Xamarin 简明教程
Xamarin - Building the App GUI
TextView
TextView 是 Android 微件的一个非常重要的组件。它主要用于在 Android 屏幕上显示文本。
为了创建一个 textView,只需打开 main.axml ,并在 linear layout 标签之间添加以下代码。
<TextView
android:text = "Hello I am a text View"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/textview1" />
Button
按钮是一个控件,在点击时用于触发一个事件。在 Main.axml 文件下,键入以下代码以创建按钮。
<Button
android:id = "@+id/MyButton"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:text = "@string/Hello" />
打开 Resources\Values\Strings.xml ,并在 <resources> 标签之间键入以下代码。
<string name="Hello">Click Me!</string>
上述代码提供了我们创建的按钮的值。接下来,我们打开 MainActivity.cs 并创建在按钮点击时要执行的动作。在 base.OnCreate (bundle)方法下键入以下代码。
Button button = FindViewById<Button>(Resource.Id.MyButton);
button.Click += delegate { button.Text = "You clicked me"; };

当用户单击该按钮时,上述代码会显示“You Clicked Me”。
FindViewById<< -→ 该方法找到已识别的视图的 ID。它在 .axml 布局文件中搜索 id。
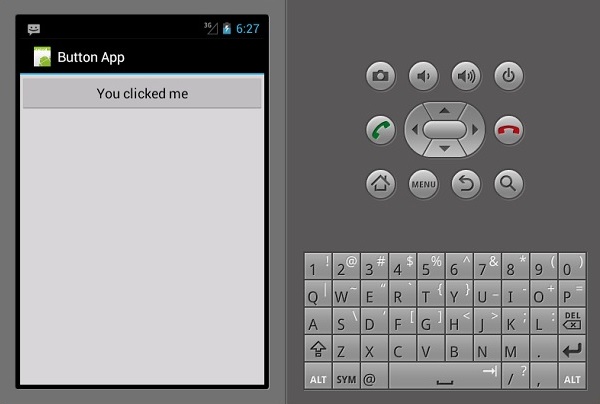
Checkbox
当希望从一组选项中选择多个选项时,使用复选框。在此示例中,我们将创建一个复选框,选中后,显示已选中,否则显示未选中。
首先,我们在项目中打开 Main.axml 文件,并键入以下代码行以创建一个复选框。
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:background = "#d3d3d3"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<CheckBox
android:text = "CheckBox"
android:padding = "25dp"
android:layout_width = "300dp"
android:layout_height = "wrap_content"
android:id = "@+id/checkBox1"
android:textColor = "@android:color/black"
android:background = "@android:color/holo_blue_dark" />
</LinearLayout>
下一步,转到 MainActivity.cs 来添加功能代码。
CheckBox checkMe = FindViewById<CheckBox>(Resource.Id.checkBox1);
checkMe.CheckedChange += (object sender, CompoundButton.CheckedChangeEventArgs e) => {
CheckBox check = (CheckBox)sender;
if(check.Checked) {
check.Text = "Checkbox has been checked";
} else {
check.Text = "Checkbox has not been checked";
}
};
从上述代码中,我们首先使用 findViewById 找到复选框。接下来,我们为复选框创建一个处理程序方法,并在处理程序中,我们创建一个 if else 语句,根据选择的结果显示消息。
CompoundButton.CheckedChangeEventArgs → 复选框的状态更改时,此方法将触发事件。

Progress Bar
进度条是一个用于显示操作进度的控件。要添加进度条,请在 Main.axml 文件中添加以下代码行。
<ProgressBar
style="?android:attr/progressBarStyleHorizontal"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/progressBar1" />
下一步,转到 MainActivity.cs 并设置进度条的值。
ProgressBar pb = FindViewById<ProgressBar>(Resource.Id.progressBar1);
pb.Progress = 35;
在上述代码中,我们创建了一个值为 35 的进度条。
Radio Buttons
这是一个 Android 小部件,允许人从一组选项中选择一个。在本部分中,我们将创建一个包含汽车列表的单选组,该组将获取选中的单选按钮。
首先,我们添加一个单选组和 textview ,如下面的代码所示 −
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:background = "@android:color/darker_gray"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<TextView
android:text = "What is your favourite Car"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/textView1"
android:textColor = "@android:color/black" />
<RadioGroup
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/radioGroup1"
android:backgroundTint = "#a52a2aff"
android:background = "@android:color/holo_green_dark">
<RadioButton
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:text = "Ferrari"
android:id = "@+id/radioFerrari" />
<RadioButton
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:text = "Mercedes"
android:id = "@+id/radioMercedes" />
<RadioButton
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:text = "Lamborghini"
android:id = "@+id/radioLamborghini" />
<RadioButton
android:text = "Audi"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:id = "@+id/radioAudi" />
</RadioGroup>
</LinearLayout>
当单击单选按钮时,执行一个动作,我们添加一个活动。转到 MainActivity.cs 并创建一个新事件处理程序,如下所示。
private void onClickRadioButton(object sender, EventArgs e) {
RadioButton cars = (RadioButton)sender;
Toast.MakeText(this, cars.Text, ToastLength.Short).Show
();
}
Toast.MakeText() → 这是一个视图方法,用于在小弹出窗口中显示消息/输出。在 OnCreate() 方法底部的 SetContentView() 之后,添加以下代码片段。这将捕获每个单选按钮并将其添加到我们创建的事件处理程序中。
RadioButton radio_Ferrari = FindViewById<RadioButton>
(Resource.Id.radioFerrari);
RadioButton radio_Mercedes = FindViewById<RadioButton>
(Resource.Id.radioMercedes);
RadioButton radio_Lambo = FindViewById<RadioButton>
(Resource.Id.radioLamborghini);
RadioButton radio_Audi = FindViewById<RadioButton>
(Resource.Id.radioAudi);
radio_Ferrari.Click += onClickRadioButton;
radio_Mercedes.Click += onClickRadioButton;
radio_Lambo.Click += onClickRadioButton;
radio_Audi.Click += onClickRadioButton;
现在,运行你的应用程序。它应该显示以下屏幕作为输出 −

Toggle Buttons
切换按钮用于在两种状态之间切换,例如,它可以在开(ON)和关(OFF)之间切换。打开 Resources\layout\Main.axml 并添加以下代码行以创建一个切换按钮。
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:background = "#d3d3d3"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<ToggleButton
android:id = "@+id/togglebutton"
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:textOn = "Torch ON"
android:textOff = "Torch OFF"
android:textColor = "@android:color/black" />
</LinearLayout>
单击切换栏时,我们可以向其中添加操作。打开 MainActivity.cs ,并在 OnCreate() 方法类之后添加以下代码行。
ToggleButton togglebutton = FindViewById<ToggleButton> (Resource.Id.togglebutton);
togglebutton.Click += (o, e) => {
if (togglebutton.Checked)
Toast.MakeText(this, "Torch is ON", ToastLength.Short).Show ();
else
Toast.MakeText(this, "Torch is OFF",
ToastLength.Short).Show();
};
现在,当您运行 App 时,它应显示以下输出 −

Ratings Bar
等级栏是由星构成的窗体元素,应用程序用户可以使用这些星来评估您为他们提供的内容。在您的 Main.axml 文件中,使用 5 颗星创建一个新的等级栏。
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:background = "#d3d3d3"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<RatingBar
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:id = "@+id/ratingBar1"
android:numStars = "5"
android:stepSize = "1.0" />
</LinearLayout>
运行应用程序后,它应显示以下输出 −

Autocomplete Textview
这是一个文本视图,在用户输入时显示全部建议。我们要创建一个自动填充文本视图,其中包含一个人员姓名列表,以及一个单击后将显示所选名称的按钮。
打开 Main.axml 并编写以下代码。
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:layout_width = "fill_parent"
android:background = "#d3d3d3"
android:layout_height = "fill_parent">
<TextView
android:text = "Enter Name"
android:textAppearance = "?android:attr/textAppearanceMedium"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:id = "@+id/textView1"
android:padding = "5dp"
android:textColor = "@android:color/black" />
<AutoCompleteTextView
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:id = "@+id/autoComplete1"
android:textColor = "@android:color/black" />
<Button
android:text = "Submit"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:id = "@+id/btn_Submit"
android:background="@android:color/holo_green_dark" />
</LinearLayout>
以上的代码将生成一个用于输入的 TextView、用于显示建议的 AutoCompleteTextView 和一个用于显示从 TextView 输入的名称的按钮。转到 MainActivity.cs 以添加该功能。
创建如下所示的新事件处理程序方法。
protected void ClickedBtnSubmit(object sender, System.EventArgs e){
if (autoComplete1.Text != ""){
Toast.MakeText(this, "The Name Entered ="
+ autoComplete1.Text, ToastLength.Short).Show();
} else {
Toast.MakeText(this, "Enter a Name!", ToastLength.Short).Show();
}
}
创建的处理程序将检查自动填充文本视图是否为空。如果它不为空,则显示所选的自动填充文本。在 OnCreate() 类中键入以下代码。
autoComplete1 = FindViewById<AutoCompleteTextView>(Resource.Id.autoComplete1);
btn_Submit = FindViewById<Button>(Resource.Id.btn_Submit);
var names = new string[] { "John", "Peter", "Jane", "Britney" };
ArrayAdapter adapter = new ArrayAdapter<string>(this,
Android.Resource.Layout.SimpleSpinnerItem, names);
autoComplete1.Adapter = adapter;
btn_Submit.Click += ClickedBtnSubmit;
ArrayAdapter − 这是一个收集处理程序,它从列表收集中读取数据项并将其作为视图返回或显示在屏幕上。
现在,当您运行应用程序时,它应显示以下输出。
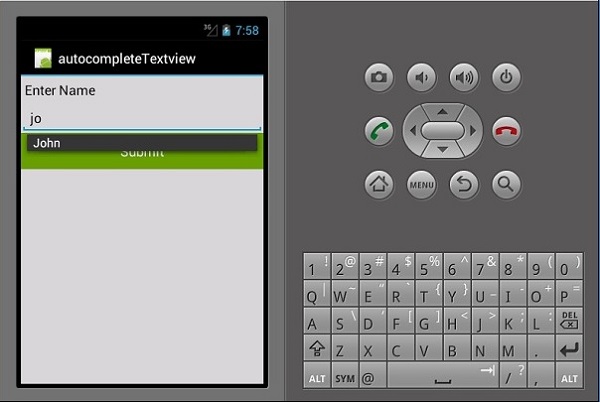