Python Pyramid 简明教程
Python Pyramid - Static Assets
通常需要在模板响应中包含一些即使存在某些动态数据也不会更改的资源。此类资源称为静态资产。媒体文件(.png、.jpg 等)、用于执行某些前端代码的 JavaScript 文件或用于格式化 HTML(.css 文件)的样式表是静态文件示例。
Pyramid 从服务器文件系统中的指定目录向客户端浏览器提供这些静态资产。Configurator 对象的 add_static_view() 方法定义了包含图像、JavaScript 和 CSS 文件等静态文件的文件夹的路由和路径名称。
根据约定,“static”目录用于存储静态资产,而 add_static_view() 的使用方法如下:
config.add_static_view(name='static', path='static')
定义静态路由后,可以使用 request.static_url() 方法在 HTML 脚本中使用静态资产的路径。
Static Image
在以下示例中,Pyramid 徽标将在 logo.html 模板中呈现。因此,首先将 "pyramid.png" 文件放在 static 文件夹中。现在可以将它用作 HTML 代码中 <img> 标记的 src 属性。
<html>
<body>
<h1>Hello, {{ name }}. Welcome to Pyramid</h1>
<img src="{{request.static_url('app:static/pyramid.png')}}">
</body>
</html>
Example
应用程序代码使用 add_static_view() 更新配置器并定义 index() 视图呈现上述模板。
from wsgiref.simple_server import make_server
from pyramid.config import Configurator
from pyramid.response import Response
from pyramid.view import view_config
@view_config(route_name='index', renderer='templates/logo.html')
def index(request):
return {'name':request.matchdict['name']}
if __name__ == '__main__':
with Configurator() as config:
config.include('pyramid_jinja2')
config.add_jinja2_renderer(".html")
config.add_route('index', '/{name}')
config.add_static_view(name='static', path='app:static')
config.scan()
app = config.make_wsgi_app()
server = make_server('0.0.0.0', 6543, app)
server.serve_forever()
Output
运行上述代码以启动服务器。在浏览器中使用 http://localhost:6543/Guest 作为 URL。在此,“Guest”是 matchdict 对象中的视图函数选取的路径参数,并作为上下文传递给 logo.html 模板。现在浏览器显示 Pyramid 徽标。
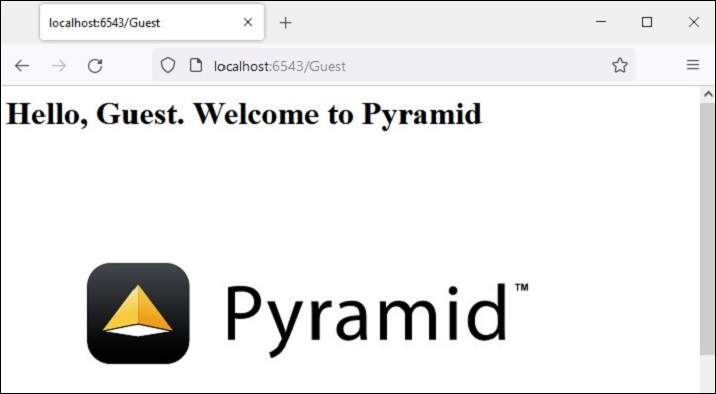
Javascript as Static Asset
以下是静态文件的另一个示例。JavaScript 代码 hello.js 包含 myfunction() 定义,将在 HTML 脚本 (templates\hello.html) 中的 onload 事件中执行
<html>
<head>
<script src="{{request.static_url('app:static/hello.js')}}"></script>
</head>
<body onload="myFunction()">
<div id="time" style="text-align:right; width="100%"></div>
<h1><div id="ttl">{{ name }}</div></h1>
</body>
</html>
Example
存储在 static 文件夹中的 hello.js 代码如下:
function myFunction() {
var today = new Date();
var h = today.getHours();
var m = today.getMinutes();
var s = today.getSeconds();
var msg="";
if (h<12)
{
msg="Good Morning, ";
}
if (h>=12 && h<18)
{
msg="Good Afternoon, ";
}
if (h>=18)
{
msg="Good Evening, ";
}
var x=document.getElementById('ttl').innerHTML;
document.getElementById('ttl').innerHTML = msg+x;
document.getElementById('time').innerHTML = h + ":" + m + ":" + s;
}