Javafx 简明教程
JavaFX - Material Property
到目前为止,当我们讨论 JavaFX 形状对象的属性时,它一直只包括形状填充的颜色和形状描边的类型。然而,JavaFX 还允许为 3D 形状指定材料的类型。
Until now, when we addressed the properties of a JavaFX shape object, it always only included the color of shape fill and type of shape strokes. However, JavaFX also allows you to assign the type of material for a 3D shape.
3D 物体的材质就是用来创建 3D 物体的纹理类型。例如,现实世界中的盒子可以用不同的材质制成;例如纸板、金属、木材或任何其他固体。所有这些都形成相同的形状,但材质不同。同样,JavaFX 允许您选择用来创建 3D 物体的材质类型。
Material of a 3D object is nothing but the type of texture used to create a 3D object. For example, a box in real world can be made of different materials; like a cardboard, a metal, wood, or just any other solid. All of these form the same shape but with a different material. Similarly, JavaFX allows you to choose the type of material used to create a 3D object.
Material Property
材质属性的类型为 Material ,用于选择 3D 形状材质表面。您可以使用以下方法设置 3D 形状的材质 setMaterial() −
The Material property is of the type Material and it is used to choose the surface of the material of a 3D shape. You can set the material of a 3D shape using the method setMaterial() as follows −
cylinder.setMaterial(material);
如上述此方法所述,您需要传递类型为材料的对象。包 javafx.scene.paint 的 PhongMaterial 类是此类的子类,并提供 7 个表示 Phong 着色材质的属性。您可以使用这些属性的 setter 方法将所有这些类型的材质应用于 3D 形状的表面。
As mentioned above for this method, you need to pass an object of the type Material. The PhongMaterial class of the package javafx.scene.paint is a sub class of this class and provides 7 properties that represent a Phong shaded material. You can apply all these type of materials to the surface of a 3D shape using the setter methods of these properties.
以下是 JavaFX 中提供的材质类型 −
Following are the type of materials that are available in JavaFX −
-
bumpMap − This represents a normal map stored as a RGB Image.
-
diffuseMap − This represents a diffuse map.
-
selfIlluminationMap − This represents a self-illumination map of this PhongMaterial.
-
specularMap − This represents a specular map of this PhongMaterial.
-
diffuseColor − This represents a diffuse color of this PhongMaterial.
-
specularColor − This represents a specular color of this PhongMaterial.
-
specularPower − This represents a specular power of this PhongMaterial.
默认情况下,3D 形状的材质是漫射颜色为浅灰色的 PhongMaterial。
By default, the material of a 3-Dimensional shape is a PhongMaterial with a diffuse color of light gray.
Example
以下是一个示例,它显示圆柱上各种材质。将此代码保存在名为 CylinderMaterials.java 的文件中。
Following is an example which displays various materials on the cylinder. Save this code in a file with the name CylinderMaterials.java.
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.PerspectiveCamera;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.paint.Color;
import javafx.scene.paint.PhongMaterial;
import javafx.scene.shape.Cylinder;
import javafx.stage.Stage;
public class CylinderMaterials extends Application {
@Override
public void start(Stage stage) {
//Drawing Cylinder1
Cylinder cylinder1 = new Cylinder();
//Setting the properties of the Cylinder
cylinder1.setHeight(130.0f);
cylinder1.setRadius(30.0f);
//Setting the position of the Cylinder
cylinder1.setTranslateX(100);
cylinder1.setTranslateY(75);
//Preparing the phong material of type bump map
PhongMaterial material1 = new PhongMaterial();
material1.setBumpMap(new Image
("http://www.tutorialspoint.com/images/tplogo.gif"));
//Setting the bump map material to Cylinder1
cylinder1.setMaterial(material1);
//Drawing Cylinder2
Cylinder cylinder2 = new Cylinder();
//Setting the properties of the Cylinder
cylinder2.setHeight(130.0f);
cylinder2.setRadius(30.0f);
//Setting the position of the Cylinder
cylinder2.setTranslateX(200);
cylinder2.setTranslateY(75);
//Preparing the phong material of type diffuse map
PhongMaterial material2 = new PhongMaterial();
material2.setDiffuseMap(new Image
("http://www.tutorialspoint.com/images/tp-logo.gif"));
//Setting the diffuse map material to Cylinder2
cylinder2.setMaterial(material2);
//Drawing Cylinder3
Cylinder cylinder3 = new Cylinder();
//Setting the properties of the Cylinder
cylinder3.setHeight(130.0f);
cylinder3.setRadius(30.0f);
//Setting the position of the Cylinder
cylinder3.setTranslateX(300);
cylinder3.setTranslateY(75);
//Preparing the phong material of type Self Illumination Map
PhongMaterial material3 = new PhongMaterial();
material3.setSelfIlluminationMap(new Image
("http://www.tutorialspoint.com/images/tp-logo.gif"));
//Setting the Self Illumination Map material to Cylinder3
cylinder3.setMaterial(material3);
//Drawing Cylinder4
Cylinder cylinder4 = new Cylinder();
//Setting the properties of the Cylinder
cylinder4.setHeight(130.0f);
cylinder4.setRadius(30.0f);
//Setting the position of the Cylinder
cylinder4.setTranslateX(400);
cylinder4.setTranslateY(75);
//Preparing the phong material of type Specular Map
PhongMaterial material4 = new PhongMaterial();
material4.setSpecularMap(new Image
("http://www.tutorialspoint.com/images/tp-logo.gif"));
//Setting the Specular Map material to Cylinder4
cylinder4.setMaterial(material4);
//Drawing Cylinder5
Cylinder cylinder5 = new Cylinder();
//Setting the properties of the Cylinder
cylinder5.setHeight(130.0f);
cylinder5.setRadius(30.0f);
//Setting the position of the Cylinder
cylinder5.setTranslateX(100);
cylinder5.setTranslateY(300);
//Preparing the phong material of type diffuse color
PhongMaterial material5 = new PhongMaterial();
material5.setDiffuseColor(Color.BLANCHEDALMOND);
//Setting the diffuse color material to Cylinder5
cylinder5.setMaterial(material5);
//Drawing Cylinder6
Cylinder cylinder6 = new Cylinder();
//Setting the properties of the Cylinder
cylinder6.setHeight(130.0f);
cylinder6.setRadius(30.0f);
//Setting the position of the Cylinder
cylinder6.setTranslateX(200);
cylinder6.setTranslateY(300);
//Preparing the phong material of type specular color
PhongMaterial material6 = new PhongMaterial();
//setting the specular color map to the material
material6.setSpecularColor(Color.BLANCHEDALMOND);
//Setting the specular color material to Cylinder6
cylinder6.setMaterial(material6);
//Drawing Cylinder7
Cylinder cylinder7 = new Cylinder();
//Setting the properties of the Cylinder
cylinder7.setHeight(130.0f);
cylinder7.setRadius(30.0f);
//Setting the position of the Cylinder
cylinder7.setTranslateX(300);
cylinder7.setTranslateY(300);
//Preparing the phong material of type Specular Power
PhongMaterial material7 = new PhongMaterial();
material7.setSpecularPower(0.1);
//Setting the Specular Power material to the Cylinder
cylinder7.setMaterial(material7);
//Creating a Group object
Group root = new Group(cylinder1 ,cylinder2, cylinder3,
cylinder4, cylinder5, cylinder6, cylinder7);
//Creating a scene object
Scene scene = new Scene(root, 600, 400);
//Setting camera
PerspectiveCamera camera = new PerspectiveCamera(false);
camera.setTranslateX(0);
camera.setTranslateY(0);
camera.setTranslateZ(-10);
scene.setCamera(camera);
//Setting title to the Stage
stage.setTitle("Drawing a cylinder");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls CylinderMaterials.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls CylinderMaterials
在执行时,上述程序将生成一个 JavaFX 窗口,其中显示 7 个带有材质、凹凸贴图、漫反射贴图、自发光贴图、镜面贴图、漫反射颜色、镜面颜色、(杏仁白)镜面强度,分别如以下屏幕截图所示 −
On executing, the above program generates a JavaFX window displaying 7 cylinders with Materials, Bump Map, Diffuse Map, Self-Illumination Map, Specular Map, Diffuse Color, Specular Color, (BLANCHEDALMOND) Specular Power, respectively, as shown in the following screenshot −
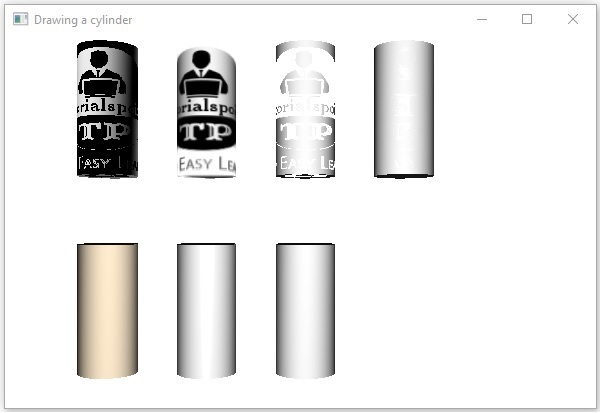