Python 简明教程
Python - While Loops
Python while Loop
Python 编程语言中的 while 循环会一直执行一个目标语句,只要指定的 boolean expression 为真。此循环以 while 关键字开头,后跟布尔表达式和冒号符号 (:)。然后,一个由缩进语句块开头。
A while loop in Python programming language repeatedly executes a target statement as long as the specified boolean expression is true. This loop starts with while keyword followed by a boolean expression and colon symbol (:). Then, an indented block of statements starts.
此处,语句可以是单个语句,也可以是具有统一缩进的语句块。该条件可以是任何表达式,而真可以是任何非零值。一旦表达式变为假,程序控制将传递到紧接在循环之后的行。
Here, statement(s) may be a single statement or a block of statements with uniform indent. The condition may be any expression, and true is any non-zero value. As soon as the expression becomes false, the program control passes to the line immediately following the loop.
如果它未能变为假,循环就会继续运行,且除非强制停止,否则就不会停止。这样的循环被称为无限循环,这是计算机程序中不需要的。
If it fails to turn false, the loop continues to run, and doesn’t stop unless forcefully stopped. Such a loop is called infinite loop, which is undesired in a computer program.
Syntax of while Loop
Python 编程语言中 while 循环的语法为 −
The syntax of a while loop in Python programming language is −
while expression:
statement(s)
在 Python 中,编程结构后用相同数量字符空白缩进的所有语句都被视为单个代码块的一部分。Python 使用缩进作为其分组语句的方法。
In Python, all the statements indented by the same number of character spaces after a programming construct are considered to be part of a single block of code. Python uses indentation as its method of grouping statements.
Example 1
以下示例说明了 while 循环的工作原理。此处,迭代一直运行,直到计数的值变为 5。
The following example illustrates the working of while loop. Here, the iteration run till value of count will become 5.
count=0
while count<5:
count+=1
print ("Iteration no. {}".format(count))
print ("End of while loop")
执行此代码后,会生成以下输出 −
On executing, this code will produce the following output −
Iteration no. 1
Iteration no. 2
Iteration no. 3
Iteration no. 4
Iteration no. 5
End of while loop
Example 2
以下是使用 while 循环的另一个示例。对于每次迭代,程序都会询问 user input ,并一直重复,直到用户输入非数字字符串。isnumeric() 函数在输入为整数时返回真,否则返回假。
Here is another example of using the while loop. For each iteration, the program asks for user input and keeps repeating till the user inputs a non-numeric string. The isnumeric() function returns true if input is an integer, false otherwise.
var = '0'
while var.isnumeric() == True:
var = "test"
if var.isnumeric() == True:
print ("Your input", var)
print ("End of while loop")
运行代码后,会生成以下输出 −
On running the code, it will produce the following output −
enter a number..10
Your input 10
enter a number..100
Your input 100
enter a number..543
Your input 543
enter a number..qwer
End of while loop
Python Infinite while Loop
如果条件从不变为假,那么循环就会变成无限循环。使用 while 循环时,您必须小心,因为存在该条件从不解析为假值的可能性。这会导致循环从不结束。这样的循环被称为无限循环。
A loop becomes infinite loop if a condition never becomes FALSE. You must be cautious when using while loops because of the possibility that this condition never resolves to a FALSE value. This results in a loop that never ends. Such a loop is called an infinite loop.
在客户端/服务器编程中,无限循环可能是非常有用的,其中服务器需要持续运行,以便客户端程序可以视需要与之通信。
An infinite loop might be useful in client/server programming where the server needs to run continuously so that client programs can communicate with it as and when required.
Example
我们举个示例来了解无限循环在 Python 中的工作原理 -
Let’s take an example to understand how the infinite loop works in Python −
var = 1
while var == 1 : # This constructs an infinite loop
num = int(input("Enter a number :"))
print ("You entered: ", num)
print ("Good bye!")
执行此代码后,会生成以下输出 −
On executing, this code will produce the following output −
Enter a number :20
You entered: 20
Enter a number :29
You entered: 29
Enter a number :3
You entered: 3
Enter a number :11
You entered: 11
Enter a number :22
You entered: 22
Enter a number :Traceback (most recent call last):
File "examples\test.py", line 5, in
num = int(input("Enter a number :"))
KeyboardInterrupt
Python while-else Loop
Python 支持具有与 while 循环关联的 else 语句。如果 else 语句与 while 循环一起使用,则在控制转移到主执行行之前,当条件变为假时,将执行 else 语句。
Python supports having an else statement associated with a while loop. If the else statement is used with a while loop, the else statement is executed when the condition becomes false before the control shifts to the main line of execution.
Flowchart of While loop with else Statement
以下流程图显示了如何将 else 语句与 while 循环一起使用 −
The following flow diagram shows how to use else statement with while loop −
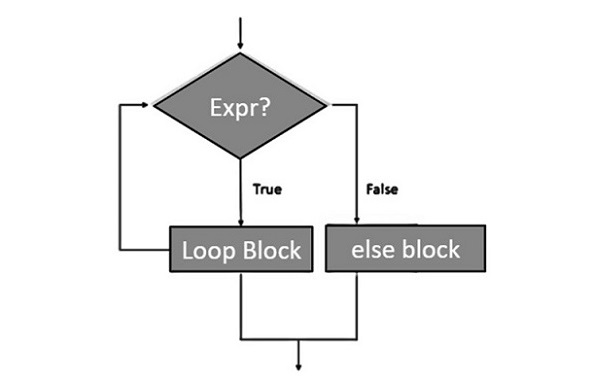
Example
以下示例展示了 else 语句与 while 语句的组合。在 count 小于 5 时,会打印迭代计数。当其变为 5 时,会在 else 块中执行 print 语句,然后才会将控制权传递到主程序中的下一条语句。
The following example illustrates the combination of an else statement with a while statement. Till the count is less than 5, the iteration count is printed. As it becomes 5, the print statement in else block is executed, before the control is passed to the next statement in the main program.
count=0
while count<5:
count+=1
print ("Iteration no. {}".format(count))
else:
print ("While loop over. Now in else block")
print ("End of while loop")
运行以上代码后,将打印以下输出 −
On running the above code, it will print the following output −
Iteration no. 1
Iteration no. 2
Iteration no. 3
Iteration no. 4
Iteration no. 5
While loop over. Now in else block
End of while loop
Single Statement Suites
与 if 语句语法类似,如果你的 while 子句仅包含一个语句,则它可以放在与 while 头相同的一行上。
Similar to the if statement syntax, if your while clause consists only of a single statement, it may be placed on the same line as the while header.
Example
以下示例显示了如何使用单行 while 子句。
The following example shows how to use one-line while clause.
flag = 0
while (flag): print ("Given flag is really true!")
print ("Good bye!")
当你运行此代码时,它将显示以下输出 −
When you run this code, it will display the following output −
Good bye!
将标志值更改为“1”,尝试上述程序。如果你这样做,它就会进入无限循环,你需要按 CTRL+C 键退出。
Change the flag value to "1" and try the above program. If you do so, it goes into infinite loop and you need to press CTRL+C keys to exit.