Spring Boot 简明教程
Spring Boot - Sending Email
通过使用 Spring Boot RESTful Web 服务,您可以通过 Gmail 传输层安全性发送电子邮件。在本章中,让我们详细了解如何使用此特性。
By using Spring Boot RESTful web service, you can send an email with Gmail Transport Layer Security. In this chapter, let us understand in detail how to use this feature.
首先,我们需要在 build 配置文件中添加 Spring Boot Starter Mail 依赖项。
First, we need to add the Spring Boot Starter Mail dependency in your build configuration file.
Maven 用户可以将下面的依赖项添加到 pom.xml 文件中。
Maven users can add the following dependency into the pom.xml file.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
Gradle 用户可以在 build.gradle 文件中添加以下依赖项。
Gradle users can add the following dependency in your build.gradle file.
compile('org.springframework.boot:spring-boot-starter-mail')
主要 Spring Boot 应用程序类文件代码如下所示:
The code of main Spring Boot application class file is given below −
package com.tutorialspoint.emailapp;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class EmailappApplication {
public static void main(String[] args) {
SpringApplication.run(EmailappApplication.class, args);
}
}
您可以编写一个简单的 Rest API,如下所示在 Rest 控制器类文件中发送电子邮件。
You can write a simple Rest API to send to email in Rest Controller class file as shown.
package com.tutorialspoint.emailapp;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class EmailController {
@RequestMapping(value = "/sendemail")
public String sendEmail() {
return "Email sent successfully";
}
}
您可以编写一个方法以便使用附件发送电子邮件。定义 mail.smtp 属性并使用 PasswordAuthentication。
You can write a method to send the email with Attachment. Define the mail.smtp properties and used PasswordAuthentication.
private void sendmail() throws AddressException, MessagingException, IOException {
Properties props = new Properties();
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", "smtp.gmail.com");
props.put("mail.smtp.port", "587");
Session session = Session.getInstance(props, new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("tutorialspoint@gmail.com", "<your password>");
}
});
Message msg = new MimeMessage(session);
msg.setFrom(new InternetAddress("tutorialspoint@gmail.com", false));
msg.setRecipients(Message.RecipientType.TO, InternetAddress.parse("tutorialspoint@gmail.com"));
msg.setSubject("Tutorials point email");
msg.setContent("Tutorials point email", "text/html");
msg.setSentDate(new Date());
MimeBodyPart messageBodyPart = new MimeBodyPart();
messageBodyPart.setContent("Tutorials point email", "text/html");
Multipart multipart = new MimeMultipart();
multipart.addBodyPart(messageBodyPart);
MimeBodyPart attachPart = new MimeBodyPart();
attachPart.attachFile("/var/tmp/image19.png");
multipart.addBodyPart(attachPart);
msg.setContent(multipart);
Transport.send(msg);
}
现在,从 Rest API 调用上述 sendmail() 方法,如下所示:
Now, call the above sendmail() method from the Rest API as shown −
@RequestMapping(value = "/sendemail")
public String sendEmail() throws AddressException, MessagingException, IOException {
sendmail();
return "Email sent successfully";
}
Note - 在发送电子邮件之前,请打开 Gmail 帐户设置中允许不安全的应用程序。
Note − Please switch ON allow less secure apps in your Gmail account settings before sending an email.
完整的构建配置文件如下所示。
The complete build configuration file is given below.
Maven – pom.xml
Maven – pom.xml
<?xml version = "1.0" encoding = "UTF-8"?>
<project xmlns = "http://maven.apache.org/POM/4.0.0"
xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation = "http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.tutorialspoint</groupId>
<artifactId>emailapp</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>emailapp</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.9.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Gradle – build.gradle
Gradle – build.gradle
buildscript {
ext {
springBootVersion = '1.5.9.RELEASE'
}
repositories {
mavenCentral()
}
dependencies {
classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}")
}
}
apply plugin: 'java'
apply plugin: 'eclipse'
apply plugin: 'org.springframework.boot'
group = 'com.tutorialspoint'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
dependencies {
compile('org.springframework.boot:spring-boot-starter-web')
compile('org.springframework.boot:spring-boot-starter-mail')
testCompile('org.springframework.boot:spring-boot-starter-test')
}
现在,您可以创建一个可执行 JAR 文件,并使用下面所示的 Maven 或 Gradle 命令运行 Spring Boot 应用程序 −
Now, you can create an executable JAR file, and run the Spring Boot application by using the Maven or Gradle commands shown below −
对于 Maven,您可以使用如下命令:−
For Maven, you can use the command as shown −
mvn clean install
“BUILD SUCCESS”之后,您可以在目标目录中找到 JAR 文件。
After “BUILD SUCCESS”, you can find the JAR file under the target directory.
对于 Gradle,您可以使用如下命令:−
For Gradle, you can use the command as shown −
gradle clean build
“BUILD SUCCESSFUL”之后,您可以在 build/libs 目录中找到 JAR 文件。
After “BUILD SUCCESSFUL”, you can find the JAR file under the build/libs directory.
现在,运行 JAR 文件,使用如下所示命令 −
Now, run the JAR file by using the command given below −
java –jar <JARFILE>
你可以看到应用程序已在 Tomcat 端口 8080 上启动。
You can see that the application has started on the Tomcat port 8080.

现在,从您的 Web 浏览器访问以下 URL,您将收到一封电子邮件。
Now hit the following URL from your web browser and you will receive an email.
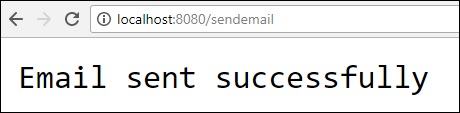
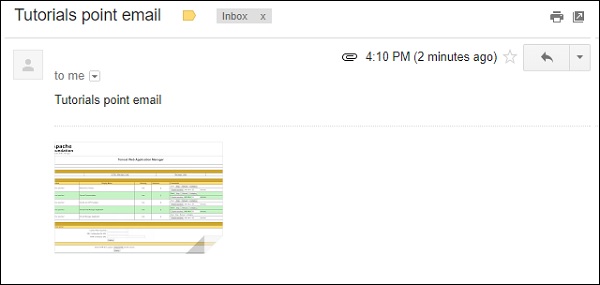