Python Pillow 简明教程
Python Pillow - Creating Thumbnails
缩略图通常用于显示图像预览或较小尺寸的原始图像。它们可用于优化网页并提高以图像为主的应用程序的加载速度。Pillow 提供了一种从图像生成缩略图的便捷方式。以下是 Pillow 中有关缩略图的一些要点。
-
Preservation of Aspect Ratio − 在创建缩略图时,Pillow 会保留原始图像的宽高比。这意味着缩略图的宽度和高度与原始图像成比例调整,这样图像就不会出现变形。
-
Reduced File Size − 与原始图像相比,缩略图的尺寸更小。缩小尺寸有助于优化网页或在画廊或列表等受限空间中显示图像。
-
Convenience − 它简化了创建缩略图的过程。它在保留宽高比的同时调整图像大小,并提供了将调整大小后的图像保存为文件的一种简单方式。
-
Quality Control − 我们可以使用各种参数控制缩略图的质量,例如尺寸、调整大小的滤镜类型和压缩设置(如果我们以 JPEG 等压缩格式保存缩略图)。
在 pillow 中,我们有名为 thumbnail() 的方法,它允许我们指定缩略图图像的尺寸和形状。我们可以创建两种不同形状的缩略图,一种是方形,另一种是圆形。
Creating square Thumbnails
在本章中,我们将看到如何使用 pillow 库的 thumbnail() 方法创建方形缩略图。
thumbnail() 方法的语法和参数如下。
image.thumbnail(size, resample=Image.BOX)
其中,
-
size (required) − 此参数指定尺寸,即缩略图的宽度和高度,以元组 (width, height) 的形式。我们也可以只指定一个尺寸,另一个尺寸将根据宽高比自动计算。
-
resample (optional) − 此参数定义调整图像大小时要使用的重采样滤镜。它可以是以下常量之一 - Image.NEAREST (default) − 最近邻采样。 Image.BOX − 盒形采样,与最近邻类似,但通常会产生更平滑的结果。 Image.BILINEAR − 双线性插值。 Image.HAMMING − 汉明窗正弦插值。 Image.BICUBIC − 三次样条插值。 Image.LANCZOS − Lanczos 窗正弦插值。
Example
在此示例中,我们通过使用 thumbnail() 方法创建方形缩略图,即将缩略图的宽度和高度参数指定为 resize 参数。
from PIL import Image
#Open the image
image = Image.open("Images/tutorialspoint.png")
#Define the thumbnail size as a tuple (width, height)
thumbnail_size = (200, 200)
#Create a thumbnail
image.thumbnail(thumbnail_size, resample = Image.BOX )
image.save("output Image/square_thumbnail_image.png")
image.show()

Example
以下是如何使用 thumbnail() 模块创建宽度为 100、高度为 100 的方形缩略图的另一个示例。
from PIL import Image
#Open the image
image = Image.open("Images/butterfly.jpg")
#Define the thumbnail size as a tuple (width, height)
thumbnail_size = (100, 100)
#Create a thumbnail
image.thumbnail(thumbnail_size, resample = Image.Resampling.BILINEAR)
image.save("output Image/square_thumbnail_image.png")
image.show()

Creating circle Thumbnails
在上文中,我们已经了解了什么是缩略图以及如何使用 pillow thumbnail() 方法创建方形缩略图。现在,我们将看到如何创建圆形缩略图。圆形缩略图表示缩略图将呈圆形。
thumbnail() 方法的语法和参数用于创建圆形缩略图与方形缩略图相同。
以下是创建圆形缩略图需要遵循的步骤。
-
从 Pillow 库中导入必要的模块 Image 和 ImageDraw 。
-
使用 Image.open() 方法加载原始图像。
-
使用 size 属性确定原始图像的尺寸。
-
使用 ImageDraw.Draw() 方法从蒙版图像创建一个新对象。
-
使用 draw.ellipse() 方法在蒙版图像上绘制一个椭圆。将图像居中对齐到椭圆的中心。
-
使用 Image.new() 方法创建一张新图像,其尺寸和原始图像相同,且背景透明。
-
使用 save() 方法保存圆形缩略图图像。
-
使用 show() 方法显示创建的圆形缩略图图像。
Example
在这个示例中,我们通过使用枕头库的 thumbnail() 方法来创建圆形缩略图。
#importing the required libraries
from PIL import Image, ImageDraw
#open image file
img = Image.open('Images/butterfly.jpg')
#resize image
img.thumbnail((2000, 2000))
#create circular mask
mask = Image.new('L', img.size, 0)
draw = ImageDraw.Draw(mask)
draw.ellipse((center[0] - radius, center[1] - radius, center[0] + radius, center[1] + radius), fill = 255)
#apply mask to image
result = Image.new('RGBA', img.size, (255, 255, 255, 0))
result.paste(img, (0, 0), mask)
#save circular thumbnail image
result.save('OutputImages/circular_thumbnail1.png')
#showing the image using show() function
result.show()
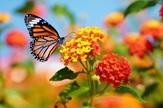
