Pdfbox 简明教程
PDFBox - Encrypting a PDF Document
在上一章中,我们已经了解如何向 PDF 文档中插入图片。在本章中,我们讨论如何加密 PDF 文档。
In the previous chapter, we have seen how to insert an image in a PDF document. In this chapter, we will discuss how to encrypt a PDF document.
Encrypting a PDF Document
可以使用 StandardProtectionPolicy 和 AccessPermission classes 提供的方法加密 PDF 文档。
You can encrypt a PDF document using the methods provided by StandardProtectionPolicy and AccessPermission classes.
AccessPermission 类用于通过向 PDF 文档分配访问权限来保护它。使用此类,可以限制用户执行以下操作。
The AccessPermission class is used to protect the PDF Document by assigning access permissions to it. Using this class, you can restrict users from performing the following operations.
-
Print the document
-
Modify the content of the document
-
Copy or extract content of the document
-
Add or modify annotations
-
Fill in interactive form fields
-
Extract text and graphics for accessibility to visually impaired people
-
Assemble the document
-
Print in degraded quality
StandardProtectionPolicy 类用于为文档添加基于密码的保护。
The StandardProtectionPolicy class is used to add a password based protection to a document.
以下是加密现有 PDF 文档的步骤。
Following are the steps to encrypt an existing PDF document.
Step 1: Loading an Existing PDF Document
使用 PDDocument 类的静态方法 load() 加载现有 PDF 文档。此方法接受一个文件对象作为参数,因为这是一个静态方法,您可使用类名调用它,如下所示:
Load an existing PDF document using the static method load() of the PDDocument class. This method accepts a file object as a parameter, since this is a static method you can invoke it using class name as shown below.
File file = new File("path of the document")
PDDocument document = PDDocument.load(file);
Step 2: Creating Access Permission Object
实例化 AccessPermission 类,如下所示。
Instantiate the AccessPermission class as shown below.
AccessPermission accessPermission = new AccessPermission();
Step 3: Creating StandardProtectionPolicy Object
使用 StandardProtectionPolicy 类,通过传递所有者密码、用户密码和 AccessPermission 对象,按照如下所示进行实例化。
Instantiate the StandardProtectionPolicy class by passing the owner password, user password, and the AccessPermission object as shown below.
StandardProtectionPolicy spp = new StandardProtectionPolicy("1234","1234",accessPermission);
Step 4: Setting the Length of the Encryption Key
使用 setEncryptionKeyLength() 方法设置加密密钥长度,按照如下所示进行。
Set the encryption key length using the setEncryptionKeyLength() method as shown below.
spp.setEncryptionKeyLength(128);
Step 5: Setting the Permissions
使用 StandardProtectionPolicy 类的 setPermissions() 方法设置权限。此方法接受 AccessPermission 对象作为参数。
Set the permissions using the setPermissions() method of the StandardProtectionPolicy class. This method accepts an AccessPermission object as a parameter.
spp.setPermissions(accessPermission);
Step 6: Protecting the Document
您可以使用 PDDocument 类的 protect() 方法来保护您的文档,按照如下所示进行。将 StandardProtectionPolicy 对象作为参数传递给此方法。
You can protect your document using the protect() method of the PDDocument class as shown below. Pass the StandardProtectionPolicy object as a parameter to this method.
document.protect(spp);
Example
假设我们有一个名为 sample.pdf 的 PDF 文档,路径为 C:/PdfBox_Examples/ ,并且页面为空,如下所示。
Suppose, we have a PDF document named sample.pdf, in the path C:/PdfBox_Examples/ with empty pages as shown below.
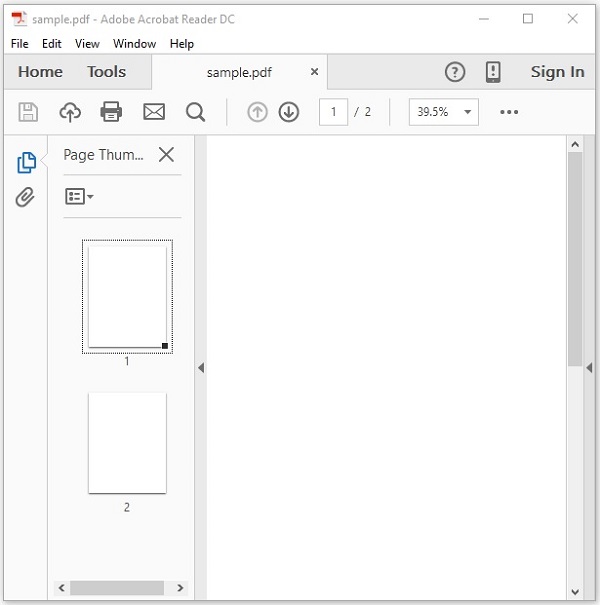
此示例演示如何加密上述的 PDF 文档。在此,我们将加载名为 sample.pdf 的 PDF 文档并对其进行加密。将此代码保存在名为 EncriptingPDF.java. 的文件中。
This example demonstrates how to encrypt the above mentioned PDF document. Here, we will load the PDF document named sample.pdf and encrypt it. Save this code in a file with name EncriptingPDF.java.
import java.io.File;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.encryption.AccessPermission;
import org.apache.pdfbox.pdmodel.encryption.StandardProtectionPolicy;
public class EncriptingPDF {
public static void main(String args[]) throws Exception {
//Loading an existing document
File file = new File("C:/PdfBox_Examples/sample.pdf");
PDDocument document = PDDocument.load(file);
//Creating access permission object
AccessPermission ap = new AccessPermission();
//Creating StandardProtectionPolicy object
StandardProtectionPolicy spp = new StandardProtectionPolicy("1234", "1234", ap);
//Setting the length of the encryption key
spp.setEncryptionKeyLength(128);
//Setting the access permissions
spp.setPermissions(ap);
//Protecting the document
document.protect(spp);
System.out.println("Document encrypted");
//Saving the document
document.save("C:/PdfBox_Examples/sample.pdf");
//Closing the document
document.close();
}
}
使用以下命令从命令提示符处编译并执行已保存的 Java 文件。
Compile and execute the saved Java file from the command prompt using the following commands.
javac EncriptingPDF.java
java EncriptingPDF
执行完上述程序之后,会对给定的 PDF 文档加密并显示以下信息。
Upon execution, the above program encrypts the given PDF document displaying the following message.
Document encrypted
如果您尝试打开文档 sample.pdf ,则您无法打开它,因为它已被加密。于是,它会提示您输入密码以打开文档,按照如下所示进行。
If you try to open the document sample.pdf, you cannot, since it is encrypted. Instead, it prompts to type the password to open the document as shown below.
