Selenium 简明教程
Selenium WebDriver - Capture Screenshots
Selenium Webdriver 可用于在运行自动化步骤时截取网页的屏幕截图。屏幕截图是借助 TakeScreenshot 方法截取的。
Selenium Webdriver can be used to capture screenshots of a web page while running the automated steps. The screenshots are captured with the help of the TakeScreenshot method.
此方法向 Selenium Webdriver 发出截取屏幕截图的指令。要在所需位置截取屏幕截图,我们需要使用 getScreenshotAs() 方法。
This method gives the instruction to the Selenium Webdriver to capture the screenshot. To capture the screenshot in a desired location, we would need to use the getScreenshotAs() method.
Element Identify on Web Page
右键单击网页,然后在 Chrome 浏览器中单击“检查”按钮。然后,将显示整个页面的相应 HTML 代码。要调查页面上的编辑框,请单击可见 HTML 代码顶部的左上箭头。
Right click on the webpage, and then click on the Inspect button in the Chrome browser. Then, the corresponding HTML code for the complete page would be available. For investigating an edit box on a page, click on the left upward arrow, available to the top of the visible HTML code.

一旦我们单击并用箭头指向编辑框(在下图中突出显示),它的 HTML 代码就会出现,反映输入标签名称。
Once we had clicked and pointed the arrow to the edit box(highlighted in the below image), its HTML code appeared, reflecting the input tagname.
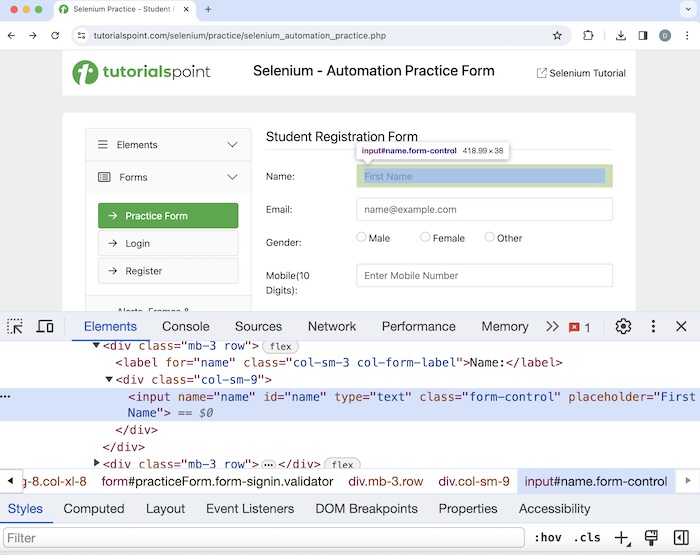
Example 1 - Take Screenshot of Complete Page
我们来看一下上页的示例,我们首先使用 sendKeys() 方法在输入框中输入一些文本,然后截取整个网页的屏幕截图。
Let us take an example of the above page, where we would first enter some text in the input box with the help of the sendKeys() method and then take the screenshot of the entire web page.
package org.example;
import org.apache.commons.io.FileUtils;
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
import java.io.File;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
public class ScreenshotCapture {
public static void main(String[] args) throws InterruptedException, IOException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage where we will identify an element
driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php");
// Identify the input box with xpath locator
WebElement e = driver.findElement(By.xpath("//*[@id='name']"));
// enter text in input box
e.sendKeys("Selenium");
// Get the value entered
String text = e.getAttribute("value");
System.out.println("Entered text is: " + text);
// take full screenshot and store in project location
File scrFile = ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE);
FileUtils.copyFile(scrFile, new File("./ImageFullPage.png"));
// Closing browser
driver.quit();
}
}
在 pom.xml 文件中添加的依赖项:
Dependencies added in pom.xml file −
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>SeleniumJava</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>16</maven.compiler.source>
<maven.compiler.target>16</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.11.0</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.12.0</version>
</dependency>
</dependencies>
</project>
一个名为 ImageFullPage.png 的屏幕截图在项目目录中被截取。点击它,我们将获得整个网页的截取的屏幕截图。
A screenshot with the filename ImageFullPage.png got captured in the project directory. On clicking it, we would get the captured screenshot of the entire web page.

Example 2 - Take Screenshot of Single Element
让我们取上面讨论的相同的示例,我们首先在编辑框中输入一些文本,然后仅截取该元素的屏幕截图。可以使用 getScreenShotAs() 方法来完成此操作。
Let us take the same example as discussed above, where we would first enter some text in the edit box and then take the screenshot of that element only. This can be done using the getScreenShotAs() method.
package org.example;
import org.apache.commons.io.FileUtils;
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
import java.io.File;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
public class ScreenshotCaptureElement {
public static void main(String[] args) throws InterruptedException, IOException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage where we will identify an element
driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php");
// Identify the input box with xpath locator
WebElement e = driver.findElement
(By.xpath("//*[@id='name']"));
// enter text in input box
e.sendKeys("Selenium");
// Get the value entered
String text = e.getAttribute("value");
System.out.println("Entered text is: " + text);
// take screenshot of input box and store in project location
File scrFile = e.getScreenshotAs(OutputType.FILE);
FileUtils.copyFile(scrFile, new File("./ImageElement.png"));
// Closing browser
driver.quit();
}
}
一个名为 ImageElement.png 的屏幕截图在项目目录中被截取。点击它,我们将获得我们输入文本 Selenium 的输入框的截取的屏幕截图。
A screenshot with the filename ImageElement.png got captured in the project directory. On clicking it, we would get the captured screenshot of only the input box where we entered the text Selenium.

Example 3 - Take Screenshot on Exception
让我们考虑上面讨论过的相同示例,其中我们将尝试在输入框中输入一些文本,然后仅在该步骤失败的情况下截取该步骤的屏幕截图。可以使用添加到 catch 块(通常用于异常处理)中的 getScreenShotAs() 方法来实现此目的。
Let us take the same example as discussed above, where we would try to enter some text in the input box and then take the screenshot of that step only if there is a failure in that step. This can be done using the getScreenShotAs() method added to the catch block(usually used for exception handling).
package org.example;
import org.apache.commons.io.FileUtils;
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
import java.io.File;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
public class ScreenshotCaptureException {
public static void main(String[] args) throws InterruptedException, IOException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage where we will identify an element
driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php");
try {
// Identify the input box with xpath locator
WebElement e = driver.findElement(By.xpath("//*[@id='names']"));
// enter text in input box
e.sendKeys("Selenium");
System.out.println("Step successfully executed");
}
catch(Exception e) {
// take screenshot on failure and store in project location
File scrFile
= ((TakesScreenshot) driver).
getScreenshotAs(OutputType.FILE);
FileUtils.copyFile(scrFile, new File("./ImageException.png"));
System.out.println("Element could not be found - Step failed");
}
// Closing browser
driver.quit();
}
}
Output
Element could not be found - Step failed
Process finished with exit code 0
在上面的示例中,我们的测试启动了一个网页并尝试与一个元素交互。在无法识别元素后,执行流转到 catch 块,然后我们在控制台中收到消息 - Element could not be found - Step failed 。
In the above example, our test launched a web page and tried to interact with an element. After the element could not be identified, the flow of execution moved to the catch block where we got the message in the console - Element could not be found - Step failed.
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
此外,带有文件名称 ImageException.png 的屏幕截图会捕获到项目目录中。单击它,我们将获得失败的测试步骤的捕获屏幕截图(这意味着,未在输入框中输入 Selenium)。
Also, the screenshot with the file name ImageException.png got captured in the project directory. On clicking it, we would get the captured screenshot of the failed test step(which means, Selenium did not get entered in the input box).

Conclusion
这就结束了我们对 Selenium Webdriver 捕获屏幕截图教程的全面介绍。我们从描述网页上的元素标识开始,并逐步讲解了如何使用 Selenium Webdriver 捕获屏幕截图的示例。这使你对 Selenium Webdriver 捕获屏幕截图有了深入的了解。明智的做法是不断实践你所学到的知识,并探索与 Selenium 相关的其他知识以加深你的理解并拓展你的视野。
This concludes our comprehensive take on the tutorial on Selenium Webdriver Capture Screenshots. We’ve started with describing element identity on a web page, and walked through examples of how to capture screenshots with Selenium Webdriver. This equips you with in-depth knowledge of the Selenium Webdriver Capture Screenshots. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.