Xamarin 简明教程
Xamarin - Installation
Xamarin 构建在 .NET Framework 之上。它允许人们创建可以跨多个平台轻松运行的应用。在此教程中,我们将说明您如何可以使用 Xamarin 来交付本机 iOS、Android 和 Windows 应用程序。
Xamarin is built on the .NET Framework. It allows one to create apps that easily run across multiple platforms. In this tutorial, we will explain how you can use Xamarin to deliver native iOS, Android, and Windows Apps.
让我们通过讨论如何在 Windows 和 Mac 系统上安装 Xamarin 来开始本教程。
Let’s start the tutorial with a discussion on how to install Xamarin in Windows and Mac systems.
System Requirements
Installation on Windows
从 https://www.xamarin.com/download 下载 Xamarin 安装程序在运行 Xamarin 安装程序之前,确保已在你的电脑上安装了 Android SDK 和 Java SDK。
Download the Xamarin Installer from https://www.xamarin.com/download Before running the Xamarin installer, make sure you have installed Android SDK and Java SDK on your computer.
运行下载的安装程序以开始安装流程 -
Run the downloaded installer to begin the installation process −
-
The Xamarin license agreement screen appears. Click the Next button to accept the agreement.
-
The installer will search for any missing components and prompt you to download and install them.
-
After the Xamarin installation is complete, click the Close button to exit and get ready to start using Xamarin.
Xamarin - First Application
在本章中,我们将了解如何使用 Xamarin 创建一个小型 Android 应用程序。
In this chapter, we will see how to create a small Android application using Xamarin.
Hello Xamarin! Application
首先,启动 Visual Studio 的一个新实例,然后转到 File → New → Project 。
First of all, start a new instance of Visual Studio and go to File → New → Project.
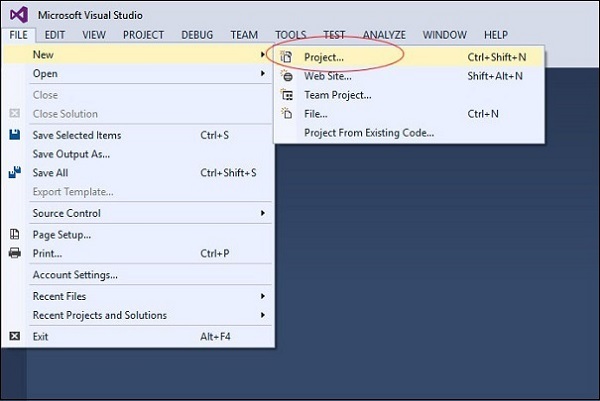
在出现的“菜单”对话框中,转到 Templates → Visual C# → Android → Blank App (Android) 。
On the Menu dialog box that appears, go to Templates → Visual C# → Android → Blank App (Android).
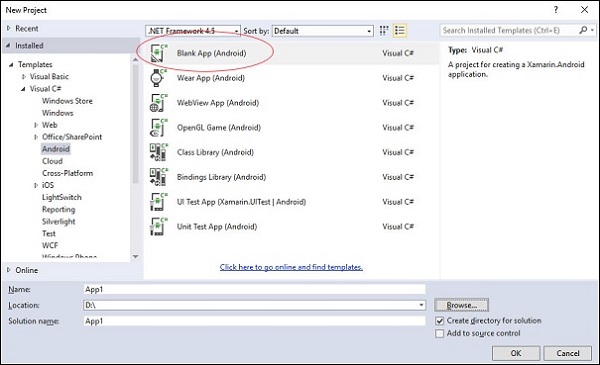
为你的应用程序起一个合适的名称。在我们的案例中,我们将其命名为 “helloWorld” ,并将其保存在提供的默认位置。接下来,单击“确定”按钮以加载新的 “helloXamarin” 项目。
Give an appropriate name for your application. In our case, we name it “helloWorld” and save it in the default location provided. Next, click the OK button for the new “helloXamarin” project to load.
在 solution 中,打开 Resources → layout → Main.axml 文件。从“设计视图”切换,并转到 Source 文件并键入以下代码行来构建你的应用程序。
On the solution, open Resources → layout → Main.axml file. Switch from Design View and go to the Source file and type the following lines of code to build your app.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:background = "#d3d3d3"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<TextView
android:text = "@string/HelloXamarin"
android:textAppearance = "?android:attr/textAppearanceLarge"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/textView2"
android:textColor = "@android:color/black" />
</LinearLayout>
在以上代码中,我们创建了一个新的 Android textview 。接下来,打开“values”文件夹,并双击 Strings.xml 以打开它。在此,我们将存储以上创建的 button 中的信息和值。
In the above code, we have created a new Android textview. Next, open the folder values and double-click Strings.xml to open it. Here, we are going to store information and values about the button created above.
<?xml version = "1.0" encoding = "utf-8"?>
<resources>
<string name = "HelloXamarin">Hello World, I am Xamarin!</string>
<string name = "ApplicationName">helloWorld</string>
</resources>
打开 MainActivity.cs 文件,并将现有代码替换为以下代码行。
Open MainActivity.cs file and replace the existing code with the following lines of code.
using System;
using Android.App;
using Android.Content;
using Android.Runtime;
using Android.Views;
using Android.Widget;
using Android.OS;
namespace HelloXamarin {
public class MainActivity : Activity {
protected override void OnCreate(Bundle bundle) {
base.OnCreate(bundle);
SetContentView(Resource.Layout.Main);
}
}
}
保存应用程序。生成然后运行它,以在 Android 模拟器中显示所创建的应用程序。
Save the application. Build and then run it to display the created app in an Android Emulator.
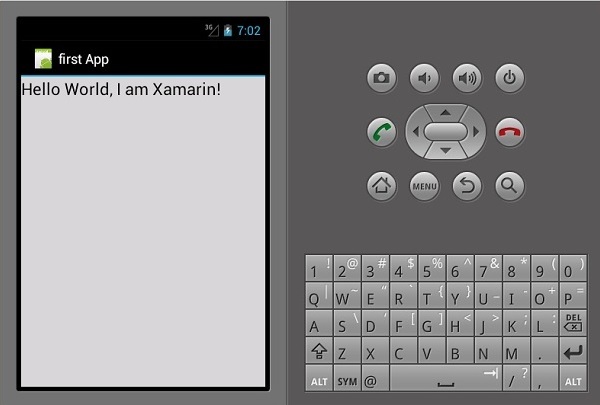
如果您没有 Android 模拟器,请按照下一部分中的步骤创建一个。
If you do not have an Android Emulator, then follow the steps given in the next section to create one.
Setting Up an Android Emulator
在 Visual Studio 菜单上,转到 Tools → Android → Android Emulator Manager 。在弹出的窗口中,单击 Create 按钮。它将显示以下屏幕。
On your Visual Studio menu, go to Tools → Android → Android Emulator Manager. On the pop-up window that appears, click the Create button. It will display the following screen.
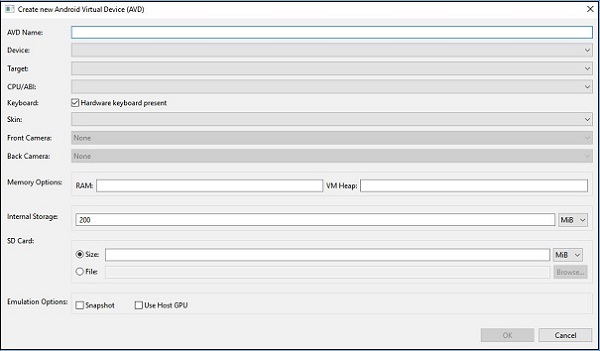
在上面的屏幕上,提供您想要的 AVD name 。为您的显示选择一个 device ,例如,Nexus 4” 显示。选择您的 target platform 。最好在最低目标平台上进行测试,例如,API 10 Android 2.3(Gingerbread)以确保您的应用程序可在所有 Android 平台上运行。
On the above screen, supply the AVD name you want. Select a device that is appropriate for your display, e.g., Nexus 4” display. Select your target platform. It is always advisable to test on a minimum target platform, e.g., API 10 Android 2.3 (Gingerbread) so as to ensure your App works across all Android platforms.
填写其余字段并单击确定按钮。您的模拟器现在已准备就绪。您可以从现有 Android 虚拟设备列表中选择它,然后单击 Start 以启动它。
Fill in the rest of the fields and click the OK button. Your emulator is now ready. You can select it from the list of existing Android Virtual Devices and then click Start to launch it.

Modifying HelloXamarin App
在此部分中,我们将修改我们的项目并创建一个在点击时显示文本的按钮。打开 main.axml 并切换到 source view 。在我们创建的 textview 后,我们将添加一个按钮,如下所示。
In this section, we will modify our project and create a button which will display text upon click. Open main.axml and switch to source view. After our textview that we created, we will add a button as shown below.
<Button
android:id = "@+id/MyButton"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:text = "@string/ButtonClick" />
添加按钮后,我们的完整代码将如下所示 −
After adding a button, our full code will look like this −
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<TextView
android:text = "@string/HelloXamarin"
android:textAppearance = "?android:attr/textAppearanceLarge"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/textView2" />
<Button
android:id = "@+id/MyButton"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:text = "@string/ButtonClick" />
</LinearLayout>
接下来,我们在 strings.xml 文件中注册我们的按钮值。
Next, we register our button values in the strings.xml file.
<string name = "ButtonClick">Click Me!</string>
在 strings.xml 文件中添加按钮后,我们打开 MainActivity.cs 文件,为按钮在点击时添加一个操作,如下面的代码所示。
After adding our button in the strings.xml file, we will open MainActivity.cs file to add an action for our button when it is clicked, as shown in the following code.
using System;
using Android.App;
using Android.Content;
using Android.Runtime;
using Android.Views;
using Android.Widget;
using Android.OS;
namespace HelloXamarin {
[Activity(Label = "HelloXamarin", MainLauncher = true, Icon = "@drawable/icon")]
public class MainActivity : Activity {
protected override void OnCreate(Bundle bundle) {
base.OnCreate(bundle);
SetContentView(Resource.Layout.Main);
Button button = FindViewById<Button>(Resource.Id.MyButton);
button.Click += delegate { button.Text = "Hello world I am your first App"; };
}
}
}
接下来,生成并运行您的应用程序。
Next, build and run your application.
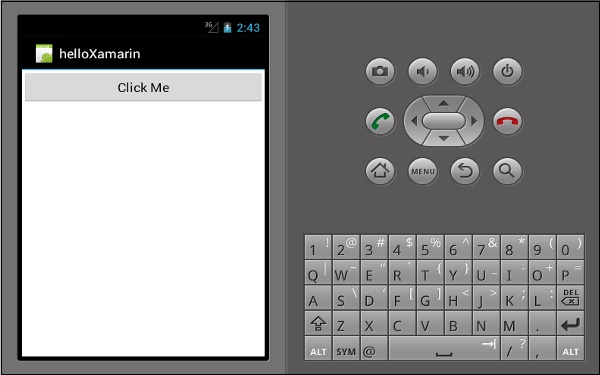
点击按钮后,将获得以下输出 −
After clicking on the button, you will get the following output −
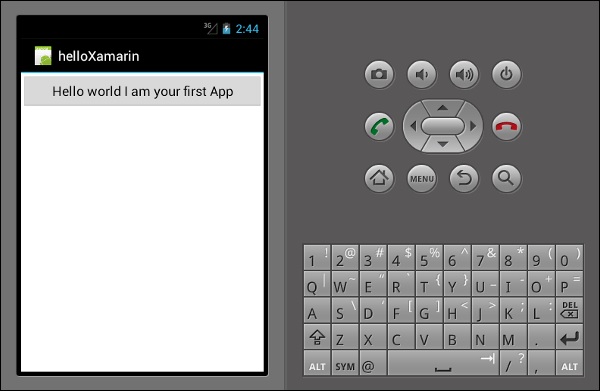
Xamarin - Application Manifest
所有 Android 应用都有一个 manifest file ,通常称为 AndroidManifest.xml 。清单文件包含 Android 平台为成功运行应用所需的一切内容。
All Android Apps have a manifest file commonly referred to as AndroidManifest.xml. The manifest file contains everything about the Android platform that an App needs in order to run successfully.
在此,我们列出了清单文件的部分重要功能 -
Here, we have listed down some of the important functions of a manifest file −
-
It declares the minimum API level required by the application.
-
It declares the permissions required by the application, e.g., camera, location, etc.
-
It gives permissions to hardware and software features used or required by the application.
-
It lists the libraries that the application must be linked.
以下截图显示了一个清单文件。
The following screenshot shows a Manifest file.
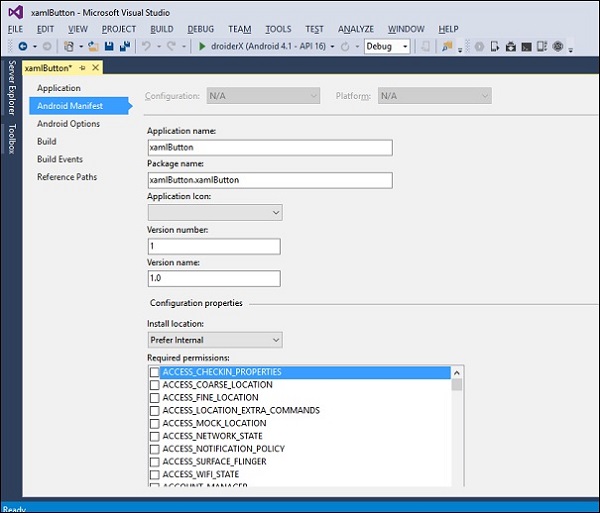
Application name - 它指您应用的标题
Application name − It refers to the title of your App
Package name - 它是用于识别您的应用的唯一名称。
Package name − It is an unique name used to identify your App.
Application Icon - 它是针对您的应用显示在 Android 主屏幕上的图标。
Application Icon − It is the icon displayed on the Android home screen for your App.
Version Number - 它是一个用于显示您应用的一个版本比另一个版本更新的单一数字。
Version Number − It is a single number that is used to show one version of your App is more recent than another.
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
android:versionCode="1" >
Version Name - 它针对您的应用的用户友好型版本字符串,用户将通过您的应用设置和 Google Play 商店查看该字符串。以下代码显示了一个版本名称示例。
Version Name − It is a user-friendly version string for your App that users will see on your App settings and on the Google PlayStore. The following code shows an example of a version name.
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
android:versionName="1.0.0">
Minimum Android Version - 它是您的应用程序所支持的最低 Android 版本平台。
Minimum Android Version − It is the lowest Android version platform which your application supports.
<uses-sdk android:minSdkVersion="16" />
在上例中,我们的最低 Android 版本是 API 级别 16,通常称为 JELLY BEAN 。
In the above example, our minimum Android version is API Level 16, commonly referred to as JELLY BEAN.
Target Android Version - 它针对您的应用进行编译的 Android 版本。
Target Android Version − It is the Android version on which your App is compiled against.
Xamarin - Android Resources
创建新的 Android 项目时,会默认将一些文件添加到项目中。我们将这些默认项目文件和文件夹称为 Android Resources 。查看以下屏幕截图。
When a new Android project is created, there are some files that are added to the project, by default. We call these default project files and folders as Android Resources. Take a look at the following screenshot.
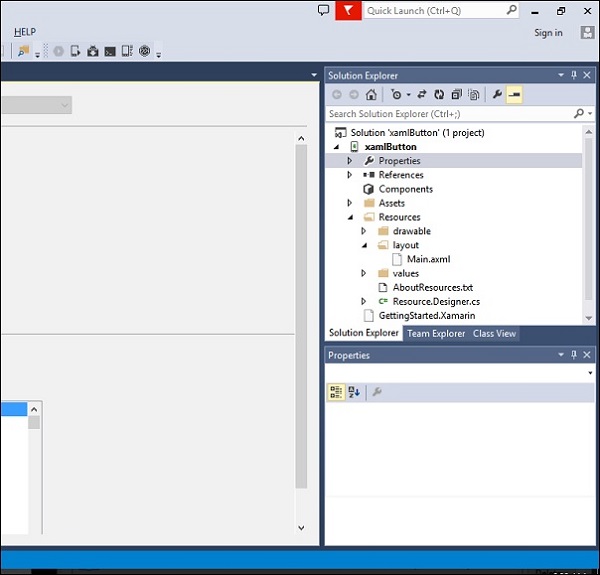
默认 Android 资源包括以下内容 −
The default Android resources include the following −
-
AndroidManifest.xml file − It contains information about your Android applications, e.g., the application name, permissions, etc.
-
Resources folder − Resources can be images, layouts, strings, etc. that can be loaded via Android’s resource system.
-
Resources/drawable folder − It stores all the images that you are going to use in your application.
-
Resources/layout folder − It contains all the Android XML files (.axml) that Android uses to build user interfaces.
-
The Resources/values folder − It contains XML files to declare key-value pairs for strings (and other types) throughout an application. This is how localization for multiple languages is normally set up on Android.
-
Resources.designer.cs − This file is created automatically when the Android projected is created and it contains unique identifiers that reference the Android resources.
-
MainActivity.cs file − This is the first activity of your Android application and from where the main application actions are launched from.
可以使用 unique ID 来以编程方式访问资源文件,该文件存储在 resources.designer.cs 文件中。该 ID 包含在一个名为 Resource 的类中。添加到项目中的任何资源都会自动生成在 resource class 中。
Resource files can be accessed programmatically through a unique ID which is stored in the resources.designer.cs file. The ID is contained under a class called Resource. Any resource added to the project is automatically generated inside the resource class.
下面的代码展示了如何创建一个包含七张图片的网格视图项目:
The following code shows how to create a gridview project containing seven images −
namespace HelloGridView {
[System.CodeDom.Compiler.GeneratedCodeAttribute
("Xamarin.Android.Build.Tas ks",
"1.0.0.0")]
public partial class Resource {
static Resource() {
global::Android.Runtime.ResourceIdManager.UpdateIdValues();
}
public static void UpdateIdValues() {}
public partial class Attribute {
static Attribute() {
global::Android.Runtime.ResourceIdManager.UpdateIdValues();
}
private Attribute() {}
}
public partial class Drawable {
// aapt resource value: 0x7f020000
public const int Icon = 2130837504;
// aapt resource value: 0x7f020001
public const int img1 = 2130837505;
// aapt resource value: 0x7f020002
public const int img2 = 2130837506;
// aapt resource value: 0x7f020003
public const int img3 = 2130837507;
// aapt resource value: 0x7f020004
public const int img4 = 2130837508;
// aapt resource value: 0x7f020005
public const int img5 = 2130837509;
// aapt resource value: 0x7f020006
public const int img6 = 2130837510;
// aapt resource value: 0x7f020007
public const int img7 = 2130837511;
static Drawable() {
global::Android.Runtime.ResourceIdManager.UpdateIdValues();
}
private Drawable() {}
}
public partial class Id {
// aapt resource value: 0x7f050000
public const int gridview = 2131034112;
static Id() {
global::Android.Runtime.ResourceIdManager.UpdateIdValues();
}
private Id() {}
}
public partial class Layout {
// aapt resource value: 0x7f030000
public const int Main = 2130903040;
static Layout() {
global::Android.Runtime.ResourceIdManager.UpdateIdValues();
}
private Layout() {}
}
public partial class String {
// aapt resource value: 0x7f040001
public const int ApplicationName = 2130968577;
// aapt resource value: 0x7f040000
public const int Hello = 2130968576;
static String() {
global::Android.Runtime.ResourceIdManager.UpdateIdValues();
}
private String() {}
}
}
}
从上面的代码中,七张照片被引用在一个名为 drawable 的类中。这些图片是通过编程方式添加的。如果用户向项目中添加另一张图片,它也将被添加到 drawable 类。项目中包含的 gridview 也被添加并存储在它自己的一个类中。 resources folder 中包含的每个项目都会自动生成并存储在一个类中。
From the above code, the seven images are referenced in a class called drawable. These images are added programmatically. If a user adds another image to the project, it will also be added to the drawable class. The gridview contained in the project is also added and stored in a class on its own. Each item contained in the resources folder is automatically generated and stored in a class.
Xamarin - Android Activity Lifecycle
当用户浏览一个 Android 应用时,会发生一系列事件。例如,当用户启动一个应用,例如 Facebook 应用,它会启动并且对用户在前台可见, onCreate() → onStart() → onResume() 。
When a user navigates through an Android App, a series of events occurs. For example, when a user launches an app, e.g., the Facebook App, it starts and becomes visible on the foreground to the user, onCreate() → onStart() → onResume().
如果另一个活动启动,例如电话呼入,那么 Facebook 应用将进入后台,通话进入前台。我们现在有两个正在运行的进程。
If another activity starts, e.g., a phone call comes in, then the Facebook app will go to the background and the call comes to the foreground. We now have two processes running.
onPause() --- > onStop()
当通话结束时,Facebook 应用返回前台。会调用三个方法。
When the phone call ends, the Facebook app returns to the foreground. Three methods are called.
onRestart() --- > onStart() --- > onResume()
Android 活动中有 7 个生命周期进程。它们包括如下:
There are 7 lifecycle processes in an Android activity. They include −
-
onCreate − It is called when the activity is first created.
-
onStart − It is called when the activity starts and becomes visible to the user.
-
onResume − It is called when the activity starts interacting with the user. User input takes place at this stage.
-
onPause − It is called when the activity runs in the background but has not yet been killed.
-
onStop − It is called when the activity is no longer visible to the user.
-
onRestart − It is called after the activity has stopped, before starting again. It is normally called when a user goes back to a previous activity that had been stopped.
-
onDestroy − This is the final call before the activity is removed from the memory.
下面的插图展示了 Android 活动生命周期:
The following illustration shows the Android Activity Lifecycle −
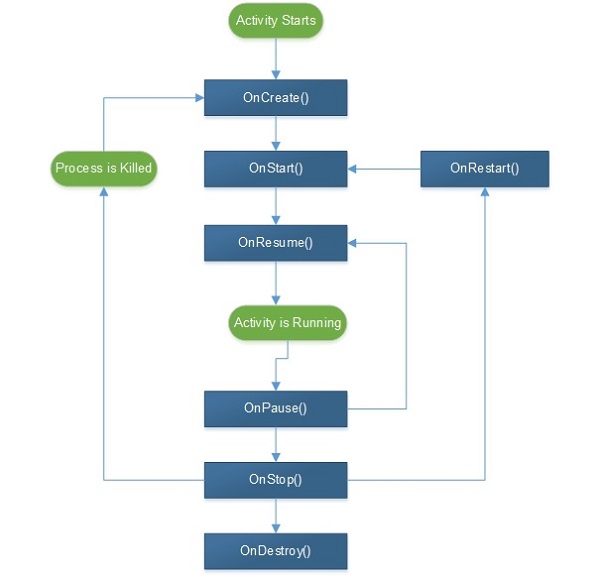
Xamarin - Permissions
在 Android 中,默认情况下,没有任何应用程序有权限对可能对用户或操作系统产生影响的任何操作执行操作。为了让 App 执行一项任务,它必须声明权限。在 Android 系统授予权限之前,App 无法执行此任务。此权限机制阻止应用程序在未经用户同意的情况下执行其希望的操作。
In Android, by default, no application has permissions to perform any operations that would have an effect on the user or the operating system. In order for an App to perform a task, it must declare the permissions. The App cannot perform the task until the permission are granted by the Android system. This mechanism of permissions stops applications from doing as they wish without the user’s consent.
权限将记录在 AndroidManifest.xml 文件中。要添加权限,请双击属性,然后转至 Android Man*所需的权限*将出现。选中你要添加的适当权限。
Permissions are to be recorded in AndroidManifest.xml file. To add permissions, we double-click on properties, then go to Android Man*Required permissions* will appear. Check the appropriate permissions you wish to add.

Camera − 它提供访问设备摄像头的权限。
Camera − It provides permission to access the device’s camera.
<uses-permission android:name="android.permission.CAMERA" />
Internet − 它提供对网络资源的访问。
Internet − It provides access to network resources.
<uses-permission android:name="android.permission.INTERNET" />
ReadContacts − 它提供访问设备上联系人的权限。
ReadContacts − It provides access to read the contacts on your device.
<uses-permission android:name="android.permission.READ_CONTACTS" />
ReadExternalStorage − 它提供对外部存储器读写数据的权限。
ReadExternalStorage − It provides access to read and store data on an external storage.
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
Calendars − 它允许应用程序访问用户设备上的日历和事件。此权限可能很危险,因为它赋予应用程序在所有者不知情的情况下向来宾发送电子邮件的能力。添加此权限的语法如下所示 −
Calendars − It allows an app access to the calendar on the user device and events. This permission can be dangerous, as it grants an app the ability to send emails to guests without the owner’s awareness. The syntax for adding this permission is as shown below −
<uses-permission android:name="android.permission-group.CALENADAR" />
SMS − 具有此权限的应用程序有能力使用设备的消息服务。它包括读取、写入和编辑短信和彩信。其语法如下所示。
SMS − An app with this permission has the ability to use the devices messaging services. It includes reading, writing, and editing SMS and MMS messages. Its syntax is as shown below.
<uses-permission android:name="android.permission-group.SMS" />
Location − 具有此权限的应用程序可以使用 GPS 网络访问设备的位置。
Location − An app with this permission can access the device’s location using the GPS network.
<uses-permission android:name="android.permission-group.LOCATION" />
Bluetooth − 具有此权限的应用程序可以使用蓝牙功能的设备无线地交换数据文件。
Bluetooth − An app with this permission can exchange data files with other Bluetooth enabled devices wirelessly.
<uses-permission android:name="android.permission.BLUETOOTH" />
Xamarin - Building the App GUI
TextView
TextView 是 Android 微件的一个非常重要的组件。它主要用于在 Android 屏幕上显示文本。
TextView is a very important component of the Android widgets. It is primarily used for displaying texts on an Android screen.
为了创建一个 textView,只需打开 main.axml ,并在 linear layout 标签之间添加以下代码。
To create a textview, simply open main.axml and add the following code between the linear layout tags.
<TextView
android:text = "Hello I am a text View"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/textview1" />
Button
按钮是一个控件,在点击时用于触发一个事件。在 Main.axml 文件下,键入以下代码以创建按钮。
A button is a control used to trigger an event when it is clicked. Under your Main.axml file, type the following code to create a button.
<Button
android:id = "@+id/MyButton"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:text = "@string/Hello" />
打开 Resources\Values\Strings.xml ,并在 <resources> 标签之间键入以下代码。
Open Resources\Values\Strings.xml and type the following line of code in between <resources> tag.
<string name="Hello">Click Me!</string>
上述代码提供了我们创建的按钮的值。接下来,我们打开 MainActivity.cs 并创建在按钮点击时要执行的动作。在 base.OnCreate (bundle)方法下键入以下代码。
The above code provides the value of the button we created. Next, we open MainActivity.cs and create the action to be performed when the button is clicked. Type the following code under base.OnCreate (bundle) method.
Button button = FindViewById<Button>(Resource.Id.MyButton);
button.Click += delegate { button.Text = "You clicked me"; };

当用户单击该按钮时,上述代码会显示“You Clicked Me”。
The above code displays “You Clicked Me” when a user clicks on the button.
FindViewById<< -→ 该方法找到已识别的视图的 ID。它在 .axml 布局文件中搜索 id。
FindViewById<< -→ This method finds the ID of a view that was identified. It searches for the id in the .axml layout file.
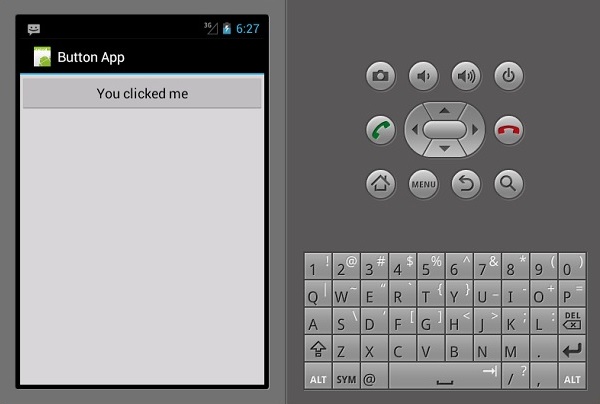
Checkbox
当希望从一组选项中选择多个选项时,使用复选框。在此示例中,我们将创建一个复选框,选中后,显示已选中,否则显示未选中。
A checkbox is used when one wants to select more than one option from a group of options. In this example, we are going to create a checkbox which on selected, displays a message that it has been checked, else it displays unchecked.
首先,我们在项目中打开 Main.axml 文件,并键入以下代码行以创建一个复选框。
To start with, we open Main.axml file in our project and type the following line of code to create a checkbox.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:background = "#d3d3d3"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<CheckBox
android:text = "CheckBox"
android:padding = "25dp"
android:layout_width = "300dp"
android:layout_height = "wrap_content"
android:id = "@+id/checkBox1"
android:textColor = "@android:color/black"
android:background = "@android:color/holo_blue_dark" />
</LinearLayout>
下一步,转到 MainActivity.cs 来添加功能代码。
Next, go to MainActivity.cs to add the functionality code.
CheckBox checkMe = FindViewById<CheckBox>(Resource.Id.checkBox1);
checkMe.CheckedChange += (object sender, CompoundButton.CheckedChangeEventArgs e) => {
CheckBox check = (CheckBox)sender;
if(check.Checked) {
check.Text = "Checkbox has been checked";
} else {
check.Text = "Checkbox has not been checked";
}
};
从上述代码中,我们首先使用 findViewById 找到复选框。接下来,我们为复选框创建一个处理程序方法,并在处理程序中,我们创建一个 if else 语句,根据选择的结果显示消息。
From the above code, we first find the checkbox using findViewById. Next, we create a handler method for our checkbox and in our handler, we create an if else statement which displays a message depending on the outcome selected.
CompoundButton.CheckedChangeEventArgs → 复选框的状态更改时,此方法将触发事件。
CompoundButton.CheckedChangeEventArgs → This method fires an event when the checkbox state changes.

Progress Bar
进度条是一个用于显示操作进度的控件。要添加进度条,请在 Main.axml 文件中添加以下代码行。
A progress bar is a control that is used to show the progression of an operation. To add a progress bar, add the following line of code in Main.axml file.
<ProgressBar
style="?android:attr/progressBarStyleHorizontal"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/progressBar1" />
下一步,转到 MainActivity.cs 并设置进度条的值。
Next, go to MainActivity.cs and set the value of the progress bar.
ProgressBar pb = FindViewById<ProgressBar>(Resource.Id.progressBar1);
pb.Progress = 35;
在上述代码中,我们创建了一个值为 35 的进度条。
In the above code, we have created a progress bar with a value of 35.
Radio Buttons
这是一个 Android 小部件,允许人从一组选项中选择一个。在本部分中,我们将创建一个包含汽车列表的单选组,该组将获取选中的单选按钮。
This is an Android widget which allows a person to choose one from a set of options. In this section, we are going to create a radio group containing a list of cars which will retrieve a checked radio button.
首先,我们添加一个单选组和 textview ,如下面的代码所示 −
First, we add a radio group and a textview as shown in the following code −
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:background = "@android:color/darker_gray"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<TextView
android:text = "What is your favourite Car"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/textView1"
android:textColor = "@android:color/black" />
<RadioGroup
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/radioGroup1"
android:backgroundTint = "#a52a2aff"
android:background = "@android:color/holo_green_dark">
<RadioButton
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:text = "Ferrari"
android:id = "@+id/radioFerrari" />
<RadioButton
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:text = "Mercedes"
android:id = "@+id/radioMercedes" />
<RadioButton
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:text = "Lamborghini"
android:id = "@+id/radioLamborghini" />
<RadioButton
android:text = "Audi"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/radioAudi" />
</RadioGroup>
</LinearLayout>
当单击单选按钮时,执行一个动作,我们添加一个活动。转到 MainActivity.cs 并创建一个新事件处理程序,如下所示。
To perform an action, when a radio button is clicked, we add an activity. Go to MainActivity.cs and create a new event handler as shown below.
private void onClickRadioButton(object sender, EventArgs e) {
RadioButton cars = (RadioButton)sender;
Toast.MakeText(this, cars.Text, ToastLength.Short).Show
();
}
Toast.MakeText() → 这是一个视图方法,用于在小弹出窗口中显示消息/输出。在 OnCreate() 方法底部的 SetContentView() 之后,添加以下代码片段。这将捕获每个单选按钮并将其添加到我们创建的事件处理程序中。
Toast.MakeText() → This is a view method used to display a message/output in a small pop up. At the bottom of the OnCreate() method just after SetContentView(), add the following piece of code. This will capture each of the radio buttons and add them to the event handler we created.
RadioButton radio_Ferrari = FindViewById<RadioButton>
(Resource.Id.radioFerrari);
RadioButton radio_Mercedes = FindViewById<RadioButton>
(Resource.Id.radioMercedes);
RadioButton radio_Lambo = FindViewById<RadioButton>
(Resource.Id.radioLamborghini);
RadioButton radio_Audi = FindViewById<RadioButton>
(Resource.Id.radioAudi);
radio_Ferrari.Click += onClickRadioButton;
radio_Mercedes.Click += onClickRadioButton;
radio_Lambo.Click += onClickRadioButton;
radio_Audi.Click += onClickRadioButton;
现在,运行你的应用程序。它应该显示以下屏幕作为输出 −
Now, run your application. It should display the following screen as the output −

Toggle Buttons
切换按钮用于在两种状态之间切换,例如,它可以在开(ON)和关(OFF)之间切换。打开 Resources\layout\Main.axml 并添加以下代码行以创建一个切换按钮。
Toggle button are used to alternate between two states, e.g., it can toggle between ON and OFF. Open Resources\layout\Main.axml and add the following lines of code to create a toggle button.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:background = "#d3d3d3"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<ToggleButton
android:id = "@+id/togglebutton"
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:textOn = "Torch ON"
android:textOff = "Torch OFF"
android:textColor = "@android:color/black" />
</LinearLayout>
单击切换栏时,我们可以向其中添加操作。打开 MainActivity.cs ,并在 OnCreate() 方法类之后添加以下代码行。
We can add actions to the toggle bar when it is clicked. Open MainActivity.cs and add the following lines of code after the OnCreate() method class.
ToggleButton togglebutton = FindViewById<ToggleButton> (Resource.Id.togglebutton);
togglebutton.Click += (o, e) => {
if (togglebutton.Checked)
Toast.MakeText(this, "Torch is ON", ToastLength.Short).Show ();
else
Toast.MakeText(this, "Torch is OFF",
ToastLength.Short).Show();
};
现在,当您运行 App 时,它应显示以下输出 −
Now, when you run the App, it should display the following output −

Ratings Bar
等级栏是由星构成的窗体元素,应用程序用户可以使用这些星来评估您为他们提供的内容。在您的 Main.axml 文件中,使用 5 颗星创建一个新的等级栏。
A Ratings Bar is a form element that is made up of stars which app users can use to rate things you have provided for them. In your Main.axml file, create a new rating bar with 5 stars.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:background = "#d3d3d3"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<RatingBar
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:id = "@+id/ratingBar1"
android:numStars = "5"
android:stepSize = "1.0" />
</LinearLayout>
运行应用程序后,它应显示以下输出 −
On running the app, it should display the following output −

Autocomplete Textview
这是一个文本视图,在用户输入时显示全部建议。我们要创建一个自动填充文本视图,其中包含一个人员姓名列表,以及一个单击后将显示所选名称的按钮。
This is a textview that shows full suggestions while a user is typing. We are going to create an autocomplete textview containing a list of people’s names and a button which on click will show us the selected name.
打开 Main.axml 并编写以下代码。
Open Main.axml and write the following code.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:layout_width = "fill_parent"
android:background = "#d3d3d3"
android:layout_height = "fill_parent">
<TextView
android:text = "Enter Name"
android:textAppearance = "?android:attr/textAppearanceMedium"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:id = "@+id/textView1"
android:padding = "5dp"
android:textColor = "@android:color/black" />
<AutoCompleteTextView
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:id = "@+id/autoComplete1"
android:textColor = "@android:color/black" />
<Button
android:text = "Submit"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:id = "@+id/btn_Submit"
android:background="@android:color/holo_green_dark" />
</LinearLayout>
以上的代码将生成一个用于输入的 TextView、用于显示建议的 AutoCompleteTextView 和一个用于显示从 TextView 输入的名称的按钮。转到 MainActivity.cs 以添加该功能。
The above code generates a TextView for typing, AutoCompleteTextView for showing suggestions, and a button to display the names entered from the TextView. Go to MainActivity.cs to add the functionality.
创建如下所示的新事件处理程序方法。
Create a new event handler method as shown below.
protected void ClickedBtnSubmit(object sender, System.EventArgs e){
if (autoComplete1.Text != ""){
Toast.MakeText(this, "The Name Entered ="
+ autoComplete1.Text, ToastLength.Short).Show();
} else {
Toast.MakeText(this, "Enter a Name!", ToastLength.Short).Show();
}
}
创建的处理程序将检查自动填充文本视图是否为空。如果它不为空,则显示所选的自动填充文本。在 OnCreate() 类中键入以下代码。
The created handler checks whether the autocomplete textview is empty. If it is not empty, then it displays the selected autocomplete text. Type the following code inside the OnCreate() class.
autoComplete1 = FindViewById<AutoCompleteTextView>(Resource.Id.autoComplete1);
btn_Submit = FindViewById<Button>(Resource.Id.btn_Submit);
var names = new string[] { "John", "Peter", "Jane", "Britney" };
ArrayAdapter adapter = new ArrayAdapter<string>(this,
Android.Resource.Layout.SimpleSpinnerItem, names);
autoComplete1.Adapter = adapter;
btn_Submit.Click += ClickedBtnSubmit;
ArrayAdapter − 这是一个收集处理程序,它从列表收集中读取数据项并将其作为视图返回或显示在屏幕上。
ArrayAdapter − This is a collection handler that reads data items from a list collection and returns them as a view or displays them on the screen.
现在,当您运行应用程序时,它应显示以下输出。
Now, when you run the application, it should display the following output.
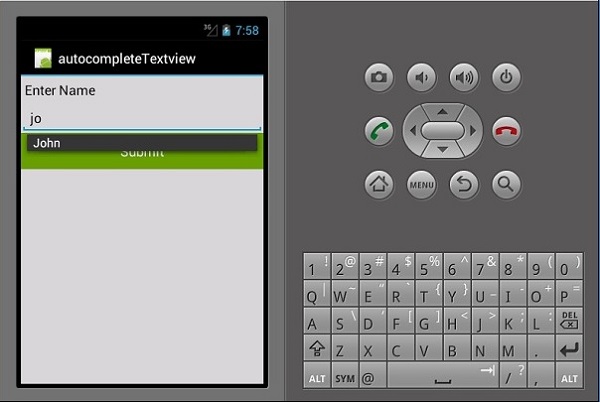
Xamarin - Menus
Popup Menus
弹出菜单是指附属于视图的菜单;它也称为 shortcut menu 。让我们来看看如何向 Android 应用程序添加弹出菜单。
A popup menu refers to a menu that is attached to a view; it is also referred to as a shortcut menu. Let’s see how to add a popup menu to an Android App.
创建一个新项目并将其命名为 popUpMenu App 。打开 Main.axml 并创建一个按钮,该按钮将用于显示弹出菜单。
Create a new project and call it popUpMenu App. Open Main.axml and create a button which will be used to display the popup menu.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:background = "#d3d3d3"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<Button
android:id = "@+id/popupButton"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:text = "Show popup menu"
android:background = "@android:color/holo_green_dark"
android:textColor = "@android:color/black" />
</LinearLayout>
在 Resources 文件夹下创建一个新文件夹,并将其命名为 Menu 。在 Menu 文件夹内,添加一个名为 popMenu.xml 的新 xml 文件。
Create a new folder under the Resources folder and call it Menu. Inside the Menu folder, add a new xml file called popMenu.xml.
在 popMenu.xml 下,添加以下菜单项。
Under popMenu.xml, add the following menu items.
<?xml version = "1.0" encoding="utf-8"?>
<menu xmlns:android = "http://schemas.android.com/apk/res/android">
<item
android:id = "@+id/file_settings"
android:icon = "@drawable/img_settings"
android:title = "Settings"
android:showAsAction = "ifRoom">
<item
android:id = "@+id/new_game1"
android:icon = "@drawable/imgNew"
android:title = "New File Settings"/>
<item
android:id = "@+id/help"
android:icon = "@drawable/img_help"
android:title = "Help" />
<item
android:id = "@+id/about_app"
android:icon = "@drawable/img_help"
android:title = "About app"/>
</item>
</menu>
添加菜单项后,转到 mainActivity.cs 以在单击按钮时显示弹出菜单。
After adding the menu items, go to mainActivity.cs to display the popup menu on button click.
protected override void OnCreate(Bundle bundle) {
base.OnCreate(bundle);
SetContentView(Resource.Layout.Main);
Button showPopupMenu = FindViewById<Button>(Resource.Id.popupButton);
showPopupMenu.Click += (s, arg) => {
PopupMenu menu = new PopupMenu(this, showPopupMenu);
menu.Inflate(Resource.Menu.popMenu);
menu.Show();
};
}
现在,构建并运行您的应用程序。它应产生以下输出 −
Now, build and run your application. It should produce the following output −
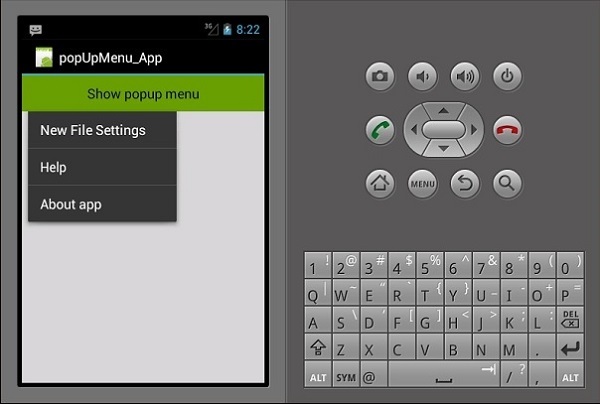
Options Menu
选项菜单是由 App 主要菜单组成的集合,主要用于存储设置、搜索等。在这里,我们将在设置菜单中创建三个项目,即 New File Settings, Help, and About App 。
Options Menu is a collection of menus that are primary to an App and are mainly used to store settings, search, etc. Here, we are going to create a menu for settings with three items inside, i.e., New File Settings, Help, and About App.
为创建一个选项菜单,我们必须在 resources 文件夹中创建一个新的 XML 布局文件。首先,我们将添加一个新的 XML 文件。鼠标右键单击 Layout folder ,然后转到 Add → New item → Visual C# → XML File 。
To create an options menu, we must create a new XML layout file in the resources folder. First of all, we will add a new XML file. Right-click on the Layout folder, then go to Add → New item → Visual C# → XML File.
为 layout file 选择一个合适的名字。在我们的示例中,我们将把我们的文件称为 myMenu.xml 。
Choose an appropriate name for the layout file. In our example, we will call our file myMenu.xml.
在 myMenu.xml 中,我们将创建一个新菜单并在其中添加项目。以下代码展示了如何执行此操作。
Inside myMenu.xml, we are going to create a new menu and add items inside. The following code shows how to do it.
<?xml version = "1.0" encoding = "utf-8"?>
<menu xmlns:android = "http://schemas.android.com/apk/res/android">
<item
android:id = "@+id/file_settings"
android:icon = "@drawable/img_settings"
android:title = "Settings"
android:showAsAction = "ifRoom">
<menu>
<item
android:id = "@+id/new_game1"
android:icon = "@drawable/imgNew"
android:title = "New File Settings" />
<item
android:id = "@+id/help"
android:icon = "@drawable/img_help"
android:title = "Help" />
<item
android:id = "@+id/about_app"
android:icon = "@drawable/img_help"
android:title = "About app"/>
</menu>
</item>
</menu>
接下来,我们将导航到 MainActivity.cs 并为 onOptionsMenu() 创建一个覆盖类。
Next, we navigate to MainActivity.cs and create an override class for onOptionsMenu().
public override bool OnCreateOptionsMenu(IMenu menu) {
MenuInflater.Inflate(Resource.Menu.myMenu, menu);
return base.OnPrepareOptionsMenu(menu);
}
接下来,我们创建一个操作以在选择 settings menu 时对其进行响应。为此,我们为 OnOptionsItemSelected() 菜单创建另一个覆盖类。
Next, we create an action to respond to the settings menu when it is selected. To do this, we create another override class for the OnOptionsItemSelected() menu.
public override bool OnOptionsItemSelected(IMenuItem item) {
if (item.ItemId == Resource.Id.file_settings) {
// do something here...
return true;
}
return base.OnOptionsItemSelected(item);
}
我们最终的完整代码如下所示:
Our final complete code will look as follows −
namespace optionsMenuApp {
[Activity(Label = "options Menu", MainLauncher = true, Icon = "@drawable/icon")]
public class MainActivity : Activity {
public override bool OnCreateOptionsMenu(IMenu menu) {
MenuInflater.Inflate(Resource.Menu.myMenu, menu);
return base.OnPrepareOptionsMenu(menu);
}
public override bool OnOptionsItemSelected(IMenuItem item) {
if (item.ItemId == Resource.Id.file_settings) {
// do something here...
return true;
}
return base.OnOptionsItemSelected(item);
}
}
}
现在,构建并运行您的应用程序。它应产生以下输出 −
Now, build and run your application. It should produce the following output −
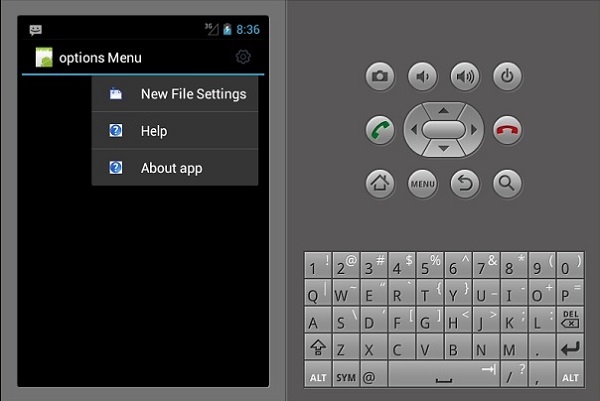
Xamarin - Layouts
Linear Layout
在线性布局中,内容水平或垂直排列。
In linear layout, the contents are arranged in either horizontal or vertical manner.
Linear Layout ─ Horizontal
此布局的内容水平排列。对于此演示,我们将创建 3 个按钮并将它们水平排列在线性布局中。
The contents of this layout are arranged horizontally. For this demo, we are going to create 3 buttons and arrange them horizontally in a linear layout.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "horizontal"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent"
android:background = "#d3d3d3"
android:minWidth="25px"
android:minHeight="25px">
<Button
android:id="@+id/MyButton1"
android:layout_width="wrap_content"
android:layout_margin="10dp"
android:layout_height="wrap_content"
android:text="Button 1"
android:background="@android:color/holo_green_dark" />
<Button
android:id="@+id/MyButton2"
android:layout_width="wrap_content"
android:layout_margin="10dp"
android:layout_height="wrap_content"
android:text="Button 2"
android:background="@android:color/holo_green_dark" />
<Button
android:id="@+id/MyButton3"
android:layout_width="wrap_content"
android:layout_margin="10dp"
android:layout_height="wrap_content"
android:text="Button 3"
android:background="@android:color/holo_green_dark" />
</LinearLayout>
结果输出如下所示 -
The resulting output is as shown below −
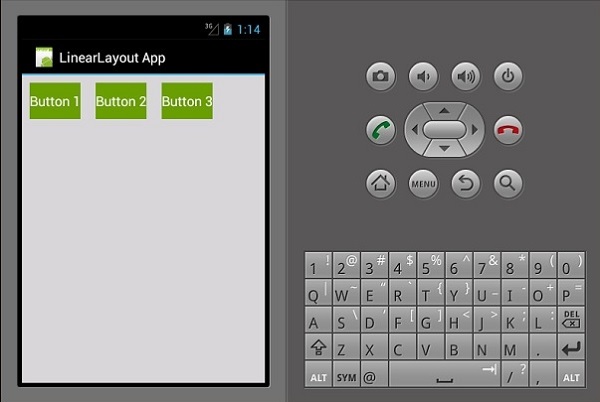
Linear Layout ─ Vertical
这种类型的布局将子视图垂直放置。
This type of layout places the child view in a vertical manner.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent"
android:background = "#d3d3d3"
android:minWidth = "25px"
android:minHeight = "25px">
<Button
android:id = "@+id/MyButton1"
android:layout_width = "fill_parent"
android:layout_margin = "10dp"
android:layout_height = "wrap_content"
android:text = "Button 1"
android:background = "@android:color/holo_green_dark" />
<Button
android:id = "@+id/MyButton2"
android:layout_width = "fill_parent"
android:layout_margin = "10dp"
android:layout_height = "wrap_content"
android:text = "Button 2"
android:background = "@android:color/holo_green_dark" />
<Button
android:id = "@+id/MyButton3"
android:layout_width = "fill_parent"
android:layout_margin = "10dp"
android:layout_height = "wrap_content"
android:text="Button 3"
android:background = "@android:color/holo_green_dark" />
</LinearLayout>
其结果输出如下 -
Its resulting output is as follows −
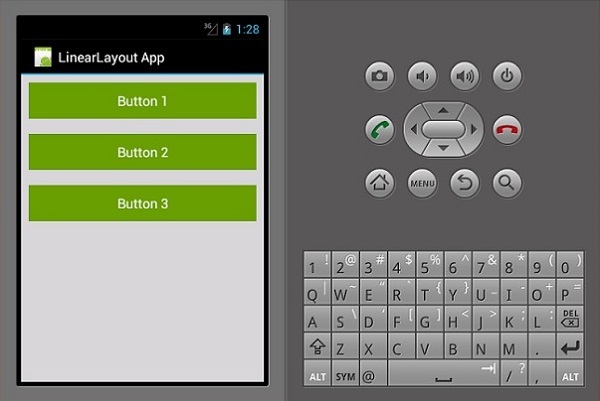
Relative Layout
在此视图中,子视图的位置相对于其父视图或其兄弟视图。在以下示例中,我们将创建 3 个 EditText 视图和一个按钮,然后相对排列它们。
In this view, the position of the child view is relative to its parent or to its sibling view. In the following example, we are going to create 3 EditText views and a button and then, align them relatively.
创建一个新项目并将其命名为 relative layout app 。打开 main.axml 并添加以下代码。
Create a new project and call it relative layout app. Open main.axml and add the following code.
<?xml version = "1.0" encoding = "utf-8"?>
<RelativeLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:layout_width = "match_parent"
android:layout_height = "match_parent"
android:paddingLeft = "16dp"
android:background = "#d3d3d3"
android:paddingRight = "16dp">
<EditText
android:id = "@+id/name"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:hint = "First Name"
android:textColorHint = "@android:color/background_dark"
android:textColor = "@android:color/background_dark" />
<EditText
android:id = "@+id/lastName"
android:layout_width = "0dp"
android:layout_height = "wrap_content"
android:hint = "Last Name"
android:layout_below = "@id/name"
android:textColorHint = "@android:color/background_dark"
android:textColor = "@android:color/background_dark"
android:layout_alignParentLeft = "true"
android:layout_toLeftOf = "@+id/age" />
<EditText
android:id = "@id/age"
android:layout_width = "80dp"
android:layout_height = "wrap_content"
android:layout_below = "@id/name"
android:hint = "Age"
android:textColorHint = "@android:color/background_dark"
android:textColor = "@android:color/background_dark"
android:layout_alignParentRight = "true" />
<Button
android:layout_width = "85dp"
android:layout_height = "wrap_content"
android:layout_below = "@id/age"
android:layout_alignParentRight = "true"
android:text = "Submit"
android:background = "@android:color/holo_green_dark" />
</RelativeLayout>
此代码中我们使用的重要参数为 -
The important parameters that we have used in this code are −
-
android:layout_below − It aligns the child view element below its parent.
-
android:layout_alignParentLeft − It aligns the parent element to the left.
-
android:layout_toLeftOf − This property aligns an element to the left of another element.
-
android:layout_alignParentRight − It aligns the parent to the right.
当您现在构建并运行该应用程序时,它将生成以下输出屏幕 -
When you build and run the App now, it would produce the following output screen −
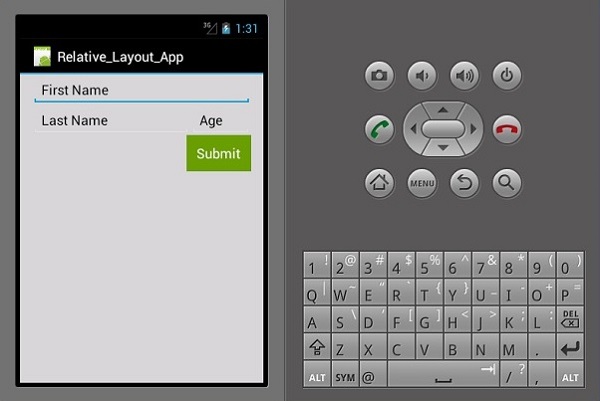
Frame Layout
框架布局用于仅显示一个项目。在此布局中排列多个项目而不会让它们相互重叠是很困难的。
The frame layout is used to display only one item. It’s difficult to arrange multiple items in this layout without having them overlap each other.
启动一个新项目并将其命名为 frameLayoutApp 。创建新的框架布局,如下所示。
Start a new project and call it frameLayoutApp. Create a new Frame Layout as shown below.
<?xml version = "1.0" encoding = "utf-8"?>
<FrameLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<ImageView
android:id = "@+id/ImageView1"
android:scaleType = "matrix"
android:layout_height = "fill_parent"
android:layout_width = "fill_parent"
android:src = "@drawable/img1" />
<TextView
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:textSize = "50dp"
android:textColor = "#000"
android:text = "This is a Lake" />
<TextView
android:gravity = "right"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:textSize = "50dp"
android:text = "A very Deep Lake"
android:layout_gravity = "bottom"
android:textColor = "#fff" />
</FrameLayout>
以上代码创建了一个 imageView ,它填满了整个屏幕。然后两个文本视图悬浮在 imageView 以上。
The above code creates an imageView which fills the entire screen. Two textviews then float above the imageView.
现在,构建并运行您的应用程序。它将显示以下输出 -
Now, build and run your application. It will display the following output −
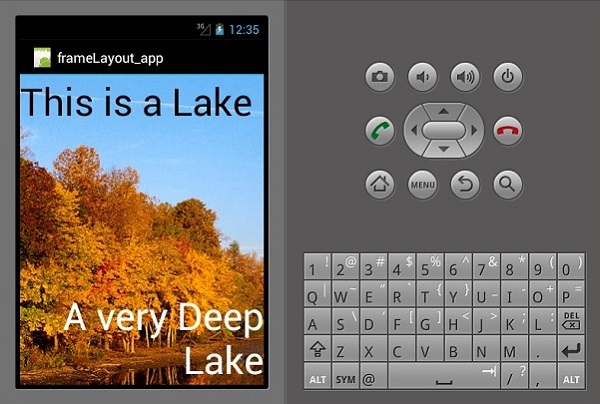
Table Layout
在此布局中,视图排列在 rows 和 columns 中。让我们看看其工作方式。
In this layout, the view is arranged into rows and columns. Let’s see how it works.
<?xml version = "1.0" encoding = "utf-8"?>
<TableLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:layout_width = "fill_parent"
android:background = "#d3d3d3"
android:layout_height = "fill_parent"
android:stretchColumns = "1">
<TableRow>
<TextView
android:text = "First Name:"
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:textColor = "@android:color/black" />
<EditText
android:width = "100px"
android:layout_width = "fill_parent"
android:layout_height = "30dp"
android:textColor = "@android:color/black" />
</TableRow>
<TableRow>
<TextView
android:text = "Last Name:"
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:textColor = "@android:color/black" />
<EditText
android:width = "50px"
android:layout_width = "fill_parent"
android:layout_height = "30dp"
android:textColor = "@android:color/black" />
</TableRow>
<TableRow>
<TextView
android:text = "Residence:"
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:textColor = "@android:color/black" />
<EditText
android:width = "100px"
android:layout_width = "fill_parent"
android:layout_height = "30dp"
android:textColor = "@android:color/black" />
</TableRow>
<TableRow>
<TextView
android:text = "Occupation:"
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:textColor = "@android:color/black" />
<EditText
android:width = "100px"
android:layout_width = "fill_parent"
android:layout_height = "30dp"
android:textColor = "@android:color/black" />
</TableRow>
<TableRow>
<Button
android:text = "Cancel"
android:layout_width = "wrap_content"
android:layout_margin = "10dp"
android:layout_height = "wrap_content"
android:background = "@android:color/holo_green_dark" />
<Button
android:text = "Submit"
android:width = "100px"
android:layout_margin = "10dp"
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:background = "@android:color/holo_green_dark" />
</TableRow>
</TableLayout>
以上代码创建了一个使用 tables 和 rows 布置的简单数据输入表单。
The above code creates a simple data entry form arranged using tables and rows.
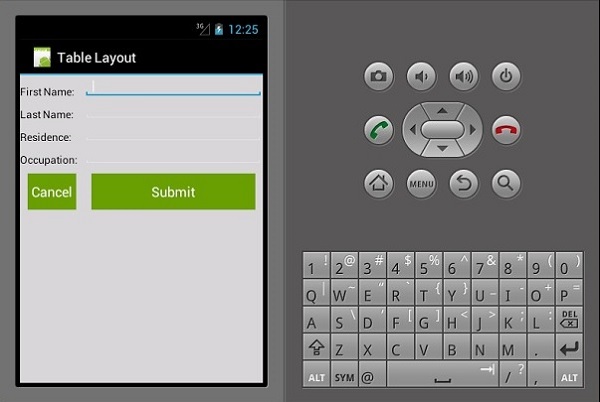
Xamarin - Android Widgets
Date Picker
这是一个用于显示日期的小组件。在此示例中,我们将创建一个日期选择器,它会在文本视图中显示设置的日期。
This is a widget used to display date. In this example, we are going to create a date picker which displays the set date on a text view.
首先,创建一个新项目并将其称为 datePickerExample 。打开 Main.axml 并创建一个 datepicker 、 textview 和一个 button 。
First of all, create a new project and call it datePickerExample. Open Main.axml and create a datepicker, textview, and a button.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<DatePicker
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/datePicker1" />
<TextView
android:text = "Current Date"
android:textAppearance = "?android:attr/textAppearanceLarge"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/txtShowDate" />
<Button
android:text = "Select Date"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/btnSetDate" />
</LinearLayout>
接下来,转到 Mainactivity.cs 。我们首先在 mainActivity:Activity 类中创建一个文本视图的私有实例。
Next, go to Mainactivity.cs. We first create a private instance of a textview inside the mainActivity:Activity class.
该实例将用于存储选定的日期或默认日期。
The instance will be used to store the date selected or the default date.
private TextView showCurrentDate;
接下来,在 setContentView() 方法之后添加以下代码。
Next, add the following code after setContentView() method.
DatePicker pickDate = FindViewById<DatePicker>(Resource.Id.datePicker1);
showCurrentDate = FindViewById<TextView>(Resource.Id.txtShowDate);
setCurrentDate();
Button button = FindViewById<Button>(Resource.Id.btnSetDate);
button.Click += delegate {
showCurrentDate.Text = String.Format("{0}/{1}/{2}",
pickDate.Month, pickDate.DayOfMonth, pickDate.Year);
};
在上面的代码中,我们通过使用 FindViewById 类从我们的 main.axml 文件中找到它们,从而引用了我们的日期选择器、文本视图和按钮。
In the above code, we have referenced our datepicker, textview, and button by finding them from our main.axml file using FindViewById class.
引用后,我们设置了按钮单击事件,该单击事件负责将选定的日期从日期选择器传递到文本视图。
After referencing, we set the button click event which is responsible for passing the selected date from the date picker to the textview.
接下来,我们创建 setCurrentDate() 方法,以将默认当前日期显示到我们的文本视图。以下代码说明了如何执行此操作。
Next, we create the setCurrentDate() method for displaying the default current date to our textview. The following code explains how it is done.
private void setCurrentDate() {
string TodaysDate = string.Format("{0}",
DateTime.Now.ToString("M/d/yyyy").PadLeft(2, '0'));
showCurrentDate.Text = TodaysDate;
}
DateTime.Now.ToString() 类将今天的日期时间绑定到字符串对象。
DateTime.Now.ToString() class binds today’s time to a string object.
现在,构建并运行该应用程序。它应该显示以下输出:
Now, build and run the App. It should display the following output −

Time Picker
时间选择器是一个用于显示时间以及允许用户选择和设置时间的小组件。我们将创建一个基本的 time picker 应用程序,该应用程序会显示时间,还允许用户更改时间。
Time Picker is a widget used to display time as well as allowing a user to pick and set time. We are going to create a basic time picker app that displays the time and also allows a user to change the time.
转到 main.axml 并添加一个新按钮、文本视图和时间选择器,如下面的代码中所示。
Go to main.axml and add a new button, textview, and a time picker as shown in the following code.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:background = "#d3d3d3"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<TimePicker
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/timePicker1" />
<TextView
android:text = "Time"
android:textAppearance = "?android:attr/textAppearanceLarge"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/txt_showTime"
android:textColor = "@android:color/black" />
<Button
android:text = "Set Time"
android:layout_width = "200dp"
android:layout_height = "wrap_content"
android:id = "@+id/btnSetTime"
android:textColor = "@android:color/black"
android:background = "@android:color/holo_green_dark" />
</LinearLayout>
转到 MainActivity.cs 来添加将设置日期显示在创建的文本视图上的功能。
Go to MainActivity.cs to add the functionality for displaying a set date on the textview we created.
public class MainActivity : Activity {
private TextView showCurrentTime;
protected override void OnCreate(Bundle bundle) {
base.OnCreate(bundle);
SetContentView(Resource.Layout.Main);
TimePicker Tpicker = FindViewById<TimePicker>(Resource.Id.timePicker1);
showCurrentTime = FindViewById<TextView>(Resource.Id.txt_showTime);
setCurrentTime();
Button button = FindViewById<Button>(Resource.Id.btnSetTime);
button.Click += delegate {
showCurrentTime.Text = String.Format("{0}:{1}",
Tpicker.CurrentHour, Tpicker.CurrentMinute);
};
}
private void setCurrentTime() {
string time = string.Format("{0}",
DateTime.Now.ToString("HH:mm").PadLeft(2, '0'));
showCurrentTime.Text = time;
}
}
在上面的代码中,我们首先通过 FindViewById<> 类引用 timepicker 、 set time 按钮和文本视图以显示时间。然后,我们为“设置时间”按钮创建了一个单击事件,该事件会在单击时将时间设置为某人选择的时间。默认情况下,它显示当前系统时间。
In the above code, we first referenced the timepicker,set time button and the textview for showing time through the FindViewById<> class. We then created a click event for the set time button which on click sets the time to the time selected by a person. By default, it shows the current system time.
setCurrentTime() 方法类初始化 txt_showTime textview 显示当前时间。
The setCurrentTime() method class initializes the txt_showTime textview to display the current time.
现在,构建并运行您的应用程序。它应显示以下输出 −
Now, build and run your application. It should display the following output −

Spinner
旋钮是用来自一组中选择一个选项的小部件。它相当于一个下拉框/组合框。首先,创建一个新项目并称其为 Spinner App Tutorial 。
A spinner is a widget used to select one option from a set. It is an equivalent of a dropdown/Combo box. First of all, create a new project and call it Spinner App Tutorial.
在 layout folder 下打开 Main.axml 并创建一个 spinner 。
Open Main.axml under the layout folder and create a new spinner.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<Spinner
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/spinner1"
android:prompt = "@string/daysOfWeek" />
</LinearLayout>
打开 values folder 中 Strings.xml 文件,并添加以下代码来创建 spinner items 。
Open Strings.xml file located under values folder and add the following code to create the spinner items.
<resources>
<string name = "daysOfWeek">Choose a planet</string>
<string-array name = "days_array">
<item>Sunday</item>
<item>Monday</item>
<item>Tuesday</item>
<item>Wednesday</item>
<item>Thursday</item>
<item>Friday</item>
<item>Saturday</item>
<item>Sunday</item>
</string-array>
</resources>
接下来,打开 MainActivity.cs 以添加用于显示已选星期的功能。
Next, open MainActivity.cs to add the functionality for displaying the selected day of the week.
protected override void OnCreate(Bundle bundle) {
base.OnCreate(bundle);
// Set our view from the "main" layout resource
SetContentView(Resource.Layout.Main);
Spinner spinnerDays = FindViewById<Spinner>(Resource.Id.spinner1);
spinnerDays.ItemSelected += new EventHandler
<AdapterView.ItemSelectedEventArgs>(SelectedDay);
var adapter = ArrayAdapter.CreateFromResource(this,
Resource.Array.days_array, Android.Resource.Layout.SimpleSpinnerItem);
adapter.SetDropDownViewResource(Android.Resource.Layout.SimpleSpinnerDropD ownItem);
spinnerDays.Adapter = adapter;
}
private void SelectedDay(object sender, AdapterView.ItemSelectedEventArgs e) {
Spinner spinner = (Spinner)sender;
string toast = string.Format("The selected
day is {0}", spinner.GetItemAtPosition(e.Position));
Toast.MakeText(this, toast, ToastLength.Long).Show();
}
现在,构建并运行此应用程序。它应显示以下输出 −
Now, build and run the application. It should display the following output −
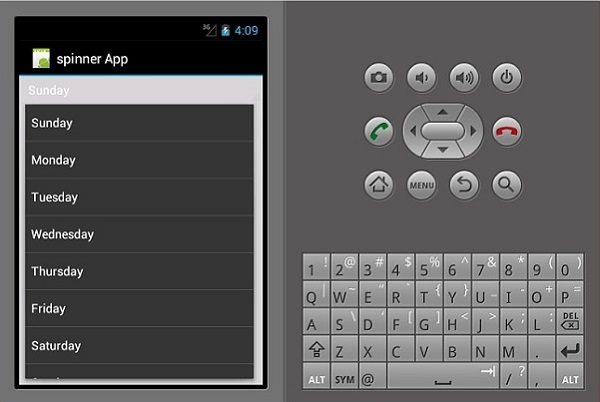
在上述代码中,我们引用了 main.axml 文件中创建的旋钮,方法是通过 FindViewById<> 类。然后,我们创建了一个 arrayAdapter() ,该类用于绑定 strings.xml 类的数组项。
In the above code, we referenced the spinner we created in our main.axml file through the FindViewById<> class. We then created a new arrayAdapter() which we used to bind our array items from the strings.xml class.
最后,我们创建了 SelectedDay() 方法,该类用于显示已选星期。
Finally we created the method SelectedDay() which we used to display the selected day of the week.
Xamarin - Android Dialogs
Alert Dialog
在本节中,我们将创建一个按钮,单击后显示一个警示对话框。对话框包含两个按钮,即 Delete 和 Cancel 按钮。
In this section, we are going to create a button which on clicked displays an alert dialog box. The dialog box contains two buttons, i.e., Delete and Cancel buttons.
首先,转至 main.axml 并创建线性布局内的按钮,如下面的代码所示。
First of all, go to main.axml and create a new button inside the linear layout as shown in the following code.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:layout_width = "fill_parent"
android:background = "#d3d3d3"
android:layout_height = "fill_parent">
<Button
android:id="@+id/MyButton"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:text = "Click to Delete"
android:textColor = "@android:color/background_dark"
android:background = "@android:color/holo_green_dark" />
</LinearLayout>
接下来,打开 MainActivity.cs 来创建警示对话框并添加其功能。
Next, open MainActivity.cs to create the alert dialog and add its functionality.
protected override void OnCreate(Bundle bundle) {
base.OnCreate(bundle);
SetContentView(Resource.Layout.Main);
Button button = FindViewById<Button>(Resource.Id.MyButton);
button.Click += delegate {
AlertDialog.Builder alertDiag = new AlertDialog.Builder(this);
alertDiag.SetTitle("Confirm delete");
alertDiag.SetMessage("Once deleted the move cannot be undone");
alertDiag.SetPositiveButton("Delete", (senderAlert, args) => {
Toast.MakeText(this, "Deleted", ToastLength.Short).Show();
});
alertDiag.SetNegativeButton("Cancel", (senderAlert, args) => {
alertDiag.Dispose();
});
Dialog diag = alertDiag.Create();
diag.Show();
};
}
完成后,构建并运行你的应用程序来查看输出。
Once done, build and run your Application to view the outcome.
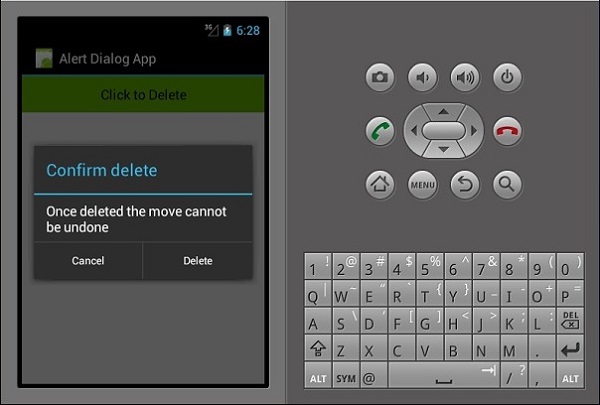
在上面的代码中,我们创建了一个称为 alertDiag 的警报对话框,它具有以下两个按钮 -
In the above code, we have created an alert dialog called alertDiag, with the following two buttons −
-
setPositiveButton − It contains the Delete button action which on clicked displays a confirmation message Deleted.
-
setNegativeButton − It contains a Cancel button which when clicked simply closes the alert dialog box.
Xamarin - Gallery
库房是一种用来显示水平可滚动列表中项的视图类型。然后,选定项目就会显示在中心。在此示例中,您将创建一个包含水平可滚动图像的库房。单击图像后,将显示选定图像的数字。
A Gallery is a type of view that is used to show items in a horizontal scrollable list. The selected item is then shown at the center. In this example, you are going to create a gallery containing images which are scrollable horizontally. An image when clicked will display a number for the selected image.
首先,创建一个项目并指定一个名称,例如“库房应用程序教程”。在您开始编码前,将图片粘贴到 resource /drawable folder 中。导航到 resources folder 中的 main.axml ,以及线性布局标记之间的库房。
First of all, create a new project and give it a name, e.g., Gallery App Tutorial. Before you start to code, paste 7 images into the resource /drawable folder. Navigate to main.axml under resources folder and a gallery in between the linear layout tags.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#d3d3d3">
<Gallery
android:id="@+id/gallery"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:padding="10dp" />
</LinearLayout>
创建一个名为 ImageAdapter 的新类。此类将用于将图像绑定到我们在上面创建的库房中。
Create a new class called ImageAdapter. This class is going to be used to bind the images to the gallery we created above.
第一步是添加一个类,其中包含我们用于存储字段的 cont 上下文。
The first step is to add a class that contains a context cont which we use to store fields.
public class ImageAdapter : BaseAdapter {
Context cont;
public ImageAdapter(Context ct) {
cont = ct;
}
}
接下来,我们计算图像数组,并返回其大小。
Next, we count the array list which contains our image and returns its size.
public override int Count {
get {
return imageArraylist.Length;
}
}
在下一步中,我们获取项目的坐标。以下代码演示了如何执行此操作。
In the next step, we get the position of the item. The following code shows how to do it.
public override Java.Lang.Object GetItem(int position) {
return null;
}
public override long GetItemId(int position) {
return 0;
}
在下一步中,我们创建 imageview ,用于适配器引用的项目。
In the next step, we create an imageview for the items referenced by the adapter.
public override View GetView(int position,View convertView, ViewGroup parent) {
ImageView img = new ImageView(cont);
img.SetImageResource(imageArraylist[position]);
img.SetScaleType(ImageView.ScaleType.FitXy);
img.LayoutParameters = new Gallery.LayoutParams(200, 100);
return img;
}
在最后一步中,我们创建 resources.drawable 文件夹中添加的图像引用。为执行此操作,我们创建一个存储图库的数组。以下代码说明了如何执行此操作。
In the final step, we create a reference to the images we added in the resources.drawable folder. To do this, we create an array to hold the collection of images. The following code explains how to do it.
int[] imageArraylist = {
Resource.Drawable.img1,
Resource.Drawable.img2,
Resource.Drawable.img3,
Resource.Drawable.img4,
Resource.Drawable.img5,
Resource.Drawable.img6,
};
}
接下来,我们转到 mainActivity.cs 并在 OnCreate() 方法下插入以下代码。
Next, we go to mainActivity.cs and insert the following code under the OnCreate() method.
Gallery myGallery = (Gallery)FindViewById<Gallery>(Resource.Id.gallery);
myGallery.Adapter = new ImageAdapter(this);
myGallery.ItemClick += delegate(object sender, AdapterView.ItemClickEventArgs args) {
Toast.MakeText(this,
args.Position.ToString(), ToastLength.Short).Show();
}
最后,构建并运行应用程序来查看输出。
Finally, build and run your application to view the output.

Xamarin - Andriod Views
ListViews
列表视图是显示可滚动项目列表的用户界面元素。
A Listview is a user interface element that displays lists of items that are scrollable.
Binding data to listviews
在此示例中,您将创建用于显示一周日期的 listView。首先,让我们创建一个新的 XML 文件,并将其命名为 listViewTemplate.xml 。
In this example, you are going to create a listView that displays the days of the week. To start with, let us create a new XML file and name it listViewTemplate.xml.
在 listViewTemplate.xml 中,我们添加一个新的 textview,如下所示。
In listViewTemplate.xml, we add a new textview as shown below.
<?xml version = "1.0" encoding = "utf-8" ?>
<TextView xmlns:android = "http://schemas.android.com/apk/res/android"
android:id = "@+id/textItem"
android:textSize ="20sp"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"/>
接下来,转到 Main.axml 并在线性布局内创建一个新的 listview。
Next, go to Main.axml and create a new listview inside the Linear Layout.
<ListView
android:minWidth="25px"
android:minHeight="25px"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/listView1" />
打开 MainActivity.cs 并输入以下代码以将数据绑定到我们创建的 listview。该代码必须写在 OnCreate() 方法中。
Open MainActivity.cs and type the following code to bind the data to the listview we created. The code must be written inside the OnCreate() method.
SetContentView(Resource.Layout.Main);
var listView = FindViewById<ListView>(Resource.Id.listView1);
var data = new string[] {
"Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"
};
listView.Adapter = new ArrayAdapter(this, Resource.Layout.ListViewTemplate, data);
Var data = new string[] 只是以数组形式保存我们的项目。
Var data = new string[] simply holds our items as an array.
数组适配器将我们集合中的项目作为视图返回。默认情况下,数组适配器使用默认的 textView 来显示每个项目。在上述代码中,我们在 ListViewTemplate.xml 中创建了自己的 textview 并使用下所示的构造函数引用它。
Array Adapter returns the items in our collection as a view. By default, the Array Adapter uses a default textView to display each item. In the above code, we created our own textview in ListViewTemplate.xml and referenced it using the constructor shown below.
ArrayAdapter(this, Resource.Layout.ListViewTemplate, data);
最后,构建并运行应用程序来查看输出。
Finally, build and run your application to view the output.

GridViews
网格视图是允许应用程序以二维方式布置内容的可滚动网格视图组。
A gridView is a view group that allows applications to lay out content in a two-dimensional way, scrollable grid.
要添加网格视图,请创建一个新项目并将其称为 gridViewApp 。转至 Main.axml 并按如下所示添加一个网格。
To add a GridView, create a new project and call it gridViewApp. Go to Main.axml and add a grid as shown below.
<?xml version = "1.0" encoding="utf-8"?>
<GridView xmlns:android = "http://schemas.android.com/apk/res/android"
android:id = "@+id/gridview"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent"
android:columnWidth = "90dp"
android:numColumns = "auto_fit"
android:verticalSpacing = "10dp"
android:horizontalSpacing = "10dp"
android:stretchMode = "columnWidth"
android:gravity = "center" />
接下来,创建一个新类并将其命名为 ImageAdpter.cs 。此类将包含所有将在网格中显示的项目的适配器类。
Next, create a new class and name it ImageAdpter.cs. This class will contain the adapter classes for all items which will be shown in the grid.
在 ImageAdapter 中,添加以下代码 −
Inside ImageAdapter, add the following code −
public class ImageAdapter : BaseAdapter {
Context context;
public ImageAdapter(Context ch) {
context = ch;
}
public override int Count {
get {
return cars.Length;
}
}
public override long GetItemId(int position) {
return 0;
}
public override Java.Lang.Object GetItem(int position) {
return null;
}
public override View GetView(int position,
View convertView, ViewGroup parent) {
ImageView imageView;
if (convertView == null) {
imageView = new ImageView(context);
imageView.LayoutParameters = new GridView.LayoutParams(100, 100);
imageView.SetScaleType(ImageView.ScaleType.CenterCrop);
imageView.SetPadding(8, 8, 8, 8);
} else {
imageView = (ImageView)convertView;
}
imageView.SetImageResource(cars[position]);
return imageView;
}
int[] cars = {
Resource.Drawable.img1, Resource.Drawable.img2,
Resource.Drawable.img3, Resource.Drawable.img4,
Resource.Drawable.img5, Resource.Drawable.img6,
};
}
在上述代码中,我们只是将汽车图片绑定到了图像适配器。接下来,打开 MainActivity.cs 并在 setContentView() 后添加以下代码。
In the above code, we have simply bound our car images to the image adapters. Next, open MainActivity.cs and add the following code after setContentView().
var gridview = FindViewById<GridView>(Resource.Id.gridview);
gridview.Adapter = new ImageAdapter(this);
gridview.ItemClick += delegate(object sender,
AdapterView.ItemClickEventArgs args) {
Toast.MakeText(this,
args.Position.ToString(), ToastLength.Short).Show();
};
上述代码在 main.axml 中找到网格视图,并将其绑定到 imageAdapter 类。 Gridview.ItemClick 创建一个 onClick 事件,当用户单击某个图像时,该事件会返回所选图像的位置。
The above code finds the gridView in main.axml and binds it to the imageAdapter class. Gridview.ItemClick creates an onClick event which returns the position of the selected image when a user clicks on an image.
现在,构建并运行您的应用程序以查看输出。
Now, build and run your application to view the output.

Xamarin - Multiscreen App
在本章中,我们将创建一个允许用户注册的登录系统。然后,我们将在成功登录后将注册用户带到我们的应用程序的主屏幕。
In this chapter, we are going to create a login system that enables a user to register. Then, we will take the registered user to the home screen of our App upon successful login.
首先,创建一个新项目并将其命名为 Login System 。在您的新项目中,转到 main.axml 并添加两个按钮和一个进度条,如下所示。
First of all, create a new project and call it Login System. On your new project, go to main.axml and add two buttons and a progress bar as shown below.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent"
android:background = "@android:color/background_light"
android:weightSum = "100"
android:minWidth = "25px"
android:minHeight = "25px">
<TextView
android:text = "Login App"
android:textAppearance = "?android:attr/textAppearanceMedium"
android:layout_width = "match_parent"
android:layout_weight = "20"
android:layout_height = "0dp"
android:textColor = "#368DEB"
android:id = "@+id/txtCreatAccount"
android:gravity = "center"
android:textStyle = "bold"
android:textSize = "25sp" />
<Button
android:text = "Sign In"
android:layout_width = "match_parent"
android:layout_weight = "15"
android:layout_height = "0dp"
android:background = "@drawable/btnSignInStyle"
android:id = "@+id/btnSignIn"
android:layout_marginLeft = "20dp"
android:layout_marginRight = "20dp"
android:textSize = "15sp" />
<Button
android:text = "Sign Up"
android:layout_width = "match_parent"
android:layout_weight = "15"
android:layout_height = "0dp"
android:background = "@drawable/btnSignUpStyle"
android:id = "@+id/btnSignUp"
android:layout_marginLeft = "20dp"
android:layout_marginRight = "20dp"
android:textSize = "15sp" />
<RelativeLayout
android:layout_width = "match_parent"
android:layout_height = "0dp"
android:layout_weight = "50"
android:minWidth = "25px"
android:minHeight = "25px">
<ProgressBar
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:id = "@+id/progressBar1"
android:background = "@drawable/progressBarStyle"
android:layout_centerInParent="true"
android:indeterminate = "true"
xmlns:tools = "
http://schemas.android.com/tools"
tools:visibility = "invisible" />
</RelativeLayout>
</LinearLayout>
在创建用户界面后,重要的是对按钮进行样式化以使它们看起来更具吸引力。为此,在 drawable folder 下创建一个新的 XML 文件并将文件命名为 btnSignInStyle.xml 。
After creating the user interface, it’s important to style the buttons to make them look more attractive. To do this, create a new XML file under drawable folder and name the file as btnSignInStyle.xml.
在 XML 文件中,添加以下代码行。
In the XML file, add the following lines of code −
<selector xmlns:android = "http://schemas.android.com/apk/res/android">
<item android:state_pressed = "false">
<layer-list>
<item android:right = "5dp" android:top = "5dp">
<shape>
<corners android:radius = "2dp"/>
<solid android:color = "#D6D6D6"/>
</shape>
</item>
<item android:left = "2dp" android:bottom = "2dp">
<shape>
<corners android:radius = "4dp"/>
<gradient android:angle = "270"
android:endColor = "#486EA9" android:startColor = "#486EA9"/>
<stroke android:width = "1dp" android:color = "#BABABA"/>
<padding android:bottom = "10dp"
android:right = "10dp" android:left = "10dp" android:top = "10dp"/>
</shape>
</item>
</layer-list>
</item>
<item android:state_pressed = "true">
<layer-list>
<item android:right = "5dp" android:top = "5dp">
<shape>
<corners android:radius = "2dp"/>
<solid android:color = "#D6D6D6"/>
</shape>
</item>
<item android:left = "2dp" android:bottom = "2dp">
<shape>
<corners android:radius = "4dp"/>
<gradient android:angle = "270"
android:endColor = "#79C791" android:startColor = "#486EA9"/>
<stroke android:radius = "4dp" android:color = "#BABABA"/>
<padding android:bottom = "10dp"
android:right = "10dp" android:left = "10dp" android:top = "10dp"/>
</shape>
</item>
</layer-list>
</item>
</selector>
以上代码在加载时设置按钮的颜色并在单击时设置按钮的边框半径。
The above code sets the colors of the button on load and on click, it also sets the border radius of the button.
接下来,我们为 signup 按钮创建一个与上面类似的样式 XML。为此,在 drawable 文件夹下创建另一个 XML 称之为 btnSignUpStyle.xml 。它将继承 btnSignInStyle.xml 中的一切。唯一的区别是按钮的渐变起始和结束颜色。
Next, we create a similar styling XML as above for the signup button. To do this, create another XML under drawable folder and call it btnSignUpStyle.xml. It will inherit everything from btnSignInStyle.xml. The only difference will be the buttons’ gradient start and end color.
在 btnSignUpStyle.xml 中将 startColor 和 endColor 更改为
Change the startColor and endColor in btnSignUpStyle.xml to
<gradient android:angle="270"
android:endColor="#008000" android:startColor="#008000"/>
转到 layout folder 并创建新的 AXML 文件,称之为 registerDailog.axml。此文件将包含我们应用程序中新用户的注册详细信息。该页面将包含三个 EditTexts 和一个用于提交数据的按钮。在您的线性布局代码中添加以下代码。
Go to layout folder and create a new AXML file and call it registerDailog.axml. This file will contain registration details for new users in our app. The page will contain three EditTexts and a button to submit the data. Add the following code inside your linear layout code.
<EditText
android:layout_width = "match_parent"
android:layout_marginBottom = "10dp"
android:layout_marginTop = "25dp"
android:layout_marginRight = "25dp"
android:layout_marginLeft = "25dp"
android:layout_height = "35dp"
android:paddingLeft = "10dp"
android:id = "@+id/txtUsername"
android:hint = "Username"
android:textColor = "#000" />
<EditText
android:layout_width = "match_parent"
android:layout_height = "35dp"
android:id = "@+id/txtEmail"
android:layout_marginBottom = "10dp"
android:layout_marginTop = "25dp"
android:layout_marginRight = "25dp"
android:layout_marginLeft = "25dp"
android:paddingLeft = "10dp"
android:textColor = "#000"
android:hint = "Email" />
<EditText
android:layout_width = "match_parent"
android:layout_height = "35dp"
android:layout_marginBottom = "10dp"
android:layout_marginTop = "25dp"
android:layout_marginRight = "25dp"
android:layout_marginLeft = "25dp"
android:paddingLeft = "10dp"
android:textColor = "#000"
android:id = "@+id/txtPassword"
android:hint = "Password" />
<Button
android:text = "Sign Up"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/btnSave"
android:textSize = "20dp"
android:textColor = "#fff"
android:textStyle = "bold"
android:height = "70dp"
android:background = "@drawable/btnSignUpStyle"
android:paddingLeft = "5dp"
android:paddingRight = "5dp"
android:paddingTop = "5dp"
android:paddingBottom = "5dp"
android:layout_marginLeft = "25dp"
android:layout_marginRight = "25dp"
android:layout_centerHorizontal = "true" />
接下来,添加一个名为 signUpDialog.cs 的新类。此类将包含创建对话框所需的代码。以下示例显示了该代码。
Next, add a new class called signUpDialog.cs. This class will contain the code required to create a dialog box. The following example shows the code.
public class OnSignUpEvent:EventArgs {
private string myUserName;
private string myEmail;
private string myPassword;
public string UserName {
get {
return myUserName;
}
set{
myUserName = value;
}
}
public string Email {
get {
return myEmail;
}
set {
myEmail = value;
}
}
public string Password {
get {
return myPassword;
}
set {
myPassword = value;
}
}
public OnSignUpEvent(string username, string
email, string password):base() {
UserName = username;
Email = email;
Password = password;
}
class SignUpDialog:DialogFragment {
private EditText txtUsername;
private EditText txtEmail;
private EditText txtPassword;
private Button btnSaveSignUp;
public event EventHandler<OnSignUpEvent> onSignUpComplete;
public override View OnCreateView(LayoutInflater inflater,
ViewGroup container, Bundle savedInstanceState) {
base.OnCreateView(inflater, container, savedInstanceState);
var view = inflater.Inflate(Resource.Layout.registerDialog, container, false);
txtUsername = view.FindViewById<EditText>(Resource.Id.txtUsername);
txtEmail = view.FindViewById<EditText>(Resource.Id.txtEmail);
txtPassword = view.FindViewById<EditText>(Resource.Id.txtPassword);
btnSaveSignUp = view.FindViewById<Button>(Resource.Id.btnSave);
btnSaveSignUp.Click += btnSaveSignUp_Click;
return view;
}
void btnSaveSignUp_Click(object sender, EventArgs e) {
onSignUpComplete.Invoke(this, new OnSignUpEvent(txtUsername.Text,
txtEmail.Text, txtPassword.Text));
this.Dismiss();
}
}
}
在以上代码中,我们使用了 get 和 set 属性。 get 方法返回一个变量,而 set 方法将某个值指定给返回的变量。以下是一个示例:
In the above code, we have used the get and set properties. The get method returns a variable, while the set method assigns a value to the returned variable. Here is an example −
public string Color {
get {
return color;
}
set {
color = value;
}
}
在我们的前一个示例中,我们创建了一个覆盖视图的方法。在该方法内,我们创建了一个名为 view 的 var ,它引用了布局文件夹中包含的 registerDialog.axml 。
In our previous example, we created a method that overrides a view. Inside the method, we created a var called view which referenced to a registerDialog.axml contained in the layout folder.
接下来,转到 mainActivity.cs 来创建对话框片段。
Next, go to mainActivity.cs to create the dialog fragment.
private Button signUp;
private Button submitNewUser;
private EditText txtUsername;
private EditText txtEmail;
private EditText txtPassword;
protected override void OnCreate(Bundle bundle) {
base.OnCreate(bundle);
SetContentView(Resource.Layout.Main);
signUp = FindViewById<Button>(Resource.Id.btnSignUp);
submitNewUser = FindViewById<Button>(Resource.Id.btnSave);
txtUsername = FindViewById<EditText>(Resource.Id.txtUsername);
txtEmail = FindViewById<EditText>(Resource.Id.txtEmail);
txtPassword = FindViewById<EditText>(Resource.Id.txtPassword);
signUp.Click += (object sender, EventArgs args) => {
FragmentTransaction transFrag = FragmentManager.BeginTransaction();
SignUpDialog diagSignUp = new SignUpDialog();
diagSignUp.Show(transFrag, "Fragment Dialog");
diagSignUp.onSignUpComplete += diagSignUp_onSignUpComplete;
};
}
void diagSignUp_onSignUpComplete(object sender, OnSignUpEvent e) {
StartActivity(typeof(Activity2));
}
上方的代码包含一个按钮点击事件,当点击时,加载注册对话框。在按钮点击内部,我们创建了一个 SignUpDialog 类来加载 registerDialog.axml 文件。
The above code contains a button click event which when clicked loads the signUp dialog. Inside the button click, we created a SignUpDialog class which loads the registerDialog.axml file.
然后我们使用 FragmentTransaction transFrag = FragmentManager.BeginTransaction(); 来将我们的 registerDialog 页面显示为 Android 对话片段。
We then used FragmentTransaction transFrag = FragmentManager.BeginTransaction(); to show our registerDialog page as an Android Dialog Fragment.
我们将添加另一个名为 home.axml 的 .axml 文件。一旦用户成功登录系统,此布局将成为登陆界面。在此布局中,我们将添加一个文本视图,如下面的代码所示。
We are going to add another .axml file called home.axml. This layout will be the landing screen once a user successfully logs into the system. Inside this layout, we are going to add a textview as shown in the following code.
<TextView
android:text = "You have been succesfully registered. Welcome!"
android:textAppearance = "?android:attr/textAppearanceLarge"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/textView1" />
接下来,我们创建一个名为 Activity2.cs 的最终活动。在这个活动中,我们将使用 findViewById 查找 home.axml 。
Next, we create a final activity called Activity2.cs. In this activity, we are going to find the home.axml using findViewById.
最后,构建并运行你的 App。它将显示以下屏幕作为输出。
Finally, build and run your App. It will display the following screens as output.

Xamarin - Deploying Your App
在完成构建您的应用的过程后,将其用于物理 Android 设备或允许其他人下载您的应用并将其安装到其设备上非常重要。
After completing the process of building your App, it’s important to use this App on a physical Android device or allow other people to download your App and install it on their devices.
Releasing Your App
在发布您的应用之前,务必将其转换为 Android 系统可以读取的格式。这种格式称为 apk file 。要创建一个 apk file 。
Before releasing your App, it is important to convert it into a format that can be read by an Android system. This type of format is called an apk file. To create an apk file.
-
Open your project.
-
Go to Build Menu and select Configuration Manager
-
On Configuration Manager, set Active Solution Configuration to release the App.
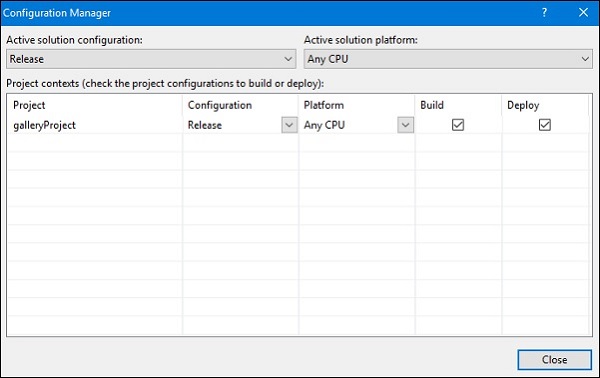
接下来,再次单击 Build Menu 并选择 Export Android Package(.apk) 。
Next, click the Build Menu again and select Export Android Package(.apk).
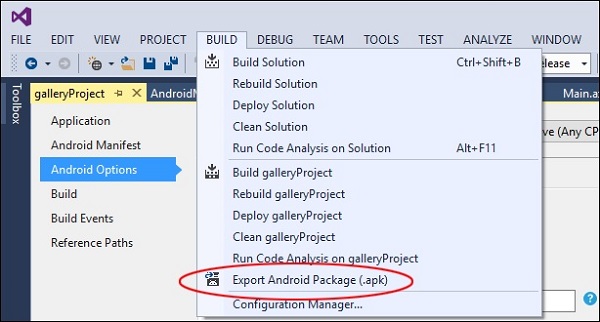
完成后, apk 文件将存储在项目文件夹 /bin/Release 中。
Once finished, the apk file will be stored in your project folder /bin/Release.
Google PlayStore
PlayStore 是最大的安卓应用市场。要将您的应用上传到 PlayStore,您需要拥有一个 Google 开发者帐户。开发者帐户创建一次,获得许可证需要花费 25 美元。
PlayStore is the largest market for Android apps. To upload your App to the PlayStore, you need to have a developer account with Google. The developer account is created once and costs $25 to get a license.
Manual Installation
手动安装涉及将生成的 .apk 文件直接安装到物理设备上。将文件复制到您的安卓设备的物理内存或 SD 卡上,然后从您的设备运行该文件。
Manual installation involves installing the .apk file generated directly on a physical device. Copy the file to your Android device’s physical memory or an SD card and then run the file from your device.
默认情况下,安卓会阻止安装并非来自 PlayStore 的应用。要安装您的应用,您必须启用它以接受来自 Settings 的应用安装。要执行此操作,请转到设备上的 Settings ,查找 Security 菜单,然后选中“允许安装来自未知来源的应用”。
Android, by default, blocks installation of Apps that are not from PlayStore. To install your App, you must enable it to accept the App installation from the Settings. To do this, go to Settings on your device, look for Security menu, and then then check "Allow installation of apps from unknown sources."
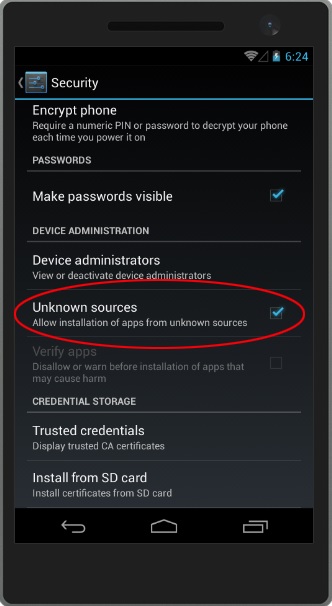