Python Pyramid 简明教程
Python Pyramid - Hello World
Example
要检查 Pyramid 及其依赖项是否正确安装,请输入以下代码并使用任何支持 Python 的编辑器将其另存为 hello.py 。
To check whether Pyramid along with its dependencies are properly installed, enter the following code and save it as hello.py, using any Python-aware editor.
from wsgiref.simple_server import make_server
from pyramid.config import Configurator
from pyramid.response import Response
def hello_world(request):
return Response('Hello World!')
if __name__ == '__main__':
with Configurator() as config:
config.add_route('hello', '/')
config.add_view(hello_world, route_name='hello')
app = config.make_wsgi_app()
server = make_server('0.0.0.0', 6543, app)
server.serve_forever()
需要 Configurator 对象来定义 URL 路由并向其绑定视图函数。可从该 config 对象获取 WSGI 应用程序对象,该对象作为 make_server() 函数的参数,以及本地主机的 IP 地址和端口。当调用 serve_forever() 方法时,服务器对象会进入侦听循环。
The Configurator object is required to define the URL route and bind a view function to it. The WSGI application object is obtained from this config object is an argument to the make_server() function along with the IP address and port of localhost. The server object enters a listening loop when serve_forever() method is called.
在命令终端中以 as 运行该程序。
Run this program from the command terminal as.
Python hello.py
Output
WSGI 服务器开始运行。打开浏览器,并在地址栏中输入 [role="bare"] [role="bare"]http://loccalhost:6543/ 。当请求得到接受时, hello_world() 视图函数得到执行。它返回“Hello world”消息。“Hello world”消息将显示在浏览器窗口中。
The WSGI server starts running. Open the browser and enter [role="bare"]http://loccalhost:6543/ in the address bar. When the request is accepted, the hello_world() view function gets executed. It returns the Hello world message. The Hello world message will be seen in the browser window.
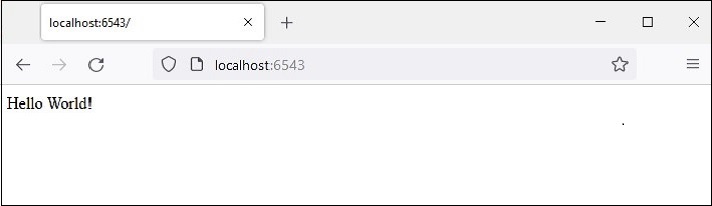
如同前面所述, wsgiref 模块中的 make_server() 函数创建的开发服务器不适合用于生产环境。因此,我们应当使用 Waitress 服务器。根据以下代码修改 hello.py −
As mentioned earlier, the development server created by make_server() function in the wsgiref module is not suited for production environment. Instead, we shall use Waitress server. Modify the hello.py as per the following code −
from pyramid.config import Configurator
from pyramid.response import Response
from waitress import serve
def hello_world(request):
return Response('Hello World!')
if __name__ == '__main__':
with Configurator() as config:
config.add_route('hello', '/')
config.add_view(hello_world, route_name='hello')
app = config.make_wsgi_app()
serve(app, host='0.0.0.0', port=6543)
所有其他功能都是相同的,不同之处在于我们使用 waitress 模块的 serve() 函数来启动 WSGI 服务器。在运行该程序后,访问浏览器中的 '/' 路由,会像之前一样显示“Hello world”消息。
All other functionality is same, except we use serve() function of waitress module to start the WSGI server. On visiting the '/' route in the browser after running the program, the Hello world message is displayed as before.
除了函数,还可以使用可调用的类作为视图。一个可调用的类是覆盖了 call() 方法的类。
Instead of a function, a callable class can also be used as a view. A callable class is the one which overrides the call() method.
from pyramid.response import Response
class MyView(object):
def __init__(self, request):
self.request = request
def __call__(self):
return Response('hello world')