Matplotlib 简明教程
Matplotlib - Multiplots
在本章中,我们将学习如何在同一画布上创建多个子图。
subplot() 函数返回给定网格位置处的Axes对象。此函数的调用签名为:
plt.subplot(subplot(nrows, ncols, index)
在当前图形中,该函数创建一个Axes对象,并将其返回到nrows by ncolsaxes网格的位置索引处。索引从1到nrows * ncols,以行优先顺序递增。如果nrows、ncols和index均小于10。索引也可以表示为单个、连接的、三位数字。
例如,subplot(2, 3, 3)和subplot(233)都在当前图形的右上角创建一个Axes,占据图形高度的一半和图形宽度的三分之一。
创建子图将删除其与超出共享边界的任何预先存在的子图重叠。
import matplotlib.pyplot as plt
# plot a line, implicitly creating a subplot(111)
plt.plot([1,2,3])
# now create a subplot which represents the top plot of a grid with 2 rows and 1 column.
#Since this subplot will overlap the first, the plot (and its axes) previously
created, will be removed
plt.subplot(211)
plt.plot(range(12))
plt.subplot(212, facecolor='y') # creates 2nd subplot with yellow background
plt.plot(range(12))
上述代码行生成以下输出 -
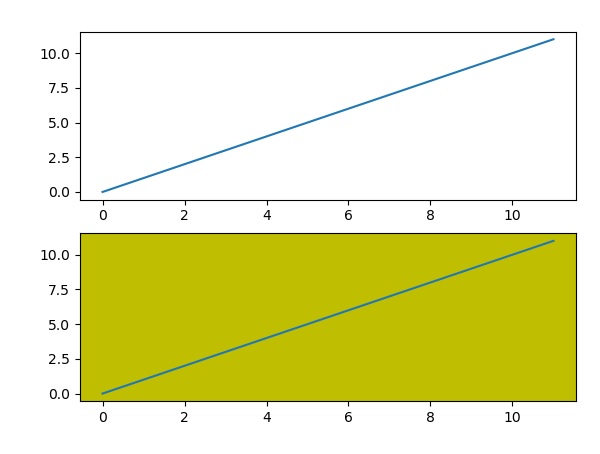
图形类的add_subplot()函数不会覆盖现有绘图:
import matplotlib.pyplot as plt
fig = plt.figure()
ax1 = fig.add_subplot(111)
ax1.plot([1,2,3])
ax2 = fig.add_subplot(221, facecolor='y')
ax2.plot([1,2,3])
当执行上述代码行时,它生成以下输出:

您可以通过在同一图形画布中添加另一个Axes对象,在同一图形中添加一个插入绘图。
import matplotlib.pyplot as plt
import numpy as np
import math
x = np.arange(0, math.pi*2, 0.05)
fig=plt.figure()
axes1 = fig.add_axes([0.1, 0.1, 0.8, 0.8]) # main axes
axes2 = fig.add_axes([0.55, 0.55, 0.3, 0.3]) # inset axes
y = np.sin(x)
axes1.plot(x, y, 'b')
axes2.plot(x,np.cos(x),'r')
axes1.set_title('sine')
axes2.set_title("cosine")
plt.show()
当执行上述代码行时,生成以下输出:
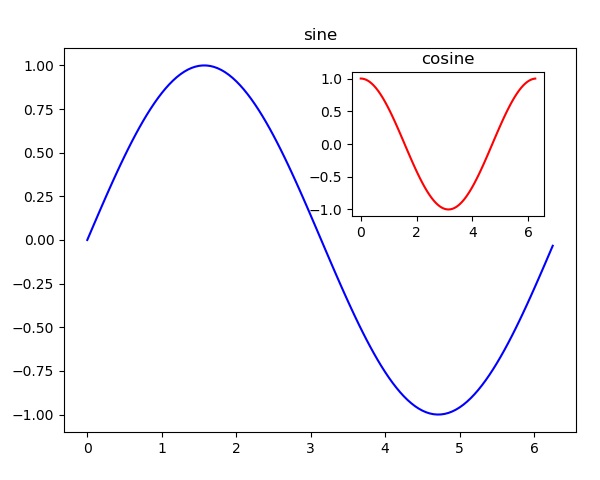