Xamarin 简明教程
Xamarin - First Application
在本章中,我们将了解如何使用 Xamarin 创建一个小型 Android 应用程序。
In this chapter, we will see how to create a small Android application using Xamarin.
Hello Xamarin! Application
首先,启动 Visual Studio 的一个新实例,然后转到 File → New → Project 。
First of all, start a new instance of Visual Studio and go to File → New → Project.
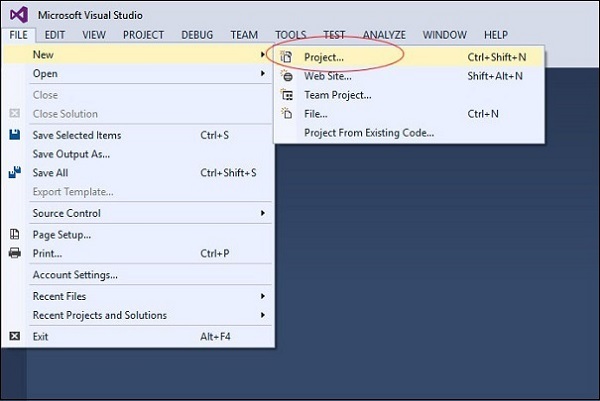
在出现的“菜单”对话框中,转到 Templates → Visual C# → Android → Blank App (Android) 。
On the Menu dialog box that appears, go to Templates → Visual C# → Android → Blank App (Android).
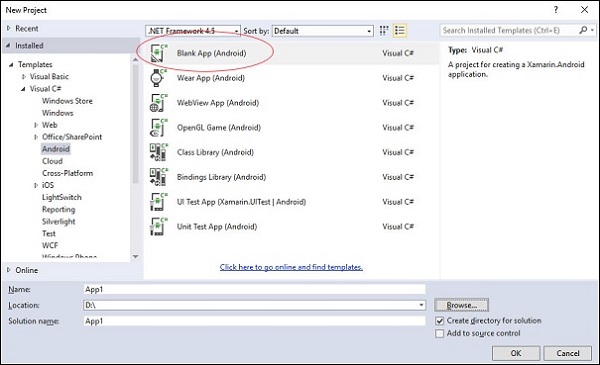
为你的应用程序起一个合适的名称。在我们的案例中,我们将其命名为 “helloWorld” ,并将其保存在提供的默认位置。接下来,单击“确定”按钮以加载新的 “helloXamarin” 项目。
Give an appropriate name for your application. In our case, we name it “helloWorld” and save it in the default location provided. Next, click the OK button for the new “helloXamarin” project to load.
在 solution 中,打开 Resources → layout → Main.axml 文件。从“设计视图”切换,并转到 Source 文件并键入以下代码行来构建你的应用程序。
On the solution, open Resources → layout → Main.axml file. Switch from Design View and go to the Source file and type the following lines of code to build your app.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:background = "#d3d3d3"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<TextView
android:text = "@string/HelloXamarin"
android:textAppearance = "?android:attr/textAppearanceLarge"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/textView2"
android:textColor = "@android:color/black" />
</LinearLayout>
在以上代码中,我们创建了一个新的 Android textview 。接下来,打开“values”文件夹,并双击 Strings.xml 以打开它。在此,我们将存储以上创建的 button 中的信息和值。
In the above code, we have created a new Android textview. Next, open the folder values and double-click Strings.xml to open it. Here, we are going to store information and values about the button created above.
<?xml version = "1.0" encoding = "utf-8"?>
<resources>
<string name = "HelloXamarin">Hello World, I am Xamarin!</string>
<string name = "ApplicationName">helloWorld</string>
</resources>
打开 MainActivity.cs 文件,并将现有代码替换为以下代码行。
Open MainActivity.cs file and replace the existing code with the following lines of code.
using System;
using Android.App;
using Android.Content;
using Android.Runtime;
using Android.Views;
using Android.Widget;
using Android.OS;
namespace HelloXamarin {
public class MainActivity : Activity {
protected override void OnCreate(Bundle bundle) {
base.OnCreate(bundle);
SetContentView(Resource.Layout.Main);
}
}
}
保存应用程序。生成然后运行它,以在 Android 模拟器中显示所创建的应用程序。
Save the application. Build and then run it to display the created app in an Android Emulator.
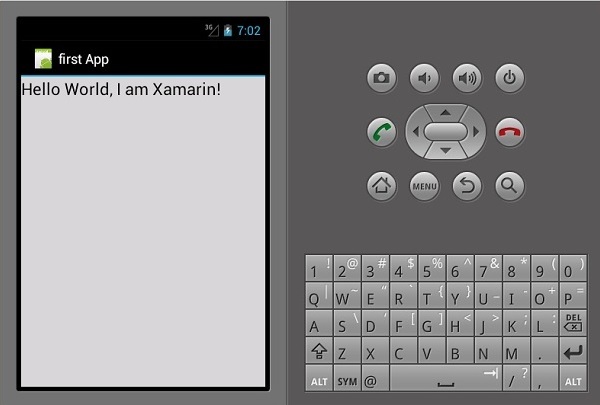
如果您没有 Android 模拟器,请按照下一部分中的步骤创建一个。
If you do not have an Android Emulator, then follow the steps given in the next section to create one.
Setting Up an Android Emulator
在 Visual Studio 菜单上,转到 Tools → Android → Android Emulator Manager 。在弹出的窗口中,单击 Create 按钮。它将显示以下屏幕。
On your Visual Studio menu, go to Tools → Android → Android Emulator Manager. On the pop-up window that appears, click the Create button. It will display the following screen.
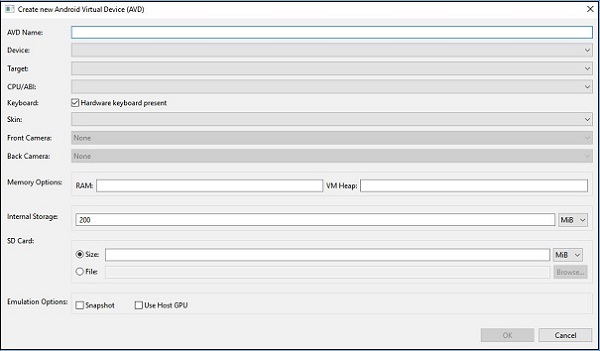
在上面的屏幕上,提供您想要的 AVD name 。为您的显示选择一个 device ,例如,Nexus 4” 显示。选择您的 target platform 。最好在最低目标平台上进行测试,例如,API 10 Android 2.3(Gingerbread)以确保您的应用程序可在所有 Android 平台上运行。
On the above screen, supply the AVD name you want. Select a device that is appropriate for your display, e.g., Nexus 4” display. Select your target platform. It is always advisable to test on a minimum target platform, e.g., API 10 Android 2.3 (Gingerbread) so as to ensure your App works across all Android platforms.
填写其余字段并单击确定按钮。您的模拟器现在已准备就绪。您可以从现有 Android 虚拟设备列表中选择它,然后单击 Start 以启动它。
Fill in the rest of the fields and click the OK button. Your emulator is now ready. You can select it from the list of existing Android Virtual Devices and then click Start to launch it.

Modifying HelloXamarin App
在此部分中,我们将修改我们的项目并创建一个在点击时显示文本的按钮。打开 main.axml 并切换到 source view 。在我们创建的 textview 后,我们将添加一个按钮,如下所示。
In this section, we will modify our project and create a button which will display text upon click. Open main.axml and switch to source view. After our textview that we created, we will add a button as shown below.
<Button
android:id = "@+id/MyButton"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:text = "@string/ButtonClick" />
添加按钮后,我们的完整代码将如下所示 −
After adding a button, our full code will look like this −
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<TextView
android:text = "@string/HelloXamarin"
android:textAppearance = "?android:attr/textAppearanceLarge"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/textView2" />
<Button
android:id = "@+id/MyButton"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:text = "@string/ButtonClick" />
</LinearLayout>
接下来,我们在 strings.xml 文件中注册我们的按钮值。
Next, we register our button values in the strings.xml file.
<string name = "ButtonClick">Click Me!</string>
在 strings.xml 文件中添加按钮后,我们打开 MainActivity.cs 文件,为按钮在点击时添加一个操作,如下面的代码所示。
After adding our button in the strings.xml file, we will open MainActivity.cs file to add an action for our button when it is clicked, as shown in the following code.
using System;
using Android.App;
using Android.Content;
using Android.Runtime;
using Android.Views;
using Android.Widget;
using Android.OS;
namespace HelloXamarin {
[Activity(Label = "HelloXamarin", MainLauncher = true, Icon = "@drawable/icon")]
public class MainActivity : Activity {
protected override void OnCreate(Bundle bundle) {
base.OnCreate(bundle);
SetContentView(Resource.Layout.Main);
Button button = FindViewById<Button>(Resource.Id.MyButton);
button.Click += delegate { button.Text = "Hello world I am your first App"; };
}
}
}
接下来,生成并运行您的应用程序。
Next, build and run your application.
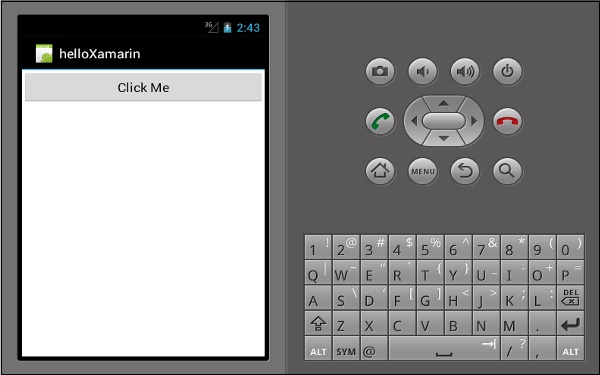
点击按钮后,将获得以下输出 −
After clicking on the button, you will get the following output −
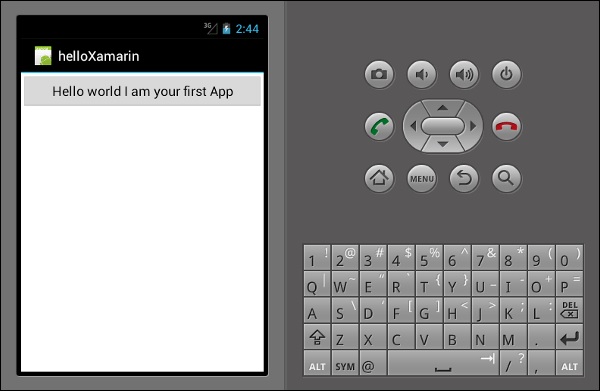