Chunk-oriented Processing
Spring Batch 在其最常见的实现中使用“分块导向”处理风格。分块导向处理指的是一次读取一个数据,并在事务边界内创建被写入的“块”。一旦读取的项目数量等于提交间隔,整个块就会被 ItemWriter
写出,然后事务就会被提交。以下图片显示了这一过程:
Spring Batch uses a “chunk-oriented” processing style in its most common
implementation. Chunk oriented processing refers to reading the data one at a time and
creating 'chunks' that are written out within a transaction boundary. Once the number of
items read equals the commit interval, the entire chunk is written out by the
ItemWriter
, and then the transaction is committed. The following image shows the
process:
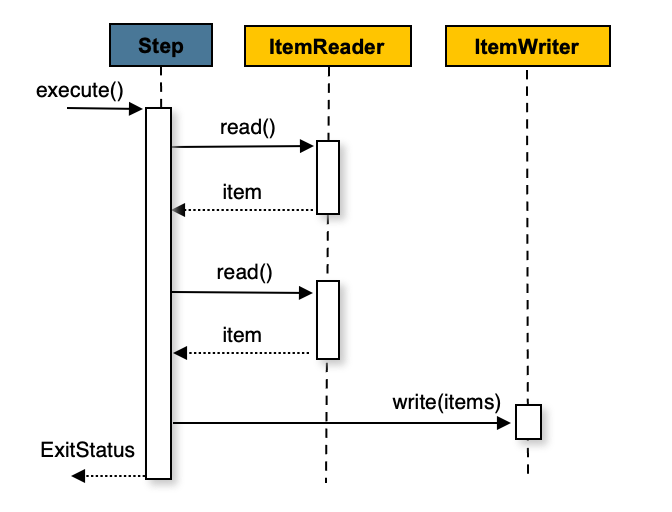
以下伪代码以简化形式展示了相同概念:
The following pseudo code shows the same concepts in a simplified form:
List items = new Arraylist();
for(int i = 0; i < commitInterval; i++){
Object item = itemReader.read();
if (item != null) {
items.add(item);
}
}
itemWriter.write(items);
您还可以使用可选的 ItemProcessor
配置面向区块的步骤,以便在将项目传递给 ItemWriter
之前处理项目。以下图像展示了在步骤中注册 ItemProcessor
时流程:
You can also configure a chunk-oriented step with an optional ItemProcessor
to process items before passing them to the ItemWriter
. The following image
shows the process when an ItemProcessor
is registered in the step:
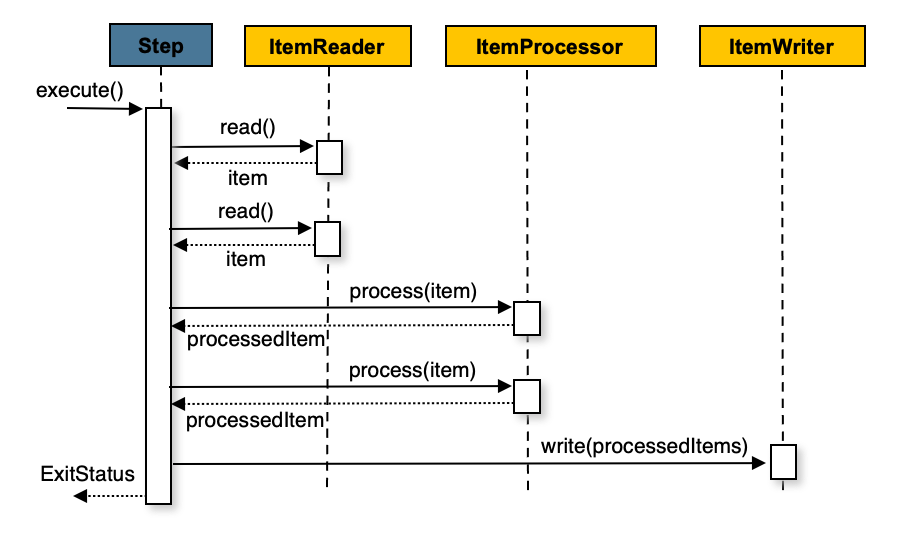
以下伪代码展示了如何以简化形式实现此代码:
The following pseudo code shows how this is implemented in a simplified form:
List items = new Arraylist();
for(int i = 0; i < commitInterval; i++){
Object item = itemReader.read();
if (item != null) {
items.add(item);
}
}
List processedItems = new Arraylist();
for(Object item: items){
Object processedItem = itemProcessor.process(item);
if (processedItem != null) {
processedItems.add(processedItem);
}
}
itemWriter.write(processedItems);
有关项目处理程序及其用例的更多详细信息,请参阅 Item processing 部分。
For more details about item processors and their use cases, see the Item processing section.