Selenium 简明教程
Selenium WebDriver - Dropdown Box
Selenium WebDriver 可用于使用 Select 类的帮助来处理网页上的下拉框。一个网页上可能有两种类型的下拉框 - 单选(选择一个选项)和多选(选择多个选项)。在 HTML 术语中,每个下拉框都由标签名 select 识别。此外,它的每个选项都使用标签名 option 识别。此外,对于多选下拉框,有一个与 select 标签名一起出现的属性 multiple。
Identify Dropdown in HTML
在浏览器(例如 Chrome)中右键单击网页,然后单击“检查”按钮。然后,网页的所有 HTML 代码都将显示出来。要研究网页上的单选下拉框,请单击 HTML 代码顶部处的左上角,如下图中突出显示所示。
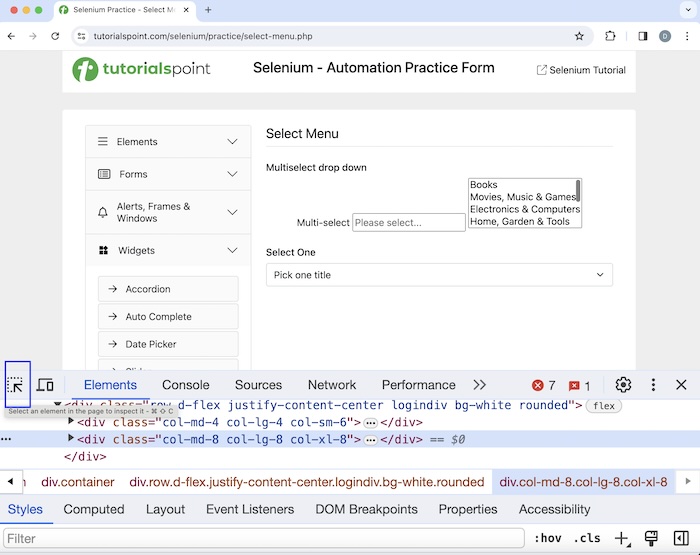
一旦我们单击箭头并将箭头指向文本 Select One 旁边的下拉框,它的 HTML 代码便可见,反映了 select 标签名(<> 中包含)及其在 option 标签名中的选项。

此处,选项 Pick one title 具有所选属性,反映了它是默认选定的事实。
Basic Select Class Methods in Selenium Webdriver
Selenium WebDriver 中有许多 Select 类的方法。它们在下面列出 −
getOptions()
返回下拉框中所有选项的列表。
WebDriver driver = new ChromeDriver();
WebElement e = driver.findElement(By.xpath("value of xpath"))
Select s = new Select(e);
List<WebElement> l = s.getOptions();
getFirstSelectedOption()
返回下拉框中选定的选项。如果选择了多个选项,则只返回第一项。
WebDriver driver = new ChromeDriver();
WebElement e = driver.findElement(By.xpath("value of xpath")
Select s = new Select(e);
l = s. getFirstSelectedOption();
isMultiple()
返回布尔值,如果下拉框允许选择多个项目,则产生 true 值。
WebDriver driver = new ChromeDriver();
WebElement e = driver.findElement(By.xpath("value of xpath")
Select s = new Select(e);
boolean l = s.isMultiple();
selectByIndex()
下拉列表中要选择的选项的索引作为参数传递。索引从 0 开始。
WebDriver driver = new ChromeDriver();
WebElement e = driver.findElement(By.xpath("value of xpath")
Select s = new Select(e);
s.selectByIndex(0);
selectByValue()
下拉列表中要选择的选项的 value 属性作为参数传递。下拉列表中的选项应具有 value 属性,才能使用此方法。
WebDriver driver = new ChromeDriver();
WebElement e = driver.findElement(By.xpath("value of xpath")
Select s = new Select(e);
s.selectByValue("option 1");
selectByVisibleText()
下拉列表中要选择的选项的可见文本作为参数传递。
WebDriver driver = new ChromeDriver();
WebElement e = driver.findElement(By.xpath("value of xpath")
Select s = new Select(e);
s.selectByVisibleText("Selenium");
deselectByVisibleText()
下拉列表中要取消选择选项的可见文本作为参数传递。它仅适用于多选下拉列表。
WebDriver driver = new ChromeDriver();
WebElement e = driver.findElement(By.xpath("value of xpath")
Select s = new Select(e);
s.deselectByVisibleText("Selenium");
deselectByValue()
下拉列表中要取消选择选项的 value 属性作为参数传递。下拉列表中的选项应具有 value 属性,才能使用此方法。它仅适用于多选下拉列表。
WebDriver driver = new ChromeDriver();
WebElement e = driver.findElement(By.xpath("value of xpath")
Select s = new Select(e);
s.deselectByValue("option 1");
deselectByIndex()
下拉列表中要取消选择选项的索引作为参数传递。索引从 0 开始。它仅适用于多选下拉列表。
WebDriver driver = new ChromeDriver();
WebElement e = driver.findElement(By.xpath("value of xpath")
Select s = new Select(e);
s.deselectByIndex(0);
Example 1
我们以以下页面为例,其中我们将访问文本 Select One 下方的下拉列表,选择值 Dr 。

代码实现
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.Select;
import java.util.List;
import java.util.concurrent.TimeUnit;
public class DropdownSingle {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage where we will get dropdown
driver.get("https://www.tutorialspoint.com/selenium/practice/select-menu.php");
// identify dropdown then select its options by value
WebElement dropdown = driver.findElement(By.xpath("//*[@id='inputGroupSelect03']"));
Select select = new Select(dropdown);
// get option selected by default
WebElement o = select.getFirstSelectedOption();
System.out.println("Option selected by default: " + o.getText());
// select an option by value
select.selectByValue("1");
// get selected option
List<WebElement> selectedOptions = select.getAllSelectedOptions();
for (WebElement opt : selectedOptions){
System.out.println("Selected Option is: " + opt.getText());
}
// get all options of dropdown
List<WebElement> options =select.getOptions();
for (WebElement opt : options){
System.out.println("Options are: " + opt.getText());
}
// check if multiselect dropdown
Boolean b = select.isMultiple();
System.out.println("Boolean value for checking is: "+ b);
// quitting browser
driver.quit();
}
}
Output
Option selected by default: Pick one title
Selected Option is: Dr.
Options are: Pick one title
Options are: Dr.
Options are: Mr.
Options are: Mrs.
Options are: Ms.
Options are: Proof.
Options are: Other
Boolean value for checking is: false
Process finished with exit code 0
在上面的示例中,我们使用控制台中的消息 - Selected Option is: Dr 获取了下拉列表中选定的选项。然后使用控制台中的消息 - Options are: Pick one title, Options are: Dr., Options are: Africa, Options are: Mr., Options are: Mrs., Options are: Ms, Options are: Proof 获取下拉列表中的所有选项,选项为:其他。
我们还使用控制台中的消息 - Boolean value for checking is: false 验证下拉列表没有多选选项。我们还使用控制台中的消息 - Option selected by default: Pick one title 检索了下拉列表中默认选定的选项。
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Example 2
我们再以以下页面为例,其中我们将访问文本多选下拉列表旁边的多选下拉列表,选择值 Books 和 Toys, Kids & Baby 。
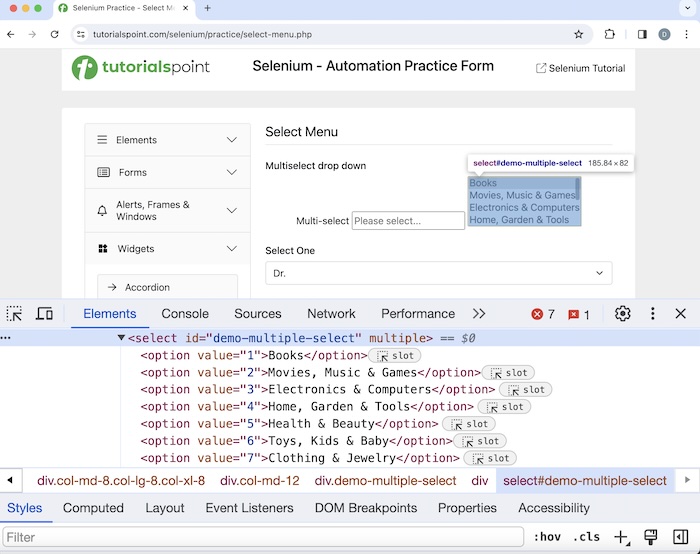
代码实现
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.Select;
import java.util.List;
import java.util.concurrent.TimeUnit;
public class DropdownMultiple {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage where we will get dropdown
driver.get("https://www.tutorialspoint.com/selenium/practice/select-menu.php");
// identify multiple dropdown
WebElement dropdown = driver.findElement(By.xpath("//*[@id='demo-multiple-select']"));
// object of Select class
Select select = new Select(dropdown);
// gets options of dropdown in list
List<WebElement> options = select.getOptions();
for (WebElement opt : options){
System.out.println("Options are: " + opt.getText());
}
// return true if multi-select dropdown
Boolean b = select.isMultiple();
System.out.println("Boolean value for multiple dropdown: "+ b);
// select item by index
select.selectByIndex(5);
// select item by visible text
select.selectByVisibleText("Books");
// get all selected options of dropdown in list
List<WebElement> selectedOptions = select.getAllSelectedOptions();
for (WebElement opt : selectedOptions){
System.out.println("Selected Options are: " + opt.getText());
}
// get first selected option in dropdown
WebElement f = select.getFirstSelectedOption();
System.out.println("First selected option is: "+ f.getText());
// deselect option by index
select.deselectByIndex(5);
// get first selected option in dropdown after deselecting
WebElement e = select.getFirstSelectedOption();
System.out.println("Second selected option is: "+ e.getText());
// deselect all selected items
select.deselectAll();
// get all selected options of dropdown after deselected
List<WebElement> delectedOptions = select.getAllSelectedOptions();
System.out.println("No. options selected: " + delectedOptions.size());
// Closing browser
driver.quit();
}
}
Output
Options are: Books
Options are: Movies, Music & Games
Options are: Electronics & Computers
Options are: Home, Garden & Tools
Options are: Health & Beauty
Options are: Toys, Kids & Baby
Options are: Clothing & Jewelry
Options are: Sports & Outdoors
Boolean value for multiple dropdown: true
Selected Options are: Books
Selected Options are: Toys, Kids & Baby
First selected option is: Books
Second selected option is: Books
No. options selected: 0
Process finished with exit code 0
在上面的示例中,我们使用控制台中的消息 - Options are: Books, Options are: Movies, Music & Games, Options are: Home, Garden & Tools, Options are: Health & Beauty, Options are: Toys, Kids & Baby, Options are: Clothing & Jewelry, Options are: Sports & Outdoors 获取了下拉列表中的所有选项。我们还使用控制台中的消息 - Boolean value for checking is: true 验证下拉列表具有多选选项。
我们使用控制台中的消息 - Selected Options are: Books, Selected Options are: Toys, Kids & Baby 检索了下拉列表中的已选选项。我们还使用控制台中的消息 - First selected option is: Books 获取了第一项已选选项。
在取消选择第一项已选选项 Books 后,我们使用控制台中的消息 - Second selected option is: Books 接收了第二项已选选项。最后,我们取消选择了下拉列表中所有已选选项,因此使用控制台中的消息 - No. options selected: 0 获取了消息。