Xamarin 简明教程
Xamarin - Android Dialogs
Alert Dialog
在本节中,我们将创建一个按钮,单击后显示一个警示对话框。对话框包含两个按钮,即 Delete 和 Cancel 按钮。
In this section, we are going to create a button which on clicked displays an alert dialog box. The dialog box contains two buttons, i.e., Delete and Cancel buttons.
首先,转至 main.axml 并创建线性布局内的按钮,如下面的代码所示。
First of all, go to main.axml and create a new button inside the linear layout as shown in the following code.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:layout_width = "fill_parent"
android:background = "#d3d3d3"
android:layout_height = "fill_parent">
<Button
android:id="@+id/MyButton"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:text = "Click to Delete"
android:textColor = "@android:color/background_dark"
android:background = "@android:color/holo_green_dark" />
</LinearLayout>
接下来,打开 MainActivity.cs 来创建警示对话框并添加其功能。
Next, open MainActivity.cs to create the alert dialog and add its functionality.
protected override void OnCreate(Bundle bundle) {
base.OnCreate(bundle);
SetContentView(Resource.Layout.Main);
Button button = FindViewById<Button>(Resource.Id.MyButton);
button.Click += delegate {
AlertDialog.Builder alertDiag = new AlertDialog.Builder(this);
alertDiag.SetTitle("Confirm delete");
alertDiag.SetMessage("Once deleted the move cannot be undone");
alertDiag.SetPositiveButton("Delete", (senderAlert, args) => {
Toast.MakeText(this, "Deleted", ToastLength.Short).Show();
});
alertDiag.SetNegativeButton("Cancel", (senderAlert, args) => {
alertDiag.Dispose();
});
Dialog diag = alertDiag.Create();
diag.Show();
};
}
完成后,构建并运行你的应用程序来查看输出。
Once done, build and run your Application to view the outcome.
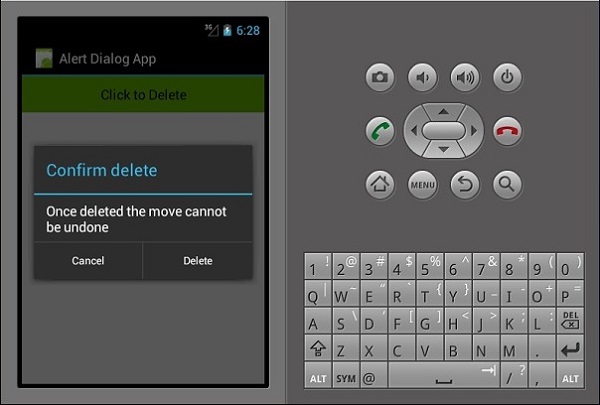
在上面的代码中,我们创建了一个称为 alertDiag 的警报对话框,它具有以下两个按钮 -
In the above code, we have created an alert dialog called alertDiag, with the following two buttons −
-
setPositiveButton − It contains the Delete button action which on clicked displays a confirmation message Deleted.
-
setNegativeButton − It contains a Cancel button which when clicked simply closes the alert dialog box.