Selenium 简明教程
Selenium with Python Tutorial
Selenium is used for developing test cases for mostly web based applications. It can be used with various programming languages like Java, Python, Kotlin, and so on.
Setup Selenium with Python and Launch Browser
Step 1 − 从链接 Latest Version for Windows 下载并安装 Python。
Step 1 − Download and install Python from the link Latest Version for Windows.
要详细了解如何设置 Python,请参考链接 Python Environment Setup 。
To get a more detailed view on how setup Python, refer to the link Python Environment Setup.
成功安装 Python 后,从命令提示符运行以下命令 python –version ,以确认其安装。执行的命令的输出将指向安装在计算机中的 Python 版本。
Once we have successfully installed Python, confirm its installation by running the command: python –version, from the command prompt. The output of the command executed would point to the Python version installed in the machine.
Step 2 − 从链接 PyCharm 安装名为 PyCharm 的 Python 代码编辑器。
Step 2 − Install the Python code editor called the PyCharm from the link PyCharm.
使用此编辑器,我们可以开始研究一个 Python 项目以启动我们的测试自动化。要详细了解如何设置 PyCharm,请参考以下链接 Pycharm Tutorial 。
Using this editor, we can start working on a Python project to start our test automation. To get a more detailed view on how to set up PyCharm, refer to the below link Pycharm Tutorial.
Step 3 − 从命令提示符运行命令 pip install selenium 。这样可以安装 Selenium。要确认机器中安装的 Selenium 版本,请运行命令 −
Step 3 − Run the command: pip install selenium from the command prompt. This is done to install the Selenium. To confirm the version of Selenium installed in the machine, run the command −
pip show selenium
此命令的输出给出了以下结果 −
The output of this command gave the below result −
Name: selenium
Version: 4.19.0
Summary: None
Home-page: https://www.selenium.dev
Author: None
Author-email: None
License: Apache 2.0.
Step 4 − 完成步骤 3 后重新启动 PyCharm。
Step 4 − Restart PyCharm once Step 3 has been completed.
Step 5 − 打开 PyCharm 并通过导航到文件菜单创建新项目。
Step 5 − Open PyCharm and create a New Project by navigating to the File menu.
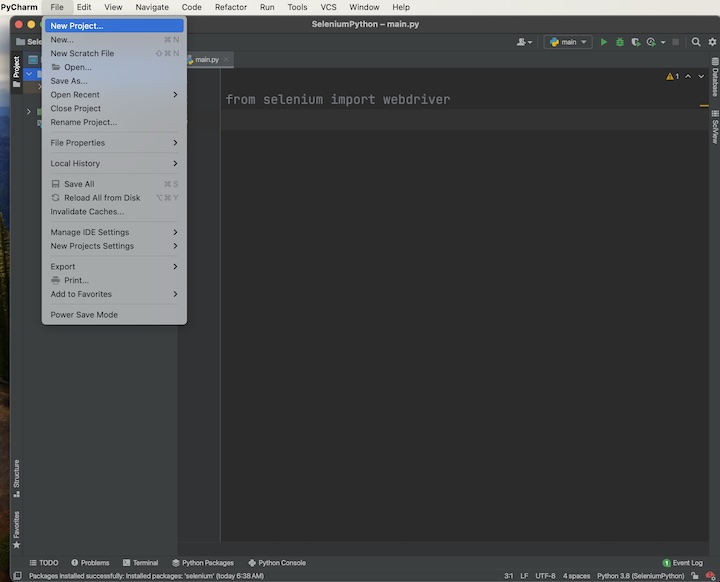
在“位置”字段中输入项目名称和位置,然后点击“创建”按钮。
Enter the project name and location within the Location field, and then click on the Create button.
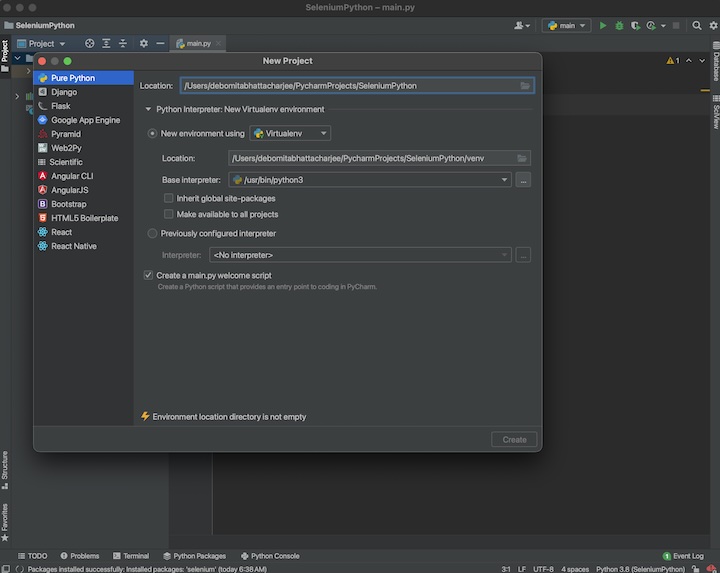
Step 6 − 从 PyCharm 编辑器的右下角,选择“解释器设置”选项。
Step 6 − From the right bottom corner of the PyCharm editor, select the option Interpreter Settings.
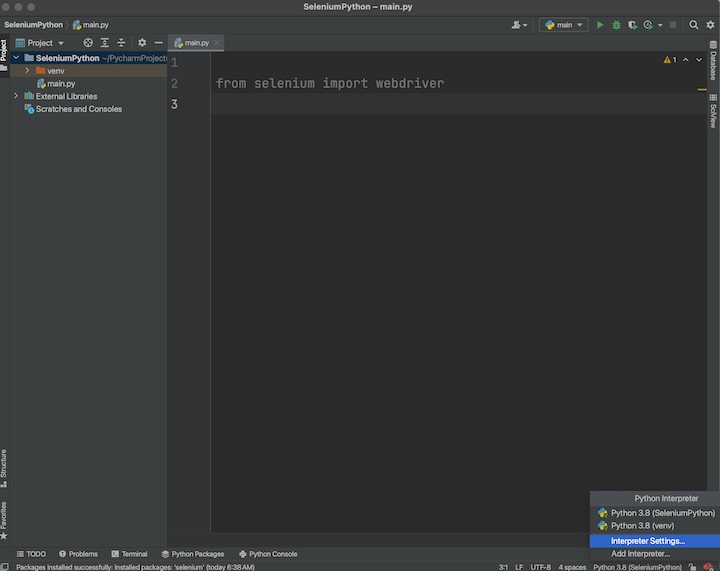
从左侧选择 Python 解释器选项,然后单击“+”。
Select the option Python Interpreter from the left, then click on '+'.
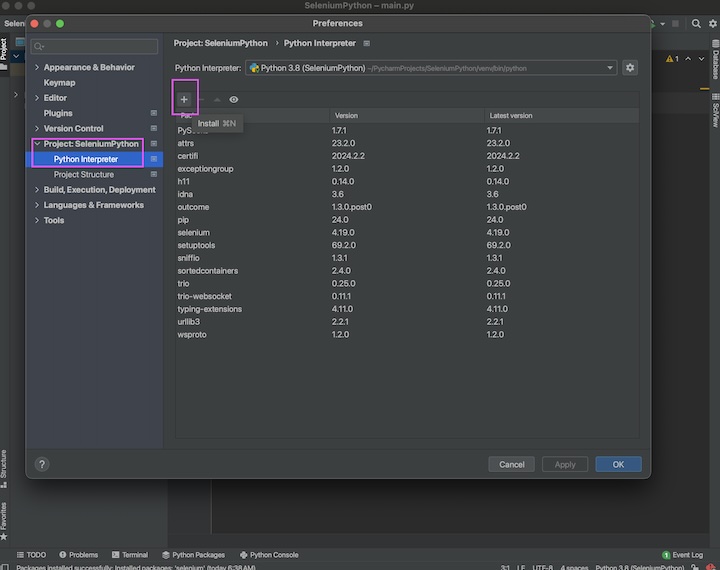
Step 7 − 在可用软件包弹出窗口中的包搜索框中输入“selenium”,然后搜索结果将与右侧说明一起出现。说明包含有关要安装的 Selenium 软件包版本的的信息。
Step 7 − Enter selenium in the package search box inside the Available Packages popup, then the search results appear along with the Description to the right. The Description contains information about the version of the Selenium package that shall be installed.
还可以选择在指定版本字段旁边安装特定版本的 Selenium 软件包。然后单击“安装软件包”按钮。安装成功后,应显示消息“软件包‘selenium’已成功安装”。
There is also an option to install a specific version of the Selenium package beside the Specify version field. Then click on the Install Package button. After successful installation, the message Package 'selenium' installed successfully should display.

Step 8 - 在可用软件包弹出窗口中的软件包搜索框中输入 webdriver-manager,并以相同方式安装它。
Step 8 − Enter webdriver-manager in the package search box inside the Available Packages popup, and install it in the same way.
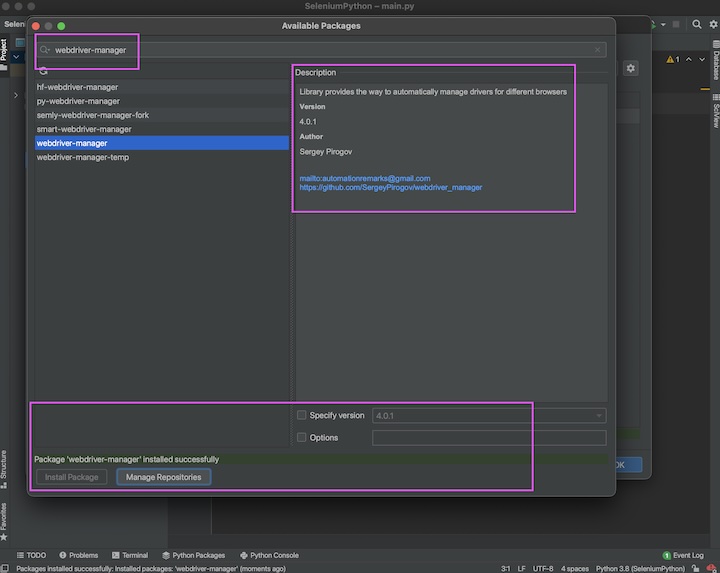
退出“可用包”弹出窗口。
Exit out of the Available Packages popup.
Step 9 - selenium 和 webdriver-manager 这两个软件包都应反映在程序包下。单击确定按钮。重新启动 PyCharm。
Step 9 − Both the packages selenium, and webdriver-manager should reflect under the Package. Click on the OK button. Restart PyCharm.
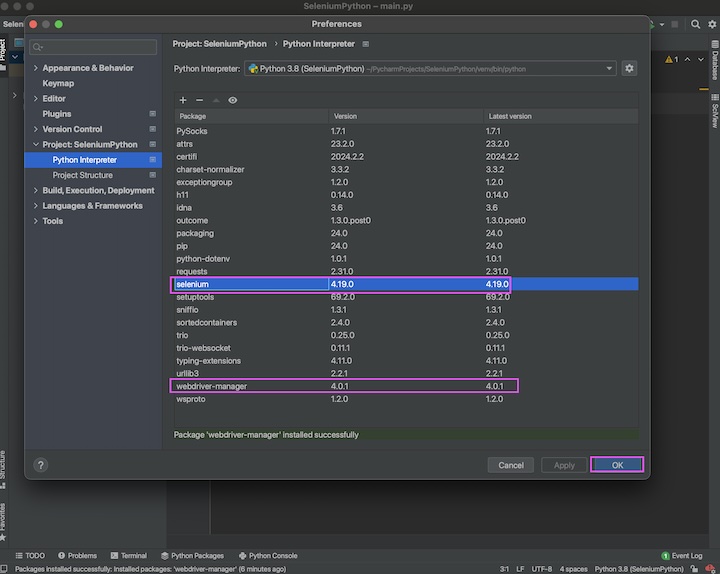
Step 10 - 右键单击项目文件夹创建第一个测试用例。在这里,我们已将项目名称指定为 SeleniumPython。然后单击新建,最后单击 Python 文件选项。
Step 10 − Create the first test case, by right clicking on the Project folder. Here, we have given the project name as SeleniumPython. Then click on New and finally click on the Python File option.
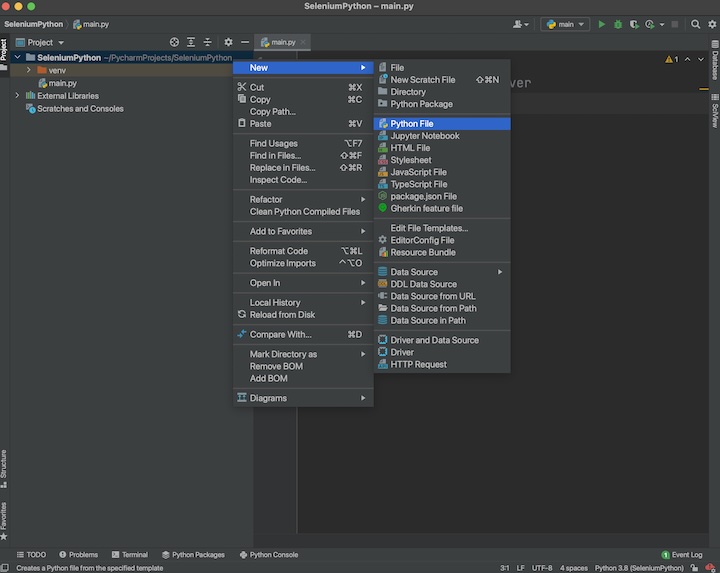
Step 11 - 输入一个文件名(例如 Test1.py),然后选择 Python 文件选项,最后单击 Enter。
Step 11 − Enter a filename, say Test1.py and select the option Python File, finally click on Enter.
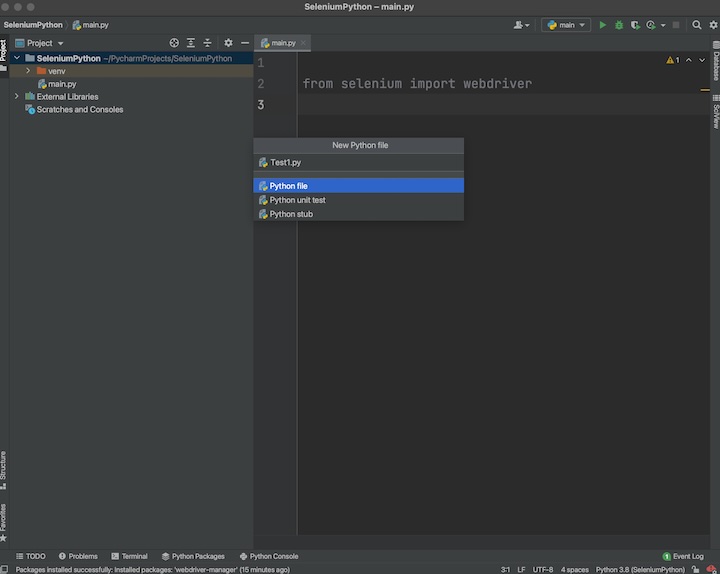
Test1.py 应出现在 SeleniumPython 项目文件夹下的左侧。
The Test1.py should appear in the left under the SeleniumPython project folder.
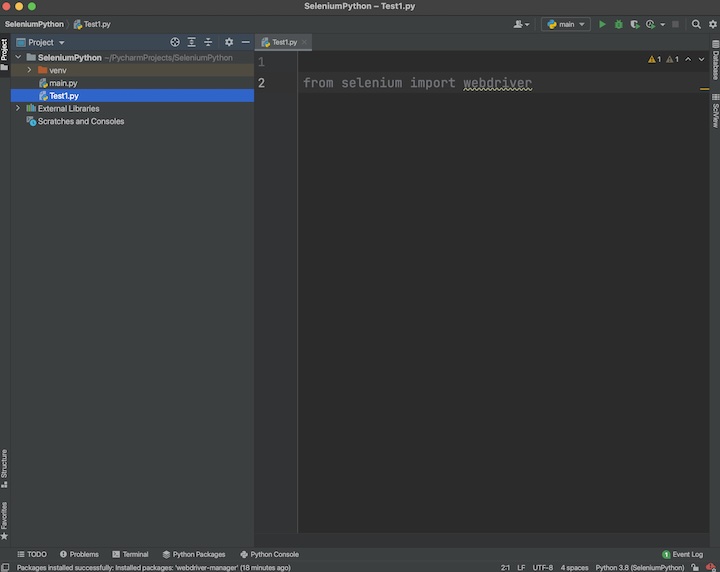
Step 12 - 打开新创建的 Python 文件(名称为 Test1.py),并获取下面页面的标题 - Selenium Practice - Alerts 。
Step 12 − Open the newly created Python file with the name Test1.py, and obtain the page title - Selenium Practice - Alerts of the below page.
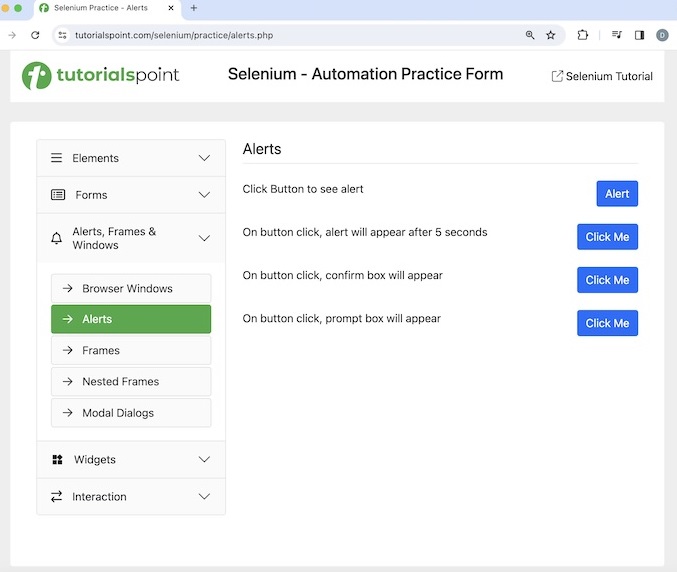
Example
from selenium import webdriver
# create instance of webdriver
driver = webdriver.Chrome()
# launch application
driver.get("https://www.tutorialspoint.com/selenium/practice/alerts.php")
# get page title
print("Page title is: " + driver.title)
# quitting browser
driver.quit
Page title is: Selenium Practice - Alerts
Process finished with exit code 0
在上例中,我们启动了一个应用程序,并通过消息 - Page title is: Selenium Practice - Alerts 在控制台中获取了其页面标题。
In the above example, we had launched an application and obtained its page title in the console with the message - Page title is: Selenium Practice - Alerts.
输出显示消息 - Process with exit code 0 ,这意味着上述代码已成功执行。
The output shows the message - Process with exit code 0 meaning that the above code executed successfully.
Identify Element and Check Its Functionality using Selenium Python
当应用程序启动时,用户在网页上对网页元素执行操作,比如单击链接或按钮、在输入框内输入文本、提交表单,等等,以自动化测试用例。
As an application gets launched, the users perform actions on the web elements on the web page like clicking on a link or a button, entering text within an input box, submitting a form, and so on for automating the test cases.
第一步是定位元素。Selenium 中提供了各种定位器,如 id、classname、class、name、tagname、partial link text、link text、tagname、xpath 和 css。这些定位器与 Python 中的 find_element() 方法搭配使用。
The first step is to locate the elements. There are various locators available in Selenium like the id, class name, class, name, tagname, partial link text, link text, tagname, xpath, and css. These locators are used with the find_element() method in Python.
例如,driver.find_element("classname", 'btn-primary') 定位类名称属性值为 btn-primary 的第一个元素。如果找不到具有此类名称属性匹配值的元素,则抛出 NoSuchElementException。
For example, driver.find_element("classname", 'btn-primary') locates the first element with the class name attribute value as btn-primary. In case there is no element with that matching value of the class name attribute, NoSuchElementException is thrown.
我们来看看下面的图片中 Click Me 按钮的 html 代码 -
Let us see the html code of the Click Me button highlighted in the below image −
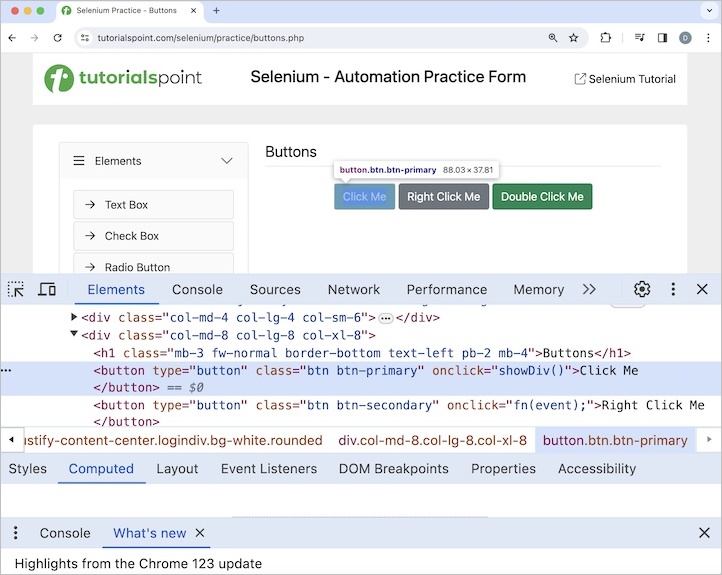
其类名称属性的值为 btn-primary 。单击点击我按钮,之后文本 You have done a dynamic click 会出现在页面上。
Its class name attribute with value btn-primary. Click on Click Me button, post that the text You have done a dynamic click appears on the page.
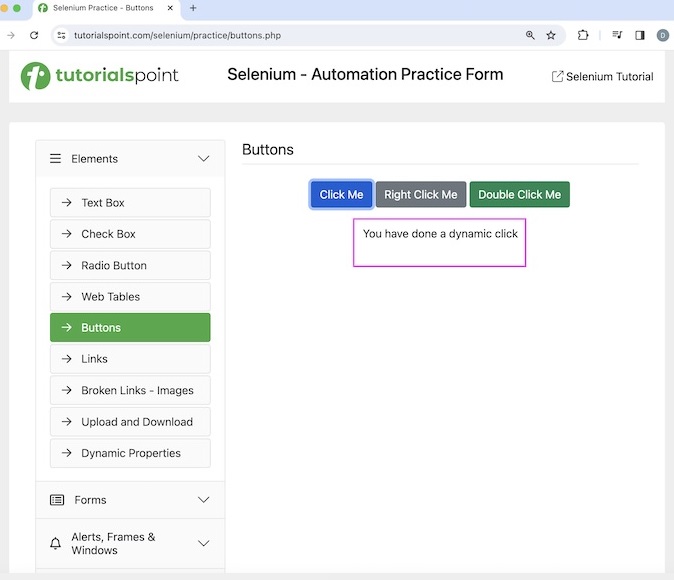
Example
from selenium import webdriver
from selenium.webdriver.common.by import By
# create instance of webdriver
driver = webdriver.Chrome()
# implicit wait of 15 seconds
driver.implicitly_wait(15)
# launch application
driver.get("https://www.tutorialspoint.com/selenium/practice/buttons.php")
# identify element with class name
button = driver.find_element(By.CLASS_NAME, 'btn-primary')
# click on button
button.click()
# identify element with id
txt = driver.find_element(By.ID, 'welcomeDiv')
# get text
print("Text obtained is: " + txt.text)
# quitting browser
driver.quit
Text obtained is: You have done a dynamic click
我们在单击 Click Me 后使用控制台中的消息获取了文本 - Text obtained is: You have done a dynamic click 。
We had retrieved the text after clicking Click Me with the message in the console - Text obtained is: You have done a dynamic click.
Conclusion
这结束了我们对 Selenium Python 教程教程的全面了解。我们从描述如何使用 Python 设置 Selenium 和启动浏览器开始,以及如何识别元素并使用 Selenium Python 检查其功能。这会使你深入了解 Selenium Python 教程。明智做法是反复练习所学知识并探索其他与 Selenium 相关的知识,以加深理解并拓宽视野。
This concludes our comprehensive take on the tutorial on Selenium Python Tutorial. We’ve started with describing how to set up Selenium with Python and launch a browser, and how to identify an element and check its functionality using Selenium Python. This equips you with in-depth knowledge of the Selenium Python Tutorial. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.