Spring Boot 简明教程
Spring Boot - Admin Server
使用 Spring Boot Actuator 端点监控您的应用程序有点困难。这是因为,如果您有“n”个应用程序,则每个应用程序都有单独的执行器端点,从而使监控变得困难。Spring Boot Admin Server 是一款用于管理和监控您的微服务应用程序的应用程序。
Monitoring your application by using Spring Boot Actuator Endpoint is slightly difficult. Because, if you have ‘n’ number of applications, every application has separate actuator endpoints, thus making monitoring difficult. Spring Boot Admin Server is an application used to manage and monitor your Microservice application.
为了处理这种情况,CodeCentric Team 提供了一个 Spring Boot Admin UI 来管理和监控所有 Spring Boot 应用程序执行器端点,并集中在一个地方。
To handle such situations, CodeCentric Team provides a Spring Boot Admin UI to manage and monitor all your Spring Boot application Actuator endpoints at one place.
对于构建 Spring Boot Admin Server,我们需要在构建配置文件中添加以下依赖项。
For building a Spring Boot Admin Server we need to add the below dependencies in your build configuration file.
Maven 用户可以在其 pom.xml 文件中添加以下依赖项 −
Maven users can add the below dependencies in your pom.xml file −
<dependency>
<groupId>de.codecentric</groupId>
<artifactId>spring-boot-admin-server</artifactId>
<version>1.5.5</version>
</dependency>
<dependency>
<groupId>de.codecentric</groupId>
<artifactId>spring-boot-admin-server-ui</artifactId>
<version>1.5.5</version>
</dependency>
Gradle 用户可以在其 build.gradle 文件中添加以下依赖项 −
Gradle users can add the below dependencies in your build.gradle file −
compile group: 'de.codecentric', name: 'spring-boot-admin-server', version: '1.5.5'
compile group: 'de.codecentric', name: 'spring-boot-admin-server-ui', version: '1.5.5'
在您的 Spring Boot 主应用程序类文件中添加 @EnableAdminServer 注解。@EnableAdminServer 注解用于将您作为 Admin Server 来监控所有其他微服务。
Add the @EnableAdminServer annotation in your main Spring Boot application class file. The @EnableAdminServer annotation is used to make your as Admin Server to monitor all other microservices.
package com.tutorialspoint.adminserver;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import de.codecentric.boot.admin.config.EnableAdminServer;
@SpringBootApplication
@EnableAdminServer
public class AdminserverApplication {
public static void main(String[] args) {
SpringApplication.run(AdminserverApplication.class, args);
}
}
现在,在 application.properties 文件中定义 server.port 和应用程序名称,如下所示 −
Now, define the server.port and application name in application.properties file a shown −
server.port = 9090
spring.application.name = adminserver
对于 YAML 用户,使用下列属性来在 application.yml 文件中定义端口号和应用程序名。
For YAML users, use the following properties to define the port number and application name in application.yml file.
server:
port: 9090
spring:
application:
name: adminserver
构建配置文件如下。
The build configuration file is given below.
For Maven users – pom.xml
For Maven users – pom.xml
<?xml version = "1.0" encoding = "UTF-8"?>
<project xmlns = "http://maven.apache.org/POM/4.0.0"
xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation = "http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.tutorialspoint</groupId>
<artifactId>adminserver</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>adminserver</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.9.RELEASE</version>
<relativePath /> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>de.codecentric</groupId>
<artifactId>spring-boot-admin-server</artifactId>
<version>1.5.5</version>
</dependency>
<dependency>
<groupId>de.codecentric</groupId>
<artifactId>spring-boot-admin-server-ui</artifactId>
<version>1.5.5</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
For Gradle users – build.gradle file
For Gradle users – build.gradle file
buildscript {
ext {
springBootVersion = '1.5.9.RELEASE'
}
repositories {
mavenCentral()
}
dependencies {
classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}")
}
}
apply plugin: 'java'
apply plugin: 'eclipse'
apply plugin: 'org.springframework.boot'
group = 'com.tutorialspoint'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
dependencies {
compile('org.springframework.boot:spring-boot-starter')
compile group: 'de.codecentric', name: 'spring-boot-admin-server', version: '1.5.5'
compile group: 'de.codecentric', name: 'spring-boot-admin-server-ui', version: '1.5.5'
testCompile('org.springframework.boot:spring-boot-starter-test')
}
您可以创建一个可执行 JAR 文件并使用以下 Maven 或 Gradle 命令运行 Spring Boot 应用程序 -
You can create an executable JAR file, and run the Spring Boot application by using the following Maven or Gradle commands −
对于 Maven,使用此处所示命令 −
For Maven, use the command shown here −
mvn clean install
在“构建成功”后,您可以在 target 目录下找到 JAR 文件。
After “BUILD SUCCESS”, you can find the JAR file under target directory.
对于 Gradle,使用此处所示命令 −
For Gradle, use the command shown here −
gradle clean build
在 “BUILD SUCCESSFUL” 后,你可以在 build/libs 目录下找到 JAR 文件。
After “BUILD SUCCESSFUL”, you can find the JAR file under build/libs directory.
现在,运行 JAR 文件,使用如下所示命令 −
Now, run the JAR file by using the command given below −
java –jar <JARFILE>
现在,应用程序已在 Tomcat 端口 9090 上启动,如下所示 −
Now, the application has started on the Tomcat port 9090 as shown here −

现在从 Web 浏览器访问以下 URL,查看 Admin Server UI。
Now hit the below URL from your web browser and see the Admin Server UI.
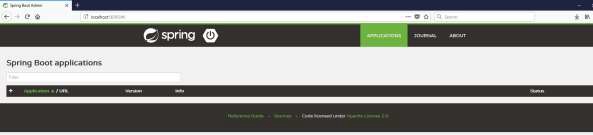