Xamarin 简明教程
Xamarin - Android Resources
创建新的 Android 项目时,会默认将一些文件添加到项目中。我们将这些默认项目文件和文件夹称为 Android Resources 。查看以下屏幕截图。
When a new Android project is created, there are some files that are added to the project, by default. We call these default project files and folders as Android Resources. Take a look at the following screenshot.
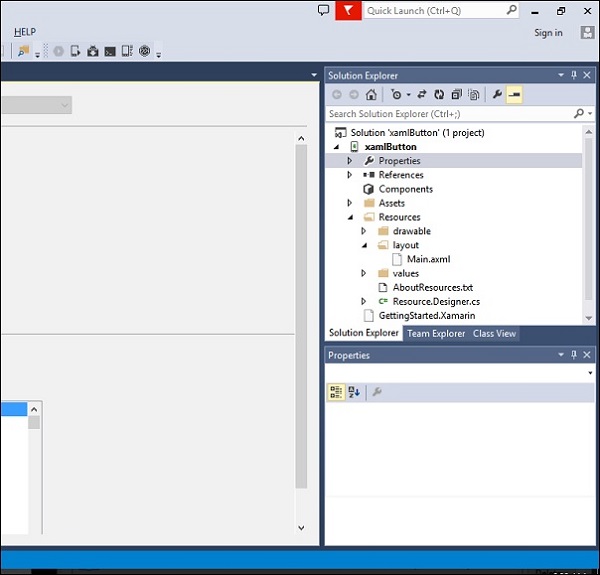
默认 Android 资源包括以下内容 −
The default Android resources include the following −
-
AndroidManifest.xml file − It contains information about your Android applications, e.g., the application name, permissions, etc.
-
Resources folder − Resources can be images, layouts, strings, etc. that can be loaded via Android’s resource system.
-
Resources/drawable folder − It stores all the images that you are going to use in your application.
-
Resources/layout folder − It contains all the Android XML files (.axml) that Android uses to build user interfaces.
-
The Resources/values folder − It contains XML files to declare key-value pairs for strings (and other types) throughout an application. This is how localization for multiple languages is normally set up on Android.
-
Resources.designer.cs − This file is created automatically when the Android projected is created and it contains unique identifiers that reference the Android resources.
-
MainActivity.cs file − This is the first activity of your Android application and from where the main application actions are launched from.
可以使用 unique ID 来以编程方式访问资源文件,该文件存储在 resources.designer.cs 文件中。该 ID 包含在一个名为 Resource 的类中。添加到项目中的任何资源都会自动生成在 resource class 中。
Resource files can be accessed programmatically through a unique ID which is stored in the resources.designer.cs file. The ID is contained under a class called Resource. Any resource added to the project is automatically generated inside the resource class.
下面的代码展示了如何创建一个包含七张图片的网格视图项目:
The following code shows how to create a gridview project containing seven images −
namespace HelloGridView {
[System.CodeDom.Compiler.GeneratedCodeAttribute
("Xamarin.Android.Build.Tas ks",
"1.0.0.0")]
public partial class Resource {
static Resource() {
global::Android.Runtime.ResourceIdManager.UpdateIdValues();
}
public static void UpdateIdValues() {}
public partial class Attribute {
static Attribute() {
global::Android.Runtime.ResourceIdManager.UpdateIdValues();
}
private Attribute() {}
}
public partial class Drawable {
// aapt resource value: 0x7f020000
public const int Icon = 2130837504;
// aapt resource value: 0x7f020001
public const int img1 = 2130837505;
// aapt resource value: 0x7f020002
public const int img2 = 2130837506;
// aapt resource value: 0x7f020003
public const int img3 = 2130837507;
// aapt resource value: 0x7f020004
public const int img4 = 2130837508;
// aapt resource value: 0x7f020005
public const int img5 = 2130837509;
// aapt resource value: 0x7f020006
public const int img6 = 2130837510;
// aapt resource value: 0x7f020007
public const int img7 = 2130837511;
static Drawable() {
global::Android.Runtime.ResourceIdManager.UpdateIdValues();
}
private Drawable() {}
}
public partial class Id {
// aapt resource value: 0x7f050000
public const int gridview = 2131034112;
static Id() {
global::Android.Runtime.ResourceIdManager.UpdateIdValues();
}
private Id() {}
}
public partial class Layout {
// aapt resource value: 0x7f030000
public const int Main = 2130903040;
static Layout() {
global::Android.Runtime.ResourceIdManager.UpdateIdValues();
}
private Layout() {}
}
public partial class String {
// aapt resource value: 0x7f040001
public const int ApplicationName = 2130968577;
// aapt resource value: 0x7f040000
public const int Hello = 2130968576;
static String() {
global::Android.Runtime.ResourceIdManager.UpdateIdValues();
}
private String() {}
}
}
}
从上面的代码中,七张照片被引用在一个名为 drawable 的类中。这些图片是通过编程方式添加的。如果用户向项目中添加另一张图片,它也将被添加到 drawable 类。项目中包含的 gridview 也被添加并存储在它自己的一个类中。 resources folder 中包含的每个项目都会自动生成并存储在一个类中。
From the above code, the seven images are referenced in a class called drawable. These images are added programmatically. If a user adds another image to the project, it will also be added to the drawable class. The gridview contained in the project is also added and stored in a class on its own. Each item contained in the resources folder is automatically generated and stored in a class.