Pybrain 简明教程
PyBrain - Reinforcement Learning Module
强化学习(RL)是机器学习中一个重要部分。强化学习根据来自环境的输入使智能体学习自己的行为。
强化过程中相互交互的组件如下:
-
Environment
-
Agent
-
Task
-
Experiment
强化学习的布局如下:
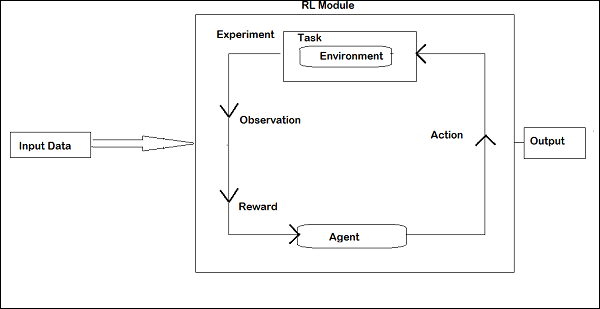
在 RL 中,智能体以迭代方式与环境对话。在每个迭代中,智能体接收具有奖励的观察结果。然后它选择动作并将其发送到环境中。环境在每次迭代中移动到新的状态,并且每次收到的奖励都会被保存。
RL 智能体的目标是尽可能多地收集奖励。在迭代之间,智能体的表现与表现优良的智能体进行比较,并且表现差异会引起奖励或失败。RL 主要用于问题解决任务,如机器人控制、电梯、电信、游戏等。
让我们看看如何在 Pybrain 中使用 RL。
我们将处理迷宫 environment ,它将使用 2 维 numpy 数组表示,其中 1 是墙壁,0 是自由场。智能体的责任是在自由场上移动并找到目标点。
以下是处理迷宫环境的分步流程。
Step 1
使用以下代码导入我们需要的包:
from scipy import *
import sys, time
import matplotlib.pyplot as pylab # for visualization we are using mathplotlib
from pybrain.rl.environments.mazes import Maze, MDPMazeTask
from pybrain.rl.learners.valuebased import ActionValueTable
from pybrain.rl.agents import LearningAgent
from pybrain.rl.learners import Q, QLambda, SARSA #@UnusedImport
from pybrain.rl.explorers import BoltzmannExplorer #@UnusedImport
from pybrain.rl.experiments import Experiment
from pybrain.rl.environments import Task
Step 2
使用以下代码创建迷宫环境:
# create the maze with walls as 1 and 0 is a free field
mazearray = array(
[[1, 1, 1, 1, 1, 1, 1, 1, 1],
[1, 0, 0, 1, 0, 0, 0, 0, 1],
[1, 0, 0, 1, 0, 0, 1, 0, 1],
[1, 0, 0, 1, 0, 0, 1, 0, 1],
[1, 0, 0, 1, 0, 1, 1, 0, 1],
[1, 0, 0, 0, 0, 0, 1, 0, 1],
[1, 1, 1, 1, 1, 1, 1, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 1, 1, 1, 1, 1, 1, 1, 1]]
)
env = Maze(mazearray, (7, 7)) # create the environment, the first parameter is the
maze array and second one is the goal field tuple
Step 3
下一步是创建智能体。
智能体在 RL 中起着重要作用。它将使用 getAction() 和 integrateObservation() 方法与迷宫环境进行交互。
代理有一个控制器(负责将状态映射到动作)和一个学习器。
PyBrain 中的控制器像一个模块,输入的是状态,输出的是动作。
controller = ActionValueTable(81, 4)
controller.initialize(1.)
ActionValueTable 需要 2 个输入,即:状态和动作的数量。标准迷宫环境有 4 个动作:北、南、东、西。
现在我们要创建一个学习器。我们将使用 SARSA() 学习算法,让学习器与代理一起使用。
learner = SARSA()
agent = LearningAgent(controller, learner)
Step 4
这一步是将代理添加到环境中。
要将代理连接到环境中,我们需要一个特殊组件,称为任务。 task 的作用是在环境中寻找目标,以及代理如何通过动作获得奖励。
环境有它自己的任务。我们使用的迷宫环境有 MDPMazeTask 任务。MDP 指的是 “markov decision process” ,意思是代理知道自己在迷宫中的位置。环境将成为任务的参数。
task = MDPMazeTask(env)
Step 5
在将代理添加到环境中后,下一步是创建实验。
现在我们需要创建实验,以便任务和代理相互协调。
experiment = Experiment(task, agent)
现在我们将运行 1000 次实验,如下所示:
for i in range(1000):
experiment.doInteractions(100)
agent.learn()
agent.reset()
当执行以下代码时,环境将在代理和任务之间运行 100 次:
experiment.doInteractions(100)
在每次迭代之后,它会将一个新状态返回给任务,由任务决定将哪些信息和奖励传递给代理。我们准备在 for 循环中学习并重新设置代理之后绘制一张新表格。
for i in range(1000):
experiment.doInteractions(100)
agent.learn()
agent.reset()
pylab.pcolor(table.params.reshape(81,4).max(1).reshape(9,9))
pylab.savefig("test.png")
以下是完整代码:
Example
maze.py
from scipy import *
import sys, time
import matplotlib.pyplot as pylab
from pybrain.rl.environments.mazes import Maze, MDPMazeTask
from pybrain.rl.learners.valuebased import ActionValueTable
from pybrain.rl.agents import LearningAgent
from pybrain.rl.learners import Q, QLambda, SARSA #@UnusedImport
from pybrain.rl.explorers import BoltzmannExplorer #@UnusedImport
from pybrain.rl.experiments import Experiment
from pybrain.rl.environments import Task
# create maze array
mazearray = array(
[[1, 1, 1, 1, 1, 1, 1, 1, 1],
[1, 0, 0, 1, 0, 0, 0, 0, 1],
[1, 0, 0, 1, 0, 0, 1, 0, 1],
[1, 0, 0, 1, 0, 0, 1, 0, 1],
[1, 0, 0, 1, 0, 1, 1, 0, 1],
[1, 0, 0, 0, 0, 0, 1, 0, 1],
[1, 1, 1, 1, 1, 1, 1, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 1, 1, 1, 1, 1, 1, 1, 1]]
)
env = Maze(mazearray, (7, 7))
# create task
task = MDPMazeTask(env)
#controller in PyBrain is like a module, for which the input is states and
convert them into actions.
controller = ActionValueTable(81, 4)
controller.initialize(1.)
# create agent with controller and learner - using SARSA()
learner = SARSA()
# create agent
agent = LearningAgent(controller, learner)
# create experiment
experiment = Experiment(task, agent)
# prepare plotting
pylab.gray()
pylab.ion()
for i in range(1000):
experiment.doInteractions(100)
agent.learn()
agent.reset()
pylab.pcolor(controller.params.reshape(81,4).max(1).reshape(9,9))
pylab.savefig("test.png")