Pdfbox 简明教程
PDFBox - Inserting Image
在上一章中,我们已经了解如何从现有 PDF 文档中提取文本。在本章中,我们将讨论如何将图像插入 PDF 文档。
Inserting Image to a PDF Document
使用 PDImageXObject 类和 PDPageContentStream 类中的 createFromFile() 和 drawImage() 方法,可以将图像插入 PDF 文档。
以下是从现有 PDF 文档中提取文本的步骤。
Step 1: Loading an Existing PDF Document
使用 PDDocument 类的静态方法 load() 加载现有 PDF 文档。此方法接受一个文件对象作为参数,因为这是一个静态方法,您可使用类名调用它,如下所示:
File file = new File("path of the document")
PDDocument doc = PDDocument.load(file);
Step 3: Creating PDImageXObject object
PDFBox 库中的 PDImageXObject 类表示一张图片。它提供了执行图片相关操作所需的所有方法,例如插入图片、设置其高度、设置其宽度等。
我们可以使用 createFromFile() 方法创建此类的对象。我们需要向此方法传递作为字符串的要添加图片的路径和要向其中添加图片的文档对象。
PDImageXObject pdImage = PDImageXObject.createFromFile("C:/logo.png", doc);
Step 4: Preparing the Content Stream
您可以使用名为 PDPageContentStream 的类的对象插入各种数据元素。您需要将文档对象和页面对象传递给此类的构造函数,因此,按如下所示传递在先前步骤中创建的这两个对象来实例化此类。
PDPageContentStream contentStream = new PDPageContentStream(doc, page);
Step 5: Drawing the Image in the PDF Document
可以使用 drawImage() 方法在 PDF 文档中插入图片。需要向此方法添加在上一步创建的图片对象和图片所需尺寸(宽度和高度),如下所示。
contentstream.drawImage(pdImage, 70, 250);
Example
假设我们有一个名为 sample.pdf 的 PDF 文档,路径为 C:/PdfBox_Examples/ ,空白页如下所示。
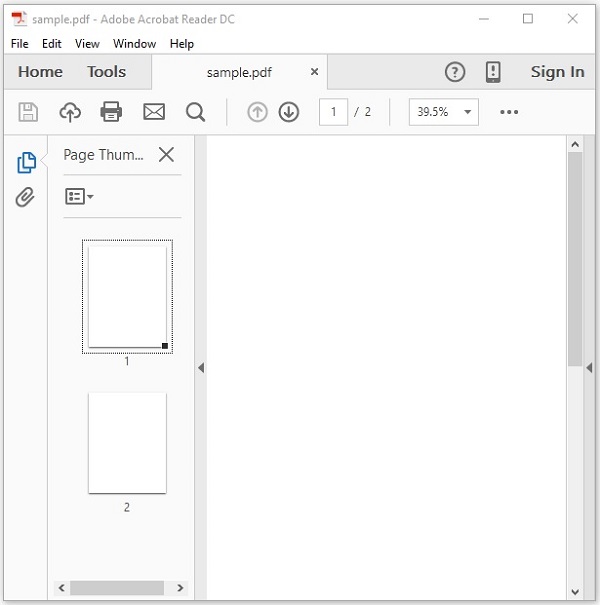
此示例演示如何将图片添加到上述 PDF 文档的空白页。此处,我们将加载名为 sample.pdf 的 PDF 文档并向其中添加图片。使用名称 InsertingImage.java. 将此代码保存在一个文件中
import java.io.File;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.PDPage;
import org.apache.pdfbox.pdmodel.PDPageContentStream;
import org.apache.pdfbox.pdmodel.graphics.image.PDImageXObject;
public class InsertingImage {
public static void main(String args[]) throws Exception {
//Loading an existing document
File file = new File("C:/PdfBox_Examples/sample.pdf");
PDDocument doc = PDDocument.load(file);
//Retrieving the page
PDPage page = doc.getPage(0);
//Creating PDImageXObject object
PDImageXObject pdImage = PDImageXObject.createFromFile("C:/PdfBox_Examples/logo.png",doc);
//creating the PDPageContentStream object
PDPageContentStream contents = new PDPageContentStream(doc, page);
//Drawing the image in the PDF document
contents.drawImage(pdImage, 70, 250);
System.out.println("Image inserted");
//Closing the PDPageContentStream object
contents.close();
//Saving the document
doc.save("C:/PdfBox_Examples/sample.pdf");
//Closing the document
doc.close();
}
}
使用以下命令从命令提示符处编译并执行已保存的 Java 文件。
javac InsertingImage.java
java InsertingImage
执行后,以上程序将图片插入到指定页的给定 PDF 文档,并显示以下消息。
Image inserted
验证文档 sample.pdf ,您可以看到其中插入的图片如下所示。
