Python Pillow 简明教程
Python Pillow - Adding Padding to an Image
为图像添加填充涉及在图像周围添加一个边框。这是在不改变图像纵横比或裁剪图像的情况下调整图像大小时一项有用的技术。可以将填充添加到图像的顶部、底部、左侧和右侧。当处理边缘包含重要信息且想要保留这些信息以用于分割等任务的图像时,这尤其重要。
Adding padding to an image involves adding a border around it. This is a useful technique while adjusting the image’s size without changing its aspect ratio or trimming it. Padding can be added to the top, bottom, left, and right sides of the image. This is particularly important when working with images that have important information at the edges, which you want to retain for tasks like segmentation.
Pillow (PIL) 库在其 ImageOps 模块中提供了两个函数 pad() 和 expand() ,用于为图像添加填充。
The Pillow (PIL) library provides two functions, pad() and expand(), within its ImageOps module for adding padding to images.
Padding Images with ImageOps.pad() function
pad() 函数用于将图像调整大小并填充到指定的大小和纵横比。它允许您指定输出大小、重采样方法、背景颜色和原始图像在填充区域内的定位。此函数的语法如下 −
The pad() function is used to resize and pad an image to a specified size and aspect ratio. It allows you to specify the output size, resampling method, background color, and positioning of the original image within the padded area. Syntax of this function as follows −
PIL.ImageOps.pad(image, size, method=Resampling.BICUBIC, color=None, centering=(0.5, 0.5))
其中,
Where,
-
image − The image to resize and pad.
-
size − A tuple specifying the requested output size in pixels, in the format (width, height). The function will resize the image to this size while maintaining the aspect ratio.
-
method − This parameter determines the resampling method used during resizing. The default method is BICUBIC, which is a type of interpolation. You can specify other resampling methods supported by PIL. Common options include NEAREST, BILINEAR, and LANCZOS.
-
color − This parameter specifies the background color of the padded area. It supports the RGBA tuple also, like (R, G, B, A). If not specified, the default background color is black.
-
centering − This parameter controls the position of the original image within the padded version. It’s specified as a tuple with two values between 0 and 1.
Example
以下是一个使用 ImageOps.pad() 函数向图像添加填充的示例。
Here is an example that adds padding to an image using the ImageOps.pad() function.
from PIL import Image
from PIL import ImageOps
# Open the input image
input_image = Image.open('Images/elephant.jpg')
# Add padding to the image
image_with_padding = ImageOps.pad(input_image, (700, 300), color=(130, 200, 230))
# Display the input image
input_image.show()
# Display the image with the padding
image_with_padding.show()
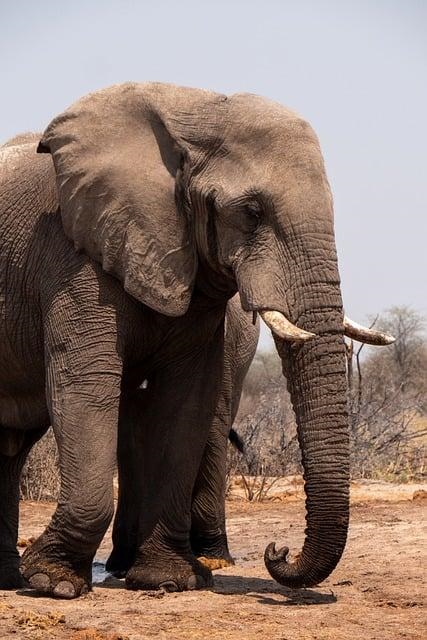
调整大小并填充后的输出图像 −
Output image After resizing with padding −
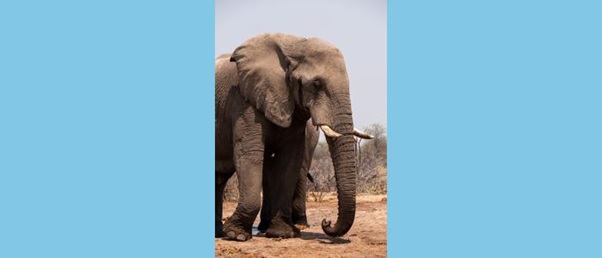
Adding Borders with the ImageOps.expand() function
expand() 函数在图像周围添加指定宽度和颜色的边框。它对于创建装饰性框架或强调图像内容很有用。其语法如下 −
The expand() function adds a border of a specified width and color around the image. It is useful for creating a decorative frame or emphasizing the content of the image. Its syntax is as follows −
PIL.ImageOps.expand(image, border=0, fill=0)
-
image − The image to expand the border.
-
border − The width of the border to add, specified in pixels. It determines how wide the border will be around the image. The default value is (0).
-
fill − The pixel fill value, which represents the color of the border. The default value is 0, which corresponds to black. You can specify the fill color using an appropriate color value.
Example
以下是一个使用 ImageOps.expand() 函数向图像添加填充的示例。
Here is an example that adds padding to an image using the ImageOps.expand() function.
from PIL import Image, ImageOps
# Open the input image
input_image = Image.open('Images/Car_2.jpg')
# Add padding of 15-pixel border
image_with_padding = ImageOps.expand(input_image, border=(15, 15, 15, 15), fill=(255, 180, 0))
# Display the input image
input_image.show()
# Display the output image with padding
image_with_padding.show()
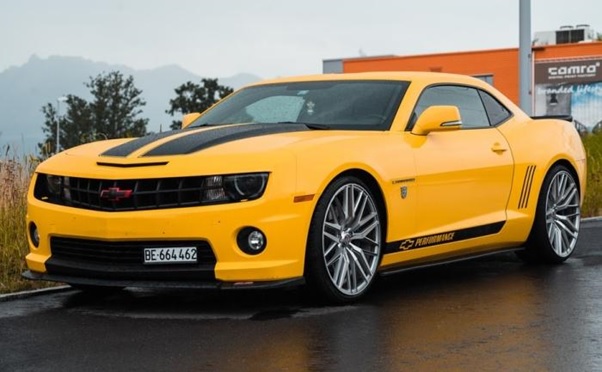
带填充的输出图像 −
Output image with padding −
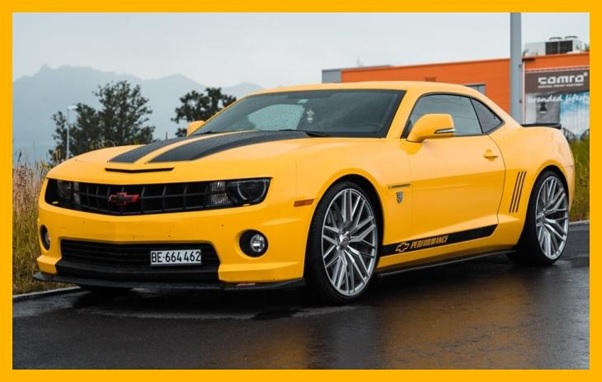