Selenium 简明教程
Selenium - JUnit Report
Junit 可用于创建详细的自动化测试报告。它是一个开源框架,可以与 Selenium 测试集成并用于报告目的。
Prerequisites to Create JUnit Report
-
从链接 Java Downloads 在系统中安装 Java(高于 8 的版本)。要获取有关安装 Java 的更多详细信息,请参阅链接 Java Environment Setup 。
-
从链接 Apache Maven 在系统中安装 Maven。要获取有关设置 Maven 的更多详细信息,请参阅链接 Maven Environment Setup 。
-
从链接 IntelliJ IDEA Ultimate 安装 IntelliJ。要获取有关设置 IntelliJ 的更多详细信息,请参阅链接 Selenium IntelliJ 。
Steps to Create JUnit Report
Step 1 − 创建一个 Maven 项目并将适当的依赖项添加到 pom.xml 文件中,用于以下项 −
-
从链接 Selenium Java 中添加 Selenium Java 依赖项。
-
从链接 JUnit 添加 JUnit 依赖项。
-
从链接 JUnit Jupiter API 添加 JUnit Jupiter 依赖项。
-
从链接 Apache Maven Site Plugin 添加 Maven site 依赖项。
-
从链接中添加 Maven Surefire 报告依赖 Maven Surefire Report Plugin 。
-
使用所有依赖项保存 pom.xml 并更新 Maven 项目。
Step 2 −使用以下示例的实现创建 JUnit 测试类,其中我们将首先单击 New User button 验证 Welcome Page 上的文本 Welcome, Login In 。
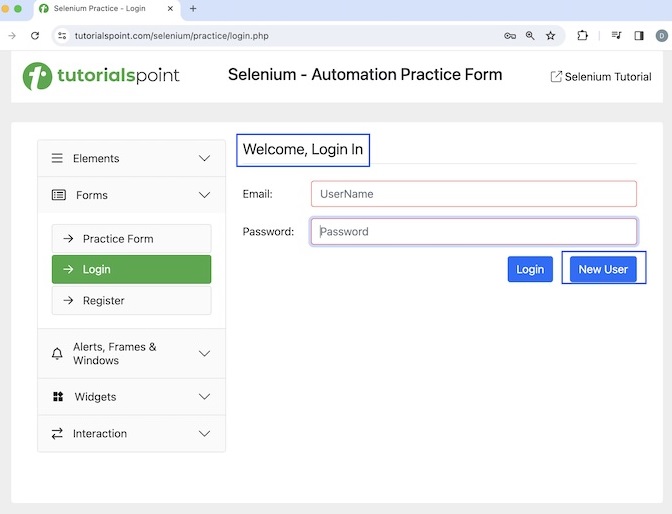
点击 New User 按钮后,我们将导航到注册页面,其中 Back to Login 按钮如以下图像中突出所示。

Code Implementation
package Report;
import org.junit.*;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.junit.jupiter.api.MethodOrderer;
import org.junit.jupiter.api.Order;
import org.junit.jupiter.api.TestMethodOrder;
import java.util.concurrent.TimeUnit;
import static org.junit.Assert.assertEquals;
@TestMethodOrder(MethodOrderer.OrderAnnotation.class)
public class JunitTest {
WebDriver driver;
@Before
public void setup() throws Exception{
// Initiate browser driver
driver = new ChromeDriver();
// adding implicit wait of 20 secs
driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS);
// Opening the webpage
driver.get("https://www.tutorialspoint.com/selenium/practice/login.php");
}
@Test
@Order(1)
public void verifyLoginAndRegisterPage() {
// identify header then get text
WebElement header = driver.findElement
(By.xpath("//*[@id='signInForm']/h1"));
String text = header.getText();
// assertions to test case to check login page
assertEquals("Welcome, Login In", text);
// navigate to register page
WebElement btn = driver.findElement
(By.xpath("//*[@id='signInForm']/div[3]/a"));
btn.click();
// assertions added to test case to check register page
WebElement btnchk = driver.findElement
(By.xpath("//*[@id='signupForm']/div[5]/a"));
boolean displayed = btnchk.isDisplayed();
// assertions to test case
assertEquals(true, displayed);
}
@After
public void teardown() {
// quitting browser
driver.quit();
}
}
pom.xml 文件中的依赖项。
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>SeleniumJava</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>16</maven.compiler.source>
<maven.compiler.target>16</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.11.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.junit.jupiter/junit-jupiter-api -->
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.10.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/junit/junit -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.maven.plugins/maven-site-plugin -->
<dependency>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-site-plugin</artifactId>
<version>4.0.0-M13</version>
</dependency>
</dependencies>
<!-- https://mvnrepository.com/artifact/org.apache.maven.plugins/maven-surefire-report-plugin -->
<reporting>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-report-plugin</artifactId>
<version>3.2.5</version>
</plugin>
</plugins>
</reporting>
</project>
Step 3 −使用以下命令从命令行运行测试: mvn clean test suite 。
Step 4 −刷新项目,名为 site 的新文件夹应在 target 文件夹内生成。
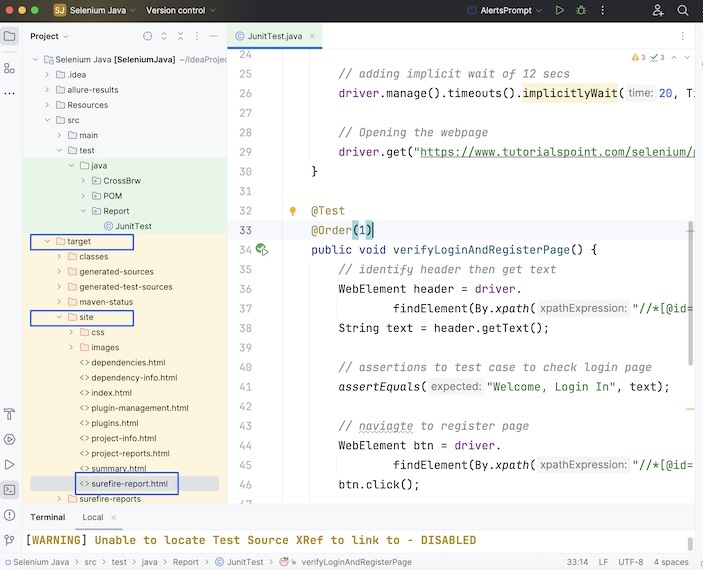
Step 5 −右键单击 surefire-report.html 并选择在浏览器中打开的选项。
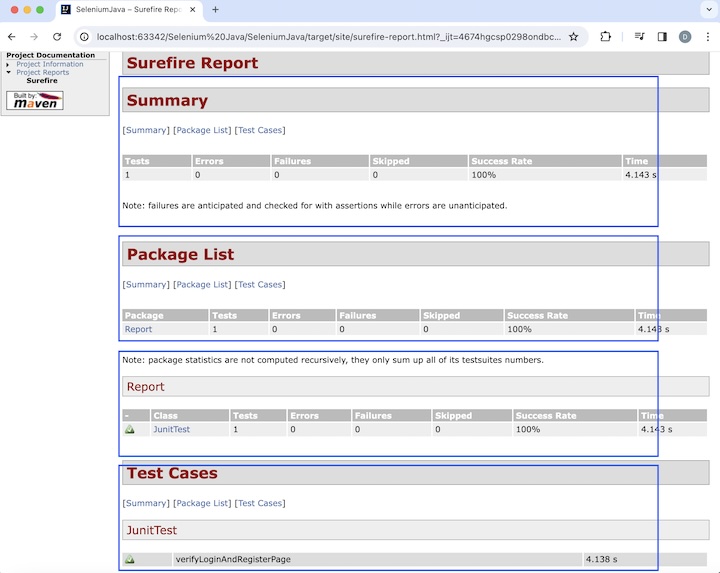
-
JUnit* 报告将在浏览器中打开,显示包含总共 1 个测试方法的摘要,通过成功率为 100%。它还显示了包列表(包的名称、测试的数量、通过数、失败数、通过成功率、测试持续时间等)的详细信息。此外,测试方法名称 verifyLoginAndRegisterPage 也包含在报告中。