Selenium 简明教程
Selenium WebDriver - Multi Windows Testing
Selenium WebDriver 可用于处理多窗口测试。所有启动的窗口都通过会话的唯一标识符进行识别。随着另一个窗口的打开,驱动程序的上下文继续保留在父窗口。要在子窗口上执行任务,需要将驱动程序的上下文从父窗口转移到子窗口。
Selenium Webdriver can be used to handle multi-windows testing. All windows launched are identified by a unique identifier for a session. As another window opens, the context of the driver continues on the parent window. To execute tasks on a child window, the context of the driver needs to be shifted from the parent to the child.
Basic Methods to Handle Multiple Windows in Selenium
Selenium 中有各种方法可用于自动化处理多个窗口的测试。要处理子窗口,需要将驱动程序上下文从父窗口转移到子窗口 windows 。
There are various methods in Selenium that can be used to automate tests dealing with multiple windows. To work on the child windows, the driver context needs to be shifted from the parent window to the child windows.
Example 1
在下面的页面中,单击 New Tab 。
In the below page, click on New Tab.

在单击 New Tab 后,我们将转到带有文本 New Tab 的另一个选项卡。
Post clicking the New Tab, we would move to another tab with text New Tab.
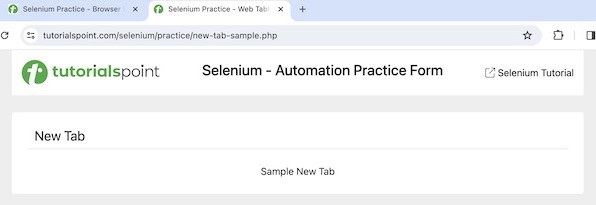
Code Implementation
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.edge.EdgeDriver;
import java.util.Set;
import java.util.concurrent.TimeUnit;
public class TabsHandling {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new EdgeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage with new tab
driver.get("https://www.tutorialspoint.com/selenium/practice/browser-windows.php");
// click link then navigate to next tab
WebElement b = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/button[1]"));
b.click();
// Get original window handle id
String oW = driver.getWindowHandle();
// get every windows handle ids
Set<String> windows = driver.getWindowHandles();
// loop through all window handles
for (String w : windows) {
if(!oW.equalsIgnoreCase(w)) {
// switch to the child tab
driver.switchTo().window(w);
// get element in new tab
WebElement e = driver.findElement(By.xpath("/html/body/main/div/div/h1"));
System.out.println("Text in new tab: " + e.getText());
break;
}
}
// quit the browser
driver.quit();
}
}
Text in new tab is: New Tab
Process finished with exit code 0
在这里,我们获取了新打开选项卡上的文本,并在控制台中收到了消息 - Text in new tab: New Tab 。
Here, we had obtained the text on the newly opened tab and got the message in the console - Text in new tab: New Tab.
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 2
在下面的页面中,单击 New Window Message 。
In the below page, click on New Window Message.
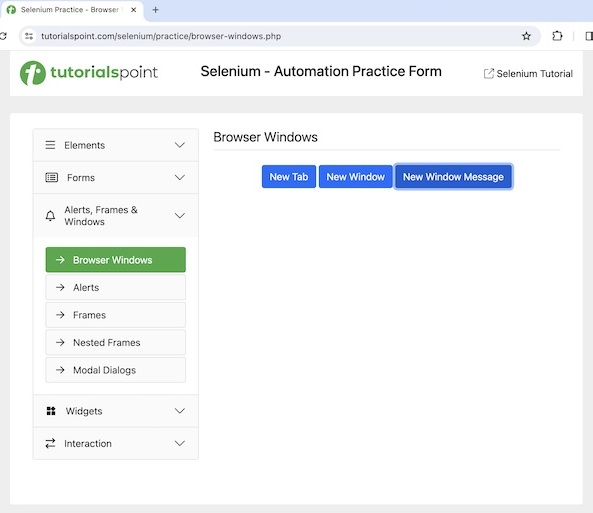
在单击 New Window Message 后,我们将转到另一个带有文本 New Window Message 的窗口。
Post clicking the New Window Message, we would move to another window with text New Window Message.
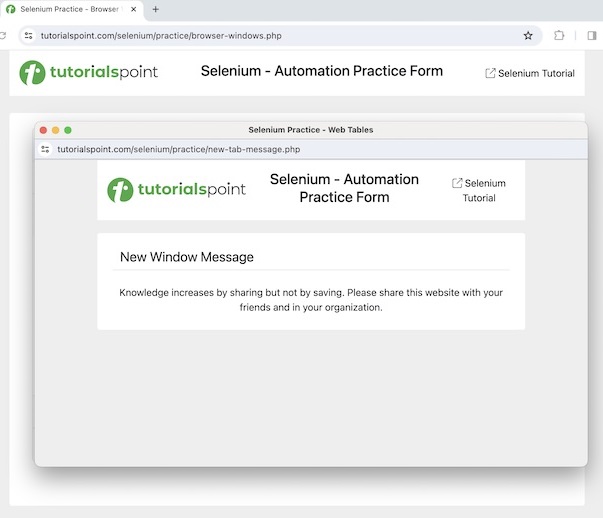
关闭新窗口,返回原始窗口,然后获取那里的文本 - Browser Windows 。最后,退出会话。
Close the new window, shift back to the original window, and obtain the text - Browser Windows there. At last, quit the session.
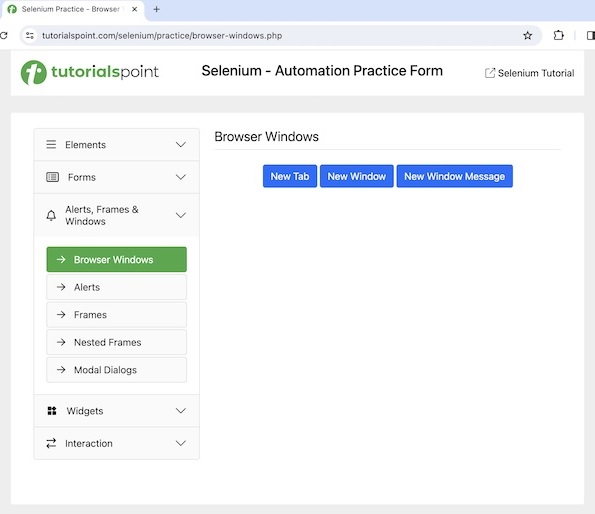
Code Implementation
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.edge.EdgeDriver;
import java.util.Set;
import java.util.concurrent.TimeUnit;
public class WindowsOpen {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new EdgeDriver();
// adding implicit wait of 20 secs
driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS);
// Opening the webpage to open a new window
driver.get("https://www.tutorialspoint.com/selenium/practice/browser-windows.php");
// click link to next window
WebElement b = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/button[3]"));
b.click();
// Obtain original window handle id
String oW = driver.getWindowHandle();
// obtain all opened windows handle ids
Set<String> windows = driver.getWindowHandles();
// Loop through all window handles
for (String w : windows) {
if(!oW.equalsIgnoreCase(w)) {
// switch to child window
driver.switchTo().window(w);
// get element in new window
WebElement e = driver.findElement(By.xpath("/html/body/main/div/div/h1"));
System.out.println("Text in new window: " + e.getText());
driver.close();
break;
}
}
// switch to parent window
driver.switchTo().window(oW);
// get element in parent window
WebElement e1 = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/h1"));
System.out.println("Text in parent window: " + e1.getText());
// quit browser session
driver.quit();
}
}
Text in new window: New Window Message
Text in parent window: Browser Windows
在这里,我们捕获了新窗口上的文本,并在控制台中收到了消息 - Text in new window: New Window Message 。然后,我们关闭了子窗口,并返回父窗口。最后,我们已在控制台中获取父窗口上的文本 - Text in parent window: Browser Windows 。
Here, we had captured text on the new window and got the message in the console - Text in new window: New Window Message. Then, we ended the child window and moved back to the parent window. At last, we had obtained text on the parent window in the console - Text in parent window: Browser Windows.
因此,close() 和 quit() 方法之间存在较小差异。close() 方法仅关闭活动浏览器窗口,quit() 方法同时终止所有打开的浏览器窗口。
Thus there is a minor difference between close() and quit() methods. The close() method only closes the active browser window, and the quit() method terminates all the opened browser windows at the same time.
Example 3
从 4 版本开始,我们可以使用以下方法打开新窗口−
From the version 4, we can open a new window using the below method −
driver.switchTo().newWindow(WindowType.WINDOW)
在浏览器窗口中打开应用程序,并获取文本 - 复选框,如下图所示−
Open an application in a browser window, and obtain text - Check Box as shown in the below image −
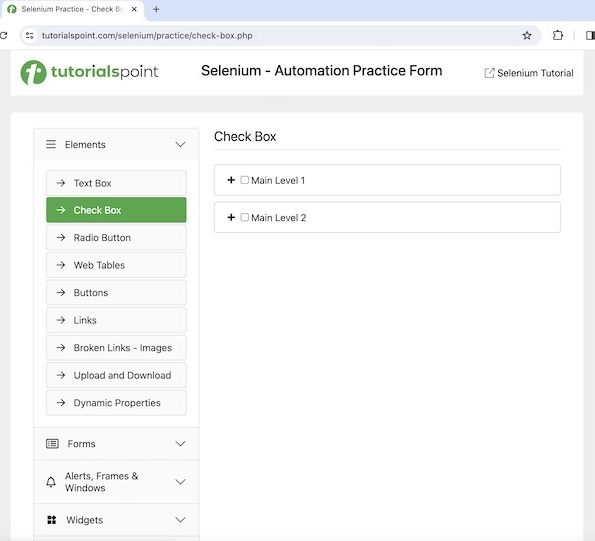
然后打开另一个新窗口并启动文本为 - Radio Button 的不同应用程序。
Then open another new window and launch a different application with text - Radio Button.
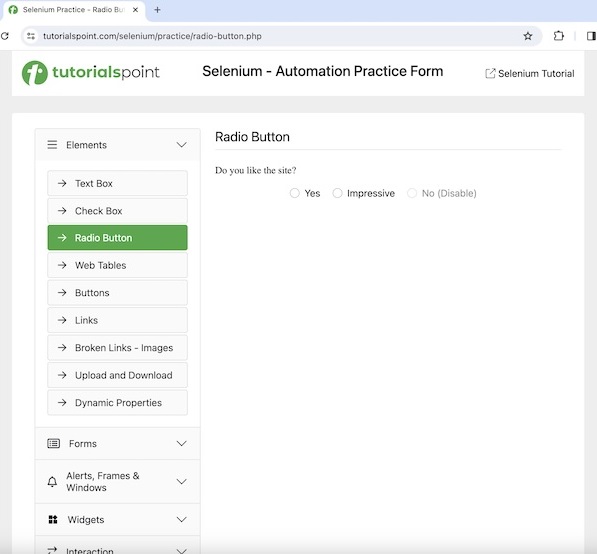
Code Implementation
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.WindowType;
import org.openqa.selenium.edge.EdgeDriver;
import java.util.concurrent.TimeUnit;
public class NewWindowOpen {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new EdgeDriver();
// adding implicit wait of 12 secs
driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS);
// Open a webpage in first window
driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php");
// get text in first window
WebElement e = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/h1"));
System.out.println("Text: " + e.getText());
// Initiate the another Webdriver
WebDriver newDriver = driver.switchTo().newWindow(WindowType.WINDOW);
// Opening another webpage in second window
driver.get("https://www.tutorialspoint.com/selenium/practice/radio-button.php");
// get text in second window
WebElement e1 = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/form/h1"));
System.out.println("Text in new window: " + e1.getText());
// quit the browser session
driver.quit();
}
}
Text: Check Box
Text in new window: Radio Button
在这里,我们已使用控制台中的消息 - Text: Check Box 捕获了第一个窗口中的文本。然后打开另一个新窗口以启动应用程序。最后,借助控制台中的消息 - Text in new window: Radio Button ,我们在新窗口中获取了文本。
Here, we had captured text in the first window with the message in the console - Text: Check Box. Then opened another new window to launch an application there. At last, we had obtained text in the new window with a message in the console - Text in new window: Radio Button.
Example 4
从 4 版本开始,我们可以使用以下方法打开新选项卡−
From the version 4, we can open a new tab using the below method −
driver.switchTo().newWindow(WindowType.TAB)
在浏览器中打开应用程序,并获取文本 - 复选框,如下图所示−
Open an application in a browser, and obtain text - Check Box as shown in the below image −
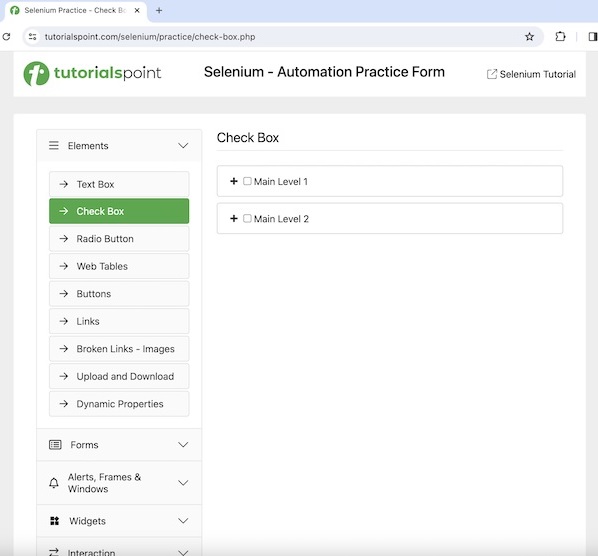
然后打开另一个新选项卡并启动文本为 - Radio Button 的不同应用程序。
Then open another new tab and launch a different application with text - Radio Button.
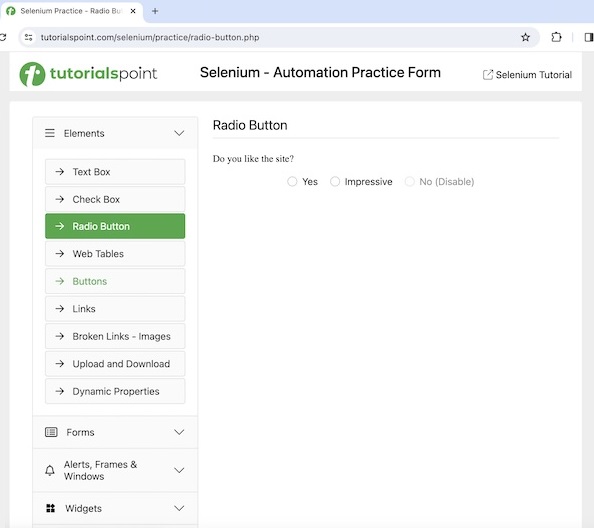
Code Implementation
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.WindowType;
import org.openqa.selenium.edge.EdgeDriver;
import java.util.concurrent.TimeUnit;
public class NewTabsOpen {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new EdgeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Open a webpage in first window
driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php");
// get text in first window
WebElement e = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/h1"));
System.out.println("Text: " + e.getText());
// Initiate the another Webdriver
WebDriver newDriver = driver.switchTo().newWindow(WindowType.TAB);
// Open a webpage in new tab
driver.get("https://www.tutorialspoint.com/selenium/practice/radio-button.php");
// obtain text in other tab
WebElement e1 = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/form/h1"));
System.out.println("Text in other tab: " + e1.getText());
// quit the browser session
driver.quit();
}
}
Text: Check Box
Text in other tab: Radio Button
在这里,我们已使用控制台中的消息 - Text: Check Box 捕获了第一个窗口中的文本。然后打开另一个新选项卡以启动应用程序。最后,借助控制台中的消息 - Text in other tab: Radio Button ,我们在新选项卡中获取了文本。
Here, we had captured the text in the first window with the message in the console - Text: Check Box. Then opened another new tab to launch an application there. Atlast, we obtained text in the new tab with the message in the console - Text in other tab: Radio Button.
Conclusion
这总结了我们在 Selenium Webdriver 多窗口测试教程中的全面介绍。我们首先描述了使用 Selenium 处理多个窗口的基本方法,并逐步介绍了使用 Selenium Webdriver 处理多个窗口的示例。这让你深入了解 Selenium Webdriver - 多窗口测试。明智的做法是不断练习你所学知识,探索与 Selenium 相关的其他知识,以加深理解并拓展视野。
This concludes our comprehensive take on the tutorial on Selenium Webdriver Multi Windows Testing. We’ve started with describing basic methods to handle multiple windows in Selenium, and walked through examples on how to handle multiple windows with Selenium Webdriver. This equips you with in-depth knowledge of the Selenium Webdriver - Multi Windows Testing. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.