Wpf 简明教程
WPF - Triggers
触发器基本上使您可以根据属性的值更改属性值或执行操作。因此,它允许您动态更改控件的外观和/或行为,而无需创建新控件。
A trigger basically enables you to change property values or take actions based on the value of a property. So, it allows you to dynamically change the appearance and/or behavior of your control without having to create a new one.
触发器用于在满足某些条件时更改任何给定属性的值。触发器通常在样式中或文档根部中定义,并应用于该特定控件。有三种类型的触发器:
Triggers are used to change the value of any given property, when certain conditions are satisfied. Triggers are usually defined in a style or in the root of a document which are applied to that specific control. There are three types of triggers −
-
Property Triggers
-
Data Triggers
-
Event Triggers
Property Triggers
在属性触发器中,当一个属性发生变化时,它将立即或以动画形式更改另一个属性。例如,您可以使用属性触发器来更改按钮外观,当鼠标悬停在按钮上时。
In property triggers, when a change occurs in one property, it will bring either an immediate or an animated change in another property. For example, you can use a property trigger to change the appearance of a button when the mouse hovers over the button.
以下示例代码展示了如何在鼠标悬停在按钮上时更改按钮的前景色。
The following example code shows how to change the foreground color of a button when mouse hovers over the button.
<Window x:Class = "WPFPropertyTriggers.MainWindow"
xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
Title = "MainWindow" Height = "350" Width = "604">
<Window.Resources>
<Style x:Key = "TriggerStyle" TargetType = "Button">
<Setter Property = "Foreground" Value = "Blue" />
<Style.Triggers>
<Trigger Property = "IsMouseOver" Value = "True">
<Setter Property = "Foreground" Value = "Green" />
</Trigger>
</Style.Triggers>
</Style>
</Window.Resources>
<Grid>
<Button Width = "100" Height = "70"
Style = "{StaticResource TriggerStyle}" Content = "Trigger"/>
</Grid>
</Window>
当你编译并执行以上代码时,它将产生以下窗口:
When you compile and execute the above code, it will produce the following window −

当鼠标悬停在按钮上时,其前景色将变为绿色。
When the mouse hovers over the button, its foreground color will change to green.

Data Triggers
数据触发器在绑定数据满足某些条件时执行某些操作。让我们来看一下以下 XAML 代码,其中创建了一个具有某些属性的复选框和一个文本块。当选中复选框时,它会将其前景色变为红色。
A data trigger performs some actions when the bound data satisfies some conditions. Let’s have a look at the following XAML code in which a checkbox and a text block are created with some properties. When the checkbox is checked, it will change its foreground color to red.
<Window x:Class = "WPFDataTrigger.MainWindow"
xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
Title = "Data Trigger" Height = "350" Width = "604">
<StackPanel HorizontalAlignment = "Center">
<CheckBox x:Name = "redColorCheckBox"
Content = "Set red as foreground color" Margin = "20"/>
<TextBlock Name = "txtblock" VerticalAlignment = "Center"
Text = "Event Trigger" FontSize = "24" Margin = "20">
<TextBlock.Style>
<Style>
<Style.Triggers>
<DataTrigger Binding = "{Binding ElementName = redColorCheckBox, Path = IsChecked}"
Value = "true">
<Setter Property = "TextBlock.Foreground" Value = "Red"/>
<Setter Property = "TextBlock.Cursor" Value = "Hand" />
</DataTrigger>
</Style.Triggers>
</Style>
</TextBlock.Style>
</TextBlock>
</StackPanel>
</Window>
编译并执行上述代码时,将产生以下输出 -
When the above code is compiled and executed, it will produce the following output −

当您勾选复选框时,文本块将将其前景色更改为红色。
When you tick the checkbox, the text block will change its foreground color to red.
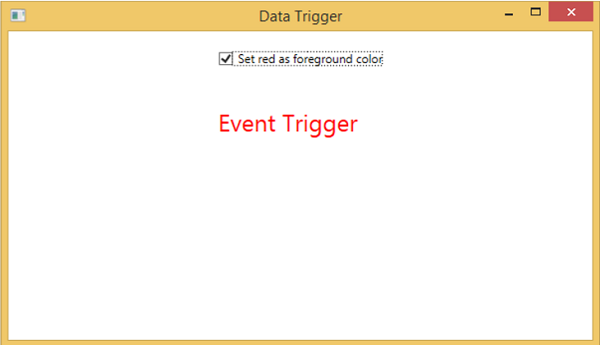
Event Triggers
事件触发器在触发特定事件时执行某些操作。它通常用于在控件上完成某些动画,例如 DoubleAnumatio、ColorAnimation 等。在以下示例中,我们将创建一个简单的按钮。当触发单击事件时,它将扩展按钮的宽度和高度。
An event trigger performs some actions when a specific event is fired. It is usually used to accomplish some animation on control such DoubleAnumatio, ColorAnimation, etc. In the following example, we will create a simple button. When the click event is fired, it will expand the button width and height.
<Window x:Class = "WPFEventTrigger.MainWindow"
xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
Title = "MainWindow" Height = "350" Width = "604">
<Grid>
<Button Content = "Click Me" Width = "60" Height = "30">
<Button.Triggers>
<EventTrigger RoutedEvent = "Button.Click">
<EventTrigger.Actions>
<BeginStoryboard>
<Storyboard>
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty =
"Width" Duration = "0:0:4">
<LinearDoubleKeyFrame Value = "60" KeyTime = "0:0:0"/>
<LinearDoubleKeyFrame Value = "120" KeyTime = "0:0:1"/>
<LinearDoubleKeyFrame Value = "200" KeyTime = "0:0:2"/>
<LinearDoubleKeyFrame Value = "300" KeyTime = "0:0:3"/>
</DoubleAnimationUsingKeyFrames>
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty = "Height"
Duration = "0:0:4">
<LinearDoubleKeyFrame Value = "30" KeyTime = "0:0:0"/>
<LinearDoubleKeyFrame Value = "40" KeyTime = "0:0:1"/>
<LinearDoubleKeyFrame Value = "80" KeyTime = "0:0:2"/>
<LinearDoubleKeyFrame Value = "150" KeyTime = "0:0:3"/>
</DoubleAnimationUsingKeyFrames>
</Storyboard>
</BeginStoryboard>
</EventTrigger.Actions>
</EventTrigger>
</Button.Triggers>
</Button>
</Grid>
</Window>
当你编译并执行以上代码时,它将产生以下窗口:
When you compile and execute the above code, it will produce the following window −
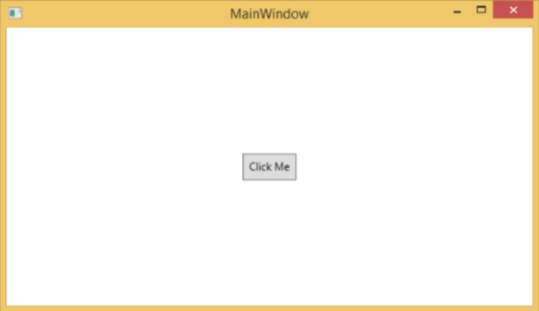
点击此按钮后,您将观察到它将开始按两个维度展开。
Upon clicking the button, you will observe that it will start expanding in both dimensions.
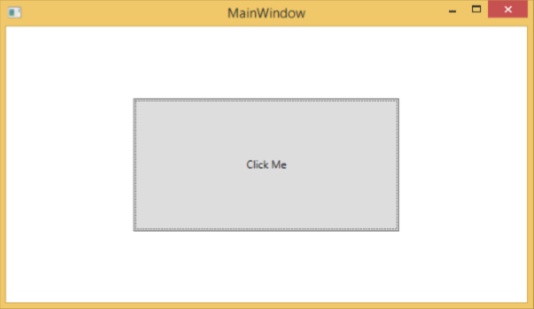
我们建议您编译并执行以上示例,并且将触发器应用于其他属性。
We recommend that you compile and execute the above examples and apply the triggers to other properties as well.