Python Pillow 简明教程
Python Pillow - Adding Borders to Images
向图像添加边框是图像处理中的一项常见任务。边框有助于勾勒内容、重点突出特定区域或添加装饰元素。在 Pillow(PIL)中, ImageOps 模块的 expand() 方法用于通过在图像周围添加边框或填充,来增加图像的尺寸。这有助于实现各种目的,例如添加边框、创建特定大小的画布或调整图像大小使其适合特定的宽高比。
The expand() method
expand() 方法可用于向图像添加边框并调整其尺寸,同时保持宽高比。我们可以自定义尺寸和边框颜色以满足我们的特定要求。
以下是 expand() 方法的基本语法:
PIL.Image.expand(size, fill=None)
其中,
-
size - 指定扩展图像的新尺寸(即宽度和高度)的元组。
-
fill (optional) - 一个可选的颜色值,用于填充边框区域。应将其指定为颜色元组 (R, G, B) 或表示颜色的整数值。
以下是本章所有示例中使用的输入图像。
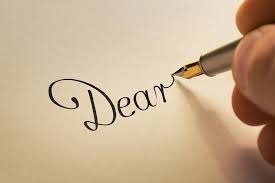
Example
在这个示例中,我们使用 expand() 方法创建带有边框的展开图像。
from PIL import Image, ImageOps
#Open an image
image = Image.open("Images/hand writing.jpg")
#Define the new dimensions for the expanded image
new_width = image.width + 40
#Add 40 pixels to the width
new_height = image.height + 40
#Add 40 pixels to the height
#Expand the image with a white border
expanded_image = ImageOps.expand(image, border=20, fill="red")
#Save or display the expanded image
expanded_image.save("output Image/expanded_output.jpg")
open_expand = Image.open("output Image/expanded_output.jpg")
open_expand.show()

Example
这里,我们使用 Image 模块的 expand() 方法通过蓝色来展开图像边框。
from PIL import Image, ImageOps
#Open an image
image = Image.open("Images/hand writing.jpg")
#Define the new dimensions for the expanded image
new_width = image.width + 40
#Add 40 pixels to the width
new_height = image.height + 40
#Add 40 pixels to the height
#Expand the image with a white border
expanded_image = ImageOps.expand(image, border=100, fill="blue")
#Save or display the expanded image
expanded_image.save("output Image/expanded_output.jpg")
open_expand = Image.open("output Image/expanded_output.jpg")
open_expand.show()
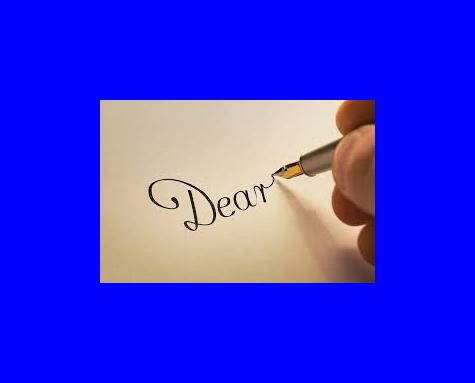