Matplotlib 简明教程
Matplotlib - Figures
在 Matplotlib 库中,图形是容纳绘图或可视化所有元素的顶级容器。可以将它视为创建绘图的窗口或画布。单个图形可以包含多个子图,即轴、标题、标签、图例和其他元素。Matplotlib 中的 figure() 函数用于创建新图形。
In Matplotlib library a figure is the top-level container that holds all elements of a plot or visualization. It can be thought of as the window or canvas where plots are created. A single figure can contain multiple subplots i.e. axes, titles, labels, legends and other elements. The figure() function in Matplotlib is used to create a new figure.
Syntax
以下是 figure() 方法的语法和参数。
The below is the syntax and parameters of the figure() method.
plt.figure(figsize=(width, height), dpi=resolution)
其中,
Where,
-
figsize=(width, height) − Specifies the width and height of the figure in inches. This parameter is optional.
-
dpi=resolution − Sets the resolution or dots per inch for the figure. Optional and by default is 100.
Creating a Figure
要创建使用 figure() 方法的图形,我们必须将图形大小和分辨率值作为输入参数传递。
To create the Figure by using the figure() method we have to pass the figure size and resolution values as the input parameters.
Adding Plots to a Figure
创建图形后,我们可以使用 matplotlib 中的 plot() 或 subplot() 函数在图形内添加绘图或子图(轴)。
After creating a figure we can add plots or subplots (axes) within that figure using various plot() or subplot() functions in Matplotlib.
Displaying and Customizing Figures
要显示和自定义图形,我们有 plt.show(),plt.title(), plt.xlabel(), plt.ylabel(), plt.legend() 函数。
To display and customize the figures we have the functions plt.show(),plt.title(), plt.xlabel(), plt.ylabel(), plt.legend().
plt.show()
这个函数以所添加的所有绘图和元素显示图形。
This function displays the figure with all the added plots and elements.
Customization
我们可以自定义图形,例如使用 plt.title(), plt.xlabel(), plt.ylabel(), plt.legend() 等函数为图形添加标题、标签、图例和其他元素。
We can perform customizations such as adding titles, labels, legends and other elements to the figure using functions like plt.title(), plt.xlabel(), plt.ylabel(), plt.legend() etc.
在这个例子中,我们使用pyplot模块的 figure() 方法通过将 figsize 作为 (8,6) 和 dpi 作为 100 来创建一个具有线图的图形,并包括自定义选项,例如标题、标签和图例。图形可以包含多个绘图或子图,从而允许在单个窗口内进行复杂的可视化。
In this example we are using the figure() method of the pyplot module by passing the figsize as (8,6) and dpi as 100 to create a figure with a line plot and includes customization options such as a title, labels and a legend. Figures can contain multiple plots or subplots allowing for complex visualizations within a single window.
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# Create a figure
plt.figure(figsize=(8, 6), dpi=100)
# Add a line plot to the figure
plt.plot(x, y, label='Line Plot')
# Customize the plot
plt.title('Figure with Line Plot')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
# Display the figure
plt.show()
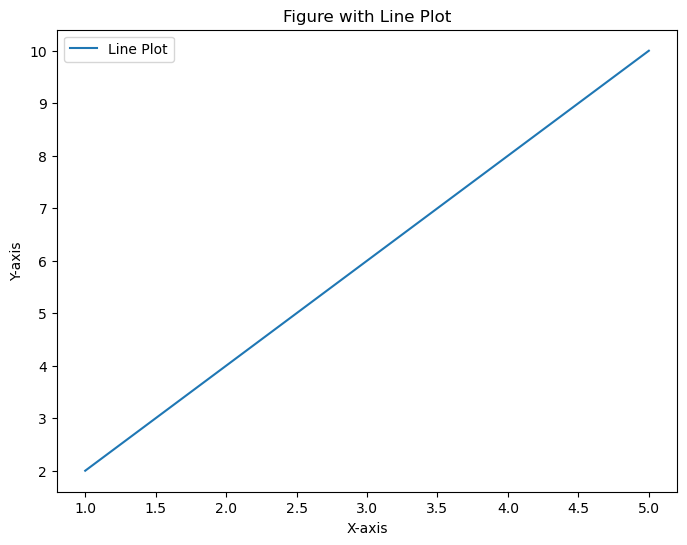
这里还有另一个使用matplotlib模块的 figure() 方法来创建子图的示例。
Here this is another example of using the figure() method of the pyplot module to create the subplots.
import matplotlib.pyplot as plt
# Create a figure
plt.figure(figsize=(8, 6))
# Add plots or subplots within the figure
plt.plot([1, 2, 3], [2, 4, 6], label='Line 1')
plt.scatter([1, 2, 3], [3, 5, 7], label='Points')
# Customize the figure
plt.title('Example Figure')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
# Display the figure
plt.show()
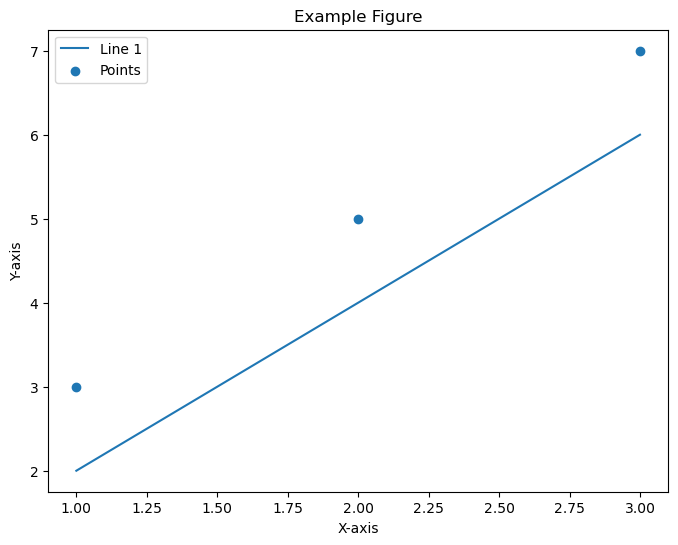