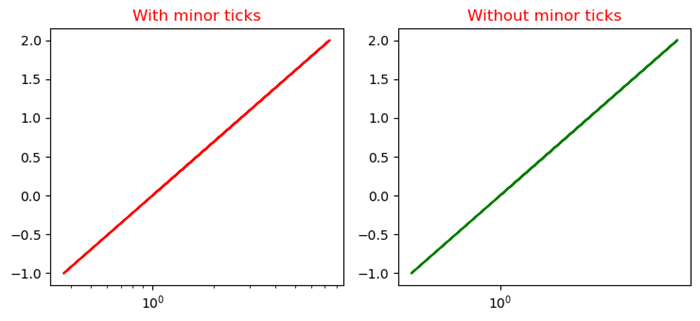