Php 简明教程
PHP - AJAX Search
AJAX 是术语异步 JavaScript 和 XML 的缩写。Ajax 用于构建快速而动态的网页。下面的示例演示了如何使用 AJAX 函数与后端 PHP 脚本交互,以便在网页上提供一个搜索字段。
AJAX is a shortform of the term Asynchronous JavaScript and XML. Ajax is used to build fast and dynamic web pages. Below example demonstrates interaction with the backend PHP script with AJAX functions to provide a search field on the webpage.
Step 1
将以下脚本另存为“example.php”−
Save the following script as "example.php" −
<html>
<head>
<style>
span {
color: green;
}
</style>
<script>
function showHint(str) {
if (str.length == 0) {
document.getElementById("txtHint").innerHTML = "";
return;
} else {
var xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (xmlhttp.readyState == 4 && xmlhttp.status == 200) {
document.getElementById("txtHint").innerHTML = xmlhttp.responseText;
}
}
xmlhttp.open("GET", "hello.php?q=" + str, true);
xmlhttp.send();
}
}
</script>
</head>
<body>
<p><b>Search your favourite tutorials:</b></p>
<form>
<input type = "text" onkeyup = "showHint(this.value)">
</form>
<p>Entered Course name: <span id="txtHint"></span></p>
</body>
</html>
此代码本质上是一个 HTML 脚本,该脚本呈现一个带有文本字段的 HTML 表单。在其 onkeyup 事件中,调用 showHint() JavaScript 函数。函数向服务器上的另一个 PHP 脚本发送 HTTP GET 请求。
This code is essentially a HTML script that renders a HTML form with a text field. On its onkeyup event, a showHint() JavaScript function is called. The function sends a HTTP GET request to another PHP script on the server.
Step 2
把以下脚本另存为“php_ajax.php”−
Save the following script as "php_ajax.php" −
<?php
// Array with names
$a[] = "Android";
$a[] = "B programming language";
$a[] = "C programming language";
$a[] = "D programming language";
$a[] = "euphoria";
$a[] = "F#";
$a[] = "GWT";
$a[] = "HTML5";
$a[] = "ibatis";
$a[] = "Java";
$a[] = "K programming language";
$a[] = "Lisp";
$a[] = "Microsoft technologies";
$a[] = "Networking";
$a[] = "Open Source";
$a[] = "Prototype";
$a[] = "QC";
$a[] = "Restful web services";
$a[] = "Scrum";
$a[] = "Testing";
$a[] = "UML";
$a[] = "VB Script";
$a[] = "Web Technologies";
$a[] = "Xerox Technology";
$a[] = "YQL";
$a[] = "ZOPL";
$q = $_REQUEST["q"];
$hint = "";
if ($q !== "") {
$q = strtolower($q);
$len = strlen($q);
foreach($a as $name) {
if (stristr($q, substr($name, 0, $len))) {
if ($hint === "") {
$hint = $name;
} else {
$hint .= ", $name";
}
}
}
}
echo $hint === "" ? "Please enter a valid course name" : $hint;
?>
Step 3
我们将通过输入网址 http://localhost/example.php 在浏览器中打开 example.php 来启动此应用程序
We will start this application by opening example.php in the browser by entering the URL http://localhost/example.php
在搜索字段中每次击键时,一个 GET 请求都会发送到服务器。服务器脚本从 $_REQUEST 数组中读取字符并搜索与其匹配的课程名称。匹配的值显示在文本字段下方的浏览器中。
On every keystroke in the search field, a GET request goes to the server. The server script reads the character from $_REQUEST array and searches for the course name that matches. The matched value is displayed below the text field in the browser.
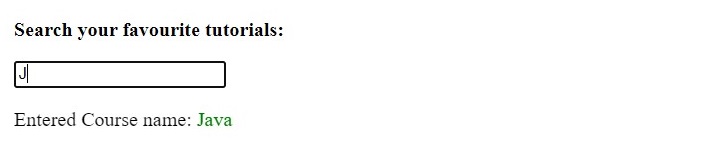