Design Pattern 简明教程
Design Patterns - Decorator Pattern
装饰器模式允许用户向现有对象添加新功能,而不改变其结构。该类型的设计模式属于结构模式,因为该模式充当现有类的包装。
Decorator pattern allows a user to add new functionality to an existing object without altering its structure. This type of design pattern comes under structural pattern as this pattern acts as a wrapper to existing class.
此模式创建一个装饰器类,它包装原始类并提供附加功能,保持类方法签名不变。
This pattern creates a decorator class which wraps the original class and provides additional functionality keeping class methods signature intact.
我们通过以下示例展示了装饰器模式的用法,其中我们将使用某种颜色装饰形状,而无需更改形状类。
We are demonstrating the use of decorator pattern via following example in which we will decorate a shape with some color without alter shape class.
Implementation
我们将创建实现形状接口的形状接口和具体类。然后我们将创建一个抽象装饰器类 ShapeDecorator 来实现形状接口并将形状对象作为其实例变量。
We’re going to create a Shape interface and concrete classes implementing the Shape interface. We will then create an abstract decorator class ShapeDecorator implementing the Shape interface and having Shape object as its instance variable.
RedShapeDecorator 是一个具体类,实现了 ShapeDecorator。
RedShapeDecorator is concrete class implementing ShapeDecorator.
DecoratorPatternDemo 是我们的演示类,它将使用 RedShapeDecorator 装饰 Shape 对象。
DecoratorPatternDemo, our demo class will use RedShapeDecorator to decorate Shape objects.
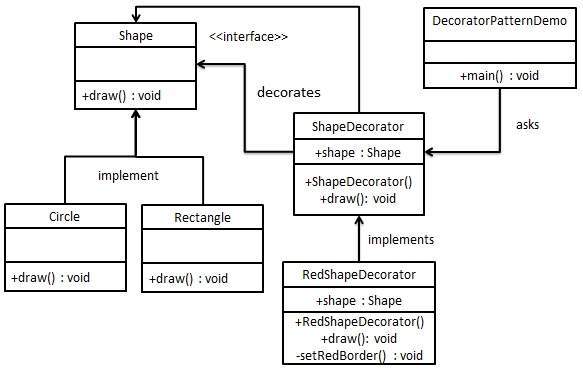
Step 2
创建实现相同接口的具体类。
Create concrete classes implementing the same interface.
Rectangle.java
public class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Shape: Rectangle");
}
}
Circle.java
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Shape: Circle");
}
}
Step 3
创建一个实现 Shape 接口的抽象装饰类。
Create abstract decorator class implementing the Shape interface.
ShapeDecorator.java
public abstract class ShapeDecorator implements Shape {
protected Shape decoratedShape;
public ShapeDecorator(Shape decoratedShape){
this.decoratedShape = decoratedShape;
}
public void draw(){
decoratedShape.draw();
}
}
Step 4
创建一个扩展 ShapeDecorator 类的具体装饰类。
Create concrete decorator class extending the ShapeDecorator class.
RedShapeDecorator.java
public class RedShapeDecorator extends ShapeDecorator {
public RedShapeDecorator(Shape decoratedShape) {
super(decoratedShape);
}
@Override
public void draw() {
decoratedShape.draw();
setRedBorder(decoratedShape);
}
private void setRedBorder(Shape decoratedShape){
System.out.println("Border Color: Red");
}
}
Step 5
使用 RedShapeDecorator 装饰 Shape 对象。
Use the RedShapeDecorator to decorate Shape objects.
DecoratorPatternDemo.java
public class DecoratorPatternDemo {
public static void main(String[] args) {
Shape circle = new Circle();
Shape redCircle = new RedShapeDecorator(new Circle());
Shape redRectangle = new RedShapeDecorator(new Rectangle());
System.out.println("Circle with normal border");
circle.draw();
System.out.println("\nCircle of red border");
redCircle.draw();
System.out.println("\nRectangle of red border");
redRectangle.draw();
}
}