Selenium 简明教程
Selenium WebDriver - Browser Commands
Selenium Webdriver 提供了多个命令,这些命令有助于打开浏览器、对打开的浏览器执行一些操作并退出浏览器。
Selenium Webdriver provides multiple commands which help to open a browser, perform some actions on an opened browser, and move out of the browser.
Basic Browser Commands in Selenium Webdriver
下面讨论了一些浏览器命令−
Some of the browser commands are discussed below −
get(String URL) command
此命令打开一个浏览器,然后启动作为参数传递的URL。
This command opens a browser then launches the URL passed as a parameter.
driver.get("https://www.tutorialspoint.com/selenium/practice/nestedframes.php");
getTitle() command
此命令获取作为字符串作为焦点的页面的标题。它不接受参数。
This command fetches the page title of the browser which is in focus as String. It accepts no parameters.
String str = driver.getTitle();
getCurrentUrl() command
此命令获取作为字符串作为焦点的浏览器的URL。它不接受参数。
This command fetches the url of the browser which is in focus as String. It accepts no parameters.
String str = driver.getCurrentUrl();
getPageSource() command
此命令获取作为字符串作为焦点的页面源代码。它不接受参数。
This command fetches the page source browser which is in focus as String. It accepts no parameters.
String str = driver.getPageSource();
How to Inspect Elements on a Web Page?
首先在网页上单击鼠标右键,然后在 Chrome 浏览器中单击“检查”按钮。这之后会打开整个页面的 HTML 代码。若要调查页面上的特定元素,请单击可见 HTML 代码顶部的左上方箭头,如下所示。
First right click on the web page, then click on the Inspect button in the Chrome browser. This will be followed by the opening of the HTML code for the complete page. For investigating on a particular element on the page, click on the left upward arrow at the top of the visible HTML code as highlighted below.
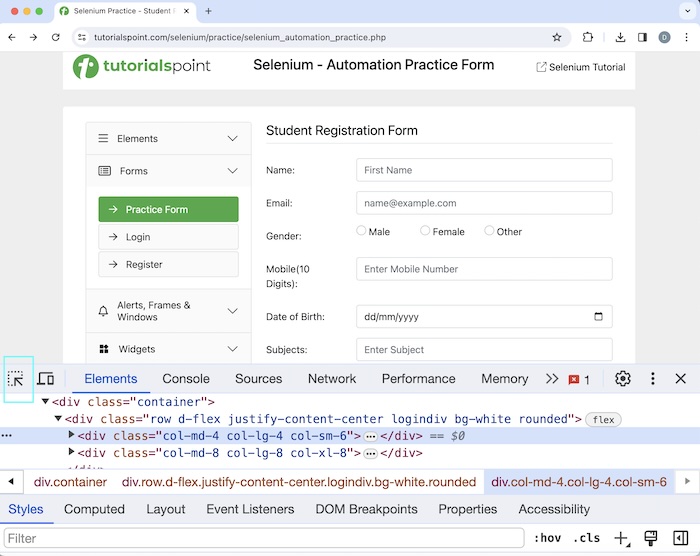
Example 1
让我们以以下页面为例,首先将通过 URL 启动一个应用程序: Selenium Automation Practice Form ,然后获取浏览器标题 Selenium Practice - Student Registration Form 。
Let us take an example on the below page, where we would first launch an application having the URL: Selenium Automation Practice Form and then obtain browser title Selenium Practice - Student Registration Form.
请注意,我们可以在 head 标签内部的 title 标签的 HTML 代码中获取网页的浏览器标题。在下图中,我们看到该页面的标题为 Selenium Practice - Student Registration Form 。
Please note, we can get the browser title of a web page in the HTML code, within the title tag which resides inside the head tag. In the below image, we see that the title of the page is Selenium Practice - Student Registration Form.
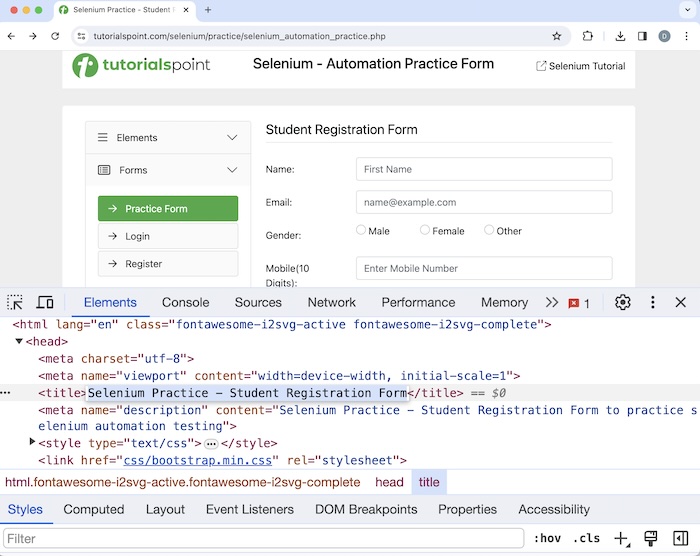
然后,我们将单击 Login 按钮,之后会导航到另一个页面的浏览器标题 Selenium Practice - Login 和 URL 为: Selenium Automation Practice Form 。最后,我们将退出 Web 驱动程序会话。
Then, we would click on the Login button, after which we would be navigated to another page having the browser title Selenium Practice - Login and url as: Selenium Automation Practice Form. Finally, we would quit the web driver session.

代码实现
Code Implementation
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.concurrent.TimeUnit;
public class BrowserCommand {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// launching a browser and open a URL
driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php");
// Getting browser title after launch
System.out.println("Getting browser title after launch: " + driver.getTitle());
// Getting browser URL after launch
System.out.println("Getting URL after launch: " + driver.getCurrentUrl());
// identify link then click
WebElement l = driver.findElement(By.xpath("//*[@id='collapseTwo']/div/ul/li[2]/a"));
l.click();
// Getting browser title after clicking link
System.out.println("Getting browser title after clicking link: " + driver.getTitle());
// Getting browser URL after launch
System.out.println("Getting URL after clicking link: " + driver.getCurrentUrl());
// Quitting browser
driver.quit();
}
}
Output
Getting browser title after launch: Selenium Practice - Student Registration Form
Getting URL after launch:
https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php
Getting browser title after clicking link: Selenium Practice - Login
Getting URL after clicking link:
https://www.tutorialspoint.com/selenium/practice/login.php
Process finished with exit code 0
在上述示例中,我们已经打开了浏览器中的一个 URL,并通过控制台中的消息分别获取了浏览器标题和当前 URL - Getting browser title after launch: Selenium Practice - Student Registration Form and Getting URL after launch: Selenium Automation Practice Form 。
In the above example, we had launched a URL in the opened browser and obtained the browser title and current URL with the message in the console - Getting browser title after launch: Selenium Practice - Student Registration Form and Getting URL after launch: Selenium Automation Practice Form respectively.
然后,我们已经单击了登录并在导航后通过控制台中的消息收到了浏览器标题和当前 URL - Getting browser title after clicking link: Selenium Practice - Login and Getting URL after clicking link: Selenium Automation Practice Form 。接下来,我们必须退出驱动程序会话。
We had then clicked on the Login and received the browser title and current URL after navigation with the message in the console - Getting browser title after clicking link: Selenium Practice - Login and Getting URL after clicking link: Selenium Automation Practice Form. Next, we had to quit the driver session.
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 2
让我们以以下图像所示的另一个示例为例,我们将在其中单击 New Tab 。
Let us take another example as shown in the below image where we would click on the New Tab.

之后,我们将获得另一个包含文本 New Tab 的窗口。
After that, we would obtain another window having text New Tab.
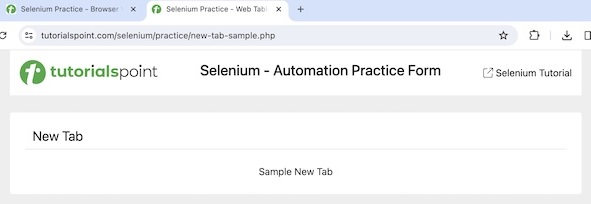
然后,我们将关闭文本 New Tab 的新窗口,返回到原始窗口,并在那里访问文本 - Browser Windows 。最后,我们将退出会话。
Then we would close the new window with text New Tab and move back to the original window and access text - Browser Windows there. Finally, we would quit the session.

代码实现
Code Implementation
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.Set;
import java.util.concurrent.TimeUnit;
public class WindowClose {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 12 secs
driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS);
// Opening the webpage where we will open a new window
driver.get("https://www.tutorialspoint.com/selenium/practice/browser-windows.php");
// click button and navigate to next window
WebElement b = driver.findElement
(By.xpath("/html/body/main/div/div/div[2]/button[1]"));
b.click();
// Get the window handle of the original window
String oW = driver.getWindowHandle();
// get all opened windows handle ids
Set<String> windows = driver.getWindowHandles();
// Iterating through all window handles
for (String w : windows) {
if(!oW.equalsIgnoreCase(w)) {
// switching to child window
driver.switchTo().window(w);
// accessing element in new window
WebElement e = driver.findElement
(By.xpath("/html/body/main/div/div/h1"));
System.out.println("Text in new window is: " + e.getText());
// closing new window
driver.close();
break;
}
}
// switching to parent window
driver.switchTo().window(oW);
// accessing element in parent window
WebElement e1 = driver.findElement
(By.xpath("/html/body/main/div/div/div[2]/h1"));
System.out.println("Text in parent window is: " + e1.getText());
// quitting the browser session
driver.quit();
}
}
Output
Text in new window is: New Tab
Text in parent window is: Browser Windows
在上述示例中,我们已经捕获了新窗口上的文本,并通过控制台中的消息收到了文本 - Text in new window is: New Tab 。然后,我们关闭了子窗口并切换回父窗口。最后,通过控制台获取父窗口中的文本 - TText in parent window is: Browser Windows 。
In the above example, we had captured the text on the new window and received the message in the console - Text in new window is: New Tab. Then we closed the child window and switched back to the parent window. Lastly, obtain the text on the parent window in the console - TText in parent window is: Browser Windows.
Example 3
让我们以上述页面的另一个示例为例,我们将在其中使用 getPageSource() 方法获取页面源代码。
Let us take another example of the above page, where we would get the page source using the getPageSource() method.
package org.example;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.concurrent.TimeUnit;
public class WindowPagSource {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 12 secs
driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS);
// Opening the webpage where we will open a new window
driver.get("https://www.tutorialspoint.com/selenium/practice/browser-windows.php");
// Getting browser page source
System.out.println("Getting page source: " + driver.getPageSource());
// quitting the browser session
driver.quit();
}
}
在上述示例中,我们通过控制台中消息捕获了网页上启动的网页的页面源代码。
In the above example, we captured the page source of the web page launched on a web page with the message in the console.
Conclusion
到这里我们就结束了关于 Selenium WebDriver 浏览器命令教程的全面讨论。我们已经开始叙述 Selenium WebDriver 中的基本浏览器命令,如何在网页上检查元素,并通过示例介绍了如何将浏览器命令与 Selenium WebDriver 结合使用。这让您可以深入了解 Selenium WebDriver 浏览器命令。最好持续练习你学到的内容,并探索与 Selenium 相关的其他内容,以加深你的理解并拓展你的视野。
This concludes our comprehensive take on the tutorial on Selenium WebDriver Browser Commands. We’ve started with describing basic browser commands in Selenium Webdriver, how to inspect elements on a web page, and walked through examples on how to use the browser commands with Selenium Webdriver. This equips you with in-depth knowledge of the Selenium WebDriver Browser Commands. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.