Xamarin 简明教程
Xamarin - Menus
Popup Menus
弹出菜单是指附属于视图的菜单;它也称为 shortcut menu 。让我们来看看如何向 Android 应用程序添加弹出菜单。
A popup menu refers to a menu that is attached to a view; it is also referred to as a shortcut menu. Let’s see how to add a popup menu to an Android App.
创建一个新项目并将其命名为 popUpMenu App 。打开 Main.axml 并创建一个按钮,该按钮将用于显示弹出菜单。
Create a new project and call it popUpMenu App. Open Main.axml and create a button which will be used to display the popup menu.
<?xml version = "1.0" encoding = "utf-8"?>
<LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
android:orientation = "vertical"
android:background = "#d3d3d3"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent">
<Button
android:id = "@+id/popupButton"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:text = "Show popup menu"
android:background = "@android:color/holo_green_dark"
android:textColor = "@android:color/black" />
</LinearLayout>
在 Resources 文件夹下创建一个新文件夹,并将其命名为 Menu 。在 Menu 文件夹内,添加一个名为 popMenu.xml 的新 xml 文件。
Create a new folder under the Resources folder and call it Menu. Inside the Menu folder, add a new xml file called popMenu.xml.
在 popMenu.xml 下,添加以下菜单项。
Under popMenu.xml, add the following menu items.
<?xml version = "1.0" encoding="utf-8"?>
<menu xmlns:android = "http://schemas.android.com/apk/res/android">
<item
android:id = "@+id/file_settings"
android:icon = "@drawable/img_settings"
android:title = "Settings"
android:showAsAction = "ifRoom">
<item
android:id = "@+id/new_game1"
android:icon = "@drawable/imgNew"
android:title = "New File Settings"/>
<item
android:id = "@+id/help"
android:icon = "@drawable/img_help"
android:title = "Help" />
<item
android:id = "@+id/about_app"
android:icon = "@drawable/img_help"
android:title = "About app"/>
</item>
</menu>
添加菜单项后,转到 mainActivity.cs 以在单击按钮时显示弹出菜单。
After adding the menu items, go to mainActivity.cs to display the popup menu on button click.
protected override void OnCreate(Bundle bundle) {
base.OnCreate(bundle);
SetContentView(Resource.Layout.Main);
Button showPopupMenu = FindViewById<Button>(Resource.Id.popupButton);
showPopupMenu.Click += (s, arg) => {
PopupMenu menu = new PopupMenu(this, showPopupMenu);
menu.Inflate(Resource.Menu.popMenu);
menu.Show();
};
}
现在,构建并运行您的应用程序。它应产生以下输出 −
Now, build and run your application. It should produce the following output −
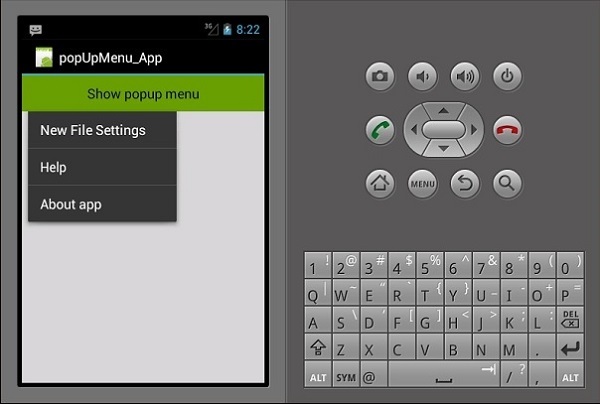
Options Menu
选项菜单是由 App 主要菜单组成的集合,主要用于存储设置、搜索等。在这里,我们将在设置菜单中创建三个项目,即 New File Settings, Help, and About App 。
Options Menu is a collection of menus that are primary to an App and are mainly used to store settings, search, etc. Here, we are going to create a menu for settings with three items inside, i.e., New File Settings, Help, and About App.
为创建一个选项菜单,我们必须在 resources 文件夹中创建一个新的 XML 布局文件。首先,我们将添加一个新的 XML 文件。鼠标右键单击 Layout folder ,然后转到 Add → New item → Visual C# → XML File 。
To create an options menu, we must create a new XML layout file in the resources folder. First of all, we will add a new XML file. Right-click on the Layout folder, then go to Add → New item → Visual C# → XML File.
为 layout file 选择一个合适的名字。在我们的示例中,我们将把我们的文件称为 myMenu.xml 。
Choose an appropriate name for the layout file. In our example, we will call our file myMenu.xml.
在 myMenu.xml 中,我们将创建一个新菜单并在其中添加项目。以下代码展示了如何执行此操作。
Inside myMenu.xml, we are going to create a new menu and add items inside. The following code shows how to do it.
<?xml version = "1.0" encoding = "utf-8"?>
<menu xmlns:android = "http://schemas.android.com/apk/res/android">
<item
android:id = "@+id/file_settings"
android:icon = "@drawable/img_settings"
android:title = "Settings"
android:showAsAction = "ifRoom">
<menu>
<item
android:id = "@+id/new_game1"
android:icon = "@drawable/imgNew"
android:title = "New File Settings" />
<item
android:id = "@+id/help"
android:icon = "@drawable/img_help"
android:title = "Help" />
<item
android:id = "@+id/about_app"
android:icon = "@drawable/img_help"
android:title = "About app"/>
</menu>
</item>
</menu>
接下来,我们将导航到 MainActivity.cs 并为 onOptionsMenu() 创建一个覆盖类。
Next, we navigate to MainActivity.cs and create an override class for onOptionsMenu().
public override bool OnCreateOptionsMenu(IMenu menu) {
MenuInflater.Inflate(Resource.Menu.myMenu, menu);
return base.OnPrepareOptionsMenu(menu);
}
接下来,我们创建一个操作以在选择 settings menu 时对其进行响应。为此,我们为 OnOptionsItemSelected() 菜单创建另一个覆盖类。
Next, we create an action to respond to the settings menu when it is selected. To do this, we create another override class for the OnOptionsItemSelected() menu.
public override bool OnOptionsItemSelected(IMenuItem item) {
if (item.ItemId == Resource.Id.file_settings) {
// do something here...
return true;
}
return base.OnOptionsItemSelected(item);
}
我们最终的完整代码如下所示:
Our final complete code will look as follows −
namespace optionsMenuApp {
[Activity(Label = "options Menu", MainLauncher = true, Icon = "@drawable/icon")]
public class MainActivity : Activity {
public override bool OnCreateOptionsMenu(IMenu menu) {
MenuInflater.Inflate(Resource.Menu.myMenu, menu);
return base.OnPrepareOptionsMenu(menu);
}
public override bool OnOptionsItemSelected(IMenuItem item) {
if (item.ItemId == Resource.Id.file_settings) {
// do something here...
return true;
}
return base.OnOptionsItemSelected(item);
}
}
}
现在,构建并运行您的应用程序。它应产生以下输出 −
Now, build and run your application. It should produce the following output −
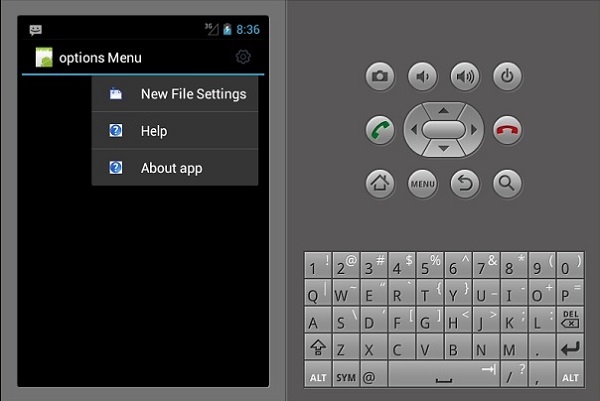