Microservice Architecture 简明教程
Microservice Architecture - Hands-On MSA
在本章中,我们将构建一个微服务应用程序,它将消耗不同的可用服务。我们都知道,微服务并不是构建应用程序的经济有效的方法,因为我们构建的每一项服务本质上都是全栈的。在本地环境中构建微服务需要高端系统配置,因为你需要四台服务器实例才能持续运行,以便在某一时间点能消耗它。为了构建我们有史以来的第一个微服务,我们将使用一些可用的 SOA 端点,并在我们的应用程序中消耗它们。
In this chapter, we will build one microservice application that will consume different available services. We all know that microservice is not a cost-effective way to build an application as each and every service we build will be full stack in nature. Building a microservice in the local environment would need high-end system configuration, as you need to have four instances of a server to keep running such that it can be consumed at a point of time. To build our first ever microservice, we will use some of the available SOA endpoints and we will consume the same in our application.
System Configuration and Setup
在进入构建阶段之前,请做好系统准备。你需要一些公共 Web 服务。你可以轻松地进行谷歌搜索。如果你想消耗 SOAP Web 服务,那么你将得到一个 WSDL 文件,然后你需要从中消耗特定的 Web 服务。对于 REST 服务,你只需要一个链接来消耗它。在这个示例中,你将把三个不同的 Web 服务“SOAP”、“REST”和“自定义”嵌入到一个应用程序中。
Before going further to the build phase, prepare your system accordingly. You would need some public web services. You can easily google for this. If you want to consume SOAP web service, then you will get one WSDL file and from there you need to consume the specific web service. For REST service, you will need only one link to consume the same. In this example, you will jam three different web services “SOAP”, “REST”, and “custom” in one application.
Application Architecture
你将使用微服务实现计划创建一个 Java 应用程序。你将创建一个自定义服务,此服务的输出将作为其他服务的输入。
You will create a Java application using microservice implementation plan. You will create a custom service and the output of this service will work as an input for other services.
下面是开发微服务应用程序的步骤。
Following are the steps to follow to develop a microservice application.
Step 1: Client creation for SOAP service − 有许多免费 Web API 可用于学习 Web 服务。为本教程的目的,使用“ http://www.webservicex.net/.” 的 GeoIP 服务。他们的网站上以“ webservicex.net. 提供了 WSDL 文件。要从该 WSDL 文件中生成客户端,你需要做的就是在你的终端中运行以下命令。
Step 1: Client creation for SOAP service − There are many free web APIs available to learn a web service. For the purpose of this tutorial, use the GeoIP service of “http://www.webservicex.net/.” The WSDL file is provided in the following link on their website “webservicex.net. To generate the client out of this WSDL file, all you need to do is run the following command in your terminal.
wsimport http://www.webservicex.net/geoipservice.asmx?WSDL
此命令将在一个名为“SEI”的文件夹(以服务端点接口命名)下生成所有必需的客户端文件。
This command will generate all the required client files under one folder named “SEI”, which is named after service end point interface.
Step 2: Create your custom web service − 按照本教程前面阶段中提到的相同过程,构建基于 Maven 的 REST api,名为“CustomRest”。完成后,你会找到一个名为“MyResource.java”的类。继续并使用以下代码更新此类。
Step 2: Create your custom web service − Follow the same process mentioned at an earlier stage in this tutorial and build a Maven-based REST api named “CustomRest”. Once complete, you will find a class named “MyResource.java”. Go ahead and update this class using the following code.
package com.tutorialspoint.customrest;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("myresource")
public class MyResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String getIt() {
return "IND|INDIA|27.7.65.215";
}
}
一切完成后,请在本机服务器上运行此应用程序。你应该在浏览器中获得以下输出。
Once everything is complete, go ahead and run this application on the server. You should get the following output in the browser.

这是 Web 服务器,它在被调用后返回一个字符串对象。这是一项输入服务,它提供可被其他应用程序消耗以生成记录的输入。
This is the web server, which returns one string object once it is called. This is the input service that provides inputs that can be consumed by other application to generate records.
Step 3: Configure another Rest API − 在此步骤中,消耗 services.groupkt.com. 可用的另一项 Web 服务。调用时,这将返回一个 JSON 对象。
Step 3: Configure another Rest API − In this step, consume another web service available at services.groupkt.com. This will return a JSON object when invoked.
Step 4: Create JAVA application − 通过选择“新项目”→“JAVA 项目”创建一个正常的 Java 应用程序,然后按如下截图所示单击“完成”。
Step 4: Create JAVA application − Create one normal Java application by selecting “New Project” → “JAVA project” and hit Finish as shown in the following screenshot.

Step 5: Add the SOAP client − 在步骤 1 中,你创建了 SOAP Web 服务的客户端文件。继续将这些客户端文件添加到你当前的项目。成功添加客户端文件后,你的应用程序目录将如下所示。
Step 5: Add the SOAP client − In step 1, you have created the client file for the SOAP web service. Go ahead and add these client files to your current project. After successful addition of the client files, your application directory will be look the following.
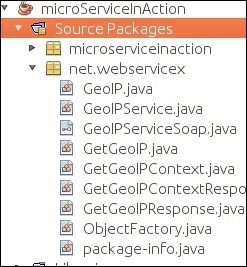
Step 6: Create your main app − 创建一个主类,你将在其中消耗所有这三个 Web 服务。右键单击源项目,并创建一个名为“MicroServiceInAction.java”的新类。接下来,从这里调用不同的 Web 服务。
Step 6: Create your main app − Create your main class where you will consume all of these three web services. Right-click on the source project and create a new class named “MicroServiceInAction.java”. Next task is to call different web services from this.
Step 7: Call your custom web service − 为此,继续添加以下代码集以实现调用自己的服务。
Step 7: Call your custom web service − For this, go ahead and add the following set of codes to implement calling your own service.
try {
url = new URL("http://localhost:8080/CustomRest/webapi/myresource");
conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setRequestProperty("Accept", "application/json");
if (conn.getResponseCode() != 200) {
throw new RuntimeException("Failed : HTTP error code : " + conn.getResponseCode());
}
BufferedReader br = new BufferedReader(new InputStreamReader(
(conn.getInputStream())));
while ((output = br.readLine()) != null) {
inputToOtherService = output;
}
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
Step 8: Consume SOAP Services − 你生成了客户端文件,但你不知道该在整个软件包中调用哪个方法?为此,你需要再次参考你用来生成客户端文件的 WSDL。每个 WSDL 文件都应有一个“wsdl:service”标签,搜索此标签。它应该是该 Web 服务的入口点。以下是该应用程序的服务端点。
Step 8: Consume SOAP Services − You have generated your client file but you don’t know which method should be called in that entire package? For this, you need to refer to the WSDL again, which you used to generate your client files. Every WSDL file should have one “wsdl:service” tag search for this tag. It should be your entry point of that web service. Following is the service endpoint of this application.
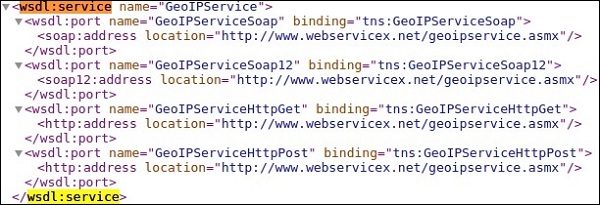
现在你需要在应用程序中实现此服务。以下是实现 SOAP Web 服务所需的 Java 代码集。
Now you need to implement this service in your application. Following is the set of Java code you need to implement your SOAP web service.
GeoIPService newGeoIPService = new GeoIPService();
GeoIPServiceSoap newGeoIPServiceSoap = newGeoIPService.getGeoIPServiceSoap();
GeoIP newGeoIP = newGeoIPServiceSoap.getGeoIP(Ipaddress);
// Ipaddress is output of our own web service.
System.out.println("Country Name from SOAP Webserivce ---"+newGeoIP.getCountryName());
Step 9: Consume REST web service − 到目前为止已经消耗了其中两项服务。在此步骤中,将在自定义 Web 服务的帮助下消耗另一项带有自定义 URL 的 REST Web 服务。请使用以下代码集执行此操作。
Step 9: Consume REST web service − Two of the services have been consumed till now. In this step, another REST web service with customized URL will be consumed with the help of your custom web service. Use the following set of code to do so.
String url1="http://services.groupkt.com/country/get/iso3code/";//customizing the Url
url1 = url1.concat(countryCode);
try {
URL url = new URL(url1);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setRequestProperty("Accept", "application/json");
if (conn.getResponseCode() != 200) {
throw new RuntimeException("Failed : HTTP error code : " + conn.getResponseCode());
}
BufferedReader br = new BufferedReader(new InputStreamReader(
(conn.getInputStream())));
while ((output = br.readLine()) != null) {
System.out.println(output);
}
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
Step 10: Consume all services − 如果你正在运行“CustomRest”Web 服务且已连接到互联网,如果一切都已成功完成,那么以下内容应为你合并的主类。
Step 10: Consume all services − Considering your “CustomRest” web service is running and you are connected to Internet, if everything is completed successfully then following should be your consolidated main class.
package microserviceinaction;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.StringTokenizer;
import net.webservicex.GeoIP;
import net.webservicex.GeoIPService;
import net.webservicex.GeoIPServiceSoap;
public class MicroServiceInAction {
static URL url;
static HttpURLConnection conn;
static String output;
static String inputToOtherService;
static String countryCode;
static String ipAddress;
static String CountryName;
public static void main(String[] args) {
//consuming of your own web service
try {
url = new URL("http://localhost:8080/CustomRest/webapi/myresource");
conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setRequestProperty("Accept", "application/json");
if (conn.getResponseCode() != 200) {
throw new RuntimeException("Failed : HTTP error code : " + conn.getResponseCode());
}
BufferedReader br = new BufferedReader(new InputStreamReader(
(conn.getInputStream())));
while ((output = br.readLine()) != null) {
inputToOtherService = output;
}
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
//Fetching IP address from the String and other information
StringTokenizer st = new StringTokenizer(inputToOtherService);
countryCode = st.nextToken("|");
CountryName = st.nextToken("|");
ipAddress = st.nextToken("|");
// Call to SOAP web service with output of your web service---
// getting the location of our given IP address
String Ipaddress = ipAddress;
GeoIPService newGeoIPService = new GeoIPService();
GeoIPServiceSoap newGeoIPServiceSoap = newGeoIPService.getGeoIPServiceSoap();
GeoIP newGeoIP = newGeoIPServiceSoap.getGeoIP(Ipaddress);
System.out.println("Country Name from SOAP Webservice ---"+newGeoIP.getCountryName());
// Call to REST API --to get all the details of our country
String url1 = "http://services.groupkt.com/country/get/iso3code/"; //customizing the Url
url1 = url1.concat(countryCode);
try {
URL url = new URL(url1);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setRequestProperty("Accept", "application/json");
if (conn.getResponseCode() != 200) {
throw new RuntimeException("Failed : HTTP error code : " + conn.getResponseCode());
}
BufferedReader br = new BufferedReader(new InputStreamReader(
(conn.getInputStream())));
while ((output = br.readLine()) != null) {
System.out.println(output);
}
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
一旦运行此文件,你将看到控制台中的以下输出。你已成功开发了你的第一个微服务应用程序。
Once you run this file, you will see the following output in the console. You have successfully developed your first microservice application.
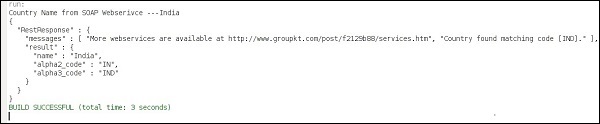