Selenium 简明教程
Selenium WebDriver - Handling Forms
Selenium Webdriver 可用于处理网页上的表单。在 HTML 术语中,表单元素通过称为 * Form* 的标签名来识别。此外,它应具备提交表单的功能,用于提交表单的元素应具有标签名 * input* ,同时属性 type 的值为 submit 。必须注意的是,网页上的表单可能包含文本框、链接、复选框、单选按钮和其他 Web 元素,这些元素将帮助用户在网页上输入详细信息。
Selenium Webdriver can be used to handle forms on a web page. In HTML terminology, a form element is identified by the tagname called Form. Also, it should have the feature to submit the form, the element used for form submission should have the tagname called input along with attribute type having the value as submit. It must be noted that a form on a web page may contain text boxes, links, checkboxes, radio buttons, and other web elements that would help the user to input details on a web page.
Identification of Forms on Web Page
右键点击在 Chrome 浏览器中打开的页面。点击“检查”按钮。之后,整个页面的 * HTML* 代码将显示出来。要调查页面上的表单元素,请点击位于 HTML 代码顶部的左上角箭头,如下所示。
Right click on a page, opened in the Chrome browser. Click on the Inspect button. Post that, the HTML code for the entire page would be visible. For investigating a form element on a page, click on the left upward arrow, available to the top of the HTML code as highlighted below.
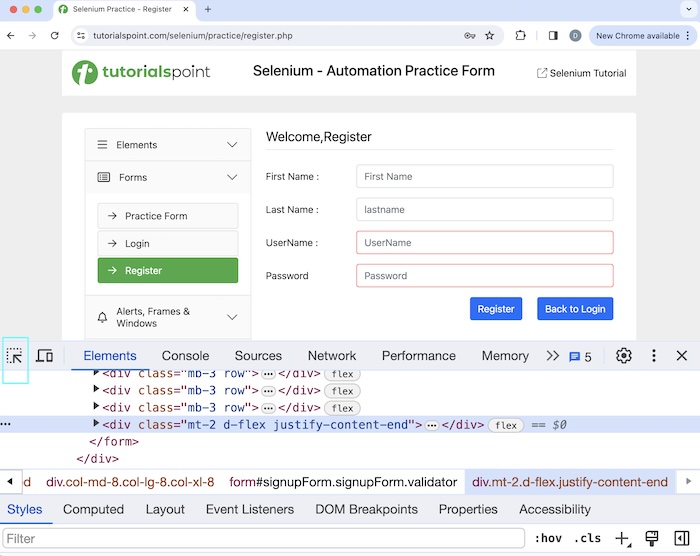
一旦我们点击并将箭头指向表单中的任何元素(例如,注册按钮),就可以看到其 HTML 代码,其中反映了 form 标签名。
Once, we had clicked and pointed the arrow to any element inside the form (for example, the Register Button), its HTML code was available, reflecting the form tagname.

在上面的表单中,我们可以使用 Register 按钮并填写详细信息来提交表单。一旦我们点击并将箭头指向 Register 按钮,就会出现其 HTML 代码,其中反映了 input 标签名,其 type 属性的值为 submit 。
In the above form, we can submit it with details, using the Register button. Once, we had clicked and pointed the arrow to the Register button, its HTML code appeared, reflecting the input tagname, and its type attribute having the value submit.

<input type="submit" class="btn btn-primary" value="Register">
我们可以借助 submit() 和 click() 方法提交表单。普通按钮和提交按钮之间的基本区别在于,普通按钮只能通过 click() 方法进行交互,但提交按钮可以通过 click() 和 submit() 方法进行交互。
We can submit a form with the help of the methods - submit() and click(). The basic difference between a normal button and submit button is that, a normal button can be interacted only with the click() method but the submit button can be interacted with both click() and submit() method.
Syntax
使用 submit 方法的语法 -
Syntax with submit method −
WebDriver driver = new ChromeDriver();
// identify input box 1
WebElement inputBx = driver.findElement
(By.xpath("<value of xpath>"));
inputBx.sendKeys("Selenium");
// submit form
WebElement btn = driver.findElement
(By.xpath("<value of xpath>"));
btn.submit();
此外,普通按钮具有 input 标签名,其 type 属性应为 button 。在下面的页面中,让我们看看网页上 Click Me 按钮的 HTML 代码。
Also, the normal button has the input tagname, and its type attribute should be the value button. In the below page, let us see the HTML code of the Click Me button on a web page.

<button type="button" class="btn btn-primary"
onclick="showDiv()">Click Me</button>
Example 1 - Using submit() Method
让我们看下面页面中的表单示例,它包含以下 Web 元素:标签、输入框、按钮、密码等。
Let us take an example of the form in the below page, which contains the web elements - label, input box, button, password, and so on.

Syntax
WebDriver driver = new ChromeDriver();
// identify input box 1
WebElement inputBx = driver.findElement
(By.xpath("<value of xpath>"));
inputBx.sendKeys("Selenium");
// get value entered
System.out.println("Value entered in FirstName: " + inputBx.getAttribute("value"));
// identify input box 2
WebElement inputBx2 = driver.findElement
(By.xpath("<value of xpath>"));
inputBx2.sendKeys("Tutorials");
// get value entered
System.out.println("Value entered in LastName: " + inputBx2.getAttribute("value"));
// identify input box 3
WebElement inputBx3 = driver.findElement
(By.xpath("<value of xpath>"));
inputBx3.sendKeys("Tutorialspoint");
// identify input box 4
WebElement inputBx4 = driver.findElement
(By.xpath("<value of xpath>"));
inputBx3.sendKeys("Tutorialspoint");
// submit form
WebElement btn = driver.findElement
(By.xpath("<value of xpath>"));
btn.submit();
代码实现
Code Implementation
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.concurrent.TimeUnit;
public class FormElements {
public static void main(String[] args) {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage where we will identify form
driver.get("https://www.tutorialspoint.com/selenium/practice/register.php");
// identify input box 1
WebElement inputBx = driver.findElement(By.xpath("//*[@id='firstname']"));
inputBx.sendKeys("Selenium");
// get value entered
System.out.println("Value entered in FirstName: " + inputBx.getAttribute("value"));
// identify input box 2
WebElement inputBx2 = driver.findElement(By.xpath("//*[@id='lastname']"));
inputBx2.sendKeys("Tutorials");
// get value entered
System.out.println("Value entered in LastName: " + inputBx2.getAttribute("value"));
// identify input box 3
WebElement inputBx3 = driver.findElement(By.xpath("//*[@id='username']"));
inputBx3.sendKeys("Tutorialspoint");
// get value entered
System.out.println("Value entered in UserName: " + inputBx3.getAttribute("value"));
// identify input box 4
WebElement inputBx4 = driver.findElement(By.xpath("//*[@id='password']"));
inputBx3.sendKeys("Tutorialspoint");
// submit form with submit method
WebElement btn = driver.findElement(By.xpath("//*[@id='signupForm']/div[5]/input"));
btn.submit();
// Close browser
driver.quit();
}
}
Value entered in FirstName: Selenium
Value entered in LastName: Tutorials
Value entered in UserName: Tutorialspoint
Process finished with exit code 0
在上面的示例中,我们在包含输入框的表单中填写内容,然后使用控制台中的消息获取输入的值(除了密码字段) - Value entered in FirstName: Selenium, Value entered in LastName: Tutorials 和 Value entered in UserName: Tutorialspoint 。
In the above example, we had filled the form having the input boxes then obtained the value entered (except the password field) with the message in the console - Value entered in FirstName: Selenium, Value entered in LastName: Tutorials, and Value entered in UserName: Tutorialspoint.
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 2 - Using click() Method
让我们看下面页面中的表单的另一个示例,它包含以下 Web 元素:标签、输入框、按钮、密码等。
Let us take another example of the form in the below page, which contains the web elements like the label, input box, button, password, and so on.

Syntax
使用 click() 方法的语法 -
Syntax with click() method −
WebDriver driver = new ChromeDriver();
// identify input box 1
WebElement inputBx = driver.findElement(By.xpath("<value of xpath>"));
inputBx.sendKeys("Selenium");
// get value entered
System.out.println("Value entered in FirstName: " + inputBx.getAttribute("value"));
// identify input box 2
WebElement inputBx2 = driver.findElement(By.xpath("<value of xpath>"));
inputBx2.sendKeys("Selenium");
// submit form
WebElement btn = driver.findElement(By.xpath("<value of xpath>"));
btn.click();
代码实现
Code Implementation
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.concurrent.TimeUnit;
public class FormElement {
public static void main(String[] args) {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage where we will identify form
driver.get("https://www.tutorialspoint.com/selenium/practice/login.php");
// identify input box 1
WebElement inputBx = driver.findElement(By.xpath("//*[@id='email']"));
inputBx.sendKeys("Selenium");
// get value entered
System.out.println("Value entered in Email: " + inputBx.getAttribute("value"));
// identify input box 2
WebElement inputBx2 = driver.findElement(By.xpath("//*[@id='password']"));
inputBx2.sendKeys("Tutorials");
// submit form with click() method
WebElement btn = driver.findElement(By.xpath("//*[@id='signInForm']/div[3]/input"));
btn.click();
// Closing browser
driver.quit();
}
}
Value entered in Email: Selenium
在上面的示例中,我们在包含输入框的表单中填写内容,然后使用控制台中的消息获取输入的值(除了密码字段) - Value entered in Email: Selenium 。
In the above example, we had filled out the form having the input boxes then obtained the value entered (except the password field) with the message in the console - Value entered in Email: Selenium.
Conclusion
这结束了我们对 Selenium WebDriver 表单处理教程的全面讲解。我们从描述网页上表单的识别开始,并逐步举例说明如何使用 Selenium Webdriver 来处理表单。这让你深入了解 Selenium WebDriver 表单处理。明智的做法是继续练习你学到的知识,并探索其他与 Selenium 相关的知识,以加深你的理解并拓宽你的视野。
This concludes our comprehensive take on the tutorial on Selenium WebDriver Handling Forms. We’ve started with describing identification of forms on a web page, and walked through examples on how to handle forms with Selenium Webdriver. This equips you with in-depth knowledge of the Selenium WebDriver Handling Forms. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.