Selenium 简明教程
Selenium WebDriver - Handle Special Keys
可以在创建自动化测试时使用 Selenium WebDriver 来处理特殊键。这是使用 Selenium 中的 * Actions class* 和 sendKeys() 方法完成的。使用 keyUp() 和 keyDown() 方法进行的向上/向下键入等操作主要用于处理特殊键。如果我们使用 sendKeys() 方法,我们将需要将 Key.chord 作为参数传递给此方法。
Selenium Webdriver can be used to handle special keys while creating the automation tests. This is done using the Actions class and sendKeys() method in Selenium. The operations like key up/down using the keyUp() and keyDown() methods are mostly used to work with special keys. In case we are using sendKeys() method we would need to pass Key.chord as a parameter to this method.
Example 1 - Copy and Paste With Special Keys
下面我们来讨论复制和粘贴操作将在网页上执行的元素标识,如下面的图片所示。首先,右键单击下面的网页,然后单击 Chrome 浏览器的“检查”按钮。要调查源元素和目标元素,请单击 HTML 代码顶部的左向上箭头。
Let us now discuss the identification of elements where the copy and paste operations are to be performed on a web page as shown in the below image. First, right click on the below web page, and then click on the Inspect button in the Chrome browser. For investigating both the source and destination elements, click on the left upward arrow, at top of the HTML code.

让我们以下面的页面为例,我们首先输入文本- JavaSelenium * beside the *Full Name: 标签。然后将同一文本复制并粘贴到 Last Name: 标签旁边的另一个输入框中。
Let us take an example on the below page, where we would first enter the text - JavaSelenium * beside the *Full Name: label. Then copy and paste the same text in another input box beside the Last Name: label.
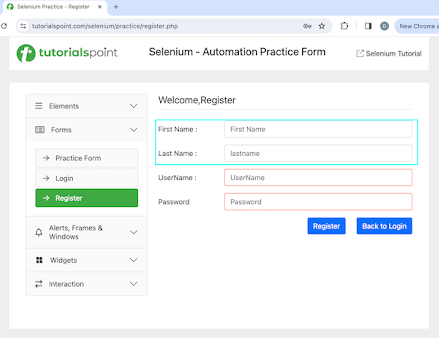
代码实现
Code Implementation
package org.example;
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
import java.util.concurrent.TimeUnit;
public class CopyPasteAction {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage where we will identify an element
driver.get("https://www.tutorialspoint.com/selenium/practice/register.php");
// Identify the first input box with xpath locator
WebElement e = driver.findElement(By.xpath("//*[@id='firstname']"));
// enter some text
e.sendKeys("Selenium");
// chose the key as per platform
Keys k = Platform.getCurrent().is(Platform.MAC) ? Keys.COMMAND : Keys.CONTROL;
// object of Actions class to copy then paste
Actions a = new Actions(driver);
a.keyDown(k);
a.sendKeys("a");
a.keyUp(k);
a.build().perform();
// Actions class methods to copy text
a.keyDown(k);
a.sendKeys("c");
a.keyUp(k);
a.build().perform();
// Action class methods to tab and reach to next input box
a.sendKeys(Keys.TAB);
a.build().perform();
// Actions class methods to paste text
a.keyDown(k);
a.sendKeys("v");
a.keyUp(k);
a.build().perform();
// Identify the second input box with xpath locator
WebElement s = driver.findElement(By.xpath("//*[@id='lastname']"));
// Getting text in the second input box
String text = s.getAttribute("value");
System.out.println("Value copied and pasted: " + text);
// Closing browser
driver.quit();
}
}
Output
Value copied and pasted: JavaSelenium
Process finished with exit code 0
在上面的示例中,我们首先在第一个输入框中输入文本 JavaSelenium ,然后将相同的文本复制并粘贴到第二个输入框中,然后将第二个输入框中的输入文本作为一条消息获取到控制台中 - Value copied and pasted: JavaSelenium 。
In the above example, we had first entered the text JavaSelenium in the first input box and then copied and pasted the same text in the second input box then obtained the entered text in the second input box as a message in the console - Value copied and pasted: JavaSelenium.
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 2 - Copy and Paste With Special Keys
让我们使用上面相同的示例,并在不使用 Actions 类的情况下实现相同的示例。
Let us take the same above example, and implement the same without using the Actions class.
package org.example;
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.concurrent.TimeUnit;
public class CopyPaste {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage where we will identify an element
driver.get("https://www.tutorialspoint.com/selenium/practice/register.php");
// Identify the first input box with xpath locator
WebElement e = driver.findElement(By.xpath("//*[@id='firstname']"));
// Identify the second input box with xpath locator
WebElement s = driver.findElement(By.xpath("//*[@id='lastname']"));
// chose the key as per platform
Keys k = Platform.getCurrent().is(Platform.MAC) ? Keys.COMMAND : Keys.CONTROL;
// enter some text
e.sendKeys("JavaSelenium");
// select the whole entered text
e.sendKeys(Keys.chord(k, "a"));
// copy the whole entered text
e.sendKeys(Keys.chord(k, "c"));
// tab and reach to next input box
e.sendKeys(Keys.TAB);
// paste the whole entered text
s.sendKeys(Keys.chord(k, "v"));
// Getting text in the second input box
String text = s.getAttribute("value");
System.out.println("Value copied and pasted: " + text);
// Closing browser
driver.quit();
}
}
Output
Value copied and pasted: JavaSelenium
在上面的示例中,我们首先在第一个输入框中输入文本 JavaSelenium ,然后使用 sendKeys() 和 Key.chord() 方法将相同的文本复制并粘贴到第二个输入框中。最后,我们获取了第二个输入框中的输入文本作为控制台中的消息 - Value copied and pasted: JavaSelenium 。
In the above example, we had first entered the text JavaSelenium in the first input box and then copied and pasted the same text in the second input box using the sendKeys() and Key.chord() methods. Finally, we had obtained the entered text in the second input box as a message in the console - Value copied and pasted: JavaSelenium.
Example 3 - Input Text in Upper Case With Special Keys
让我们再举一个例子,我们将在其中使用特殊键以大写字母输入文本 AUTOMATION 。请注意,在将值发送到 sendKeys() 方法时,我们将传递 automation 并按下 SHIFT 键。
Let us take another example, where we would enter text AUTOMATION in capital letters using the special keys. Please note that, while sending the value to the sendKeys() method, we would pass automation and pressing SHIFT keys.
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
import java.util.concurrent.TimeUnit;
public class KeyAction {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage
driver.get("https://www.tutorialspoint.com/selenium/practice/text-box.php");
// Identify the first input box with xpath locator
WebElement e = driver.findElement(By.xpath("//*[@id='fullname']"));
// Actions class
Actions a = new Actions(driver);
// moving to an input box and clicking on it
a.moveToElement(e).click();
// key UP and DOWN action for SHIFT
a.keyDown(Keys.SHIFT);
a.sendKeys("automation").keyUp(Keys.SHIFT).build().perform();
// get value entered
System.out.println("Text entered: " + e.getAttribute("value"));
// Closing browser
driver.quit();
}
}
Output
Text entered: AUTOMATION
Process finished with exit code 0
在上面的示例中,我们输入了文本 automation 并同时按下了 SHIFT 键,然后在控制台中获取了大写的输入文本并显示了消息 - Text entered: AUTOMATION 。
In the above example, we had entered the text automation along with SHIFT key press and then obtained the entered text in upper case with the message in the console - Text entered: AUTOMATION.
Conclusion
这就结束了我们对 Selenium WebDriver 处理特殊键教程的全面讲解。我们首先描述了一个使用诸如 CONTROL、SHIFT、TAB、CONTROL + A、CONTROL + V、CONTROL + C 等特殊键复制和粘贴文本的示例,并说明如何使用 Selenium 输入大写文本。这样做让你对在 Selenium WebDriver 中处理特殊键有了深入的了解。明智的做法是不断实践你所学到的知识并探索与 Selenium 相关的其他知识以加深你的理解并拓宽你的视野。
This concludes our comprehensive take on the tutorial on Selenium Webdriver Handle Special Keys. We’ve started with describing an example of copying and pasting text taking help of special keys like CONTROL, SHIFT, TAB, CONTROL + A, CONTROL + V, CONTROL + C, and so on, and illustrating how to input text in upper case with Selenium. This equips you with in-depth knowledge of handling special keys in Selenium Webdriver. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.