Wpf 简明教程
WPF - Exception Handling
异常是在程序执行期间遇到的任何错误条件或意外行为。异常可以因多种原因而引发,其中一些原因如下:
An exception is any error condition or an unexpected behavior that is encountered during the execution of a program. Exceptions can be raised due to many reasons, some of them are as follows −
-
Fault in your code or in code that you call (such as a shared library),
-
Unavailable operating system resources,
-
Unexpected conditions that a common language runtime encounters (such as code that cannot be verified)
Syntax
异常有能力将程序的流程从一部分转移到另一部分。在 .NET 框架中,异常处理有以下四个关键字:
Exceptions have the ability to transfer the flow of a program from one part to another. In .NET framework, exception handling has the following four keywords −
-
try − In this block, the program identifies a certain condition which raises some exception.
-
catch − The catch keyword indicates the catching of an exception. A try block is followed by one or more catch blocks to catch an exception with an exception handler at the place in a program where you want to handle the problem.
-
finally − The finally block is used to execute a given set of statements, whether an exception is thrown or not thrown. For example, if you open a file, it must be closed whether an exception is raised or not.
-
throw − A program throws an exception when a problem shows up. This is done using a throw keyword.
使用这四个关键字的语法如下:
The syntax to use these four keywords goes as follows −
try {
///This will still trigger the exception
}
catch (ExceptionClassName e) {
// error handling code
}
catch (ExceptionClassName e) {
// error handling code
}
catch (ExceptionClassName e) {
// error handling code
}
finally {
// statements to be executed
}
在根据程序流的情况,一个 try 块可以引发多个异常的那些情况下,会使用多个 catch 语句。
Multiple catch statements are used in those cases where a try block can raise more than one exception depending on the situation of a program flow.
Hierarchy
Almost all the exception classes in the .NET framework are directly or indirectly derived from the Exception class. The most important exception classes derived from the Exception class are −
-
ApplicationException class − It supports exceptions which are generated by programs. When developer want to define exception then class should be derived from this class.
-
SystemException class − It is the base class for all predefined runtime system exceptions. The following hierarchy shows the standard exceptions provided by the runtime.

下表列出了运行时提供的标准异常和您应该创建派生类的条件。
The following table lists the standard exceptions provided by the runtime and the conditions under which you should create a derived class.
Exception type |
Base type |
Description |
Exception |
Object |
Base class for all exceptions. |
SystemException |
Exception |
Base class for all runtime-generated errors. |
IndexOutOfRangeException |
SystemException |
Thrown by the runtime only when an array is indexed improperly. |
NullReferenceException |
SystemException |
Thrown by the runtime only when a null object is referenced. |
AccessViolationException |
SystemException |
Thrown by the runtime only when invalid memory is accessed. |
InvalidOperationException |
SystemException |
Thrown by methods when in an invalid state. |
ArgumentException |
SystemException |
Base class for all argument exceptions. |
ArgumentNullException |
ArgumentException |
Thrown by methods that do not allow an argument to be null. |
ArgumentOutOfRangeException |
ArgumentException |
Thrown by methods that verify that arguments are in a given range. |
ExternalException |
SystemException |
Base class for exceptions that occur or are targeted at environments outside the runtime. |
SEHException |
ExternalException |
Exception encapsulating Win32 structured exception handling information. |
Example
让我们用一个简单的示例更深入地理解这个概念。首先创建一个带有名称 WPFExceptionHandling 的新 WPF 项目。
Let’s take a simple example to understand the concept better. Start by creating a new WPF project with the name WPFExceptionHandling.
将一个文本框从工具箱拖拽到设计窗口。以下 XAML 代码创建一个文本框并用一些属性对其进行初始化。
Drag one textbox from the toolbox to the design window. The following XAML code creates a textbox and initializes it with some properties.
<Window x:Class = "WPFExceptionHandling.MainWindow"
xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d = "http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local = "clr-namespace:WPFExceptionHandling"
mc:Ignorable = "d"
Title = "MainWindow" Height = "350" Width = "604">
<Grid>
<TextBox x:Name = "textBox" HorizontalAlignment = "Left"
Height = "241" Margin = "70,39,0,0" TextWrapping = "Wrap"
VerticalAlignment = "Top" Width = "453"/>
</Grid>
</Window>
下面是在 C# 中使用异常处理来进行文件读取。
Here is the file reading with exception handling in C#.
using System;
using System.IO;
using System.Windows;
namespace WPFExceptionHandling {
public partial class MainWindow : Window {
public MainWindow() {
InitializeComponent();
ReadFile(0);
}
void ReadFile(int index) {
string path = @"D:\Test.txt";
StreamReader file = new StreamReader(path);
char[] buffer = new char[80];
try {
file.ReadBlock(buffer, index, buffer.Length);
string str = new string(buffer);
str.Trim();
textBox.Text = str;
}
catch (Exception e) {
MessageBox.Show("Error reading from "+ path + "\nMessage = "+ e.Message);
}
finally {
if (file != null) {
file.Close();
}
}
}
}
}
当你编译并执行以上代码时,它将在其中一个文本框内显示一个文本的窗口。
When you compile and execute the above code, it will produce the following window in which a text is displayed inside the textbox.

当抛出一个异常或手动抛出一个异常时(如以下代码中所示),它会显示一个带错误的消息框。
When there is an exception raised or you throw it manually (as in the following code), then it will show a message box with error.
using System;
using System.IO;
using System.Windows;
namespace WPFExceptionHandling {
public partial class MainWindow : Window {
public MainWindow() {
InitializeComponent();
ReadFile(0);
}
void ReadFile(int index) {
string path = @"D:\Test.txt";
StreamReader file = new StreamReader(path);
char[] buffer = new char[80];
try {
file.ReadBlock(buffer, index, buffer.Length);
string str = new string(buffer);
throw new Exception();
str.Trim();
textBox.Text = str;
}
catch (Exception e) {
MessageBox.Show("Error reading from "+ path + "\nMessage = "+ e.Message);
}
finally {
if (file != null) {
file.Close();
}
}
}
}
}
当在执行以上代码时抛出一个异常时,它将显示以下消息。
When an exception is raised while executing the above code, it will display the following message.
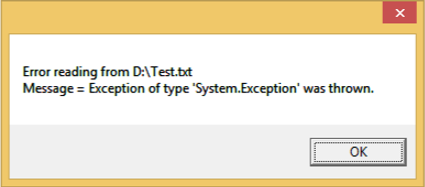
我们建议你执行以上的代码并体验其功能。
We recommend that you execute the above code and experiment with its features.