Dotnet Core 简明教程
.NET Core - Create .NET Standard Library
一个类库定义类型和方法,这些类型和方法可以从任何应用程序调用。
-
使用 .NET Core 开发的类库支持 .NET 标准库,这将允许你的库被支持该版本 .NET 标准库的任何 .NET 平台调用。
-
当你完成你的类库时,你可以决定是否要将它作为第三方组件分发,或者你是否希望将它作为与一个或多个应用程序捆绑在一起的组件包含在内。
让我们以在我们的控制台应用程序中添加一个类库项目作为开始,在解决方案资源管理器中右键单击 src 文件夹并且选择 Add → New Project…

在 Add New Project 对话框中选择 .NET Core 节点,然后选择类库(.NET Core)项目模板。
在“名称”文本框中,输入“UtilityLibrary”作为项目名称,如下图所示。

单击“确定”创建一个类库项目。在创建项目之后,我们添加一个新类。右键单击“解决方案资源管理器”中的 project 并选择 Add → Class…
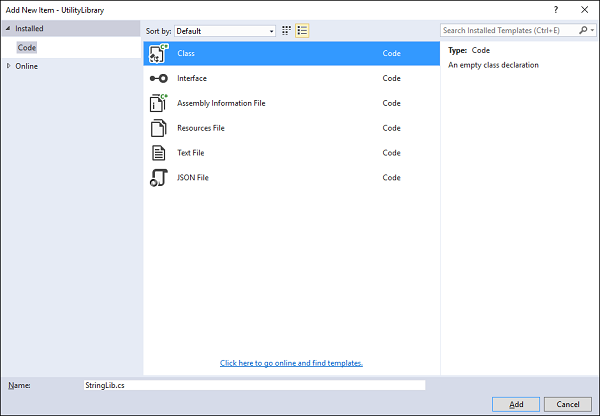
在中间窗格中选择类并在“名称”和“字段”中输入StringLib.cs,然后单击 Add 。一旦添加了类,则替换StringLib.cs文件中的以下代码。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace UtilityLibrary {
public static class StringLib {
public static bool StartsWithUpper(this String str) {
if (String.IsNullOrWhiteSpace(str))
return false;
Char ch = str[0];
return Char.IsUpper(ch);
}
public static bool StartsWithLower(this String str) {
if (String.IsNullOrWhiteSpace(str))
return false;
Char ch = str[0];
return Char.IsLower(ch);
}
public static bool StartsWithNumber(this String str) {
if (String.IsNullOrWhiteSpace(str))
return false;
Char ch = str[0];
return Char.IsNumber(ch);
}
}
}
-
类库 UtilityLibrary.StringLib 包含一些方法,如 StartsWithUpper 、 StartsWithLower 和 StartsWithNumber ,这些方法将返回一个布尔值,该布尔值指示当前字符串实例分别以大写、小写和数字开头。
-
在.NET Core中,如果某个字符是大写,则 Char.IsUpper 方法返回true;如果某个字符是小写,则Char.IsLower方法返回true;同样,如果某个字符是数字字符,则Char.IsNumber方法返回true。
-
在菜单栏中,选择“生成”,然后选择“生成解决方案”。项目应该能够在没有错误的情况下编译。
-
我们的.NET Core控制台项目无法访问我们的类库。
-
现在,若要使用此类库,我们需要在控制台项目中添加此类库的引用。
为此,请展开FirstApp,然后右键单击“引用”,最后选择 Add Reference…

在“引用管理器”对话框中,选择我们的类库项目UtilityLibrary,然后单击 OK 。
现在让我们打开控制台项目的Program.cs文件,并将所有代码替换为以下代码。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using UtilityLibrary;
namespace FirstApp {
public class Program {
public static void Main(string[] args) {
int rows = Console.WindowHeight;
Console.Clear();
do {
if (Console.CursorTop >= rows || Console.CursorTop == 0) {
Console.Clear();
Console.WriteLine("\nPress <Enter> only to exit; otherwise, enter a string and press <Enter>:\n");
}
string input = Console.ReadLine();
if (String.IsNullOrEmpty(input)) break;
Console.WriteLine("Input: {0} {1,30}: {2}\n", input, "Begins with uppercase? ",
input.StartsWithUpper() ? "Yes" : "No");
} while (true);
}
}
}
现在让我们运行应用程序,您将看到以下输出。

为了更好地理解,让我们在您的项目中使用您的类库的其他扩展方法。